Claim Your Offer
Unlock an amazing offer at www.programminghomeworkhelp.com with our latest promotion. Get an incredible 20% off on your second programming assignment, ensuring top-quality assistance at an affordable price. Our team of expert programmers is here to help you, making your academic journey smoother and more cost-effective. Don't miss this chance to improve your skills and save on your studies. Take advantage of our offer now and secure exceptional help for your programming assignments.
We Accept
- Understanding the Need for Interfacing
- The Strengths of Haskell
- Real-world Applications
- Interfacing Techniques
- Foreign Function Interface (FFI)
- Example: Calling C Functions
- Using Haskell's Foreign Function Interface (FFI)
- Example: Calling a C Function in Haskell
- Haskell and Python Integration
- Example: Using inline-python
- WebAssembly Integration
- Example: Haskell and WebAssembly
- Java Interoperability
- Example: Haskell and JNI
- Challenges and Best Practices
- Managing Memory
- Type Mismatch and Marshalling
- Error Handling
- Conclusion
Haskell, renowned for its functional paradigm, robust typing, and elegant syntax, occupies a distinct position in the programming language landscape. Despite its unique approach to programming, developers often encounter scenarios demanding the integration of Haskell with other languages, particularly within the realm of multi-language assignments. This blog embarks on a journey to explore a spectrum of techniques facilitating the seamless interfacing of Haskell with diverse languages. By doing so, it aims to unravel insights into achieving smooth integration, thereby elevating the versatility of programming projects. From leveraging Foreign Function Interface (FFI) for languages like C and C++ to embracing integration with Python, WebAssembly, and Java, this exploration promises to equip developers with practical knowledge to navigate the intricacies of multi-language programming. The ensuing discussion is poised to shed light on challenges such as memory management, type mismatches, and error handling, offering a comprehensive understanding of best practices in ensuring the reliability of multi-language applications. If you're exploring Haskell or need assistance with your Haskell assignment, this blog aims to be a valuable guide for developers seeking to enhance their skills in multi-language programming.
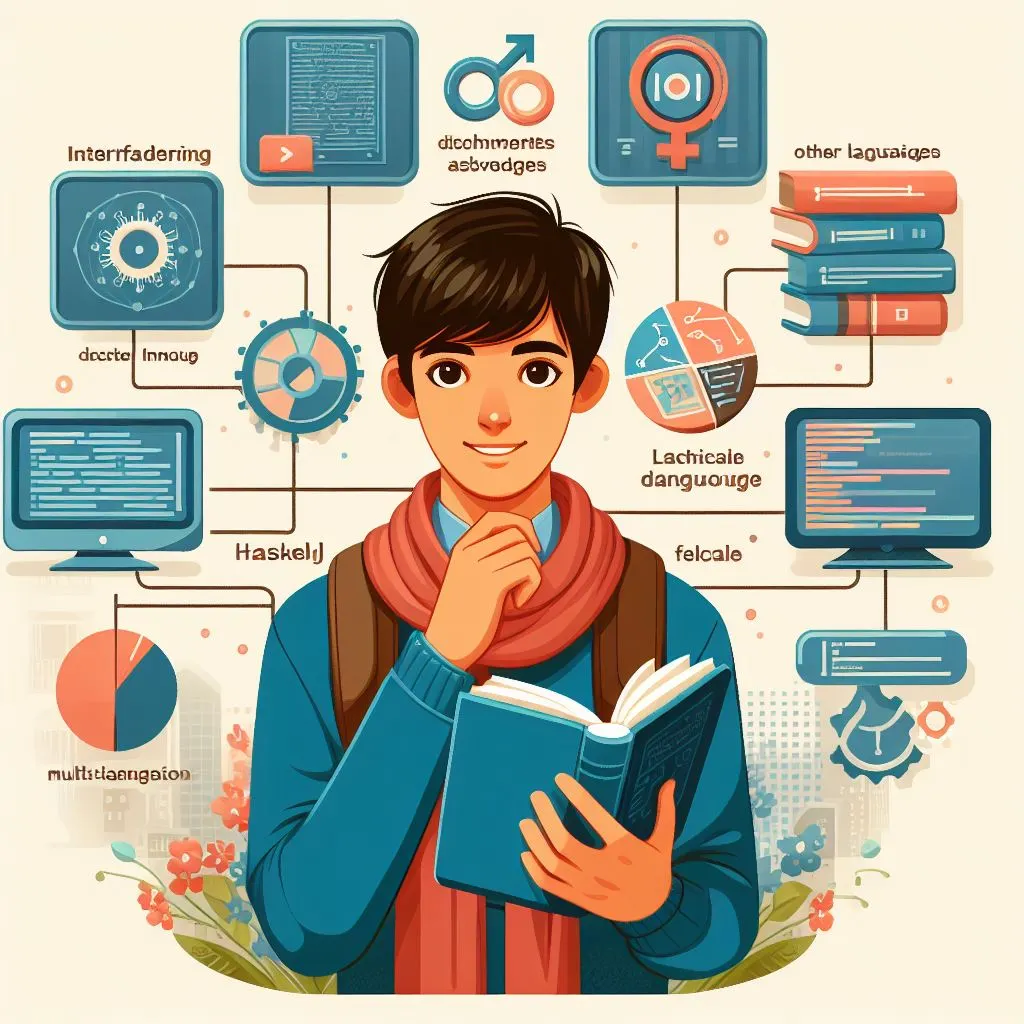
Understanding the Need for Interfacing
Delving into the imperative realm of multi-language programming, comprehending the necessity for interfacing Haskell with other languages becomes pivotal. While Haskell boasts strengths in purity, strong typing, and functional programming, its uniqueness can pose challenges in scenarios demanding collaboration with different programming paradigms or the utilization of external libraries. Recognizing the need for this cross-language interaction is foundational to addressing real-world complexities where a blend of languages may be required. This section explores the motivations behind the imperative to interface Haskell, shedding light on the practical scenarios and use cases where seamless integration with other languages becomes not just beneficial but often essential for the success of diverse software projects. Understanding the nuanced requirements and contexts that drive the need for interfacing sets the stage for the subsequent exploration of techniques aimed at achieving cohesion between Haskell and a spectrum of other programming languages.
The Strengths of Haskell
Haskell's strengths lie in its commitment to purity and the functional programming paradigm, making it an ideal choice for tasks that demand clarity, conciseness, and mathematical precision. The robust type system ensures code reliability, and lazy evaluation facilitates the creation of efficient algorithms. Despite these advantages, the uniqueness of Haskell can pose challenges when attempting to interact with existing codebases or tapping into libraries from other languages. This duality of strength and challenge prompts developers to explore techniques for interfacing Haskell with other languages, allowing them to maximize the benefits of Haskell while seamlessly integrating with diverse programming ecosystems.
Real-world Applications
In the complex landscape of real-world software development, projects often demand a polyglot approach to leverage the unique strengths of different languages. Interfacing Haskell with languages like C, Python, or Java becomes not just a convenience but a necessity in scenarios where integration with existing systems is paramount. Additionally, the ability to tap into specialized libraries or achieve performance optimizations further underscores the practical relevance of interfacing Haskell with other languages. Whether it's enhancing interoperability or meeting specific project requirements, the real-world applications of multi-language interfacing showcase the versatility and adaptability required in modern software development endeavors.
Interfacing Techniques
Navigating the intricacies of interfacing Haskell with other languages involves a diverse set of techniques, each offering unique advantages and considerations. This section delves into the practical approaches that empower developers to seamlessly integrate Haskell with languages such as C, C++, Python, Java, and WebAssembly. From harnessing the power of the Foreign Function Interface (FFI) to bridging the gap between Haskell's functional prowess and Python's versatility, the exploration covers a spectrum of methods. Integrating with WebAssembly opens doors to high-performance web applications, while Java interoperability facilitates collaboration in Java-centric environments. Understanding these interfacing techniques not only broadens the developer's toolkit but also provides insights into overcoming challenges related to memory management, type mismatches, and error handling. By grasping the nuances of these techniques, developers can strategically leverage the strengths of different languages, enhancing the overall flexibility and capability of their programming projects.
Foreign Function Interface (FFI)
Haskell's Foreign Function Interface (FFI) stands as a robust mechanism, acting as a gateway for seamlessly calling functions written in languages such as C, C++, and Fortran. This powerful feature establishes a standardized pathway for declaring foreign functions and data types, fostering a cohesive environment for interoperability. By providing a bridge between Haskell and other languages, the FFI empowers developers to integrate existing libraries and functionalities seamlessly. This interoperability ensures that Haskell can leverage the strengths of languages with different paradigms, contributing to the development of versatile and high-performance applications.
Example: Calling C Functions
```haskell -- Haskell code using FFI to call a C function foreign import ccall "math.h sin" c_sin :: CDouble -> CDouble ```
The practical application of Haskell's FFI becomes evident in scenarios where calling functions from external languages is paramount. Illustrated in the example code snippet, the seamless integration with C is showcased, with the foreign import ccall directive allowing Haskell to invoke a C function (sin from math.h). This exemplifies how Haskell's FFI acts as a linguistic bridge, facilitating the incorporation of foreign functionality into Haskell projects and underlining its significance in enhancing the language's adaptability.
Using Haskell's Foreign Function Interface (FFI)
Haskell's Foreign Function Interface (FFI) emerges as a potent tool for integrating functions from languages like C, C++, and Fortran into Haskell projects. By enabling a smooth channel of communication between Haskell and external languages, developers gain the capability to tap into existing libraries and functionalities seamlessly. The provided example highlights the practical use of Haskell's FFI to call a C function (sin from math.h). This exemplifies the power of FFI in extending the reach of Haskell, allowing developers to harness the strengths of other languages and libraries within the Haskell ecosystem. The FFI, therefore, becomes a linchpin for achieving a harmonious coexistence of languages and functionalities in multi-language programming projects.
Example: Calling a C Function in Haskell
```haskell -- Haskell code using FFI to call a C function foreign import ccall "math.h sin" c_sin :: CDouble -> CDouble ```
This Haskell code demonstrates the use of the Foreign Function Interface (FFI) to call a C function, specifically the sin function from the math.h library. The foreign import ccall directive establishes a connection between Haskell and the C function, allowing the invocation of c_sin in Haskell code. The type signature CDouble -> CDouble indicates that the c_sin function takes a CDouble argument and returns a CDouble. This seamless integration exemplifies how Haskell can leverage the capabilities of other languages through FFI, providing a practical illustration of interfacing Haskell with C functions.
Haskell and Python Integration
When the strengths of both Haskell and Python are essential for a particular task, tools like hpython or inline-python emerge as valuable resources. These tools facilitate the seamless integration of Python code within Haskell programs or vice versa. The example code utilizing inline-python showcases the convenience these tools offer. Through the use of quasi-quotes, Haskell code can directly incorporate Python functionality, providing developers with a flexible and efficient means to combine the capabilities of both languages. This integration not only enhances the versatility of programming projects but also exemplifies the collaborative potential of Haskell and Python in solving complex problems that require the strengths of both languages.
Example: Using inline-python
```haskell -- Haskell code with inline Python {-# LANGUAGE QuasiQuotes #-} import Language.Python.Inline add :: Int -> Int -> Int add a b = [python| $a + $b |] ```
The provided Haskell code utilizing inline-python illustrates a practical scenario where Python code is seamlessly embedded within Haskell. The add function combines Haskell variables ($a and $b) with Python's arithmetic operation, demonstrating the ease with which developers can harness the strengths of both languages within a unified codebase. This example underscores the practicality of Haskell and Python integration through tools like inline-python, showcasing a harmonious fusion of their respective capabilities.
WebAssembly Integration
In the realm of web development and performance-critical applications, integrating Haskell with WebAssembly introduces a transformative dimension. WebAssembly enables the execution of high-performance code written in languages like C, C++, and Rust directly in web browsers. The presented Haskell code targeting WebAssembly employs the JavaScriptFFI language extension, showcasing the integration with WebAssembly capabilities. By loading a WebAssembly module and instantiating it within Haskell, developers gain access to the performance benefits offered by WebAssembly. This example emphasizes the game-changing potential of combining Haskell with WebAssembly, particularly in scenarios where optimal performance in web applications is paramount. The ability to tap into this capability positions Haskell as a versatile language capable of addressing diverse application requirements.
Example: Haskell and WebAssembly
```haskell -- Haskell code targeting WebAssembly {-# LANGUAGE JavaScriptFFI #-} import Language.JavaScript.WebAssembly fibonacci :: Int -> IO Int fibonacci n = do wasmModule <- loadModule "path/to/fibonacci.wasm" instance_ <- instantiate wasmModule call instance_ "fibonacci" [toJSVal n] ```
The showcased Haskell code targeting WebAssembly exemplifies a paradigm-shifting integration, particularly relevant in the domains of web development and performance-intensive applications. Utilizing the JavaScriptFFI language extension, this example underscores Haskell's ability to tap into WebAssembly's high-performance capabilities. The fibonacci function, a classic computational algorithm, serves as a practical use case. The loading of the WebAssembly module from a specified path, instantiation of the module, and subsequent invocation of the fibonacci function within Haskell demonstrate the seamless interoperation between Haskell and WebAssembly. This integration empowers developers to leverage the efficiency of languages like C, C++, or Rust within the Haskell ecosystem, enhancing the overall performance of web applications. The example not only provides a glimpse into the technical intricacies of Haskell and WebAssembly integration but also highlights the potential transformative impact on applications requiring optimal performance in the dynamic landscape of modern web development.
Java Interoperability
When navigating Java-based projects or incorporating Java libraries, the Java Native Interface (JNI) emerges as a vital tool for interfacing Haskell with Java. JNI facilitates seamless integration by bridging the gap between Haskell and Java, enabling access to existing Java codebases and leveraging Java libraries directly within Haskell projects. The example Haskell code utilizing JNI serves as a practical demonstration of this interoperability. The foreign import ccall directive establishes a connection with Java, allowing Haskell to wrap functions and seamlessly communicate with Java components. This integration not only showcases the versatility of Haskell but also underscores its ability to collaborate effortlessly with Java, making it a valuable asset for developers working in environments where Java plays a pivotal role.
Example: Haskell and JNI
```haskell -- Haskell code using JNI to interface with Java foreign import ccall "wrapper" wrapHello :: IO () -> IO (FunPtr (IO ())) foreign export ccall "helloFromHaskell" helloFromHaskell :: IO () helloFromHaskell :: IO () helloFromHaskell = putStrLn "Hello from Haskell!" main :: IO () main = do callback <- wrapHello helloFromHaskell withCString "com/example/Hello" $ \str -> [C.exp| void { Java_com_example_Hello_registerCallback($str, $fun:(void (*callback)())) } |] ```
In the provided Haskell code utilizing JNI, a bridge is established between Haskell and Java, demonstrating the seamless interoperability between the two languages. The foreign import ccall and foreign export ccall directives facilitate the interaction, allowing Haskell to call Java functions and export Haskell functions for use in Java. The example further highlights the integration of callback mechanisms, showcasing the bidirectional nature of this interoperability. By employing JNI, developers can unlock the potential for incorporating Haskell into existing Java ecosystems and enhancing the overall functionality of their projects. This integration exemplifies the adaptability of Haskell in diverse environments, especially when collaborating with Java-based systems or libraries.
Challenges and Best Practices
Unraveling the intricacies of interfacing Haskell with other languages brings forth a set of challenges and best practices crucial for ensuring the success and reliability of multi-language projects. Managing memory effectively stands out as a primary challenge, particularly when interfacing with languages like C, where Haskell's garbage collector does not extend its reach. Addressing type mismatches and mastering the art of data marshalling becomes paramount to maintain cohesion across diverse data representations. Moreover, the complex landscape of error handling, especially when traversing language boundaries, necessitates robust mechanisms. This section explores the hurdles developers encounter when bridging Haskell with other languages, offering insights into best practices for tackling issues related to memory, types, and errors. By understanding and implementing these practices, developers can navigate the nuances of multi-language programming, ensuring the creation of resilient and efficient applications that seamlessly integrate the strengths of various programming languages.
Managing Memory
In the intricate realm of interfacing Haskell with languages like C, meticulous memory management becomes a critical consideration. Haskell's garbage collector operates within its own domain and does not extend its reach to foreign memory. As a result, developers shoulder the responsibility of allocating and deallocating memory correctly to avert potential memory leaks. This aspect of memory management necessitates a nuanced understanding of both Haskell and the foreign language's memory handling mechanisms. By ensuring proper memory management, developers can enhance the reliability and stability of multi-language applications, particularly in scenarios where meticulous control over memory is imperative for seamless interfacing.
Type Mismatch and Marshalling
Navigating the disparities in data representations between Haskell and other languages introduces challenges such as type mismatches. The effective marshalling of data types becomes paramount to facilitate coherent communication and prevent runtime errors. The process of translating data structures between languages requires careful consideration of their respective representations. Developers must implement robust marshalling techniques to bridge the gap and ensure harmonious data exchange. This aspect of interfacing demands a meticulous approach to data management, emphasizing the importance of understanding and addressing type mismatches to guarantee the integrity of data across language boundaries.
Error Handling
Handling errors across the boundaries of different languages presents a distinctive set of challenges. Robust error-handling mechanisms must be implemented to address potential issues seamlessly. Understanding how exceptions propagate between languages is vital for building reliable multi-language applications. Errors occurring in one language must be gracefully communicated to and handled by the other. This requires a comprehensive understanding of the error-handling mechanisms inherent in both languages. The ability to gracefully manage errors enhances the overall robustness of multi-language applications, ensuring that issues are identified, communicated, and resolved effectively across the diverse landscape of languages involved in a project.
Conclusion
In conclusion, the act of interfacing Haskell with other languages presents developers with a myriad of opportunities, fostering the amalgamation of diverse programming paradigms and the exploitation of pre-existing libraries. Whether employing the Foreign Function Interface (FFI), integrating with Python, delving into WebAssembly, or establishing interoperability with Java, each avenue unfolds its unique set of advantages and challenges. A comprehensive understanding of these interfacing techniques not only equips developers to navigate the intricacies of multi-language programming but also empowers them to construct resilient and adaptable applications. As the landscape of software development continues its dynamic evolution with advancing technology, the seamless integration of Haskell with other languages emerges as a pivotal capability, shaping the trajectory of innovation and efficiency in the ever-changing realm of programming. Embracing the versatility enabled by these interfacing methods becomes instrumental in ensuring that developers can meet the demands of contemporary software development challenges and stay at the forefront of the evolving technological landscape.