Claim Your Offer
Unlock an amazing offer at www.programminghomeworkhelp.com with our latest promotion. Get an incredible 10% off on your all programming assignment, ensuring top-quality assistance at an affordable price. Our team of expert programmers is here to help you, making your academic journey smoother and more cost-effective. Don't miss this chance to improve your skills and save on your studies. Take advantage of our offer now and secure exceptional help for your programming assignments.
We Accept
- Understanding Memory Leaks in Programming
- Causes of Memory Leaks
- Identifying Memory Leaks in Your Code
- Understanding Manual Memory Management in C/C++
- Avoiding Memory Leaks in Java
- Memory Leak Detection Techniques
- Coding Practices to Prevent Memory Leaks
- Testing for Memory Leaks
- Conclusion
Memory management is a critical aspect of programming, directly influencing the efficiency, reliability, and performance of software applications. Improper handling of memory can lead to memory leaks, which occur when a program consumes more memory than necessary, ultimately leading to slower execution or system crashes. For students working on programming assignments, mastering memory management skills is essential. In this article, we’ll explore the causes of memory leaks, discuss how to detect and handle them, and outline best practices for avoiding them in programming assignments.
Whether you are new to programming or a seasoned coder, this guide will give you practical insights to improve your code. If you encounter any issues with memory leaks or require expert help, remember that programming assignment help is available to guide you through the process. Let’s dive into understanding and preventing memory leaks.
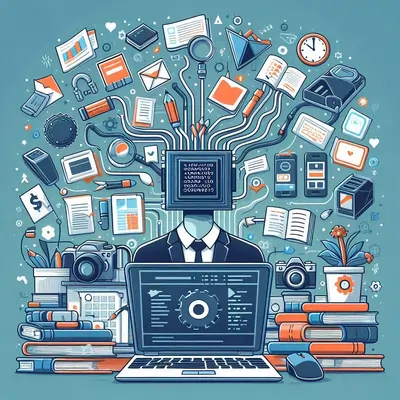
Understanding Memory Leaks in Programming
Memory leaks happen when a program allocates memory that is no longer needed but fails to release it, causing a buildup of unused memory. Over time, this can lead to system slowdowns, crashes, and memory exhaustion, especially in long-running programs.
The problem of memory leaks is prevalent in languages with manual memory management, such as C and C++, where developers must allocate and free memory explicitly. While garbage-collected languages like Java, Python, and C# mitigate this issue to some extent, they are not immune. Recognizing where memory leaks originate and implementing strategies to avoid them is key to writing efficient code.
Causes of Memory Leaks
Memory leaks can occur for various reasons, including:
- Poorly Managed Dynamic Memory Allocation: In languages like C and C++, failing to deallocate memory using free() (C) or delete (C++) after allocation with malloc() or new is a common cause.
- Unreleased References in Garbage-Collected Languages: In languages like Java or Python, if objects are held by references even after they are no longer needed, garbage collection cannot reclaim their memory.
- Unintentional Global Variables: Declaring variables globally when they are only needed locally can cause memory issues, as these variables persist for the program’s lifetime.
- Circular References: In garbage-collected languages, circular references can prevent garbage collection, causing memory to accumulate unintentionally. For example, two objects referencing each other cannot be collected, even if they’re no longer used.
- Complex Data Structures: Improperly managed structures, such as linked lists or trees, where not all nodes are freed after use, can lead to memory leaks.
Identifying Memory Leaks in Your Code
Before fixing memory leaks, you must be able to detect them. Here are a few approaches and tools commonly used to find memory leaks:
- Code Review and Manual Inspection: Reviewing your code is often the first step in identifying memory issues. Checking that each dynamically allocated object has a corresponding deallocation statement can prevent leaks.
- Memory Profiling Tools:
- Valgrind (Linux): Valgrind’s memcheck tool is widely used to detect memory leaks in C and C++ programs. It shows unfreed memory blocks, helping identify allocation and deallocation issues.
- Visual Studio Profiler (Windows): For C++ developers on Windows, Visual Studio includes a diagnostic tool to check for memory leaks.
- Java VisualVM (Java): For Java applications, VisualVM is useful for identifying memory leaks by visualizing object retention in memory.
- Python’s Tracemalloc: Python’s tracemalloc module can help track memory allocations and identify leaks by capturing and comparing memory snapshots.
- Automated Testing and Static Analysis Tools: Tools like Clang Static Analyzer or CodeSonar analyze code for memory issues before runtime, allowing developers to spot potential leaks during the coding phase.
- Using Logging and Debugging Techniques: Adding logs to your code, especially around memory allocations and deallocations, can give insights into where leaks occur.
Understanding Manual Memory Management in C/C++
In languages like C and C++, dynamic memory allocation is typically managed with malloc and free (in C) or new and delete (in C++). Here’s a quick example:
#include <iostream>
void memoryLeakExample() {
int* ptr = new int[100]; // Allocates memory for an array of 100 integers
// Process data
// Forgetting to delete the memory leads to a leak
}
int main() {
memoryLeakExample();
return 0;
}
In the above code, a memory leak occurs because the dynamically allocated memory using new is not freed. To fix this, add a delete[] statement:
void memoryLeakExample() {
int* ptr = new int[100];
// Process data
delete[] ptr; // Frees memory
}
Tips to Avoid Leaks in C/C++:
- Always Match Allocation and Deallocation: If you allocate memory with new, make sure to deallocate it with delete.
- Use Smart Pointers: Smart pointers in C++ (e.g., std::unique_ptr and std::shared_ptr) automatically handle memory deallocation.
Example with std::unique_ptr:
#include <memory>
void smartPointerExample() {
std::unique_ptr<int[]> ptr(new int[100]); // Memory will be automatically released
}
Smart pointers are ideal when you want automatic memory management and are especially useful in larger projects. Many students seeking online programming assignment help often benefit from understanding smart pointers to avoid leaks.
Avoiding Memory Leaks in Java
In Java, memory management is generally handled by the Garbage Collector (GC). However, memory leaks can still occur through improper references, especially with static variables and inner classes.
Example of a Memory Leak in Java
Consider the following code where an inner class is used:
import java.util.ArrayList;
import java.util.List;
class MemoryLeakExample {
private List<String> dataList = new ArrayList<>();
public void addData() {
dataList.add("data"); // Adds data continuously
}
}
If the dataList is a long-lived static variable, it can lead to memory leaks since it won’t be eligible for garbage collection.
Tips to Avoid Leaks in Java:
- Avoid Static Variables: Use local variables instead of static ones where possible.
- Clear Unused Data: Explicitly set objects to null when you’re done with them if they hold significant memory.
- Use Weak References: Use WeakReference for objects that can be garbage-collected if no strong references exist.
WeakReference<String> weakRef = new WeakReference<>(new String("Temporary data"));
Memory Leak Detection Techniques
Detecting memory leaks early can save you from troubleshooting performance issues later. Here are some tools and techniques for detecting memory leaks in programming assignments.
Tools for Memory Leak Detection
- Valgrind: Valgrind is a popular tool for memory leak detection in C/C++. It analyzes memory allocations and deallocations and points out leaks.
Example usage of Valgrind:
valgrind --leak-check=full ./program
- Java VisualVM: For Java, VisualVM provides a memory profiling tool that can help identify leaks by monitoring heap usage.
- Python Memory Profiler: In Python, memory_profiler helps in tracking memory usage line by line.
Coding Practices to Prevent Memory Leaks
To prevent memory leaks, you need to adopt certain practices while writing code. Here are some programming techniques that can make a difference.
- Use RAII in C++
Resource Acquisition Is Initialization (RAII) is a principle in C++ that ensures resources are properly released when an object goes out of scope.
#include <iostream> #include <fstream> class FileHandler { public: FileHandler(const std::string& filename) { file.open(filename); } ~FileHandler() { if (file.is_open()) { file.close(); } } private: std::fstream file; };
The FileHandler class above ensures that the file resource is released in the destructor, reducing the risk of memory leaks.
- Avoid Circular References in Java
In Java, circular references in collections or inner classes can prevent the GC from reclaiming memory.
import java.util.ArrayList; import java.util.List; class Node { Node next; } public class CircularReference { public static void main(String[] args) { Node node1 = new Node(); Node node2 = new Node(); node1.next = node2; node2.next = node1; // Circular reference } }
To prevent leaks, avoid circular references or use WeakReference where applicable.
Testing for Memory Leaks
Testing is crucial to detect memory leaks before they become an issue. Here are some testing strategies:
- Use Unit Tests with Valgrind: Integrate Valgrind in your unit tests to monitor memory usage continuously.
- Monitor Resource Usage: Run your program on loop or stress tests and monitor memory usage over time.
Example of a simple memory test in Python:
import tracemalloc
def memory_leak_test():
tracemalloc.start()
# Your function calls here
snapshot = tracemalloc.take_snapshot()
top_stats = snapshot.statistics('lineno')
print(top_stats[:10]) # Prints top 10 memory usage lines
memory_leak_test()
Conclusion
Memory management can be tricky, but mastering it will improve the efficiency and reliability of your code. By adopting careful practices, using smart pointers in C++, avoiding circular references in Java, and regularly testing for memory leaks, you can ensure that your assignments are leak-free.
If you’re working on an assignment and feel unsure about managing memory, consider reaching out to programming assignment help resources. Many online programming assignment help services provide guidance and can help you understand complex concepts in memory management, giving you an edge in mastering these skills.
Remember, learning to handle memory leaks not only helps in academic projects but is also a valuable skill in professional software development.