Creating a 3D Breakout Game Using OpenGL: A Student's Guide
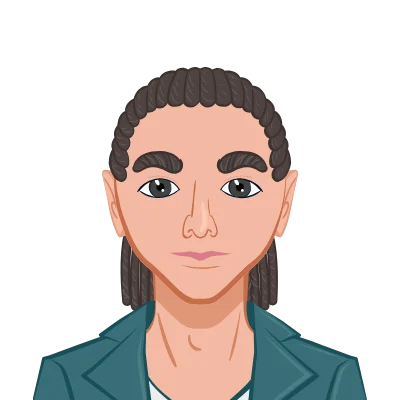
Breakout, a cherished classic of the arcade world, has captivated gamers for decades. Its charm is in its simplicity: you command a paddle at the screen's base, nimbly guiding a ball to demolish bricks above. Beyond its pure entertainment value, Breakout provides invaluable assistance with your OpenGL assignment and presents a dynamic learning opportunity for students keen on exploring the realms of computer graphics and game development. In this blog, we embark on a journey that expertly guides you through the creation of your own 3D Breakout game using OpenGL. This comprehensive guide is tailored to students eager to gain practical experience and enhance their skills in computer graphics and game development. We'll cover setting up your development environment, understanding game components, creating the game window, rendering game elements, managing user input, implementing game logic, mastering collision detection, adding 3D effects, integrating sound effects and music, and optimizing and debugging. By the end, you'll have not only a captivating 3D game but also the knowledge and expertise to tackle OpenGL assignments with confidence.
Setting Up Your Development Environment
Before we dive into the coding process, it's essential to set up your development environment for a smooth experience. Let's break down the key components you'll need:
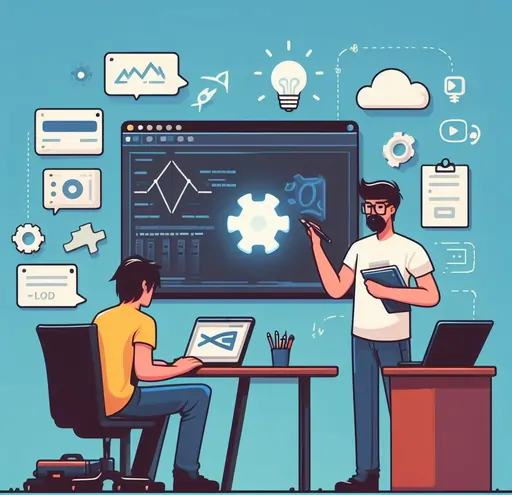
- Choose the Right IDE: Start by selecting a suitable Integrated Development Environment (IDE) such as Visual Studio or Code::Blocks. The IDE will be your workspace, offering features like code editing, debugging, and project management.
- Compiler Selection: Ensure you have a C++ compiler at your disposal, like the popular GCC (GNU Compiler Collection). The compiler translates your C++ code into machine-readable instructions.
- OpenGL Library: OpenGL is at the heart of this project. Make sure you have the OpenGL library installed. It's the tool that will enable you to work with graphics in 3D.
- Operating System Requirements: Different operating systems have specific requirements for setting up OpenGL. Familiarize yourself with the particulars of your OS to ensure proper configuration.
Once these pieces are in place and properly configured, you'll be well-prepared to venture into the world of 3D Breakout game development.
Understanding the 3D Breakout Game Components
In the realm of our 3D Breakout game, it's pivotal to grasp the fundamental components that make up the gameplay. These elements form the core of the gaming experience:
- Paddle: Positioned at the base of the screen, the paddle is the player's means of control. It becomes the dynamic tool for deflecting the game's central character—the ball.
- Ball: The dynamic entity at the heart of the game, the ball moves with vigor, bouncing around and obliterating the formidable brick structures. It's the source of anticipation and excitement in the game.
- Bricks: Positioned resolutely at the game's zenith, the bricks are the destructible targets. These formidable structures are the focus of the player's efforts, demanding precision and strategy.
- Walls: The walls encircle the game's domain, defining its boundaries and serving as the resilient containment structure for the ball and paddle.
Employing OpenGL, you'll harness the power to draw, manipulate, and breathe life into these essential components, transforming them into a captivating 3D gaming experience.
Creating the Game Window
The game window is your canvas for showcasing the 3D Breakout world you're about to create. In the realm of OpenGL, crafting this window is often facilitated by the GLFW library, a potent tool for window management. The process begins with the initialization of a GLFW window, laying the foundation for your game's rendering context.
```cpp
#include
#include
int main() {
// Initialize GLFW
if (!glfwInit()) {
// Handle initialization error
return -1;
}
// Create a windowed mode window
GLFWwindow* window = glfwCreateWindow(800, 600, "3D Breakout Game", NULL, NULL);
if (!window) {
// Handle window creation error
glfwTerminate();
return -1;
}
// Make the window's context current
glfwMakeContextCurrent(window);
// Initialize GLEW
if (glewInit() != GLEW_OK) {
// Handle GLEW initialization error
return -1;
}
// Game loop goes here
// Terminate GLFW
glfwTerminate();
return 0;
}
```
The code provided serves as your gateway into this pivotal step. By executing it, you'll manifest a window boasting an 800x600 resolution and an identifying title. Yet, an event loop is the pulse of your game, ensuring the window remains active, responsive, and capable of handling the user's interactions.
So, with your game window set and a responsive event loop in place, you've primed your canvas for the 3D Breakout masterpiece that's yet to come.
Drawing the Game Elements
OpenGL operates by rendering objects through a careful orchestration of vertices and shaders. In the realm of 3D Breakout, the ability to visually present your game's vital elements hinges on your proficiency in defining vertices and composing shaders. Shaders are your pocket-sized programs, executed on the Graphics Processing Unit (GPU), orchestrating the act of rendering.
To elucidate, consider a rudimentary example: drawing a rectangle. This fundamental graphic element can be brought to life in OpenGL by specifying its vertices, coupled with the appropriate shaders, which determine how it's presented. Here's a basic example of how to draw a rectangle using OpenGL:
```cpp
// Vertex data for a rectangle
float vertices[] = {
// Position // Color
0.0f, 0.0f, 0.0f, 1.0f, 0.0f, 0.0f, // Bottom-left
1.0f, 0.0f, 0.0f, 0.0f, 1.0f, 0.0f, // Bottom-right
1.0f, 1.0f, 0.0f, 0.0f, 0.0f, 1.0f, // Top-right
0.0f, 1.0f, 0.0f, 1.0f, 1.0f, 1.0f // Top-left
};
// Vertex shader
const char* vertexShaderSource = R"(
#version 330 core
layout(location = 0) in vec3 aPos;
layout(location = 1) in vec3 aColor;
out vec3 fColor;
void main() {
gl_Position = vec4(aPos, 1.0);
fColor = aColor;
}
)";
// Fragment shader
const char* fragmentShaderSource = R"(
#version 330 core
in vec3 fColor;
out vec4 FragColor;
void main() {
FragColor = vec4(fColor, 1.0);
}
)";
```
The logical next step involves compiling and linking these shaders, dispatching the meticulously defined vertex data to the GPU's capable hands, and then initiating the process of rendering. Bear in mind, the code's adaptability is key as you'll need to tailor it to each distinctive game element in your 3D Breakout world. This foundational knowledge forms the basis for constructing the captivating visuals that your players will interact with.
Handling User Input
To empower players to skillfully guide the paddle, you must master the art of capturing user input. The GLFW library equips you with a toolbox of functions for monitoring a plethora of user actions - from key presses to mouse movements and button clicks. Let's delve into a fundamental example to demonstrate the handling of keyboard input:
```cpp
while (!glfwWindowShouldClose(window)) {
// Process input
if (glfwGetKey(window, GLFW_KEY_LEFT) == GLFW_PRESS) {
// Move paddle left
}
if (glfwGetKey(window, GLFW_KEY_RIGHT) == GLFW_PRESS) {
// Move paddle right
}
// ...
// Game logic and rendering
}
```
Implementing Game Logic
At the heart of your 3D Breakout game lies the intricate web of game logic. This web entails tasks like propelling the ball forward, scrutinizing for exhilarating collisions, and meticulously keeping track of the player's score. Imagine this game logic as the cerebral cortex, coordinating every facet of the game.
```cpp
while (!glfwWindowShouldClose(window)) {
// Process input
// Update game logic
// Render game elements
}
```
A rudimentary game loop structure, the engine that powers your game's operations, could resemble the following. It tirelessly iterates, ensuring that the game's rules are enforced and that every element interacts seamlessly, leading to the immersive and dynamic experience your players seek:
Collision Detection and Response
In the Breakout game, collisions form the backbone of the gameplay experience. Your next challenge is to detect these collisions and respond accordingly. This crucial task involves monitoring the interactions between the ball, paddle, and bricks, ensuring that the game's rules are upheld. You can employ various collision detection algorithms, each tailored to your specific needs. Options range from the straightforward Axis-Aligned Bounding Box (AABB) method for simplicity, to more advanced techniques such as circle-to-circle collisions or the powerful Separating Axis Theorem (SAT).
Adding 3D Effects
Elevating your 3D Breakout game to new heights of visual appeal requires the incorporation of depth and perspective effects. This transformation entails crafting intricate 3D models for your in-game objects, enhancing them with textures and shaders that breathe life into their appearance. You might also contemplate introducing lighting and shadows, which can add depth and realism to the 3D environment, creating a richer and more immersive experience for players. These 3D effects are the brushstrokes that make your game's world come alive, inviting players into a captivating and dynamic universe.
Sound Effects and Music
Sound effects and music play a pivotal role in elevating the gaming experience from ordinary to extraordinary. The integration of audio elements can evoke emotions, intensify player engagement, and make your 3D Breakout game memorable. Utilize audio libraries like OpenAL or SDL_mixer to seamlessly weave sounds into the gameplay. Craft sound effects to accentuate ball hits, emphasize brick destruction, and consider background music to set the mood, immersing players into the game's ambiance.
Putting It All Together
With every game component in place, the time has come to harmonize these intricate parts into a cohesive whole. The key to success is meticulous testing and integration. Initially, evaluate each component independently, ensuring that they function precisely as intended. Subsequently, weave them into your primary game loop, the engine that drives your gaming world. The game loop operates as a well-orchestrated symphony, executing the following core steps:
- Handle user input: The bridge between player intent and in-game action.
- Update game logic: The driving force behind the game's dynamics, including position, velocity, and collision detection.
- Render game elements: The artist's brushstroke, painting the visual aspects of the game.
- Play audio: The soundtrack to your player's journey, reinforcing the immersive experience.
This succinct structure epitomizes the essence of your 3D Breakout game, a labor of passion and knowledge resulting in a captivating and interactive masterpiece. Here's a simplified structure of the game loop:
while (!glfwWindowShouldClose(window)) {
// Process input
// Update game logic
// Render game elements
// Play audio
}
Optimizing and Debugging
Once the pieces of your 3D Breakout game are in place, optimizing is the key to ensuring seamless performance. Techniques like frustum culling, level-of-detail (LOD) rendering, and efficient memory management can be your allies in ensuring that your game runs smoothly, even on hardware with lower specifications. However, perfection is a process, and debugging is an essential part of that journey. Leverage debugging tools and strategically placed print statements to swiftly identify and address issues lurking in your code.
Conclusion and Next Steps
Congratulations on your accomplishment! You've successfully brought to life a 3D Breakout game using the powerful toolset of OpenGL. This project stands as an exceptional learning opportunity for students passionate about the realms of computer graphics and game development. But remember, the world of game development is vast, offering boundless opportunities for growth and innovation.
Consider these next steps on your path to mastery:
- Experiment with Game Mechanics: Infuse your game with unique features and power-ups to make it distinct and more challenging.
- Advanced Rendering Techniques: Explore advanced rendering methods like shadow mapping, normal mapping, and post-processing effects to elevate your game's visual appeal.
- 3D Modeling: Dive into the fascinating world of 3D modeling and learn to craft your game assets using tools like Blender or Maya.
- Networked Gameplay: Explore networking to design multiplayer versions of your game, fostering a social dimension to your creation.
- Performance Optimization: Fine-tune your game for even broader hardware compatibility, ensuring players across a wide spectrum can enjoy your creation.
Always bear in mind that game development is a perpetual journey. It thrives on constant learning and practice, paving the way to enhanced skills and ever more captivating game experiences.
Conclusion
In conclusion, embarking on the journey of creating a 3D Breakout game using OpenGL is not only a rewarding endeavor but also a perfect stepping stone for students passionate about game development. This project seamlessly melds together various disciplines, from graphics programming to physics simulation and user interaction, resulting in a holistic learning experience that encapsulates the essence of modern game development.
The insights and guidelines presented in this guide serve as your launchpad, offering a firm foundation to start your adventure. Yet, the realm of game development is vast and ever-evolving. As you progress, don't hesitate to delve deeper into the intricacies, explore innovative gameplay mechanics, and experiment with advanced graphical effects. Each iteration, each new challenge you tackle, will contribute to your growth as a game developer.
In closing, your game development journey is a thrilling odyssey, filled with boundless possibilities and creativity. So, march forward with ambition and a thirst for knowledge, for the world of game development eagerly awaits your contributions. Good luck on your path to becoming a proficient game developer, and may your creations bring joy to players around the globe.