A Student's Guide to Excellence: Adding JUnit 5 Unit Testing in Java
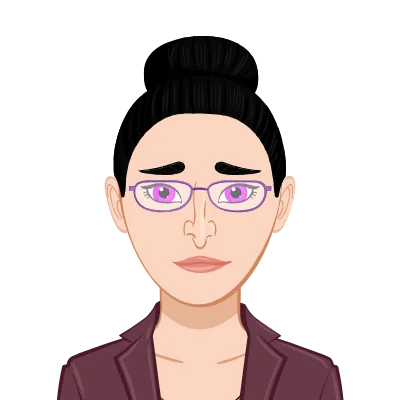
As a student learning Java programming, you'll often find yourself tackling assignments that require you to create and manage code. One pivotal aspect of software development that plays a pivotal role in these tasks is testing. In this blog post, we'll delve into the process of integrating unit tests using JUnit 5 into your pre-existing Java codebase. This skill is crucial for ensuring the correctness and reliability of your code, and it can greatly help you with your Java assignment and projects.
Unit testing isn't just an added layer of work; it's an investment in the quality and robustness of your code. By implementing these tests effectively, you can detect and rectify issues early in the development process, ensuring that your assignments meet the desired specifications. Whether you're solving mathematical equations or creating intricate software applications, understanding how to use JUnit 5 to create unit tests will equip you with a valuable skill set that will undoubtedly serve you well in your academic and future professional endeavors.

Why Unit Testing Matters
Before diving into the details of adding unit tests, let's briefly discuss why unit testing is essential. Unit testing is a critical practice in software development that serves as a robust safety net for your codebase. It helps identify and rectify issues early in the development process, reducing the likelihood of bugs making their way into the final product. Unit tests act as documentation for your code, providing clear examples of how to use it correctly. They also facilitate regression testing, ensuring that new changes or additions to your code do not inadvertently break existing functionality. Additionally, writing unit tests encourages the creation of modular, maintainable code, fostering better software design practices. In essence, unit testing is not just a best practice; it's a fundamental aspect of producing high-quality, reliable software that meets the needs of users and stakeholders alike.
1. Error Detection
Unit tests are invaluable for error detection in software development. By systematically testing individual units of code, you can identify bugs and issues at an early stage of the development process. This early detection is crucial because it allows developers to address problems promptly before they propagate throughout the codebase. Bugs that are caught early are typically easier and less expensive to fix, as they haven't yet led to complex interdependencies or caused cascading issues. In essence, unit tests act as a safety net, helping you catch and resolve errors while they are still manageable, preventing them from evolving into more challenging and costly problems.
2. Documentation
Well-crafted unit tests serve a dual purpose as documentation for your code. They provide concrete examples of how to interact with and utilize your code correctly. This documentation is invaluable for both yourself and your colleagues who may need to work with or maintain the code in the future. By reading the unit tests, developers can gain insights into the expected behavior and usage patterns of the code, reducing the likelihood of misunderstandings or misuse. This form of self-documentation not only aids in code comprehension but also accelerates the onboarding process for new team members, making it easier for them to understand and contribute to the project effectively.
3. Regression Testing
Unit tests provide a crucial component of regression testing, which is the process of verifying that changes or additions to your code do not adversely affect existing functionality. As software evolves, developers frequently introduce modifications to enhance or extend its capabilities. Without a solid set of unit tests, these changes can inadvertently introduce new bugs or disrupt previously functioning features. Unit tests act as a safety net that automatically checks the existing functionality each time you make alterations. This not only helps you detect regressions early but also ensures that your code remains stable over time. With comprehensive unit tests in place, you can confidently evolve your software while mitigating the risk of unintended consequences.
4. Improved Code Quality
One of the less obvious but incredibly beneficial outcomes of writing unit tests is the improvement in code quality. Unit testing encourages developers to write code that is modular, loosely coupled, and inherently testable. This practice leads to cleaner and more maintainable code, as each component is designed to perform a specific, well-defined task. By breaking down complex systems into smaller, manageable units, code becomes more comprehensible and easier to modify and extend. Additionally, unit tests push developers to consider various scenarios and edge cases, promoting robust error handling and defensive coding practices. Ultimately, the pursuit of effective unit testing naturally fosters better software design and coding habits, resulting in a higher-quality codebase.
Now that we've established the significance of unit testing in software development, let's delve into the practical steps of adding unit tests to your Java code using JUnit 5.
Setting Up JUnit 5
JUnit is renowned as a widely used testing framework for Java, and its latest iteration, JUnit 5, brings with it a plethora of new features and enhancements that improve the testing experience. To harness the power of JUnit 5 for your Java projects, you must take the initial step of setting it up within your development environment. This setup process is fundamental to your testing endeavors, as it lays the foundation for creating, executing, and managing your unit tests effectively. By integrating JUnit 5 seamlessly into your project, you gain access to a robust testing framework that empowers you to write comprehensive and reliable unit tests, ensuring the quality and correctness of your Java code. In the following sections, we will guide you through the essential steps required to configure JUnit 5, enabling you to harness its capabilities to their fullest extent in your software development journey.
1. Add JUnit 5 to Your Project
Integrating JUnit 5 into your project is the first crucial step towards leveraging its testing capabilities. If you're employing a popular build tool such as Maven or Gradle, the process is streamlined and straightforward. You'll need to add the JUnit 5 dependency to your project's build file. This dependency declaration ensures that your build tool fetches the necessary JUnit 5 libraries during the build process, enabling you to utilize its features seamlessly. For instance, in a Maven-based project, you can effortlessly include JUnit 5 by specifying the appropriate dependency in your project's pom.xml file. This fundamental setup ensures that your project is equipped with the tools required for efficient unit testing using JUnit 5, setting the stage for productive and reliable testing practices.
```xml
org.junit.jupiter
junit-jupiter-api
5.7.2
test
```
2. Create a Test Class
With JUnit 5 successfully integrated into your project, the next step is to create test classes that correspond to the classes you intend to test. In Java, adhering to naming conventions is essential for clarity and organization. Test classes typically share the same name as the class being tested, with the addition of "Test" at the end. For instance, if you have a Java class named "Calculator," your corresponding test class should be named "CalculatorTest." This naming convention not only simplifies the association between tests and the classes they target but also helps automated testing frameworks like JUnit 5 identify and execute your tests systematically. Creating well-structured test classes is pivotal in maintaining an organized and efficient testing suite, facilitating effective testing of your Java codebase.
Writing Your First Unit Test
Now that we've set up JUnit 5 in our project, it's time to dive into the practical aspect of writing your first unit test. To illustrate this process, let's consider a scenario where we have a Java class named "Calculator." This class contains a method called "add" designed to perform the addition of two numbers.
The Calculator Class
Before we proceed with writing the unit test, let's take a quick look at the "Calculator" class. It encapsulates the logic for adding two integers and is a simple example to demonstrate unit testing.
```java
public class Calculator {
public int add(int a, int b) {
return a + b;
}
}
```
Writing the Test
Now, let's shift our focus to creating a JUnit 5 test for the "Calculator" class. In JUnit 5, test classes are identified by annotations, and test methods are annotated with @Test. Our test method, "testAdd," will verify the functionality of the "add" method in the "Calculator" class. We will create an instance of the "Calculator" class, use its "add" method to add two numbers, and then assert that the result matches our expectations. This simple example serves as a starting point for understanding how to structure and write effective unit tests in Java using JUnit 5. Here's how you can write a JUnit 5 test for the `Calculator` class:
```java
import org.junit.jupiter.api.Test;
import static org.junit.jupiter.api.Assertions.assertEquals;
public class CalculatorTest {
@Test
public void testAdd() {
Calculator calculator = new Calculator();
int result = calculator.add(2, 3);
assertEquals(5, result);
}
}
```
Let's Break Down This Test
Now that we've written our first unit test for the "Calculator" class, let's break down the test into its individual components to better understand how it works and why each step is crucial in the context of unit testing using JUnit 5.
1. Annotating the Test Method with @Test
In JUnit 5, the process of designating a method as a test case is achieved through the use of annotations. We begin by annotating our test method with @Test. This annotation signals to JUnit that the method should be executed as a test case during testing. It serves as a marker for JUnit to identify and run the tests you intend to conduct, making it an essential part of structuring your test suite.
2. Creating an Instance of the Calculator Class and Calling its add Method
To validate the functionality of the "add" method in the "Calculator" class, we first create an instance of the "Calculator" class. This step simulates the real-world scenario where you would use this class. By invoking the "add" method on the instantiated object, we trigger the method's execution and capture its result. This step is fundamental in exercising the code under test, ensuring that it behaves as expected.
3. Using assertEquals to Verify the Result
To verify the correctness of our "add" method, we employ the assertEquals method provided by JUnit. This method allows us to compare the actual result produced by the "add" method with an expected value. In our example, we expect the addition of 2 and 3 to yield 5. Using assertEquals with this expected value ensures that the code under test behaves as intended. If the result differs from our expectations, the test will fail, alerting us to potential issues or regressions in our code.
By breaking down these essential components of our unit test, we gain insight into the systematic approach that JUnit 5 facilitates. This approach not only helps us write effective tests but also enhances our understanding of how our code functions, leading to more robust and reliable software.
Running Your Tests
Now that you've diligently written your unit tests, the next step is to execute them to ensure that your code behaves as expected. Thankfully, JUnit 5 provides multiple options for running your tests, allowing you to choose the method that best suits your workflow.
1. Using Integrated Development Environments (IDEs)
Many modern Integrated Development Environments (IDEs), such as IntelliJ IDEA and Eclipse, offer built-in support for running JUnit tests. This built-in integration simplifies the process significantly. All you need to do is navigate to your test class, right-click on it, and select "Run" or "Debug" from the context menu. This straightforward approach streamlines the testing process within your familiar coding environment, making it convenient and efficient.
2. Utilizing Command Line with Build Tools
Alternatively, you can run your JUnit tests from the command line using popular build tools like Maven or Gradle. This method is particularly useful when you need to automate your testing process or integrate it into your continuous integration (CI) pipeline. For instance, with Maven, you can execute your tests by running a simple command, like:
```shell
mvn test
```
This command triggers the execution of your JUnit tests, providing you with test results and reports that can be invaluable for tracking the health of your codebase.
These multiple avenues for running tests in JUnit 5 cater to diverse development environments and workflows, ensuring that you can effortlessly verify the correctness of your code under various scenarios.
Writing More Comprehensive Tests
While the example provided demonstrates a straightforward unit test, real-world applications frequently demand more intricate and comprehensive testing strategies. To ensure that your software functions reliably and accurately under diverse conditions, it's essential to expand your testing repertoire. The following tips will guide you in crafting comprehensive unit tests that cover a wide range of scenarios, including edge cases and potential sources of errors. These strategies will bolster your confidence in your code's correctness and robustness, making it well-prepared to handle the complexities of real-world applications. Here are some tips for writing comprehensive unit tests:
1. Test Different Cases
Writing comprehensive unit tests involves exploring a spectrum of scenarios. It's not enough to validate the common use cases; you must also assess how your code behaves in edge cases and when presented with invalid inputs. For instance, consider scenarios where negative numbers are passed to the "add" method or when you attempt to add zero to another number. Testing these scenarios helps uncover potential flaws and ensures that your code gracefully handles unexpected inputs, fortifying your codebase against errors and enhancing its reliability.
2. Use Assertions
JUnit 5 equips developers with a versatile set of assertion methods, such as assertEquals, assertTrue, and assertFalse. The choice of the appropriate assertion method is paramount in ensuring the accuracy and effectiveness of your unit tests. Each assertion method is designed to validate specific conditions or criteria within your tests. For instance, assertEquals is ideal for confirming the equality of expected and actual values, while assertTrue and assertFalse are suitable for verifying Boolean conditions. By employing the right assertion method for each test case, you can precisely scrutinize your code's behavior and ensure that it adheres to the expected logic and outcomes. This meticulous approach enhances the precision and efficacy of your unit tests, contributing to a more robust and dependable software system.
3. Set Up and Tear Down
In the realm of unit testing, establishing a consistent and controlled environment for your tests is paramount. JUnit 5 offers the @BeforeEach and @AfterEach annotations to help you set up initial conditions before each test and perform necessary clean-up tasks afterward. This feature is invaluable for ensuring that your test environment is in a predictable state, free from external influences, before you commence testing. Moreover, it aids in the efficient allocation and release of resources, promoting both the accuracy and tidiness of your test suite. By harnessing these annotations effectively, you can foster a well-organized and stable testing ecosystem that enhances the reliability and maintainability of your unit tests.
4. Parameterized Tests
JUnit 5 introduces support for parameterized tests, a powerful technique that enables you to execute the same test method with multiple sets of inputs. Parameterized tests eliminate redundancy in your test code by allowing you to define the test once and run it with various inputs, making your tests more concise and maintainable. This capability is particularly advantageous when you need to evaluate the behavior of a method under diverse scenarios or validate a range of input combinations. Leveraging parameterized tests streamlines your testing efforts, reduces code duplication, and provides a comprehensive assessment of your code's functionality across a spectrum of input values.
5. Mocking
In complex software systems, components often rely on external services or dependencies that can be challenging to control or replicate in a testing environment. Here, the use of mocking frameworks like Mockito comes to the rescue. Mockito allows you to create mock objects that simulate the behavior of these external dependencies, enabling you to isolate the class under test and focus solely on its functionality. By mimicking the interactions and responses of real services or components, you can systematically validate how your code interacts with its dependencies, without the need for the actual external resources. This approach simplifies testing, enhances test repeatability, and isolates potential issues within the class being tested, making it an invaluable tool for comprehensive unit testing.
Test-Driven Development (TDD)
Test-Driven Development (TDD) is an innovative software development methodology where tests are authored before writing the actual code. Although it may not always be applicable in assignments with rigid specifications, TDD represents a compelling technique for crafting superior code and ensuring that it aligns seamlessly with the intended functionality. By starting with the creation of tests that define the expected behavior of a specific feature or function, developers gain a clear roadmap for their coding endeavors. TDD promotes a structured approach, fostering a deeper understanding of requirements and the development process. It serves as a guiding force, steering developers toward code that is more maintainable, adaptable, and robust. While TDD can be initially challenging due to its inversion of traditional coding practices, its ultimate payoff is evident in the form of higher-quality software, fewer defects, and enhanced confidence in the code's reliability and functionality.
Here's a basic TDD cycle:
- Write a failing test case for the functionality you want to implement: The TDD process begins with the creation of a test case that clearly defines the expected behavior of the code you plan to develop. This initial test is intentionally designed to fail, serving as a concrete specification of what you aim to achieve. It outlines the expected inputs, outputs, and edge cases, providing a roadmap for your development efforts.
- Write just enough code to make the test pass: With the failing test in place, you proceed to write the minimum amount of code necessary to satisfy the test's conditions and make it pass successfully. This incremental approach encourages developers to focus on small, manageable portions of code, resulting in a steady, step-by-step progress toward the desired functionality.
- Refactor your code if necessary while ensuring the test still passes: After successfully passing the initial test, you can assess and refine your code. Refactoring involves improving the structure, readability, and efficiency of your code without altering its external behavior. Importantly, throughout the refactoring process, the test cases continue to ensure that the code meets the desired functionality. This iterative cycle of testing, coding, and refactoring fosters a continuous improvement mindset, leading to cleaner, more maintainable code.
By adhering to the principles of Test-Driven Development (TDD), developers can instill greater confidence in the correctness of their code. TDD's emphasis on rigorous testing from the outset of development helps identify and rectify issues early, significantly reducing the likelihood of common programming errors. This approach promotes a disciplined and systematic coding process that ultimately results in higher-quality software, increased reliability, and a more streamlined development workflow.
Conclusion
Incorporating unit tests using JUnit 5 into your Java code is a fundamental skill with far-reaching benefits, both for students and professional developers. It serves as a protective shield for your codebase, enabling you to detect and rectify issues at an early stage of development. By systematically validating your code through testing, you not only ensure its correctness but also create a valuable form of documentation that aids both yourself and your colleagues.
Effective unit testing is a skill that improves with practice. Start with simple tests, gradually progressing to more intricate scenarios. Over time, you'll develop the proficiency to craft tests that comprehensively assess your code's functionality. This journey toward mastery will ultimately transform you into a more proficient and discerning Java programmer.
Unit testing isn't just a technical exercise; it's a professional ethos that prepares you for a successful career in software development. As you navigate the challenges of assignments and projects, the discipline of unit testing will instill in you the ability to write reliable, maintainable code. In the dynamic world of software development, this is an invaluable asset. So, with the knowledge gained from this journey, embrace your coding adventures with confidence and enthusiasm. Happy coding!