-
Home
-
Game Homework Solution In C++
Game of Nim
It is an intro to programming C++. The main objective of this course is to familiarize students with the fundamental concepts of programming using C++. Throughout the course, students will learn about variables, data types, control structures, functions, and more. For one of the assignments, students will need to write a C++ assignment program that demonstrates their grasp of the concepts taught in the class. The code should be written in a clear and organized manner, adhering to best practices and the principles of good coding style. This assignment will not only test their programming skills but also encourage them to think critically and creatively to solve real-world problems using C++.
Sample output 1:
Welcome to the game of Nim.

There are 16 marbles in the pile.
Φ Φ Φ Φ Φ Φ Φ Φ Φ Φ Φ Φ Φ Φ Φ Φ
Computer plays:
The computer takes 3 marbles.
There are 13 marbles in the pile.
Φ Φ Φ Φ Φ Φ Φ Φ Φ Φ Φ Φ Φ
Your play .. you may take 1 to 6 marbles. You take: I will move 6 marbles↵
Invalid! Please try again...
Your play .. you may take 1 to 6 marbles. You take: six marbles↵
Invalid! Please try again...
Your play .. you may take 1 to 6 marbles. You take: 6 marbles ok?↵
You will take 6 marbles.
There are 7 marbles in the pile.
Φ Φ Φ Φ Φ Φ Φ
Computer plays:
The computer takes 3 marbles.
There are 4 marbles in the pile.
Φ Φ Φ Φ
Your play .. you may take 1 to 2 marbles. You take: 4↵
Ooops, you picked too many or not enough. Please try again...
Your play .. you may take 1 to 2 marbles.
You take: three↵
Invalid! Please try again...
Your play .. you may take 1 to 2 marbles. You take: 3↵
Ooops, you picked too many or not enough. Please try again...
Your play .. you may take 1 to 2 marbles. You take: 2↵
You will take 2 marbles.
There are 2 marbles in the pile.
Φ Φ
Computer plays:
The computer takes 1 marble.
You must take the last marble. You lose!
Sample output 2:
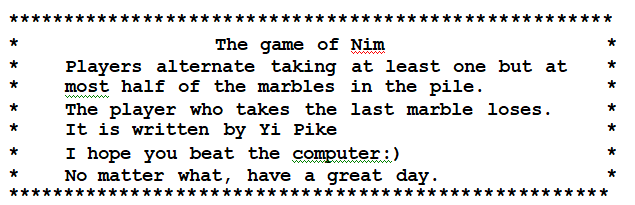
You will play first.
The computer will play smart.
There are 10 marbles in the pile.
Φ Φ Φ Φ Φ Φ Φ Φ Φ Φ
In your play .. you may take 1 to 5 marbles.
You take: 5 marbles and I will see how you move:)↵ You will take 5 marbles.
There are 5 marbles in the pile.
Φ Φ Φ Φ Φ
Computer plays:
The computer takes 2 marbles.
There are 3 marbles in the pile.
Φ Φ Φ
In your play .. you may take 1 to 1 marble. You take: -5↵
Ooops, you picked too many or not enough. Please try again...
In your play .. you may take 1 to 1 marble. You take: 7↵
Ooops, you picked too many or not enough. Please try again...
In your play .. you may take 1 to 1 marble. You take: 1↵
You will take 1 marble.
There are 2 marbles in the pile.
Φ Φ
Computer plays:
The computer takes 1 marble.
You must take the last marble. You lose!
Sample output 3:

The computer will play first.
The computer will not play smart.
There are 15 marbles in the pile.
Φ
Φ Φ Φ Φ Φ Φ Φ Φ Φ Φ Φ Φ Φ Φ
Computer plays:
The computer takes 6 marbles.
There are 9 marbles in the pile.
Φ Φ Φ Φ Φ Φ Φ Φ Φ
Your play .. you may take 1 to 4 marbles. You take: three is my choice↵
Invalid! Please try again...
Your play .. you may take 1 to 4 marbles. You take: 3 is my smart choice↵
You will take 3 marbles.
There are 6 marbles in the pile.
Φ Φ Φ Φ Φ Φ
Computer plays:
The computer takes 2 marbles.
There are 4 marbles in the pile.
Φ Φ
Φ Φ
Your play .. you may take 1 to 2 marbles. You take 1 and I am smart:) ↵
You will take 1 marble.
There are 3 marbles in the pile.
Φ Φ Φ
Computer plays:
The computer takes 1 marble.
There are 2 marbles in the pile.
Φ Φ
In your play, you may take 1 to 1 marble. You take: 1 and I will win the game:) ↵ You will take 1 marble.
The computer must take the last marble. You win!
Solution:
*/
#include < cstdlib>
#include < iostream>
#include < string>
#include < time.h>
using namespace std;
const int PILE_MAX = 50;
const int PILE_MIN = 10;
const char MARBLE = static_cast(232); // "Φ";
/*
* It represents the legal move when it is the computer's turn to play
* computer's legal move is under either smart-move or
* non-smart-move depending on the parameter named smart
*@param pile int&: the pile size before its turn and after its turn,
call by reference
*@param smart bool: representing the computer takes smart move (if true)
or non-smart move (if false)
*@return: void function
*/
void computer_play(int& pile, bool smart) {
int computer = 0;
// Display the number of marbles on current pile
cout << "There are " << pile << " marbles in the pile." << endl;
for ( int i = 0; i < pile; i++ ) {
cout << " " << MARBLE << endl;
}
if ( smart ) {
// For smart-move mode
// Check whether pile is equal to 2's power - 1
if ( pile == 1 || pile == 3 || pile == 7 || pile == 15 || pile == 31 ) {
// make a random move taking at least one but at most half of the marbles
computer = rand() % ( pile / 2 ) + 1;
} else {
// otherwise, take off enough marbles to make the size of the remaining pile
// a power of two minus one
if ( pile > 31 ) {
computer = pile - 31;
} else if ( pile > 15 ) {
computer = pile - 15;
} else if ( pile > 7 ) {
computer = pile - 7;
} else if ( pile > 3 ) {
computer = pile - 3;
} else if ( pile > 1 ) {
computer = pile - 1;
}
}
} else {
// For non-smart-move mode, make a random move taking at least one but at most half of the marbles
computer = rand() % ( pile / 2 ) + 1;
}
// Print how many marbles do the computer player takes
cout << "Computer plays:" << endl;
cout << "The computer takes " << computer << " marbles." << endl;
// Update the number of marbles in the pile
pile -= computer;
}
/*
* It represents the legal move when it is the user's turn to play the game
* (it should repeatedly ask the user to enter how many marbles to take
* until the user makes a legal move.)
*@param pile int&: the pile size before user's turn and after user's turn,
call by reference
*@return: void function
*/
void player_play(int& pile) {
string line;
bool isValid;
int max_player;
int player;
// Display the number of marbles on current pile
cout << "There are " << pile << " marbles in the pile." << endl;
for ( int i = 0; i < pile; i++ ) {
cout << " " << MARBLE << endl;
}
do {
// Calculate the maximum allowable marbles to be taken by the user player
max_player = pile / 2;
// Prompt to the user player to show what is the available range
cout << "Your play .. you may take 1 to " << max_player << " marbles." << endl;
cout << "You take: ";
getline( cin, line );
try {
// Use the stoi function to convert the input string line into integer
player = atoi( line.c_str() );
// If the user player takes too many or not enough marble
if ( player < 1 || player > max_player ) {
// print the error message, and request the user to input again
cout << "Ooops, you picked too many or not enough." << endl;
cout << "Please try again..." << endl;
isValid = false;
} else {
// If the user input is valid, print how many marbles do the user player takes
cout << "You will take " << player << " marbles." << endl;
isValid = true;
}
} catch (invalid_argument ex) {
// If the user input is not a valid number, the stoi function will generate exception
// and catch the exception with the exception handler to print the error message
cout << "Invalid! Please try again..." << endl;
isValid = false;
}
} while (isValid == false);
// Update the number of marbles in the pile
pile -= player;
}
int main(int argc, char** argv) {
int pile; /* Randomize the total number of marbles in the pile. */
bool first; /* Randomize which player (the computer or the user) takes the first turn. */
/* 1 - User player first; 0 - Computer player first */
bool smart; /* Randomize whether the computer takes either “smart†move or “non-smart†move. */
// Print out the follow information at the beginning of the game
cout << "*****************************************************" << endl;
cout << "* The game of Nim *" << endl;
cout << "* Players alternate taking at least one but at *" << endl;
cout << "* most half of the marbles in the pile. *" << endl;
cout << "* The player who takes the last marble loses. *" << endl;
cout << "* It is written by XXX *" << endl;
cout << "* I hope you beat the computer:) *" << endl;
cout << "* No matter what, have a great day. *" << endl;
cout << "*****************************************************" << endl;
// Using time function to randomize the seed
srand ((unsigned int)time(NULL));
// Generate a random integer between PILE_MIN and PILE_MAX to denote the initial pile size
pile = rand() % ( PILE_MAX - PILE_MIN + 1 ) + PILE_MIN;
// Generate 0 or 1 randomly to decide which player (the computer or the user) takes the first turn
first = rand() % 2;
// Generate 0 or 1 randomly to decide if the computer takes either “smart†move or “non-smart†move
smart = rand() % 2;
// Based on the random variable, first, print out which player will go first
if ( first ) {
cout << "You will play first." << endl;
} else {
cout << "The computer will play first." << endl;
}
// Based on the random variable, smart, print out what strategy will the computer player use
if ( smart ) {
cout << "The computer will play smart." << endl;
} else {
cout << "The computer will not play smart." << endl;
}
cout << endl;
// If the user player does not go first
if ( first == false ) {
/* Computer Round */
computer_play( pile, smart );
// Since the smallest number of marbles is 10, therefore after the first round will not
// have only 1 marble left, so that no need to handle who will lose the game
cout << endl;
}
do {
/* Player Round */
player_play( pile );
// If there is only one marble left after the user player's round
if ( pile == 1 ) {
// Print the computer player will lose the game
cout << "Computer must take the last marble. You win!" << endl;
break;
}
cout << endl;
/* Computer Round */
computer_play( pile, smart );
// If there is only one marble left after the computer player's round
if ( pile == 1 ) {
// Print the user player will lose the game
cout << "You must take the last marble. You lose!" << endl;
break;
}
cout << endl;
} while ( pile > 0 );
return 0;
}