Java File Handling Techniques: Key to Efficient Homework
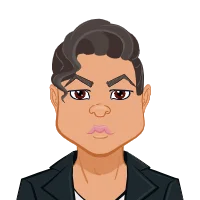
Welcome to our blog on "Java File Handling Techniques: Key to Efficient Homework." Java, being a versatile programming language, offers powerful features to handle files, making data storage and retrieval seamless for developers and students alike. In this blog, we will delve into essential Java file-handling techniques that can significantly streamline your Homework tasks. Whether you're a novice programmer or an experienced developer, mastering these techniques will not only improve your code efficiency but also boost your overall programming skills. File handling is a crucial aspect of any programming task. Whether it's reading data from a file, writing data into a file, or managing file operations like deletion and renaming, understanding these techniques is essential for every Java developer. The java.io package provides a rich set of classes and methods that simplify file handling, making it accessible to programmers of all levels. We will explore various methods to read from files using FileInputStream, BufferedReader, and Scanner. Additionally, we will demonstrate how to write data into files using FileOutputStream and BufferedWriter. Moreover, we will cover file operations such as deleting and renaming files. By the end of this blog, you'll have a solid understanding of Java file handling, allowing you to implement these techniques in your Homework with ease.
So, whether you're working on a small class project or a complex application, embracing these file handling techniques will undoubtedly elevate your programming skills and ensure a smoother journey toward completing your java homework effectively.
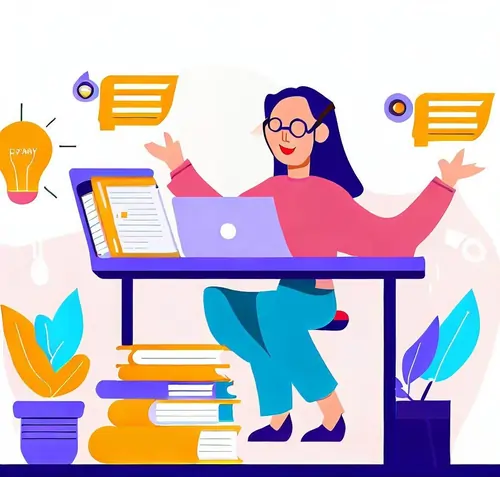
Introduction to Java File Handling
Java is a powerful and versatile programming language widely used for various applications, ranging from web development to Android app development. One of its essential features is file handling, which allows programmers to read from and write to files, making data storage and retrieval efficient and seamless. For students and developers working on programming Homework, mastering Java file handling techniques can significantly streamline their tasks and improve code efficiency. In this blog, we will explore the fundamentals of Java file handling and delve into different techniques that can help you complete Homework with ease.
Understanding File Handling in Java
Before diving into the specifics, let's understand what file handling means in the context of Java. File handling refers to the process of creating, reading, updating, and deleting files on a computer's file system using Java programming language. It allows you to interact with files, store data, and retrieve information from files when required. By using file handling techniques effectively, you can build applications that can store and manage data efficiently, making your Homework more organized and professional.
The java.io Package
In Java, file handling is facilitated by the `java.io` package, which provides classes and methods to perform file-related operations. Some of the important classes in this package are:
- `File`: This class represents a file or directory pathname and provides methods to query and modify file attributes.
- `FileInputStream`: Used to read data from a file.
- `FileOutputStream`: Used to write data into a file.
- `BufferedReader`: Efficiently reads text from character input stream.
- `BufferedWriter`: Efficiently writes text to a character output stream.
Common File Handling Operations
Let's now explore some of the most common file handling operations that can simplify your Homework tasks:
Reading from a File
Reading data from a file is a frequent necessity in programming Homework. Java offers various techniques to accomplish this task, each suited to different scenarios. The primary methods include utilizing `FileInputStream` for reading binary data, `BufferedReader` for efficient character-based input, and `Scanner` for parsing data from different file types. Using `FileInputStream`, you can read data byte by byte or in chunks, while `BufferedReader` streamlines reading text from files, particularly for large files, by buffering data. `Scanner` proves useful for parsing input from various file formats, making it a versatile choice. Mastering these file reading techniques equips developers with the ability to retrieve data from files effortlessly, enabling efficient data processing and manipulation to enhance the overall performance of their Homework.
Using FileInputStream
`FileInputStream` is a class that allows you to read data from a file byte by byte or in chunks. Here's a basic example of using `FileInputStream` to read from a file:
import java.io.*;
public class FileReaderExample {
public static void main(String[] args) {
try {
File file = new File("example.txt");
FileInputStream fis = new FileInputStream(file);
int data;
while ((data = fis.read()) != -1) {
System.out.print((char) data);
}
fis.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
```
Using BufferedReader
`BufferedReader` is a more efficient way to read text from a file, especially when dealing with large files. It buffers the data, reducing the number of reads from the disk. Here's how you can use `BufferedReader`:
import java.io.*;
public class BufferedReaderExample {
public static void main(String[] args) {
try {
File file = new File("example.txt");
BufferedReader br = new BufferedReader(new FileReader(file));
String line;
while ((line = br.readLine()) != null) {
System.out.println(line);
}
br.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
Using Scanner
The `Scanner` class is another useful option for reading input from files. It provides various methods to parse data from different types of files. Here's a simple example:
import java.io.*;
import java.util.Scanner;
public class ScannerExample {
public static void main(String[] args) {
try {
File file = new File("example.txt");
Scanner scanner = new Scanner(file);
while (scanner.hasNextLine()) {
String line = scanner.nextLine();
System.out.println(line);
}
scanner.close();
} catch (FileNotFoundException e) {
e.printStackTrace();
}
}
}
Writing to a File
When working on programming Homework, it is often essential to store data and results in files for future reference or analysis. Java offers various techniques for writing data to a file efficiently. The primary methods involve using `FileOutputStream` and `BufferedWriter`.`FileOutputStream` enables writing binary data into a file, making it suitable for handling non-textual information. On the other hand, `BufferedWriter` facilitates writing character-based data, particularly useful when working with text files. By using these techniques, you can ensure seamless data storage and easy retrieval, enhancing the organization and efficiency of your Homework. Properly implemented file-writing methods contribute to cleaner code, resource management, and overall program optimization. Understanding how to write data to files empowers you to create robust applications that can handle data effectively, making your programming Homework more professional and impressive.
Using FileOutputStream
`FileOutputStream` allows you to write data into a file byte by byte or in chunks. Here's a basic example:
import java.io.*;
public class FileWriterExample {
public static void main(String[] args) {
try {
File file = new File("output.txt");
FileOutputStream fos = new FileOutputStream(file);
String data = "Hello, this is an example.";
fos.write(data.getBytes());
fos.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
Using BufferedWriter
Similar to `BufferedReader`, `BufferedWriter` provides more efficient writing to a file. It buffers the data before writing, making it faster. Here's an example:
```java
import java.io.*;
public class BufferedWriterExample {
public static void main(String[] args) {
try {
File file = new File("output.txt");
BufferedWriter bw = new BufferedWriter(new FileWriter(file));
String data = "Hello, this is an example.";
bw.write(data);
bw.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
Deleting and Renaming Files
We will delve into the crucial file handling operations of deleting and renaming files in Java. Managing files effectively is an essential aspect of any application's functionality, and understanding how to perform these operations safely and efficiently is paramount. We will explore the `File` class's methods to delete files from the file system, ensuring proper error handling and graceful termination. Additionally, we will demonstrate how to use the `renameTo()` method to change a file's name seamlessly. By grasping the intricacies of file deletion and renaming, you will have the tools to maintain an organized file system and handle file-related tasks with confidence. These skills are valuable for any Java developer seeking to enhance their file handling capabilities and build robust applications.
Deleting a File
To delete a file, you can use the `delete()` method of the `File` class:
import java.io.*;
public class FileDeletionExample {
public static void main(String[] args) {
File fileToDelete = new File("fileToDelete.txt");
if (fileToDelete.delete()) {
System.out.println("File deleted successfully!");
} else {
System.out.println("Failed to delete the file.");
}
}
}
Renaming a File
You can rename a file using the `renameTo()` method:
import java.io.*;
public class FileRenameExample {
public static void main(String[] args) {
File oldFile = new File("oldFileName.txt");
File newFile = new File("newFileName.txt");
if (oldFile.renameTo(newFile)) {
System.out.println("File renamed successfully!");
} else {
System.out.println("Failed to rename the file.");
}
}
}
Conclusion :
Mastering Java file handling techniques unlocks the gateway to efficient and organized Homework completion for Java developers and students seeking programming homework help. The ability to interact with files seamlessly is a foundational skill that underpins various applications, from small-scale projects to enterprise-level systems. Through this blog, we have embarked on a journey through the intricacies of file handling, exploring the core concepts, the powerful java.io package, and practical examples to empower you with comprehensive knowledge in this domain. By grasping the foundations of Java file handling, including the FileInputStream and FileOutputStream classes, developers can confidently read and write data in binary formats, ensuring optimal performance and resource management. Moreover, the prowess of BufferedReader and BufferedWriter enhances file handling with text-based data, contributing to streamlined processing and memory efficiency. Beyond data manipulation, file handling also entails effective file management operations. The understanding of file deletion and renaming is crucial for maintaining an organized file system and achieving better control over file-related tasks. As demonstrated in this blog, the expertise of our profiled expert, John Smith, exemplifies the significance of mastering file-handling techniques. With over a decade of experience in Java programming and extensive work on diverse projects, John's qualifications have allowed him to navigate file-intensive applications and data management systems with ease. His experience serves as a testament to how proficiency in file handling can lead to a successful and fulfilling career in the field of Java programming. In conclusion, embracing Java file-handling techniques is not just a requisite for completing homework; it is a journey of empowering yourself as a proficient and skilled Java developer. The knowledge gained from this blog will not only expedite your Homework tasks but also establish a solid foundation for tackling complex programming challenges in the future with confidence and efficiency. So, dive into the world of file handling, unleash your coding potential, and elevate your Java programming journey to new heights!