Mastering Exception Handling in Java Homework: A Key to Error-Free Programming
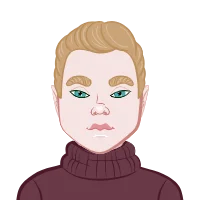
Exception handling is a crucial aspect of Java programming, ensuring that programs can gracefully respond to unexpected events or errors that may occur during execution. As a Java developer working on programming Homework, mastering exception handling is essential for creating robust and reliable applications. In this comprehensive guide, we will delve into the fundamentals of exception handling in Java and explore best practices to handle exceptions effectively to achieve Java homework help success. Understanding the types of exceptions in Java, such as checked and unchecked exceptions, is vital for making informed decisions when designing error-handling strategies. We will explore the primary exception-handling mechanisms, including the try-catch block, the finally block, and the try-with-resources statement, each offering unique benefits in managing exceptions and resources. By adopting best practices, like being specific in exception handling, implementing proper logging, and avoiding common mistakes such as catching Throwable, you can develop code that is more maintainable and less prone to hidden bugs. Exception handling is not only about preventing crashes; it's also an essential part of creating user-friendly applications that handle errors gracefully.
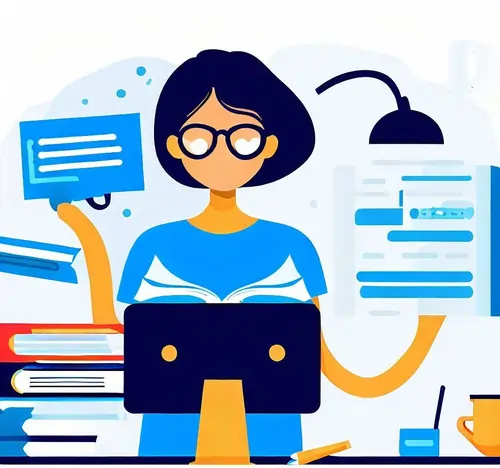
Types of Exceptions in Java
Java categorizes exceptions into two main types: checked exceptions and unchecked exceptions. Checked exceptions are exceptions that the compiler requires you to handle explicitly in your code using a try-catch block or declare them in the method signature using the "throws" keyword. These exceptions are generally associated with external factors or resources that might not be available during program execution, such as file I/O or network operations. On the other hand, unchecked exceptions, also known as runtime exceptions, do not need to be declared in the method signature or handled using try-catch blocks explicitly. They are typically caused by logical errors in the code or other exceptional conditions beyond the programmer's control, such as dividing by zero or accessing an out-of-bounds array index. Understanding the distinction between these two types is crucial for designing effective exception handling strategies and writing reliable Java programs.
Checked Exceptions
Checked exceptions are exceptions that the compiler requires you to handle explicitly in your code using a try-catch block or declare them in the method signature using the "throws" keyword. These exceptions are generally associated with external factors or resources that might not be available during program execution, such as file I/O or network operations. Some commonly encountered checked exceptions include IOException, FileNotFoundException, and SQLException.
Unchecked Exceptions
Unchecked exceptions, also known as runtime exceptions, do not need to be declared in the method signature or handled using try-catch blocks explicitly. They are typically caused by logical errors in the code or other exceptional conditions beyond the programmer's control, such as dividing by zero or accessing an out-of-bounds array index. Examples of unchecked exceptions are NullPointerException, ArithmeticException, and ArrayIndexOutOfBoundsException.
The Importance of Choosing the Right Exception Type
When handling exceptions in your Java Homework, it's crucial to choose the appropriate exception type for different scenarios. Checked exceptions are typically used for recoverable errors that can be handled gracefully, ensuring that the program continues its execution smoothly. On the other hand, unchecked exceptions are reserved for situations where recovery might be challenging or even impossible, often indicating critical issues in the program's logic or environment. Making the right choice between checked and unchecked exceptions ensures that your exception handling strategy aligns with the nature of the error and promotes robust error management in your Java applications. By understanding the significance of selecting the right exception type, you can build more reliable and fault-tolerant code.
Exception Handling Mechanisms
Java provides several mechanisms to handle exceptions effectively. Understanding these mechanisms will empower you to write more resilient and efficient code. The try-catch block is the primary construct used to handle exceptions in Java, allowing you to wrap the code that may throw an exception within a "try" block and specify how to handle the exception in the corresponding "catch" block. The catch block is executed when an exception occurs within the try block, allowing you to provide error-specific instructions or log the issue for debugging. Additionally, the "finally" block ensures that certain code is executed, regardless of whether an exception is thrown or not, making it useful for cleanup operations or resource management. Furthermore, the try-with-resources statement simplifies resource management by automatically closing resources that implement the `AutoCloseable` interface. By mastering these exception-handling mechanisms, you can create Java programs that handle errors gracefully and maintain high levels of reliability and performance.
The try-catch Block
The try-catch block is the primary construct used to handle exceptions in Java. It allows you to wrap the code that might throw an exception within a "try" block and specify how to handle the exception in the corresponding "catch" block. The catch block is executed when an exception occurs within the try block, allowing you to provide error-specific instructions or log the issue for debugging.
try {
// Code that may throw an exception
} catch (ExceptionType e) {
// Exception handling and recovery
}
The finally Block
In some cases, you might want to ensure that certain code is executed, regardless of whether an exception is thrown or not. The "finally" block allows you to define such code, making it useful for cleanup operations or resource management. The finally block is executed after the try-catch block, regardless of whether an exception is caught or not.
try {
// Code that may throw an exception
} catch (ExceptionType e) {
// Exception handling and recovery
} finally {
// Code that always gets executed
}
The try-with-resources Statement
Introduced in Java 7, the try-with-resources statement simplifies resource management by automatically closing resources that implement the AutoCloseable interface. It ensures that resources, such as file streams or database connections, are properly closed, even if an exception occurs during execution.
try (ResourceType resource = new ResourceType()) {
// Code that uses the resource
} catch (ExceptionType e) {
// Exception handling and recovery
}
// The resource is automatically closed after the try-catch block
By leveraging these powerful exception handling mechanisms in Java, you can effectively manage exceptions and resources in your code, ensuring that your applications remain robust and stable even in the face of unexpected errors. Whether it's gracefully recovering from recoverable exceptions, cleaning up resources in the finally block, or streamlining resource management with try-with-resources, these constructs enhance the overall reliability and maintainability of your Java programs.
Best Practices for Exception Handling in Java Homework
Mastering the art of exception handling involves not only understanding the mechanics but also following best practices to write clean and maintainable code. When catching exceptions, avoid using overly broad catch blocks like catching `Exception` or `Throwable` unless absolutely necessary. Be specific in exception handling, allowing you to handle different types of exceptions differently and ensuring that you don't inadvertently catch exceptions that require special treatment. Implement robust logging mechanisms to record exceptions and relevant information during program execution, aiding in the diagnosis and debugging of issues. Consider incorporating error reporting functionality, allowing users to provide feedback and report errors for further investigation and improvement. Avoid catching exceptions without action, as catching an exception and doing nothing in the catch block can lead to silent failures and obscure bugs. By adhering to these best practices, you can enhance the reliability and maintainability of your Java Homework while building a solid foundation for your programming expertise.
Be Specific in Exception Handling
When catching exceptions, avoid using overly broad catch blocks like catching `Exception` or `Throwable` unless absolutely necessary. Being specific allows you to handle different types of exceptions differently and ensures that you don't inadvertently catch exceptions that require special treatment.
// Avoid using: catch (Exception e) { ... }
catch (IOException e) {
// Handle IOException
} catch (SQLException e) {
// Handle SQLException
}
Logging and Error Reporting
Implement robust logging mechanisms to record exceptions and relevant information during program execution. Proper logging helps you diagnose and debug issues in your application. Additionally, consider incorporating error reporting functionality, allowing users to provide feedback and report errors for further investigation and improvement.
Handling exceptions without taking appropriate action can lead to serious issues, such as silent failures that are hard to trace. Always include meaningful actions in the catch block, such as logging the exception or informing the user appropriately.
try {
// Code that may throw an exception
} catch (Exception e) {
// Avoid doing nothing here
}
Catching Throwable
Catching `Throwable` is generally considered a bad practice, as it includes even errors and other critical conditions that should not be caught in most cases. Stick to catching specific exceptions that you can handle gracefully.
// Avoid using: catch (Throwable t) { ... }
catch (ExceptionType e) {
// Handle the specific exception
}
By being specific in your exception handling and implementing robust logging and error reporting, you can effectively manage exceptions in your Java applications. Avoiding common mistakes like catching overly broad exceptions or catching `Throwable` will lead to more maintainable and debuggable code, improving the overall reliability and user experience of your software.
Common Mistakes to Avoid in Exception Handling
While exception handling is essential, improper practices can lead to code that is difficult to maintain and debug. Here are some common mistakes to avoid: Catching exceptions without action, where catching an exception and doing nothing in the catch block can lead to silent failures and obscure bugs. Catching `Throwable` is generally considered a bad practice, as it includes even errors and other critical conditions that should not be caught in most cases. Swallowing exceptions by using overly broad catch blocks like catching `Exception` or `Throwable` can hide valuable error information and hinder effective debugging. Relying solely on print statements for error reporting and debugging can be inadequate, especially in large and complex applications. Neglecting to handle or log exceptions in critical parts of the code can result in unexpected behavior and undermine the stability of the program. By avoiding these common mistakes, you can create more robust and reliable Java applications with a smoother exception handling process.
Catching Exceptions Without Action
Catching an exception and doing nothing in the catch block, also known as "swallowing" the exception, can lead to silent failures and obscure bugs. Always include meaningful actions in the catch block, such as logging the exception or informing the user appropriately.
try {
// Code that may throw an exception
} catch (Exception e) {
// Avoid doing nothing here
}
Catching Throwable
Catching `Throwable` is generally considered a bad practice, as it includes even errors and other critical conditions that should not be caught in most cases. Stick to catching specific exceptions that you can handle gracefully.
// Avoid using: catch (Throwable t) { ... }
catch (ExceptionType e) {
// Handle the specific exception
}
When catching exceptions, it is essential to take appropriate action to handle the error scenario effectively. Simply catching an exception and not doing anything about it can lead to unanticipated issues, as the program may proceed with incorrect or incomplete data. Always consider logging the exception details for future debugging and analysis. Informing the user about the error in a user-friendly manner allows them to understand the problem and, if applicable, take necessary actions. On the other hand, catching `Throwable` is discouraged because it encompasses not only exceptions but also errors and other severe conditions that may indicate unrecoverable problems in the application. By avoiding these pitfalls and adopting a specific and thoughtful approach to exception handling, you can create more robust and reliable Java applications.
Conclusion
In Java, handling exceptions skillfully is vital for writing reliable and efficient code. Exception handling allows programs to respond gracefully to unexpected events or errors that may occur during execution, ensuring a smoother user experience and preventing abrupt crashes. By understanding the various types of exceptions, such as checked and unchecked exceptions, developers can choose the appropriate handling approach for different scenarios, leading to better error management. Utilizing the provided exception handling mechanisms, including the try-catch block, the finally block, and the try-with-resources statement, empowers developers to create code that effectively manages resources and gracefully recovers from exceptional situations. Adopting best practices in exception handling, such as being specific in catch blocks, implementing robust logging, and avoiding common mistakes, can significantly enhance the maintainability and debugging process of Java applications. Ultimately, by mastering exception handling, you not only ensure the success of completing your programming Homework but also become a more proficient and accomplished Java developer, capable of building robust and user-friendly applications. Happy coding.