Claim Your Discount Today
Kick off the fall semester with a 20% discount on all programming assignments at www.programminghomeworkhelp.com! Our experts are here to support your coding journey with top-quality assistance. Seize this seasonal offer to enhance your programming skills and achieve academic success. Act now and save!
We Accept
- Understanding JavaScript Arrays
- What Are Arrays?
- Declaring and Initializing Arrays
- Accessing Array Elements
- Leveraging Loops in JavaScript
- Introduction to Loops
- The For Loop: Iterating with Precision
- The While Loop: Conditional Iteration
- The ForEach Loop: Simplifying Iteration
- Simplifying Complex Assignments with Arrays and Loops
- Sorting Arrays
- Filtering Arrays
- Mapping Arrays
- Best Practices and Pitfalls to Avoid
- Best Practices
- Pitfalls to Avoid
- Conclusion
In the realm of programming, the symbiotic relationship between arrays and loops is integral, serving as the bedrock for developers to efficiently handle, manipulate, and traverse data. JavaScript, known for its versatility, stands out by offering robust support for arrays and loops, thereby providing a powerful toolkit for simplifying intricate assignments. This blog post is poised to delve deep into the nuanced world of JavaScript arrays and loops, unraveling their multifaceted functionalities and showcasing their prowess in streamlining complex tasks. The introductory passage aptly captures the essence of this exploration, emphasizing the intrinsic value of these programming constructs and alluding to the transformative capabilities they bring to the table. From this point of departure, the narrative is poised to unfold, shedding light on the finer details of array and loop manipulation, demonstrating not only their technical intricacies but also their practical applications through illustrative examples and real-world scenarios. As we embark on this journey, the aim is to equip readers with a comprehensive understanding of how JavaScript arrays and loops form the backbone of effective programming, making seemingly convoluted assignments more manageable through elegant and efficient solutions. Whether you're looking to solve your JavaScript assignment or simply enhance your coding proficiency, this exploration promises to provide valuable insights into the powerful world of arrays and loops.
Understanding JavaScript Arrays
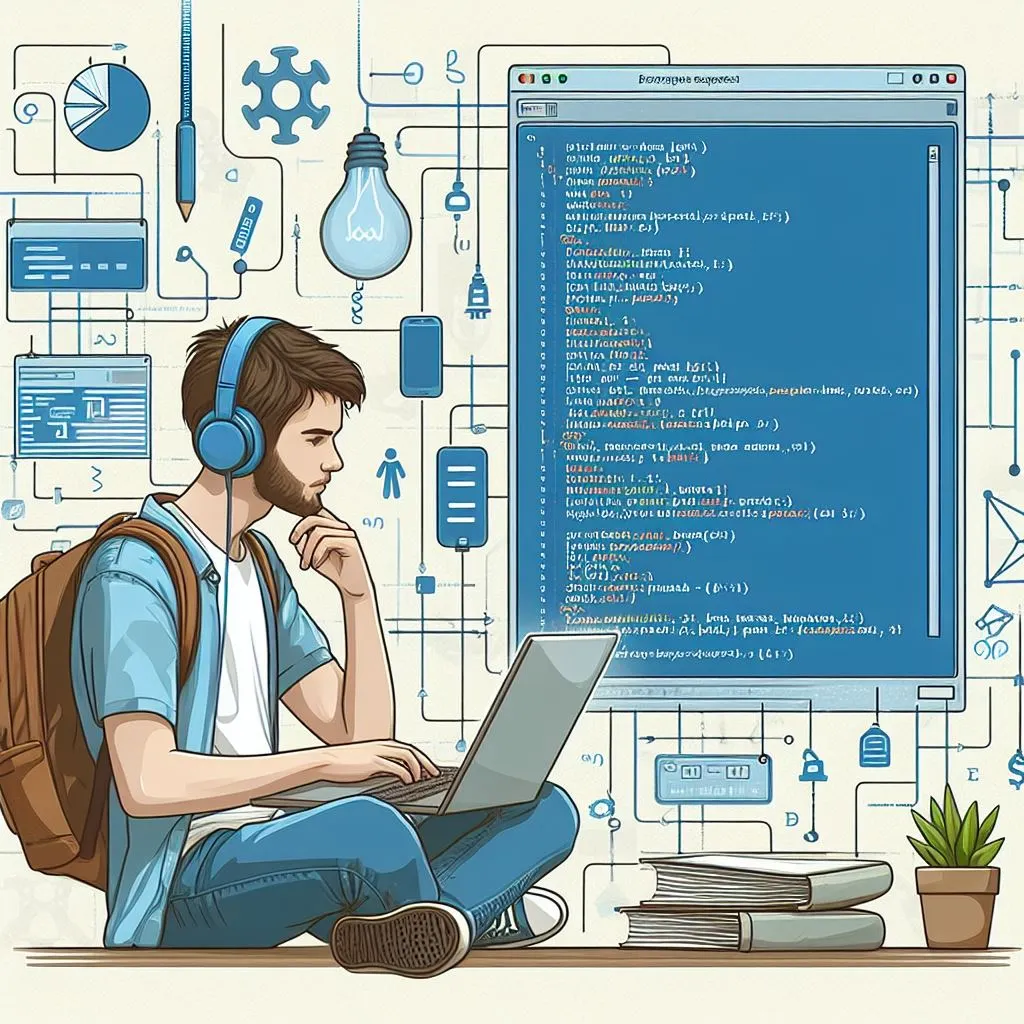
In the intricate ecosystem of JavaScript programming, understanding arrays stands as a cornerstone for developers aiming to harness the language's full potential. Arrays in JavaScript transcend mere data structures; they serve as dynamic containers, enabling the storage and organization of multiple values within a single entity. Grasping the intricacies of JavaScript arrays involves delving into their foundational principles, including declaration, initialization, and manipulation. As developers navigate the complexities of array functionalities, they encounter a myriad of methods and techniques tailored for efficient data management and retrieval. Furthermore, understanding JavaScript arrays facilitates seamless integration with other programming constructs, fostering cohesive and robust codebases. By immersing oneself in the nuances of array operations, developers can optimize performance, enhance scalability, and streamline development processes. Thus, comprehending JavaScript arrays transcends theoretical knowledge; it empowers developers to craft elegant solutions, drive innovation, and navigate the multifaceted landscape of modern web development with confidence and proficiency.
What Are Arrays?
An array in JavaScript serves as a foundational data structure designed to accommodate multiple values within a singular variable. This capability transcends the boundaries of data types, allowing developers to amalgamate heterogeneous elements—ranging from primitive types such as numbers and strings to complex entities like objects and nested arrays—within a cohesive structure. The versatility inherent to arrays in JavaScript underpins their ubiquity across diverse programming contexts, enabling developers to construct organized, scalable data repositories that facilitate efficient data manipulation, retrieval, and iteration. Moreover, arrays encapsulate a hierarchical paradigm, empowering developers to implement multidimensional data models that align with complex application requirements, thereby fostering modularity, extensibility, and maintainability within codebases.
Declaring and Initializing Arrays
The process of creating an array in JavaScript adheres to a streamlined syntax, leveraging square brackets [] as delimiters to encapsulate array elements. This declarative approach transcends mere syntactic conventions, serving as a catalyst for dynamic array instantiation and population. By employing a comma-separated list of values within the brackets, developers can initialize arrays with predetermined elements, thereby establishing a foundational framework for subsequent data manipulation operations. Furthermore, the declarative nature of array initialization in JavaScript fosters code clarity and conciseness, enabling developers to articulate data structures with precision while adhering to established coding standards and best practices.
let fruits = ['apple', 'banana', 'cherry'];
Accessing Array Elements
Arrays in JavaScript adhere to a zero-based indexing paradigm, wherein the first element occupies the index position 0, followed by subsequent elements in sequential order. This indexing convention underscores the array's intrinsic hierarchical structure, facilitating granular element retrieval and manipulation through targeted index references. By leveraging index-based access mechanisms, developers can interact with array elements in a deterministic manner, thereby enabling precise data extraction, modification, and traversal operations. Additionally, the zero-indexed nature of arrays fosters computational efficiency, as it mitigates the need for complex offset calculations, streamlining algorithmic implementations and enhancing code performance within diverse programming contexts.
console.log(fruits[0]); // Output: apple
Leveraging Loops in JavaScript
Navigating the realm of JavaScript programming necessitates a profound understanding of loops, pivotal constructs that facilitate repetitive execution and iterative operations within codebases. Loops in JavaScript transcend mere procedural constructs; they embody the essence of automation, enabling developers to iterate through data structures, execute conditional statements, and implement dynamic functionalities with precision and efficiency. Grasping the intricacies of JavaScript loops involves exploring a diverse array of loop types, each tailored to specific scenarios and requirements. As developers delve deeper into the realm of loop functionalities, they unlock a plethora of techniques and methodologies designed to enhance code readability, optimize performance, and foster modular development practices. Furthermore, leveraging loops in JavaScript empowers developers to streamline complex algorithms, automate repetitive tasks, and orchestrate intricate workflows seamlessly. Thus, mastering the art of JavaScript loops transcends technical proficiency; it cultivates a mindset of innovation, efficiency, and adaptability, enabling developers to navigate the evolving landscape of web development with agility and confidence.
Introduction to Loops
Loops represent indispensable control structures within JavaScript, facilitating repetitive code execution based on predefined conditions. These constructs imbue developers with the flexibility to orchestrate iterative workflows, thereby automating repetitive tasks and optimizing code efficiency. JavaScript encapsulates a diverse array of loop mechanisms, encompassing constructs such as for, while, and do-while, each tailored to accommodate unique programming paradigms and use-cases. By harnessing the inherent capabilities of these loop structures, developers can navigate intricate algorithms, manage complex data structures, and orchestrate dynamic control flows, thereby fostering code reusability, scalability, and maintainability within expansive codebases.
The For Loop: Iterating with Precision
The for loop emerges as a quintessential construct within JavaScript, facilitating deterministic iteration by encapsulating three fundamental components: initialization, condition evaluation, and iterative update mechanisms. This tripartite structure imbues the for loop with unparalleled versatility, enabling developers to articulate precise iteration parameters and orchestrate granular code execution sequences. By delineating explicit initialization routines and conditional constraints, developers can establish a robust framework for iterative data traversal, manipulation, and aggregation. Furthermore, the iterative incrementation mechanism inherent to the for loop fosters dynamic control flow orchestration, enabling developers to navigate intricate data structures, implement conditional logic, and optimize algorithmic efficiency. Thus, the for loop epitomizes a cornerstone construct within JavaScript, empowering developers to craft elegant, efficient solutions that resonate with performance excellence and code maintainability.
for (let i = 0; i < fruits.length; i++) { console.log(fruits[i]); }
The While Loop: Conditional Iteration
The while loop serves as a pivotal construct within JavaScript, facilitating conditional iteration by perpetuating code execution as long as a specified predicate remains true. Unlike the for loop, which necessitates predetermined iteration counts, the while loop encapsulates a dynamic execution paradigm, adapting to evolving runtime conditions and data structures. By articulating explicit conditional constraints within the loop's syntax, developers can orchestrate adaptive control flows, enabling nuanced data traversal, manipulation, and aggregation. Furthermore, the inherent flexibility of the while loop fosters iterative precision, empowering developers to navigate complex algorithms, implement dynamic decision-making logic, and optimize code efficiency within diverse programming contexts.
let i = 0; while (i < fruits.length) { console.log(fruits[i]); i++; }
The ForEach Loop: Simplifying Iteration
JavaScript encapsulates a myriad of iteration mechanisms, among which the forEach method stands out as an elegant, concise construct designed to streamline array traversal and manipulation. By abstracting iterative complexities behind a declarative interface, the forEach method facilitates intuitive data processing, enabling developers to focus on core logic implementation rather than intricacies of loop management. This functional programming paradigm fosters code readability, scalability, and maintainability, aligning with modern JavaScript development paradigms. Moreover, by encapsulating iterative operations within a higher-order function, the forEach method promotes code modularity and reusability, empowering developers to craft robust, efficient solutions that resonate with performance excellence, adaptability, and innovation within the dynamic landscape of web development.
fruits.forEach(function(fruit) { console.log(fruit); });
Simplifying Complex Assignments with Arrays and Loops
In the dynamic domain of JavaScript programming, simplifying complex assignments emerges as a paramount objective, achievable through adept utilization of arrays and loops. The synergy between arrays and loops transcends traditional coding paradigms, offering developers a robust framework to dissect intricate problems, streamline workflows, and optimize resource utilization. By harnessing the combined capabilities of arrays and loops, developers can orchestrate seamless data transformations, implement scalable algorithms, and facilitate modular code design. As they navigate the complexities of modern development landscapes, the integration of arrays and loops empowers developers to craft elegant, efficient solutions that resonate with performance excellence and code maintainability. Furthermore, by embracing the transformative potential of arrays and loops, developers cultivate a strategic approach to problem-solving, enabling them to transcend limitations, innovate with confidence, and redefine benchmarks within the burgeoning field of web development.
Sorting Arrays
Sorting arrays emerges as a foundational operation within programming, facilitating data organization and manipulation across diverse applications. JavaScript arrays incorporate intrinsic sorting capabilities, leveraging built-in methods such as sort() to orchestrate seamless element arrangement in ascending or descending sequences. This native functionality encapsulates algorithmic complexities behind a streamlined interface, enabling developers to optimize data structures, enhance search efficiency, and facilitate data visualization within interactive applications. Furthermore, by harnessing the inherent capabilities of the sort() method, developers can tailor sorting criteria, accommodate custom comparison logic, and adaptively manage complex datasets, thereby fostering code reusability, scalability, and maintainability within expansive development ecosystems.
let numbers = [3, 1, 4, 1, 5, 9]; numbers.sort(); // Output: [1, 1, 3, 4, 5, 9]
Filtering Arrays
Filtering arrays epitomizes a quintessential data manipulation paradigm within JavaScript, facilitating targeted element extraction based on predetermined criteria. The filter() method encapsulates this functionality, enabling developers to create refined arrays populated exclusively with elements that satisfy specified conditions. This declarative approach fosters code clarity, modularity, and reusability, enabling developers to articulate precise data selection criteria while minimizing computational overhead. Moreover, by leveraging functional programming paradigms inherent to the filter() method, developers can orchestrate intricate data transformations, implement dynamic filtering logic, and optimize algorithmic efficiency within diverse programming contexts, thereby fostering innovation, adaptability, and scalability within modern web development landscapes.
let evenNumbers = numbers.filter(function(number) {return number % 2 === 0;}); // Output: [4]
Mapping Arrays
Mapping arrays encapsulates a transformative data manipulation paradigm within JavaScript, enabling developers to apply custom transformations to individual array elements. The map() method facilitates this process, empowering developers to craft new arrays populated with elements that undergo specified functional modifications. By leveraging higher-order functions inherent to the map() method, developers can orchestrate intricate data transformations, facilitate dynamic value mapping, and optimize algorithmic efficiency within diverse programming contexts. Furthermore, by embracing the declarative and functional programming paradigms encapsulated within the map() method, developers can streamline data processing workflows, enhance code modularity, and foster innovation within expansive development ecosystems, thereby catalyzing the evolution of robust, efficient solutions tailored to meet contemporary web development challenges.
let squaredNumbers = numbers.map(function(number) { return number * number; }); // Output: [9, 1, 16, 1, 25, 81]
Best Practices and Pitfalls to Avoid
Within the intricate fabric of JavaScript development, adhering to best practices while navigating potential pitfalls stands as a critical determinant of success. Embracing best practices empowers developers to foster code integrity, enhance readability, and promote scalability, laying a solid foundation for sustainable growth and innovation. Conversely, neglecting essential guidelines and overlooking potential pitfalls can precipitate a cascade of challenges, ranging from performance bottlenecks to code vulnerabilities. By meticulously crafting strategies that prioritize best practices, developers cultivate an environment conducive to collaboration, efficiency, and excellence. Concurrently, by vigilantly identifying and mitigating potential pitfalls, they fortify codebases against unforeseen complications, fostering resilience and adaptability within dynamic development landscapes. Thus, the dichotomy between best practices and pitfalls elucidates a nuanced paradigm within JavaScript programming, underscoring the imperative of strategic planning, continuous learning, and proactive engagement to navigate the complexities of modern web development with poise and proficiency.
Best Practices
- Use Descriptive Variable Names: When delving into the realm of arrays and loops, the significance of employing descriptive variable names cannot be overstated. By adopting meaningful and contextually relevant variable nomenclature, developers can foster code clarity, simplify debugging processes, and enhance collaborative development endeavors. Moreover, leveraging descriptive variable names transcends mere syntactic conventions, fostering a cohesive coding environment characterized by transparency, accessibility, and comprehensibility. This best practice underscores the importance of semantic integrity within codebases, enabling developers to articulate intent, functionality, and scope with precision, thereby optimizing code readability, maintainability, and extensibility within dynamic development landscapes.
- Avoid Nested Loops:The proliferation of nested loops within codebases necessitates vigilant scrutiny, as these constructs can precipitate performance bottlenecks and computational inefficiencies. By proactively evaluating code structures and minimizing nested loop configurations, developers can mitigate latency issues, enhance application responsiveness, and optimize algorithmic efficiency. Furthermore, avoiding nested loops fosters modular code design, enabling developers to articulate coherent, scalable solutions that resonate with performance excellence and maintainability. This best practice underscores the imperative of strategic code optimization, iterative refactoring, and proactive performance profiling within diverse development ecosystems, thereby fostering innovation, adaptability, and scalability within contemporary programming paradigms.
- Optimize Array Manipulations: Optimizing array manipulations stands as a pivotal best practice within JavaScript development, emphasizing the utilization of built-in methods—such as push(), pop(), shift(), and unshift()—to facilitate efficient data operations. By harnessing these intrinsic array methods, developers can streamline data insertion, deletion, and traversal processes, thereby optimizing code performance, enhancing computational efficiency, and fostering code modularity. Moreover, embracing built-in array methods encapsulates algorithmic complexities behind declarative interfaces, enabling developers to articulate succinct, readable solutions that resonate with code reusability, scalability, and maintainability. This best practice underscores the symbiotic relationship between methodological rigor and operational efficiency, empowering developers to navigate complex data structures, implement dynamic workflows, and optimize code quality within expansive development landscapes.
Pitfalls to Avoid
- Overusing Loops: The propensity to overutilize loops within JavaScript codebases necessitates prudent evaluation and discernment, as such practices can engender suboptimal performance and code maintainability challenges. While loops represent versatile constructs for iterative tasks, indiscriminate reliance on them for operations better suited for specialized methods or functions can introduce unnecessary complexity, diminish code clarity, and impede algorithmic efficiency. This pitfall underscores the importance of aligning code structures with task-specific requirements, fostering a balanced approach to code design characterized by modularity, scalability, and adaptability. By resisting the allure of ubiquitous loop usage, developers can cultivate a nuanced understanding of programming paradigms, optimizing codebases for performance excellence and future scalability within dynamic development landscapes.
- Ignoring Edge Cases: The inadvertent neglect of edge cases and boundary conditions within arrays and loops can precipitate unforeseen behaviors, erratic application states, and latent software vulnerabilities. Developers must adopt a comprehensive approach to code validation, encompassing rigorous testing, error handling, and boundary condition assessment to fortify applications against potential pitfalls. By prioritizing edge case evaluation, developers can preemptively identify and mitigate anomalous scenarios, enhancing application robustness, reliability, and user satisfaction. This pitfall underscores the imperative of meticulous code review processes, comprehensive testing strategies, and proactive stakeholder engagement within multifaceted development ecosystems, thereby fostering a culture of quality assurance, continuous improvement, and innovation.
- Not Leveraging Array Methods: The failure to leverage JavaScript array methods constitutes a critical pitfall within modern development paradigms, depriving developers of streamlined, efficient solutions for array manipulation tasks. By neglecting to familiarize oneself with intrinsic array methods—such as push(), pop(), shift(), and unshift()—developers inadvertently reinvent the wheel, compromising code efficiency, readability, and maintainability. This pitfall underscores the importance of embracing established programming idioms, best practices, and methodologies, enabling developers to optimize codebases for performance excellence, scalability, and adaptability. By cultivating proficiency in JavaScript array methods, developers can streamline data operations, mitigate algorithmic complexities, and foster code modularity within expansive development landscapes, thereby catalyzing innovation, efficiency, and operational excellence.
Conclusion
In conclusion, JavaScript arrays and loops stand as pivotal components within the programming landscape, providing developers with invaluable resources to tackle intricate tasks effectively. Their versatility and robust capabilities empower developers to navigate, modify, and iterate through vast datasets with unparalleled ease and efficiency. As we reflect on their significance, it becomes evident that mastering the intricacies of arrays and loops is not merely a technical endeavor but a strategic one. By delving deep into their functionalities and nuances, developers can unlock the full potential of JavaScript, leveraging its rich ecosystem to craft elegant and optimized solutions. Moreover, adherence to best practices amplifies the efficacy of arrays and loops, ensuring code integrity, scalability, and maintainability. Therefore, as we navigate the dynamic realm of programming, it is imperative to recognize and harness the transformative power of JavaScript arrays and loops. They transcend mere coding constructs, serving as catalysts that transform convoluted assignments into coherent, streamlined solutions. Embracing their capabilities and nuances fosters a culture of innovation, enabling developers to push boundaries, overcome challenges, and redefine what is possible within the ever-evolving landscape of software development.