Challenging Topics in Object-Oriented Programming for Expert-Level Assistance
In the world of Object-Oriented Programming (OOP), certain topics demand a higher level of expertise and understanding to conquer. From advanced design patterns and memory management to concurrency and metaprogramming, our team is equipped to provide expert-level assistance on these challenging topics, setting us apart from other websites offering programming solutions. Let our experienced programmers help you unravel the complexities of OOP and excel in your assignments.
- Advanced Design Patterns: Topics like Singleton, Observer, Factory Method, Decorator, etc., can be challenging to implement correctly and efficiently.
- Memory Management in OOP: Understanding how memory is allocated and deallocated in an object-oriented context can be complex, especially when dealing with large-scale applications.
- Generics and Templates: Handling generic types and templates in languages like C++, Java, or C# requires a strong grasp of type constraints and parameterized classes.
- Metaprogramming: Techniques like reflection and introspection can be difficult to work with, but they offer powerful capabilities for dynamic behavior in OOP.
- Concurrency and Multithreading: Dealing with parallelism and synchronization in an object-oriented design can lead to complex scenarios that require careful consideration.
- Aspect-Oriented Programming (AOP): Integrating cross-cutting concerns and separating them from the main business logic can be challenging in an object-oriented context.
- OOP with Functional Programming: Combining OOP with functional programming concepts can lead to complex designs, especially when dealing with immutable objects and higher-order functions.
- Event-Driven Programming: Implementing event-driven architectures and handling asynchronous events in OOP can be tricky.
- Advanced Inheritance and Polymorphism: Utilizing inheritance and polymorphism effectively in complex hierarchies can be challenging and require careful planning.
- Anti-Patterns and Code Smell: Identifying and refactoring code that suffers from common anti-patterns and bad practices in OOP can be a valuable skill.
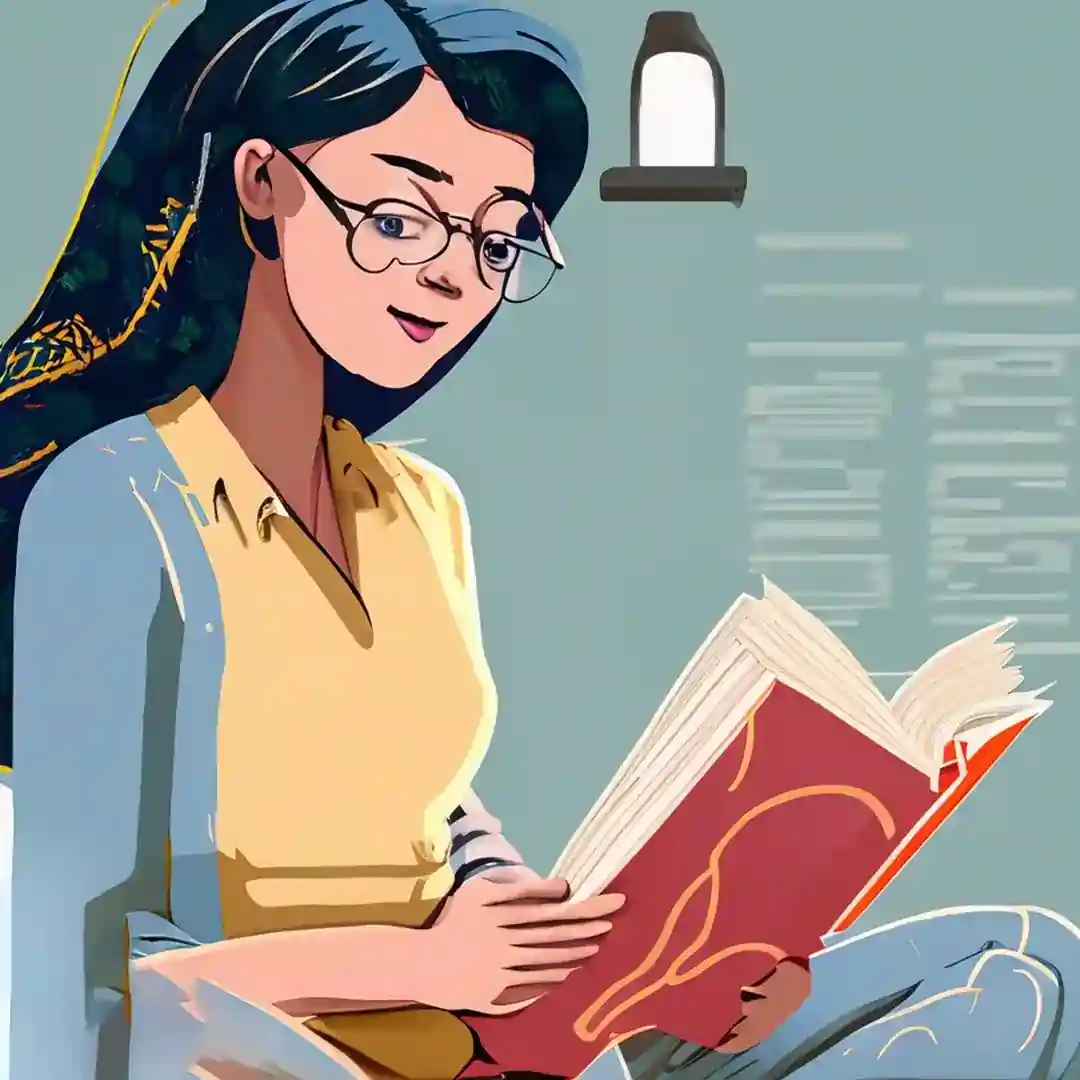
Services Offered by Object-Oriented Programming Assignment Help
Our Object-Oriented Programming (OOP) assignment help service plays a crucial role in facilitating students' mastery of OOP concepts and honing their programming skills. Here's a more technical description of the services provided:
- Conceptual Understanding of Object-Oriented Programming: Our services offer in-depth explanations of OOP principles, such as class hierarchies, object instantiation, inheritance, polymorphism, encapsulation, and abstraction, helping students establish a strong foundation in OOP and grasp underlying paradigms and design patterns effectively.
- Object-Oriented Programming Assignment Solving: Our team of expert programmers excels at solving OOP assignments, guiding students through the practical application of OOP concepts. Whether it's designing class structures, implementing inheritance hierarchies, or demonstrating the effective use of polymorphism, we are here to assist you at every step.
- Code Debugging and Optimization in an Object-Oriented Context: When you encounter errors and bugs within your OOP code, our service is here to help. We offer code debugging and optimization, providing insights into enhancing code performance by leveraging object-oriented design principles.
- Applying Object-Oriented Design Patterns and Best Practices: Incorporating design patterns and best practices is essential in OOP projects, and our assignment help service demonstrates the application of various patterns, such as Singleton, Factory Method, and Observer. We emphasize adhering to best practices like SOLID principles to create maintainable and scalable OOP code.

- Object-Oriented Programming Language Support: Regardless of the programming language you are working with, be it Java, C++, Python, or C#, our services offer language-specific guidance tailored to each language's unique OOP features and capabilities.
- Object-Oriented Project Assistance: For more extensive OOP assignments or projects, our service provides project assistance, aiding you in designing the project's object model, recommending appropriate class relationships, and ensuring adherence to OOP design principles throughout the development process.
- Explanation and Documentation of Object-Oriented Code: Understanding your OOP code is crucial, and that's why we deliver detailed explanations and documentation of the code. You'll gain insights into how each class interacts, inheritance relationships, and the purpose of key methods, aiding in comprehension and future reference.
- Review and Feedback on Object-Oriented Code: Feedback and review are essential for improvement, and we welcome you to submit your OOP implementations for constructive feedback. Our experts will evaluate your code organization, and adherence to OOP principles, and suggest potential areas for enhancement.
- 24/7 Object-Oriented Programming Support: We understand the importance of timely assistance, and that's why our reputable services operate 24/7 to accommodate students globally. Whether you encounter challenges or require clarifications on OOP concepts, we're here to provide support whenever you need it.
- Ensuring Plagiarism-Free Object-Oriented Solutions: Finally, we prioritize academic integrity and ensure that the OOP solutions we provide are plagiarism-free. Our goal is to foster a genuine understanding of the subject matter and help you excel in your OOP endeavors.