Claim Your Offer
Unlock an amazing offer at www.programminghomeworkhelp.com with our latest promotion. Get an incredible 20% off on your second programming assignment, ensuring top-quality assistance at an affordable price. Our team of expert programmers is here to help you, making your academic journey smoother and more cost-effective. Don't miss this chance to improve your skills and save on your studies. Take advantage of our offer now and secure exceptional help for your programming assignments.
We Accept
- Why Practice Golang Assignments?
- Benefits of Golang Assignment Practice
- Solidify Core Concepts
- Build Problem-Solving Skills
- Prepare for Real-world Scenarios
- Example 1: Golang Concurrency
- Assignment
- Example 2: Golang Interfaces
- Assignment:
- Example 3: Golang Error Handling
- Assignment:
- Example 4: Golang Web Application
- Assignment:
- Conclusion
Golang, commonly known as Go, has garnered widespread acclaim in recent years owing to its straightforward syntax, high efficiency, and robust support for concurrent programming. For individuals venturing into Golang programming with a desire to bolster their skills through practical applications, this blog provides an opportune platform. Within these digital pages, we embark on a journey to explore a myriad of Golang assignment examples, strategically curated to facilitate a comprehensive understanding of the language's intricacies through hands-on experiences. Whether you are a novice eager to grasp the fundamentals or an adept developer seeking to refine your expertise, the blog promises a valuable resource for solving your Golang assignment and honing Golang proficiency. Join us as we navigate through real-world scenarios and practical problem-solving, unlocking the potential for enriched learning and mastery of Golang programming concepts.
Why Practice Golang Assignments?
Understanding the significance of practicing Golang assignments is paramount before delving into specific examples. The rationale behind this emphasis lies in the recognition that coding, as a skill, is most effectively acquired through direct, hands-on experience. Golang, with its unique characteristics and concurrent programming capabilities, is no exception. Engaging with real-world problems through assignments serves a dual purpose—it not only reinforces theoretical concepts but also fosters the development of indispensable problem-solving skills crucial for every developer. The practical application of coding principles in assignments serves as a bridge between theory and real-world scenarios, providing a dynamic learning environment where developers can navigate complexities and fine-tune their analytical abilities. In essence, the practice of Golang assignments is not merely an exercise in code-writing; it is a deliberate and strategic approach to cultivating a well-rounded, problem-solving mindset that is instrumental in the ever-evolving landscape of software development.
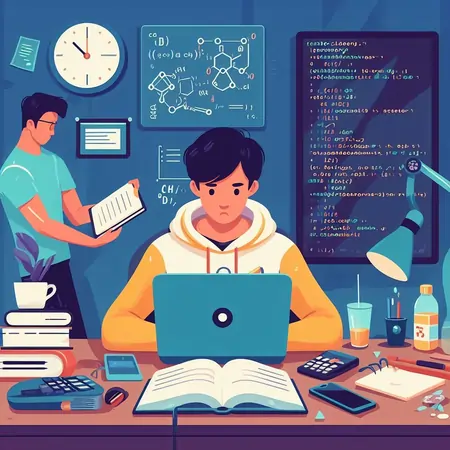
Benefits of Golang Assignment Practice
Engaging in Golang assignment practice yields a spectrum of benefits that extend beyond mere code proficiency. As developers navigate through the intricacies of assignments, they solidify core Golang concepts, such as goroutines and interfaces, fostering a deeper understanding of the language's nuances. This hands-on approach not only reinforces theoretical knowledge but also cultivates invaluable problem-solving skills essential for navigating real-world coding challenges. Beyond theoretical comprehension, Golang assignment practice prepares developers for practical scenarios, aligning their skills with industry demands. The dynamic nature of Golang, known for its simplicity and efficiency, becomes more apparent as developers grapple with diverse assignments, ensuring a holistic grasp of the language. Moreover, consistent practice enhances one's ability to design scalable and efficient solutions, a crucial skill in the evolving landscape of software development. Ultimately, the benefits of Golang assignment practice transcend the confines of conventional learning, empowering developers with a practical skill set poised for success in Golang programming.
Solidify Core Concepts
Assignments serve as a robust tool for solidifying core Golang concepts, providing a structured environment to reinforce fundamental principles. By working through problems that involve goroutines, channels, and interfaces, developers gain hands-on experience that goes beyond theoretical understanding. This practical application of knowledge not only cements foundational concepts but also allows for a more nuanced comprehension of Golang's unique features. As developers tackle assignments, they navigate the intricacies of concurrent programming and interface implementation, building a strong foundation that is essential for mastery of the language.
Build Problem-Solving Skills
The essence of Golang assignment practice lies in its ability to cultivate adept problem-solving skills. Real-world problems necessitate a multifaceted approach, requiring developers to analyze, design, and implement solutions efficiently. Through the iterative process of grappling with diverse assignments, individuals refine their ability to break down complex problems into manageable components. This iterative problem-solving not only enhances their coding proficiency but also instills a mindset geared towards tackling challenges methodically. As developers confront a variety of problem domains, from concurrency issues to interface implementations, they are equipped with the analytical and creative skills crucial for success in the ever-evolving landscape of software development.
Prepare for Real-world Scenarios
Golang's extensive use in the industry underscores the practical relevance of assignment practice. By engaging with assignments that mirror real-world scenarios, developers proactively prepare for the challenges they might encounter in professional projects. This pragmatic approach ensures that the skills acquired through Golang assignment practice seamlessly translate into the demands of real-world development. Whether it's optimizing code for performance, managing concurrent processes, or implementing robust interfaces, the challenges posed by assignments reflect the intricacies of Golang application in industry settings. Consequently, developers emerge from this practice better equipped to navigate the complexities of real-world Golang programming, bridging the gap between theoretical knowledge and practical application.
Example 1: Golang Concurrency
Concurrency stands as a pivotal feature in Golang, emphasizing the importance of mastering goroutines and channels. To illustrate this, let's delve into a straightforward example involving concurrent tasks. The assignment prompts the creation of a Golang program tasked with calculating the factorial of a specified number concurrently, employing goroutines and channels for effective implementation.
Assignment
The assignment at hand challenges developers to craft a Golang program capable of calculating the factorial of a given number concurrently, utilizing goroutines. The incorporation of channels becomes crucial for collecting and displaying the results seamlessly.
Solution:
```go package main import ( "fmt" "sync" ) func factorial(n int, wg *sync.WaitGroup, ch chan int) { defer wg.Done() result := 1 for i := 1; i <= n; i++ { result *= i } ch <- result } func main() { num := 5 var wg sync.WaitGroup ch := make(chan int) wg.Add(1) go factorial(num, &wg, ch) wg.Wait() close(ch) result := <-ch fmt.Printf("Factorial of %d is %d\n", num, result) } ```
In the ensuing example, we leverage goroutines to concurrently compute the factorial. The integration of sync.WaitGroup ensures synchronization, compelling the main goroutine to await the completion of concurrent tasks. Furthermore, a channel facilitates the organized collection and display of the computed results, showcasing a practical application of Golang's concurrency features. This hands-on exercise not only reinforces theoretical knowledge but also exemplifies the practical utility of concurrent programming in Golang.
Example 2: Golang Interfaces
Golang interfaces stand as powerful constructs facilitating polymorphism, providing a versatile means to achieve abstraction. In this example, we embark on an assignment that delves into the implementation of interfaces for various geometric shapes, showcasing the practical utility of Golang's interface feature.
Assignment:
The assignment prompts the creation of a Golang program designed to define an interface named Shape incorporating methods Area() and Perimeter(). Developers are challenged to implement this interface for three distinct geometric shapes: circle, rectangle, and triangle.
Solution:
```go package main import ( "fmt" "math" ) // Shape interface type Shape interface { Area() float64 Perimeter() float64 } // Circle type type Circle struct { Radius float64 } // Rectangle type type Rectangle struct { Width float64 Height float64 } // Triangle type type Triangle struct { Side1, Side2, Side3 float64 } // Implementing Shape interface for Circle func (c Circle) Area() float64 { return math.Pi * c.Radius * c.Radius } func (c Circle) Perimeter() float64 { return 2 * math.Pi * c.Radius } // Implementing Shape interface for Rectangle func (r Rectangle) Area() float64 { return r.Width * r.Height } func (r Rectangle) Perimeter() float64 { return 2*r.Width + 2*r.Height } // Implementing Shape interface for Triangle func (t Triangle) Area() float64 { s := (t.Side1 + t.Side2 + t.Side3) / 2 return math.Sqrt(s * (s - t.Side1) * (s - t.Side2) * (s - t.Side3)) } func (t Triangle) Perimeter() float64 { return t.Side1 + t.Side2 + t.Side3 } func printShapeDetails(s Shape) { fmt.Printf("Area: %.2f, Perimeter: %.2f\n", s.Area(), s.Perimeter()) } func main() { circle := Circle{Radius: 5} rectangle := Rectangle{Width: 3, Height: 4} triangle := Triangle{Side1: 5, Side2: 12, Side3: 13} printShapeDetails(circle) printShapeDetails(rectangle) printShapeDetails(triangle) } ```
In this illustrative example, we witness the seamless use of interfaces to establish a unified API for diverse geometric shapes. The Golang program showcases the implementation of the Shape interface for specific shapes, namely circle, rectangle, and triangle. The incorporation of the printShapeDetails function further emphasizes the dynamic nature of Golang interfaces, as it can accept any shape adhering to the Shape interface. This example not only underscores the elegance of Golang interfaces in achieving abstraction but also highlights their practical application in creating scalable and adaptable code structures for different geometric entities.
Example 3: Golang Error Handling
Assignment:
Create a Golang program that reads a file and handles errors effectively. Implement a custom error type for a specific file-related issue and ensure the program gracefully handles various error scenarios, providing informative messages.
Solution:
```go package main import ( "fmt" "os" ) // CustomFileError represents a custom file-related error type CustomFileError struct { FileName string Err error } func (e CustomFileError) Error() string { return fmt.Sprintf("File Error (%s): %v", e.FileName, e.Err) } func readFile(fileName string) ([]byte, error) { file, err := os.Open(fileName) if err != nil { return nil, CustomFileError{FileName: fileName, Err: err} } defer file.Close() data := make([]byte, 1024) _, err = file.Read(data) if err != nil { return nil, CustomFileError{FileName: fileName, Err: err} } return data, nil } func main() { fileName := "nonexistent.txt" data, err := readFile(fileName) if err != nil { fmt.Println(err) return } fmt.Printf("File content: %s\n", data) } ```
In this example, the Golang program addresses the crucial aspect of error handling when dealing with file operations. The assignment prompts the creation of a program that reads a file and implements effective error handling. The solution introduces a custom error type, CustomFileError, specifically tailored for file-related issues. The readFile function is designed to read the content of a file, and in case of any errors, it returns a CustomFileError containing detailed information about the encountered issue. The main function demonstrates the usage of this error handling mechanism when attempting to read a non-existent file. By providing meaningful error messages, this example showcases a robust approach to handling errors in Golang file operations.
Example 4: Golang Web Application
Assignment:
Develop a simple Golang web application using the "net/http" package. Create two routes - one for displaying a welcome message and another for handling a form submission. Implement form validation to ensure all required fields are filled, and display an appropriate message.
Solution:
```go package main import ( "fmt" "html/template" "net/http" ) // PageData represents data to be displayed in the template type PageData struct { Message string } func welcomeHandler(w http.ResponseWriter, r *http.Request) { data := PageData{Message: "Welcome to the Golang Web App!"} renderTemplate(w, "welcome.html", data) } func formHandler(w http.ResponseWriter, r *http.Request) { if r.Method != http.MethodPost { http.Error(w, "Method Not Allowed", http.StatusMethodNotAllowed) return } err := r.ParseForm() if err != nil { http.Error(w, "Internal Server Error", http.StatusInternalServerError) return } name := r.FormValue("name") if name == "" { http.Error(w, "Name is required", http.StatusBadRequest) return } data := PageData{Message: fmt.Sprintf("Hello, %s! Form submitted successfully.", name)} renderTemplate(w, "form.html", data) } func renderTemplate(w http.ResponseWriter, tmpl string, data PageData) { tmpl, err := template.New("").ParseFiles(tmpl) if err != nil { http.Error(w, "Internal Server Error", http.StatusInternalServerError) return } err = tmpl.ExecuteTemplate(w, tmpl, data) if err != nil { http.Error(w, "Internal Server Error", http.StatusInternalServerError) return } } func main() { http.HandleFunc("/welcome", welcomeHandler) http.HandleFunc("/form", formHandler) fmt.Println("Server is running on http://localhost:8080") http.ListenAndServe(":8080", nil) } ```
This solution focuses on creating a simple Golang web application using the "net/http" package. The assignment requires the implementation of two routes: one for displaying a welcome message and another for handling a form submission. The welcomeHandler function renders a welcome message using a template, while the formHandler function manages the form submission, ensuring that the required fields are filled. The form validation is implemented to catch errors and provide appropriate HTTP responses. The renderTemplate function is introduced to streamline the template rendering process. This example highlights the creation of a basic web application with Golang, emphasizing routing, form handling, and error management for a cohesive user experience.
Conclusion
In conclusion, immersing oneself in Golang assignments proves to be a highly effective strategy for mastering the language and unlocking its distinctive features. This blog has delved into two illustrative examples, each concentrating on essential aspects of Golang—concurrency and interfaces. Through hands-on engagement with these examples, readers can cultivate a profound comprehension of Golang, equipping themselves with the skills needed to tackle real-world coding challenges with confidence. The emphasis here is on the transformative power of consistent practice and direct application of knowledge, underscoring that proficiency in Golang is best attained through an ongoing commitment to learning by doing. As you embark on your coding endeavors, keep in mind that the journey to becoming adept in Golang is marked by the continuous refinement of skills through practical experience. Happy coding, and may your programming ventures be both enlightening and fulfilling!