Claim Your Offer
Unlock an amazing offer at www.programminghomeworkhelp.com with our latest promotion. Get an incredible 20% off on your second programming assignment, ensuring top-quality assistance at an affordable price. Our team of expert programmers is here to help you, making your academic journey smoother and more cost-effective. Don't miss this chance to improve your skills and save on your studies. Take advantage of our offer now and secure exceptional help for your programming assignments.
We Accept
- Why Create a Text-Based Chess Game?
- Setting Up the Chessboard
- Implementing Chess Rules
- Managing Player Turns
- Handling User Input
- Displaying the Chessboard
- Checkmate and Game Over
- Conclusion
Chess, a timeless board game, has captivated and delighted individuals for centuries. It is not merely an enjoyable pastime but also a potent instrument for cultivating critical thinking, strategy, and problem-solving abilities. Within the confines of this blog post, we shall delve into the creation of a text-based chess game in C#, a project that can serve as an invaluable asset for students. This endeavor can greatly assist with your C# assignment, delivering a practical and engaging approach to honing programming skills while concurrently tackling chess-related assignments.
Constructing this text-based chess game is an undertaking that encourages the development of essential programming proficiencies. It fosters the art of problem-solving and entails the design of algorithms to govern the intricate rules of chess. The project aligns seamlessly with object-oriented programming principles, wherein each chess piece becomes an object with distinctive characteristics and behaviors. Furthermore, it presents students with opportunities to test and debug their code rigorously, ensuring that the game adheres meticulously to the rules and functions as envisioned.
As players take alternating turns, they gain hands-on experience in managing user input and rendering the chessboard for a seamless gaming experience. This initiative not only imparts programming skills but also enriches logical thinking, fostering a deeper understanding of chess's strategic intricacies.
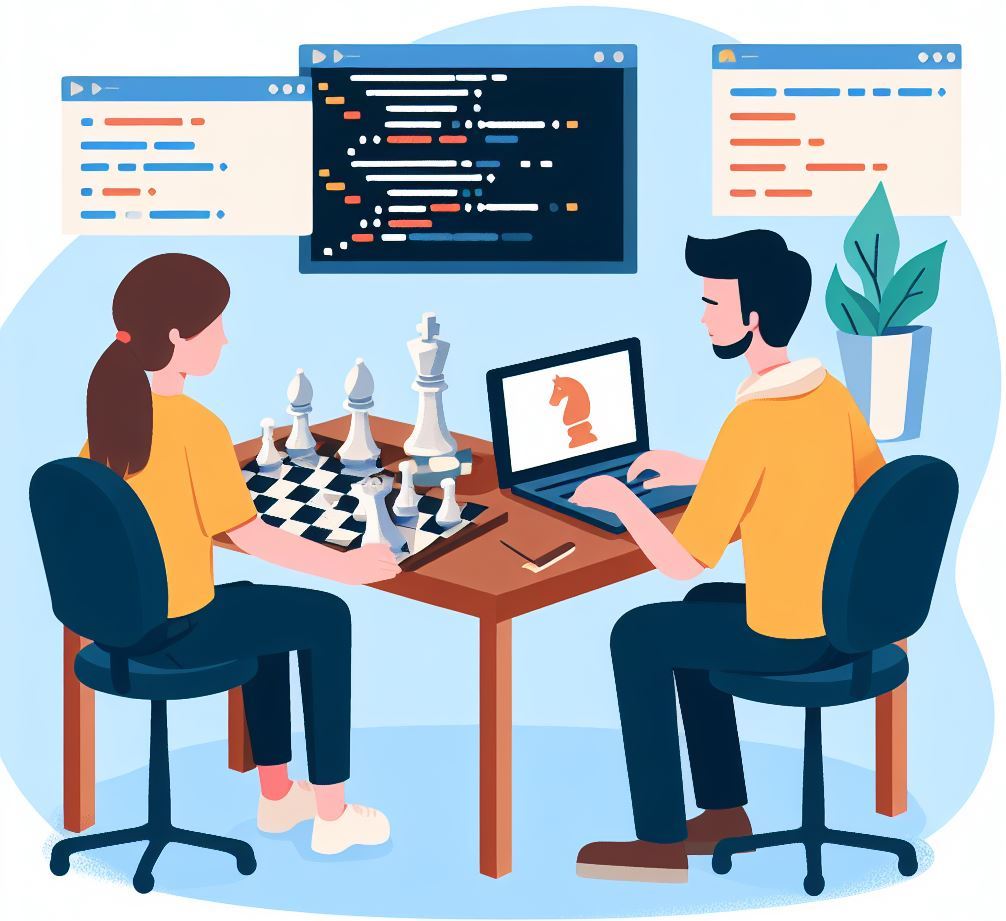
Why Create a Text-Based Chess Game?
Text-based games are an excellent way to introduce students to programming concepts and encourage them to think algorithmically. Chess, with its well-defined rules and complexity, is an ideal candidate for such a project. It offers a platform for students to dive into algorithm development, problem-solving, and object-oriented programming. By creating a text-based chess game, students can gain valuable hands-on experience in these critical aspects of programming, all while engaging with a classic and intellectually stimulating game. This project not only equips students with technical skills but also nurtures their ability to break down complex problems into manageable components. Additionally, the strategic nature of chess enriches their logical thinking and decision-making abilities, making it an educational and enjoyable endeavor for students seeking to enhance their programming skills. Here are some reasons why building a text-based chess game in C# can be beneficial for students:
- Problem Solving: Chess involves making strategic decisions and thinking ahead. Programming a chess game requires breaking down complex problems into smaller, manageable parts, fostering problem-solving skills. As students embark on this project, they'll confront the challenge of translating intricate chess rules into code. Whether it's validating a move, detecting a checkmate, or handling various game scenarios, each step demands logical thinking and creative solutions. These problem-solving skills extend beyond the realm of chess and become invaluable assets in tackling diverse programming challenges.
- Algorithm Design: Implementing chess rules like piece movements, captures, and checks involves designing algorithms. This project will give students hands-on experience in algorithm development. Crafting algorithms for chess moves and rule enforcement is akin to constructing a puzzle where each piece fits perfectly into the larger framework. Students will gain proficiency in breaking down complex actions into precise sequences, a skill transferrable to numerous programming contexts. By meticulously designing algorithms for each chess piece, they learn to think algorithmically, optimizing code efficiency and performance.
- Object-Oriented Programming (OOP): Chess naturally maps to OOP principles. Each chess piece can be represented as an object with specific attributes and behaviors, making it an excellent case study for OOP. In the chessboard's world, pieces like knights, bishops, and kings become distinct objects with properties and methods. This association between real-world entities and code structures helps students grasp the essence of object-oriented programming. They learn to model complex systems, encapsulate data, and utilize inheritance and polymorphism, all while working on a familiar and engaging project.
- Testing and Debugging: Debugging is a crucial skill for programmers. Developing a chess game will require testing and debugging to ensure the game follows the rules and behaves as expected. Students will learn the art of systematic testing, meticulously verifying each rule and move to guarantee that the game operates flawlessly. Debugging, a skill honed through this process, equips students to identify and rectify errors efficiently. This hands-on experience in troubleshooting and error resolution is a fundamental aspect of software development, applicable across various programming projects.
- User Interface: While this project focuses on the text-based version of chess, students can later expand it to include graphical elements or even create a web-based version. The versatility of this project allows students to explore user interface design and development. They can enhance the gaming experience by transitioning from a console-based interface to a visually engaging GUI or web application, providing opportunities to experiment with different technologies and design principles.
- Learning Through Play: Chess is an engaging and intellectually stimulating game. By programming a chess game, students can learn while having fun. The fusion of education and entertainment is a remarkable aspect of this project. Students not only gain programming skills but also enjoy the strategic challenges of chess. Learning through play fosters a deep understanding of the game's intricacies and a genuine passion for programming. It demonstrates that coding can be an enjoyable and rewarding endeavor, encouraging students to pursue further learning and creativity in the field.
Now that we understand the benefits, let's dive into the steps to create our text-based chess game."
Setting Up the Chessboard
The first step is to set up the chessboard. In a text-based version, you can represent the board using a two-dimensional array, where each cell holds information about the piece occupying it or if it's empty. Here's a simplified example of a 3x3 chessboard:
```csharp char[,] chessboard = new char[3, 3] { {'R', 'N', 'B'}, {'P', 'P', 'P'}, {' ', ' ', ' '} }; ```
In this example, 'R' represents a white rook, 'N' a white knight, 'B' a white bishop, and 'P' a white pawn. We use uppercase for white pieces and lowercase for black pieces. An empty cell is represented by a space (' '). This initial setup of the chessboard is crucial as it forms the foundation for all subsequent moves and interactions. Creating a clear and structured representation of the board is a fundamental part of the chess game's implementation, allowing players to visualize and strategize their moves effectively.
Implementing Chess Rules
Next, we need to implement the rules of chess. This includes how each piece moves, captures, and any special rules like castling or en passant. Here's a simplified example of how a knight's movement can be implemented:
```csharp public bool IsKnightMoveValid(int fromX, int fromY, int toX, int toY) { int dx = Math.Abs(toX - fromX); int dy = Math.Abs(toY - fromY); return (dx == 2 && dy == 1) || (dx == 1 && dy == 2); } ```
This code checks if the movement from (fromX, fromY) to (toX, toY) is valid for a knight. Implementing chess rules is a critical part of the project. It involves defining the allowed movements and constraints for each type of chess piece. By coding these rules, students gain insights into conditional logic and decision-making processes, reinforcing their understanding of programming fundamentals while simultaneously deepening their grasp of chess strategy and tactics. The knight's move validation example above illustrates the translation of a chess rule into code, showcasing how the principles of chess align with programming logic.
Managing Player Turns
To create a playable game, you need to manage player turns. Each player should take turns making moves. You can use a simple boolean variable to track whose turn it is:
```csharp bool isWhiteTurn = true; ``` Then, after each move, toggle the variable to switch turns: ```csharp isWhiteTurn = !isWhiteTurn; ```
Managing player turns is a fundamental aspect of creating a functional chess game. It ensures fair gameplay and adherence to the rules. By introducing this element, students learn about control flow in programming. They understand how to structure the game's logic to alternate between player turns and validate moves accordingly. This implementation also provides a foundation for more advanced features, such as detecting check and checkmate, where the order of moves becomes crucial. Moreover, it enhances their problem-solving skills as they address challenges related to turn management and gameplay flow, further reinforcing their programming expertise.
Handling User Input
To allow players to input their moves, you can use the Console.ReadLine() method to read input from the console. Parse the input to determine the source and destination squares. For example:
```csharp string input = Console.ReadLine(); int fromX = input[0] - 'A'; int from = input[1] - '1'; int toX = input[2] - 'A'; int toY = input[3] - '1'; ```
Handling user input is a critical component of interactive game development. In this phase of the project, students delve into input/output operations and user interaction, building essential skills for any software developer. They learn to read and process user commands, translating human-readable moves into actionable commands for the game. This experience not only reinforces their understanding of C# but also provides practical insights into user interface design and user experience considerations.
Displaying the Chessboard
After each move, display the updated chessboard to the players. You can use loops to iterate through the array and print the pieces. Here's a simplified example:
```csharp for (int y = 0; y < 8; y++) { for (int x = 0; x < 8; x++) { Console.Write(chessboard[x, y] + " "); } Console.WriteLine(); } ```
Displaying the chessboard is vital for providing players with a visual representation of the game state. It allows them to strategize and make informed decisions. Students acquire valuable experience in data visualization and presentation, skills applicable in a wide range of software development scenarios. Implementing this feature enhances their ability to convey information effectively through a user interface, whether it's a simple text-based display as shown or a more complex graphical representation in future iterations of the project.
Checkmate and Game Over
Implementing checkmate and game-over conditions is essential. Checkmate occurs when a player's king is in check, and there are no legal moves to remove the threat. Detecting checkmate can be complex, as it involves checking all possible moves and analyzing the board's state. This aspect of the project introduces students to the intricacies of game logic and decision-making algorithms. They delve into the challenge of evaluating the entire game board to identify critical situations like checkmate, which is an advanced problem-solving exercise.
The implementation of checkmate and game-over conditions not only enhances their programming skills but also underscores the importance of systematic thinking. Students learn to create algorithms that explore multiple scenarios, considering various piece movements and their consequences. This complexity underscores the depth of chess as a game of strategy and tactics. Additionally, it empowers students to develop their debugging and testing skills, ensuring the accuracy and reliability of their code as they strive to create a complete and functioning chess game.
Conclusion
Creating a text-based chess game in C# is a rewarding project that can help students develop essential programming skills while enjoying the game of chess. This project allows students to practice problem-solving, algorithm design, and object-oriented programming, all while creating a fun and educational tool.
This blog post has provided a foundational understanding of creating a text-based chess game, but the possibilities for expansion and improvement are vast. Students can enrich their projects by incorporating advanced chess features such as castling, en passant captures, pawn promotion, and rigorous move validation. Additionally, they have the opportunity to elevate the user experience by transitioning from a text-based interface to a graphical one or by introducing a sophisticated AI opponent.
Building a chess game represents an ideal fusion of passion and programming prowess for students. It not only harnesses their enthusiasm for games but also nurtures their coding skills, offering a platform to showcase their creativity and problem-solving abilities. Furthermore, this project serves as a valuable educational resource, aiding students in their C# assignments and providing a hands-on, engaging approach to mastering programming concepts and techniques.