A Comprehensive Guild For Creating Word Puzzle Game For Web Server
May 20, 2023
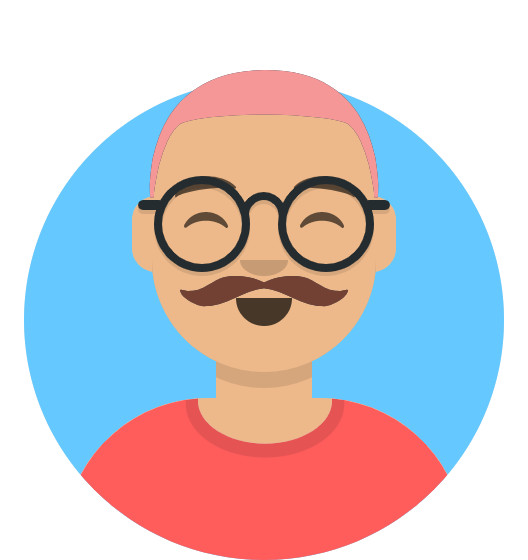
Devin Mark
United States
C++ For Backend
With a Bachelor's degree in Computer Science from a reputable institute, John has extensive experience in designing and implementing robust backends for interactive games.
Are you looking for assistance with developing a C++ code for the web backend of your word puzzle game homework? Look no further! Our team of experienced Programming Homework Help Experts. We can help you create a robust and efficient codebase that will power your game's backend functionalities. Whether you need assistance with database integration, user authentication, or game logic implementation, our experts are here to support you every step of the way. Don't let coding challenges hold you back - reach out to us and let's bring your word puzzle game to life! Working on a Word Puzzle Game's Web back-end Introduction to C++ Web back-end development doesn't typically use C++, the enduring high-performance language popular for system and application software, embedded programming, and even game development. Traditional leaders in the field include Python, Ruby, JavaScript (Node.js), and more recently, Go. However, using C++ for web back-end development could have significant advantages in certain situations with high computational demands. This article will explore a similar situation and show how to use C++ to develop a web back-end for a word puzzle game.

Let's Talk About Why Web Servers Should Use C++ as a Back-end Language:
Performance:
The efficiency and performance of C++ are well-known. Heavy data processing or real-time tasks are frequently present in web back-ends. For instance, C++ is particularly good at the kind of high-speed computation needed for data analytics, machine learning algorithms, or multiplayer gaming. Being a compiled language, C++ executes more quickly than interpreted languages because it translates directly into machine code. Server applications that are highly optimized and effective can be created thanks to the low-level memory access it provides and the elimination of overheads like garbage collection.
A web server must be able to handle multiple requests at once, which is known as multithreading. Strong multithreading support is provided by C++, enabling concurrent processing and greatly increasing server throughput. Multiple clients can be served concurrently by a multithreaded server without blocking or waiting for one request to complete before handling another. For large-scale applications, where servers must manage thousands or even millions of requests per second, this feature is essential.
Control over Hardware Resources:
C++ gives programmers unprecedented access to system resources. It enables efficient use of server resources by enabling fine-tuning of memory usage, system calls, and processor time. This control can result in significant performance improvements and cost savings in environments with constrained resources, like embedded systems or high-load servers.
C++ is interoperable with a variety of other languages and technologies. When integrating with third-party libraries, integrating with legacy systems, or when parts of the system are written in different languages, this interoperability is especially helpful. Additionally, C++ supports direct calls to system-level APIs, enabling the development of strong, effective system tools.
The C++ Standard Library (STL) offers a wide range of ready-to-use data structures, algorithms, and utility functions. It is a mature and robust library. Because of the STL's highly effective and tried-and-true data structures and algorithms, you can cut down on development time while also improving the code's quality and performance.
Security:
In C++, memory management is directly under the developers' control. The likelihood of specific security flaws, such as those involving automatic memory management or garbage collection, is decreased by this feature. Strong type checking in C++ at compile time also aids in catching many errors that could otherwise result in security problems.
Cross-platform Compatibility: C++ runs on a variety of operating systems, including Windows, Linux, and macOS. This means that your C++ server code can run on various operating systems with little to no modification, if any at all. When developing applications for various server environments, this feature is crucial.
Flexibility:
C++ is used in a wide range of applications, from desktop programs to real-time systems to sophisticated game engines. Due to its adaptability, it works well for a variety of back-endfunctionalities. C++ is capable of handling any task, including real-time media streaming, intensive mathematical calculations, and complex business logic.
Strong Typing: C++ is a strongly-typed, statically-typed language, which helps to reduce the likelihood of many programming errors. Because type-related errors are caught during compilation rather than runtime, the software is safer and more reliable. Additionally, strong typing promotes better coding techniques and makes the code simpler to comprehend and update.
A sizable developer community and a wealth of resources are available for C++, including a sizable number of libraries, tools, tutorials, and forums. This established ecosystem aids developers in overcoming obstacles more quickly, learning from others' mistakes, and keeping up with new standards and practices. It is simple to use a variety of open-source libraries to accelerate development and eliminate the need to assemble complex components from scratch.
Framework for the Crow
To begin, we require a suitable C++ framework that makes the process of developing a web server easier. Crow enters the picture at this point. Crow is a C++ micro web framework that was created to be quick and simple to use. Crow enables you to quickly and easily create HTTP servers with REST APIs, and it was inspired by Python's Flask.
After finishing the introduction, let's move on to the main course: creating the back-endfor a Word Puzzle game using Crow and C++.
Word Puzzle game
The Word Puzzle game we create will be easy to play but entertaining. The client will try to guess the word after the game server has chosen it at random. When a client requests a new game, a new game session starts, and it ends when the client correctly guesses the word.
Let's break down our development plan into phases:
Step One: Determining the Game Structure
To hold the game's state, we will first define a straightforward structure called Game. The actual word to guess and the player's guess will both be contained in this structure.
struct Game {
std::string currentWord;
std::string wordGuessed;
};
--------------------------------------------
Step Two: keeping active games
We require a system for managing and storing multiple active games. Using a std::map is a straightforward but efficient strategy. The Game struct will serve as the value and each game will have a distinct ID as the key.
std::map games;
---------------------------------------------
Step Three: Making a New Game
We now require a method for players to launch a fresh game. This entails initializing the game state and adding a fresh entry to the map of the game with a special ID.
To do this, we'll create a route in Crow called /start. A new game will be started by the server whenever a client sends a GET request to this route.
CROW_ROUTE(app, "/start")([]() {
// find an unused id for a new game
int gameId = 0;
while(games.find(gameId) != games.end()) {
++gameId;
}
// initialize the game with a random word
games[gameId] = Game{"HELLO", ""};
// respond with the game id
return std::to_string(gameId);
});
----------------------------------------------------------------------
Step 4: Speculating
The player must be able to guess after the game has begun. We'll create a new route called /guess/int>/string>, where int> stands for the game ID and string> stands for the player's guess of the word.
CROW_ROUTE(app, "/guess//")([](const crow::request& req, int gameId, std::string guessedWord) {
// if game exists, compare the guessed word with the current word
if(games.find(gameId) != games.end()) {
games[gameId].wordGuessed = guessedWord;
if(guessedWord == games[gameId].currentWord) {
// if word guessed correctly, remove game and return success message
games.erase(gameId);
return "Congrats! You've guessed the word correctly.";
}
else {
// if word guessed incorrectly, return failure message
return "Oops! You've guessed the word incorrectly. Try again!";
}
}
else {
// if no game found with the provided id, return error message
return "Game not found.";
}
});
The server compares the player's guess with the current word using this code. If they match, the game is over and a success message is sent by the server. If not, a failure message is sent by the server.
----------------------------------------------------
Step Five :Running the Server
Finally, we must operate our server. This is as easy to do with Crow as calling an app.port(8080).multithreaded().run();.
Run the server using this command on port 8080.
Conclusion
This example showed how to create a straightforward web back-endfor a C++ Word Puzzle game. The code is lacking features like authentication, error handling, and complex game logic that you would typically find in a full-featured game, but the game itself is simple and serves as a good starting point. You can improve the user experience, add more game features, and even connect this code to a database to store player and game state information.
Finally, even though C++ may not be the most popular language for web back-enddevelopment, it shouldn't be disregarded. By enabling the use of C++'s computational power and control for web applications, frameworks like Crow expand the language's potential applications. Even though it might not completely replace the standard languages for back-endweb development, it offers a different option that is worth considering in situations where performance is crucial. Remember that selecting the appropriate tool frequently depends on the task at hand, and C++ may occasionally be that tool.