Claim Your Offer
Unlock an amazing offer at www.programminghomeworkhelp.com with our latest promotion. Get an incredible 10% off on your all programming assignment, ensuring top-quality assistance at an affordable price. Our team of expert programmers is here to help you, making your academic journey smoother and more cost-effective. Don't miss this chance to improve your skills and save on your studies. Take advantage of our offer now and secure exceptional help for your programming assignments.
We Accept
- What is a Chatbot?
- Why Build a Chatbot for a College Project?
- Step 1: Setting Up the Environment
- Step 2: Building the Chatbot
- Step 3: Adding a Web Interface with Flask
- Step 4: Advanced: Training the Chatbot with Machine Learning
- Step 5: Testing and Deployment
- Conclusion
In this blog, we’ll dive into how you can create a chatbot from scratch using Python and Natural Language Processing (NLP). Whether you’re a student tackling this project for your college assignment, searching for someone to do your Python assignment, or simply exploring the world of AI, this guide will provide you with step-by-step instructions and plenty of code examples. Plus, if you need extra help, services like Programming Assignment Help can make your life easier when you’re stuck.
What is a Chatbot?
A chatbot is an AI-powered program designed to simulate human conversation. It can respond to queries, provide information, and assist users in various tasks. From simple keyword matching to advanced machine learning models, chatbots vary in complexity.
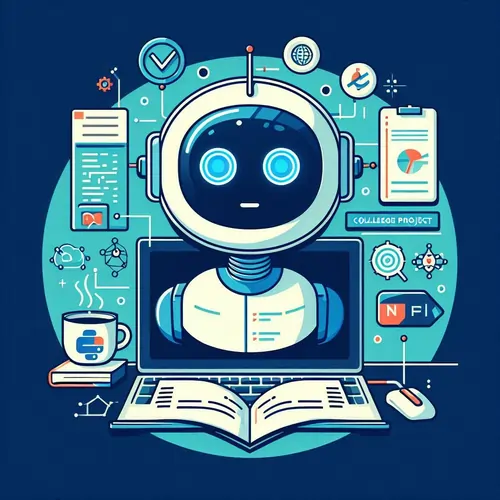
Why Build a Chatbot for a College Project?
- Hands-On Learning: It helps you apply Python and NLP concepts practically.
- Career Readiness: Chatbot development is a highly sought-after skill in tech industries.
- Customizable Scope: You can create a simple or complex bot based on your assignment requirements.
Step 1: Setting Up the Environment
To build a chatbot, you’ll need the following tools:
- Python: The programming language for implementation.
- NLTK (Natural Language Toolkit): For basic NLP tasks.
- Flask: To create a simple web interface for the chatbot.
Install Required Libraries
pip install nltk flask numpy pandas
Step 2: Building the Chatbot
We’ll create a simple rule-based chatbot with an optional extension to an AI-based model using NLP.
Rule-Based Chatbot
- Define Keywords and Responses
Start by creating a dictionary where keywords map to appropriate responses.
responses = { "hello": "Hi there! How can I assist you today?", "help": "I'm here to help! What do you need assistance with?", "bye": "Goodbye! Have a great day!", "default": "I'm sorry, I didn't understand that. Could you rephrase?" }
- Basic Chatbot Logic
Write a function to process user input and return a response.
def chatbot_response(user_input): user_input = user_input.lower() for keyword in responses: if keyword in user_input: return responses[keyword] return responses["default"] # Test the chatbot while True: user_input = input("You: ") if user_input.lower() == "bye": print("Bot:", chatbot_response(user_input)) break print("Bot:", chatbot_response(user_input))
Enhancing the Chatbot with NLP
For more advanced functionality, let’s use NLP techniques to process user input.
- Tokenization and Lemmatization
Tokenization breaks sentences into words, while lemmatization reduces words to their base forms.
import nltk from nltk.tokenize import word_tokenize from nltk.stem import WordNetLemmatizer nltk.download('punkt') nltk.download('wordnet') lemmatizer = WordNetLemmatizer() def preprocess_input(user_input): tokens = word_tokenize(user_input) return [lemmatizer.lemmatize(token.lower()) for token in tokens]
- Cosine Similarity for Matching
We’ll compare the similarity between user input and predefined responses using TF-IDF Vectorization.
from sklearn.feature_extraction.text import TfidfVectorizer from sklearn.metrics.pairwise import cosine_similarity responses_list = [ "Hi there! How can I assist you today?", "I'm here to help! What do you need assistance with?", "Goodbye! Have a great day!" ] def find_best_response(user_input): vectorizer = TfidfVectorizer() tfidf = vectorizer.fit_transform([user_input] + responses_list) similarity_scores = cosine_similarity(tfidf[0], tfidf[1:]) best_match = similarity_scores.argmax() return responses_list[best_match] # Test the NLP chatbot user_input = "Can you help me?" response = find_best_response(user_input) print("Bot:", response)
Step 3: Adding a Web Interface with Flask
To make your chatbot interactive, you can deploy it using Flask.
- Flask Application
Create a Flask app to handle user input and return chatbot responses.
from flask import Flask, request, jsonify app = Flask(__name__) @app.route('/chat', methods=['POST']) def chat(): user_input = request.json.get('message') response = chatbot_response(user_input) return jsonify({"response": response}) if __name__ == "__main__": app.run(debug=True)
- Test the API
Use tools like Postman to send messages and receive responses.
Step 4: Advanced: Training the Chatbot with Machine Learning
For an AI-based chatbot, you can train it on a dataset of conversations.
- Dataset Preparation
Find or create a dataset with question-answer pairs.
import pandas as pd # Example dataset data = { "question": ["hello", "help", "bye"], "response": ["Hi there!", "How can I assist you?", "Goodbye!"] } df = pd.DataFrame(data)
- Train a Classification Model
Use an ML model to classify user input.
from sklearn.feature_extraction.text import CountVectorizer from sklearn.naive_bayes import MultinomialNB vectorizer = CountVectorizer() X = vectorizer.fit_transform(df["question"]) y = df["response"] model = MultinomialNB() model.fit(X, y) def ai_chatbot_response(user_input): input_vec = vectorizer.transform([user_input]) return model.predict(input_vec)[0] # Test the ML chatbot print("Bot:", ai_chatbot_response("hello"))
Step 5: Testing and Deployment
- Test the chatbot thoroughly.
- Deploy the Flask app to a platform like Heroku for public access.
Conclusion
Creating a chatbot from scratch is an exciting project that allows you to explore Python, NLP, and machine learning. Whether you’re building a basic rule-based bot or an advanced AI model, this project is a great way to enhance your skills.
If you need additional assistance with your chatbot or similar projects, don’t hesitate to reach out to Programming Assignment Help. Our team is here to guide you and help you ace your college assignments. Got stuck? Simply ask, “Can you do my programming assignment for me?”, and we’ll provide expert assistance.