Claim Your Offer
Unlock an amazing offer at www.programminghomeworkhelp.com with our latest promotion. Get an incredible 10% off on your all programming assignment, ensuring top-quality assistance at an affordable price. Our team of expert programmers is here to help you, making your academic journey smoother and more cost-effective. Don't miss this chance to improve your skills and save on your studies. Take advantage of our offer now and secure exceptional help for your programming assignments.
We Accept
Sample Text-Based Adventure Games Developed by Our Experts
Our sample section offers a firsthand look at the quality and expertise we bring to every Text-based adventure game assignment. Here, you can browse through completed projects that showcase our proficiency in game design, programming logic, and interactive storytelling.
Haskell
Haskell
Haskell
Haskell
Haskell
Haskell
Haskell
Haskell
Haskell
Haskell
Haskell
Haskell
Haskell
Haskell
Haskell
Haskell
Haskell
Haskell
Haskell
Haskell
Procure Affordable Text-Based Adventure Game Assignment Writing Help
At ProgrammingHomeworkHelp.com, we understand the importance of affordability when it comes to Text-based adventure game assignment writing help. That's why we go the extra mile to customize our rates, ensuring that our services remain accessible to students of all budgets. By offering flexible pricing options, we cater to individual needs and requirements, providing a personalized experience that prioritizes your academic success without breaking the bank. Our transparent pricing structure is designed to be fair and competitive, with no hidden fees or unexpected charges. Check out the sample price ranges below to see how our rates can fit within your budget:
Service Type | Price Range (USD) |
---|---|
Basic Text-based adventure game assignment | $50 - $100 |
Intermediate Text-based adventure game assignment | $100 - $200 |
Advanced Text-based adventure game assignment | $200 - $300 |
Urgent Text-based adventure game assignment | $150 - $250 |
Customized Text-based adventure game assignment | $250+ |
- What is Text-based Adventure Games?
- Popular Programming Languages Our Text-Based Adventure Game Assignment Helpers Can Use for Your Homework
- Exclusive Discounts for Availing Our Online Text-Based Adventure Game Assignment Help Service
- Pay Us to Do Your Text-Based Adventure Game Homework Regardless of the Topic
- Benefits of Availing Help with Text-Based Adventure Game Assignment
What is Text-based Adventure Games?
Text-based adventure games are interactive stories where players navigate through a virtual world by reading descriptive text and inputting commands to progress the narrative. These games typically lack graphical elements, relying solely on text to convey environments, characters, and actions. Key concepts involved in text-based adventure games include:
- Interactive Fiction: Players engage with the game by making decisions and solving puzzles, shaping the outcome of the story.
- Parser: A parser interprets player input, allowing them to interact with the game world by typing commands such as "go north" or "take key".
- World State: The game maintains a state of the virtual world, including the player's location, items they possess, and any changes caused by their actions.
- Narrative Design: Crafting compelling storylines, characters, and settings is crucial to immerse players in the game world and keep them engaged.
- Game Mechanics: Implementing rules and systems that govern how the game operates, including combat, puzzle-solving, and exploration.
- Player Agency: Providing players with meaningful choices that impact the outcome of the game, promoting replayability and immersion.
Text-based adventure games offer a unique gaming experience that emphasizes storytelling, problem-solving, and imagination, making them a beloved genre among gamers and developers alike.
Popular Programming Languages Our Text-Based Adventure Game Assignment Helpers Can Use for Your Homework
Text-based adventure games present an exciting challenge for students, requiring a blend of creative storytelling and technical expertise. At ProgrammingHomeworkHelp.com, our team of Text-based adventure game assignment helpers is made up of skilled game developers and programming experts who excel in using various programming languages to develop these immersive games. Below are some of the popular tools we specialize in:
- Python: Renowned for its simplicity and readability, Python is an excellent choice for Text-based adventure game assignments. Our experts leverage Python's rich libraries and easy syntax to develop captivating game mechanics and narrative structures, ensuring your homework meets the highest standards.
- Java: With its platform independence and robust object-oriented programming features, Java is another favored language for Text-based adventure game development. Our skilled developers harness Java's power to create dynamic game environments and implement complex interactions, guaranteeing your assignment stands out.
- C++: Ideal for students seeking to delve into low-level programming and optimize game performance, C++ offers unparalleled control and efficiency. Our team of programming experts utilizes C++'s flexibility to craft intricate game systems and mechanics, providing tailored solutions for your Text-based adventure game homework.
- JavaScript: As the language of the web, JavaScript is perfect for developing browser-based Text-based adventure games. Our adept developers leverage JavaScript's event-driven paradigm and extensive libraries to create interactive game experiences, ensuring your assignment captivates players and earns top grades.
Whether you need assistance with Python, Java, C++, or JavaScript, our Text-based adventure game assignment helpers are here to support you every step of the way. Trust ProgrammingHomeworkHelp.com for expert guidance and impeccable solutions tailored to your academic needs.
Exclusive Discounts for Availing Our Online Text-Based Adventure Game Assignment Help Service
We value our students and strive to make our services accessible and affordable. That's why we offer a range of exclusive discounts to ensure you get the most out of your experience with us. Here are the various types of discounts you can enjoy:
- Refer a Friend: Spread the word about our services and reap the rewards! Refer a friend and get a whopping 50% off on your next Text-based adventure game assignment. It's our way of saying thank you for your loyalty and for introducing us to new students in need of assistance.
- 20% Discounts on Your Second Order: Once you've experienced the quality of our services, we want to reward your continued trust and patronage. Enjoy a generous 20% discount on your second Text-based adventure game assignment order as a token of our appreciation.
- Bulk Orders Discount: Planning to avail our services for multiple assignments? We've got you covered! Enjoy special discounts on bulk orders, making it even more cost-effective to get the help you need for all your Text-based adventure game assignments.
- Seasonal Discounts: Keep an eye out for our seasonal discounts and promotions throughout the year. Whether it's back-to-school season or holiday specials, we regularly offer exclusive deals to help you save on your Text-based adventure game assignments.
Take advantage of these exclusive discounts and make the most out of your experience with ProgrammingHomeworkHelp.com. With our affordable rates and quality services, achieving academic success has never been more accessible. Avail our online programming assignment help service now.
Pay Us to Do Your Text-Based Adventure Game Homework Regardless of the Topic
At ProgrammingHomeworkHelp.com, our team of programming experts excels in crafting top-notch assignments across a range of topics. Whether you need help with coding, design, or narrative development, we've got you covered. Below are eight Text-based adventure game homework topics we specialize in:
- Game Design Principles: Our experts are well-versed in the fundamental principles of game design, ensuring your homework incorporates engaging mechanics, balanced challenges, and a captivating storyline.
- Programming Logic and Algorithms: Crafting efficient algorithms and implementing logical solutions are essential for Text-based adventure game development. Pay someone to do my Text-based adventure game homework and our experts will deliver meticulously crafted code that meets your requirements.
- Interactive Storytelling: Crafting immersive narratives that respond to player choices requires a delicate balance of creativity and technical skill. Trust our team to develop dynamic storylines and branching narratives that enhance player engagement in your homework.
- User Interface (UI) Design: Designing intuitive user interfaces that enhance the player experience is crucial for Text-based adventure games. Let our experts create sleek and user-friendly interfaces for your homework, optimizing player interaction and enjoyment.
- World Building and Environment Design: From sprawling landscapes to intricate dungeons, our team specializes in crafting rich and immersive game worlds. Pay someone to do my Text-based adventure game homework and rest assured that your game environment will be meticulously designed and brimming with detail.
- Puzzle Design and Implementation: Engaging puzzles are a cornerstone of Text-based adventure games, challenging players to think critically and creatively. Trust our experts to design and implement mind-bending puzzles that will leave players eager for more in your homework.
- Character Development and AI: Bringing characters to life with realistic behaviors and personalities enhances the immersion of Text-based adventure games. Let our team create compelling characters and sophisticated AI systems for your homework, enriching the player experience with dynamic interactions.
- Testing and Debugging: Thorough testing and debugging are essential to ensure your Text-based adventure game functions smoothly and flawlessly. Pay someone to do my programming homework and our meticulous experts will rigorously test and debug your code, delivering a polished final product.
With our expertise and dedication to excellence, ProgrammingHomeworkHelp.com is your ultimate destination for high-quality Text-based adventure game homework solutions. Trust us to help you succeed in your academic endeavors with expertly crafted assignments tailored to your needs.
Benefits of Availing Help with Text-Based Adventure Game Assignment
When seeking help with Haskell assignments, you deserve nothing but the best. At ProgrammingHomeworkHelp.com, we pride ourselves on delivering unparalleled assistance tailored to your needs. Here are the benefits of choosing our services:
- Certified Game Developers: With a team of certified game developers at your disposal, you can trust us to provide expert guidance and assistance throughout your assignment.
- Error-Free Programs Running Perfectly: Our meticulous attention to detail ensures that your Text-based adventure game programs are error-free and run seamlessly, guaranteeing a smooth gaming experience for your players.
- Affordable Rates: We understand the budget constraints of students, which is why we offer our services at affordable rates without compromising on quality. Get top-notch assistance without breaking the bank.
- Prompt Deliveries: Deadlines are sacred, and we take them seriously. Count on us to deliver your Text-based adventure game assignment on time, every time, allowing you to submit your work without any worries.
- 24/7 Customer Support: Have a question or need assistance at any time of the day or night? Our dedicated customer support team is available 24/7 to address your concerns and provide prompt solutions via live chat.
- Safe and Secure Payment Gateways: Your security is our priority. We ensure safe and secure payment transactions through trusted gateways, giving you peace of mind when availing our services.
- Confidentiality Guaranteed: Your privacy is paramount to us. Rest assured that your personal information and assignment details are kept confidential and will never be shared with third parties.
- Free Unlimited Revisions: We believe in delivering satisfaction guaranteed. If you're not completely satisfied with the results, we offer free unlimited revisions until you're fully content with your Text-based adventure game assignment.
Experience these benefits firsthand by availing our help with Text-based adventure game assignment today.
Blogs on the Techniques & Best Practices of Developing Text-Based Adventure Games
Delve deeper into the world of Text-based adventure game development by exploring our informative blog. Our blog covers a wide range of topics, including coding techniques, design principles, and industry trends, to help you stay updated and enhance your skills. Whether you're a beginner looking to learn the basics or an experienced developer seeking advanced tips, our blog has something for everyone. By providing valuable resources and insights, we empower you to succeed in your Text-based adventure game assignments and beyond.
More than 1.2K Reviews Shared by Students Who Have Benefitted from Our Services
Our review section provides valuable insights into the experiences of students who have availed our Text-based adventure game assignment help. Here, you can read testimonials from satisfied clients who have benefited from our top-notch services. These reviews highlight our commitment to quality, reliability, and customer satisfaction. By showcasing feedback from past clients, we aim to give you confidence in our ability to deliver exceptional results and exceed your expectations with every assignment.
Hire the Best Text-Based Adventure Game Assignment Experts
At ProgrammingHomeworkHelp.com, our expert team consists of seasoned professionals with extensive experience in Text-based adventure game development. From skilled programmers proficient in various languages to creative designers with a knack for immersive storytelling, each member of our team brings a unique set of skills and expertise to the table. We understand the intricacies of game design and programming, allowing us to provide tailored solutions that meet your specific requirements and academic goals.
.webp)
Freddie Morris
PhD in Programming
🇨🇦 Canada
Freddie Morris, a Haskell developer with over 10 years of experience, specializes in functional programming and software design.
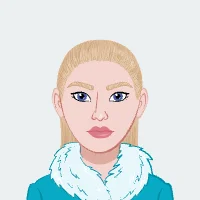
Pamela Tuller
PhD in Programming
🇺🇸 United States
Pamela Tuller is a computer science expert with extensive experience in Haskell programming and data structures. She specializes in recursive algorithms and binary trees, offering practical insights and in-depth analyses.
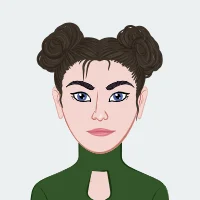
Dr. Emily Lawson
Ph.D. in Computer Science
🇬🇧 United Kingdom
Dr. Emily Lawson, a Ph.D. holder in Computer Science, is an esteemed Haskell Assignment Expert with a decade of experience. With expertise in functional programming and algorithmic problem-solving, she provides tailored guidance to students, fostering their mastery of Haskell and functional data structures for academic success.
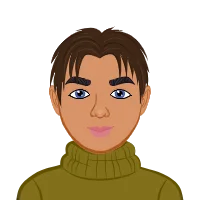
Dr. Ronald Harrison
Ph.D. in Haskell
🇬🇧 United Kingdom
Dr. Ronald Harrison is a Haskell specialist with 15+ years of experience in functional programming, lambda calculus, and compiler design. With a Ph.D. from Cambridge and extensive teaching and consulting experience, he offers expert guidance on Haskell assignments, combining deep knowledge with effective teaching skills.
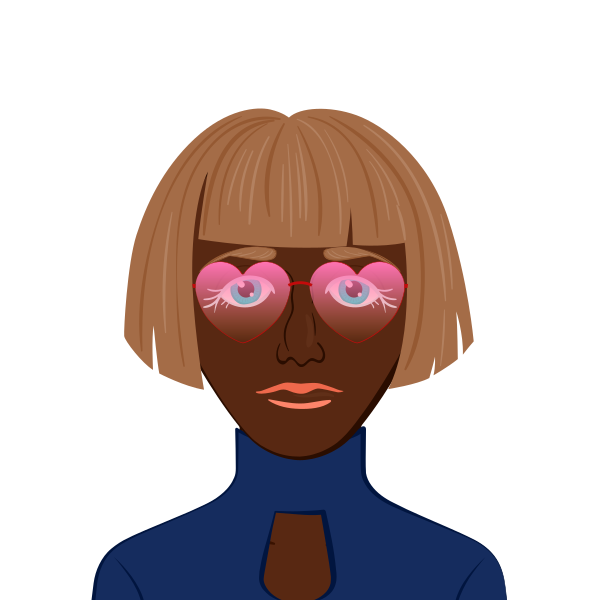
Prof. Emily Thompson
Master's degree in Computer Engineering
🇦🇺 Australia
Prof. Emily Thompson, with a Master's degree in Computer Engineering from Stanford University, has completed over 900 HSPEC assignments with excellence. Prof. Thompson specializes in HSPEC test result analysis, debugging techniques, and interpreting Haskell code coverage reports. With her expertise, she assists students in optimizing their Haskell code and improving overall code quality.
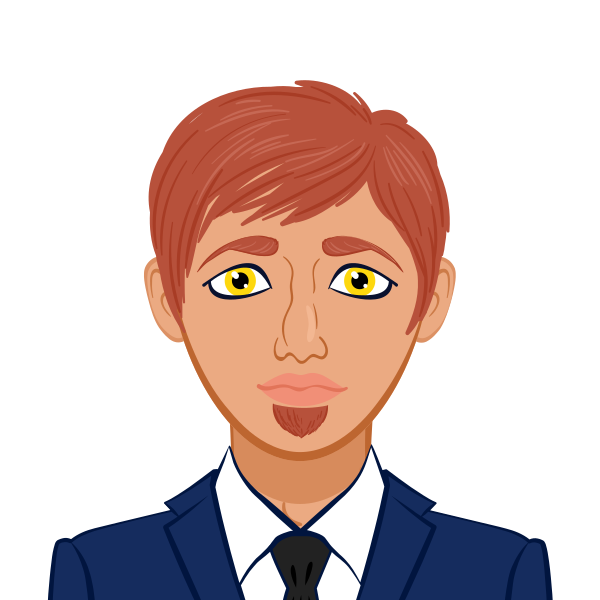
Dr. Liam Patel
Ph.D. in Computer Science
🇨🇦 Canada
Dr. Liam Patel, a Ph.D. holder in Computer Science from Monash University, has completed over 600 HSPEC assignments with distinction. Dr. Patel specializes in designing complex test cases, mocking and stubbing techniques, and integrating HSPEC tests into existing Haskell projects. With his deep understanding of functional programming principles, Dr. Patel offers insightful guidance to students seeking HSPEC assignment help.
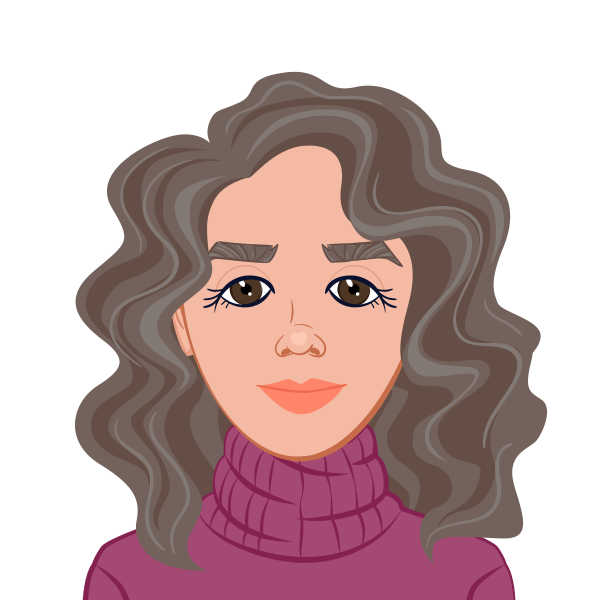
Prof. Samantha Murphy
Master's degree in Software Engineering
🇸🇬 Singapore
Prof. Samantha Murphy, with a Master's degree in Software Engineering from MIT, has successfully completed over 700 HSPEC assignments. Proficient in Haskell and HSPEC testing framework, Prof. Murphy specializes in basic and advanced HSPEC configurations, integration with other tools, and custom test report generation. With her expertise, she provides tailored support to students across various academic levels.
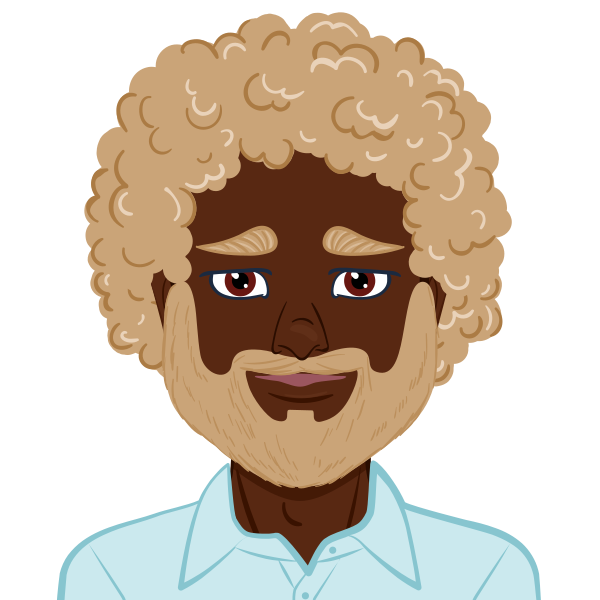
Dr. Benjamin Wilson
Ph.D. in Computer Science
🇦🇺 Australia
Dr. Benjamin Wilson holds a Ph.D. in Computer Science from Oxford University and has completed over 800 HSPEC assignments with exceptional results. With extensive experience in functional programming and testing methodologies, Dr. Wilson specializes in HSPEC setup, test-driven development, and performance testing. His expertise extends to advanced Haskell concepts, ensuring comprehensive support for students seeking assistance.
-(1).png)
Aisha Tan
Master's degree in Interactive Media
🇸🇬 Singapore
Aisha Tan is an expert game developer with a Master's degree in Interactive Media from the National University of Singapore. She has completed over 950 Text-based adventure game assignments, focusing on puzzle design and user interface development. Aisha's keen eye for detail and her innovative solutions ensure that every game she works on is both challenging and user-friendly.
.png)
Liam Chen
Bachelor's degree in Game Design
🇦🇺 Australia
Liam Chen, a graduate of the University of Melbourne with a Bachelor's degree in Game Design, has extensive experience in creating dynamic game environments and character interactions. With over 750 assignments completed, Liam is known for his creative approach to game design and his proficiency in integrating interactive elements seamlessly.
.png)
John Williams
a Ph.D. in Software Engineering
🇸🇬 Singapore
John Williams is a seasoned programmer with a Ph.D. in Software Engineering from MIT. Specializing in algorithm design and optimization, John has successfully completed more than 900 Text-based adventure game assignments. His ability to solve intricate coding challenges and optimize game performance makes him an invaluable asset to our team.
.png)
Emma Smith
Master's in Computer Science
🇬🇧 United Kingdom
Emma Smith holds a Master's degree in Computer Science from the University of Cambridge. With a strong background in game development and interactive storytelling, she has completed over 850 Text-based adventure game assignments. Her expertise lies in crafting engaging narratives and designing complex game mechanics, ensuring that each project she handles is both immersive and technically sound.
Prof. Liam Carter
Ph.D. in Computer Science
🇦🇹 Austria
Prof. Liam Carter, a renowned educator from McGill University, has completed over 900 Haskell assignments with exceptional proficiency. With expertise in Haskell programming, Prof. Carter specializes in deep sequencing optimization, lazy evaluation techniques, and performance tuning. His commitment to academic excellence and personalized guidance makes him a sought-after mentor for students seeking help with Haskell assignments Using DEEPSEQ.
Prof. Brandon Mitchell
Ph.D. in Computer Science
🇦🇺 Australia
Prof. Brandon Mitchell, an esteemed faculty member at Stanford University, brings extensive experience and expertise in Haskell programming to our team. With over 700 successfully completed Haskell assignments, Prof. Mitchell specializes in performance optimization, lazy data structures, and selective application of Deepseq. His strong academic background and practical insights make him a trusted advisor for students seeking help with Haskell assignments using DEEPSEQ.
Dr. Sophie Anderson
Ph.D. in Computer Science
🇬🇧 United Kingdom
Dr. Sophie Anderson holds a Ph.D. in Computer Science from the University of Melbourne and has completed over 600 Haskell assignments with excellence. With expertise in Haskell programming, Dr. Anderson specializes in balancing strictness and laziness, error-free code development, and well-commented solutions. Her dedication to delivering high-quality assistance ensures that students receive comprehensive support for their Haskell assignments.
Dr. Samantha Bennett
Ph.D. in Computer Science
🇺🇸 United States
Dr. Samantha Bennett holds a Ph.D. in Computer Science from the University of Cambridge and has completed over 800 Haskell assignments with exceptional results. With expertise in Haskell programming, Dr. Bennett specializes in lazy evaluation, strictness annotations, and deep sequencing techniques. Her vast experience and in-depth knowledge make her a valuable resource for students seeking assistance in Haskell assignments.
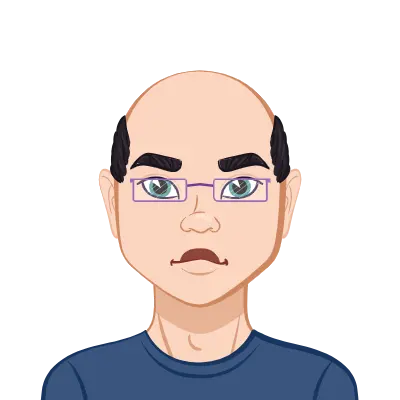
David Lee
Master's degree in Software Engineering
🇺🇸 United States
David holds a Master's degree in Software Engineering and has been passionate about functional programming since his early studies. With over 500 WAI assignments under his belt, he excels at explaining core WAI concepts like request handling, middleware, and logging. David's expertise extends to functional testing frameworks like QuickCheck, ensuring students write well-tested and reliable WAI applications.
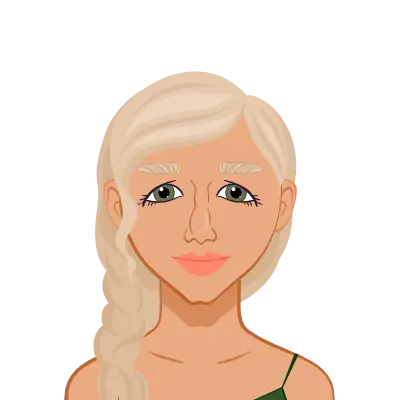
Nadia Dubois
Master's in Computer Science
🇨🇦 Canada
Nadia is a seasoned developer with over 6 years of experience in building dynamic web applications using Haskell and WAI frameworks. Having completed over 700 assignments, she thrives on helping students understand real-world use cases of WAI, including form handling, user interaction, and data validation. Nadia also enjoys delving into front-end integration with frameworks like Elm.

Dr. Chen Wang
Ph.D. in Functional Programming Languages
🇦🇺 Australia
Dr. Wang brings a wealth of academic expertise with a Ph.D. in Functional Programming Languages. With over 900 completed WAI assignments, she excels in guiding students through complex topics like monads, lazy evaluation, and type systems. Dr. Wang is also passionate about web security and enjoys helping students implement robust security measures in their WAI applications.
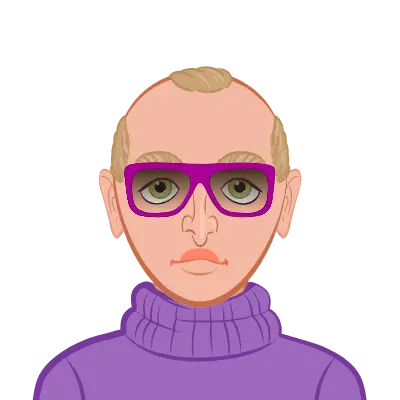
Alistair Cunningham
Master's in Computer Science
🇬🇧 United Kingdom
Alistair boasts a master's degree in computer science and over 7 years of professional experience in functional programming. He's completed over 800 WAI assignments, specializing in areas like server-side development, routing, templating engines, and user authentication. Alistair's in-depth knowledge also covers integration with databases and security best practices.
Frequently Asked Questions
Have questions about our Text-based adventure game assignment help service? Our FAQs section provides answers to common queries to help you make informed decisions. From inquiries about our pricing and delivery process to questions about our expertise and guarantees, we've got you covered. If you need further assistance, our friendly customer support team is available via live chat to provide prompt and helpful responses, ensuring a seamless experience from start to finish.
Certainly! We offer debugging and troubleshooting support to help resolve any issues or errors in Text-based adventure game assignments. Our team conducts rigorous testing, identifies bugs, and provides tailored solutions to ensure that your game functions flawlessly and meets the desired specifications.
Our team prioritizes optimizing Text-based adventure game assignments for performance and efficiency. We follow best practices in coding and algorithm design, conduct thorough testing to identify and resolve bottlenecks, and utilize optimization techniques to ensure smooth gameplay and responsiveness.
Yes, we have expertise in coding and programming challenges commonly encountered in Text-based adventure game development. Whether it's implementing player movement, handling inventory systems, or managing game states, we provide comprehensive support to address your specific needs.
Absolutely! We specialize in designing and implementing challenging puzzles tailored to your Text-based adventure game assignments. Our team creates puzzles with logical solutions and integrates them seamlessly into the game environment for an enjoyable player experience.
Our team meticulously crafts immersive narratives and engaging storylines for Text-based adventure game assignments. We focus on character development, world-building, and interactive storytelling techniques to ensure coherence and player immersion throughout the game.