Claim Your Offer
Unlock an amazing offer at www.programminghomeworkhelp.com with our latest promotion. Get an incredible 20% off on your second programming assignment, ensuring top-quality assistance at an affordable price. Our team of expert programmers is here to help you, making your academic journey smoother and more cost-effective. Don't miss this chance to improve your skills and save on your studies. Take advantage of our offer now and secure exceptional help for your programming assignments.
We Accept
- Understanding JavaScript Events
- Basic Event Handling Syntax
- Practical Applications in Web Programming Assignments
- Form Validation: Ensuring Data Integrity
- Real-time Data Updates: Keeping Content Fresh
- Drag-and-Drop Interfaces: Intuitive User Interactions
- Conclusion
JavaScript is a cornerstone in modern web development, empowering developers to create dynamic and interactive websites. One of the key features that makes this possible is event handling. Events in JavaScript are user interactions or browser actions that trigger specific functions, allowing developers to respond to user input and create a more engaging user experience.
JavaScript reigns supreme in transforming static websites into dynamic, interactive experiences. At the heart of this transformation lies the power of event handling. Events, triggered by user actions or browser interactions, form the backbone of responsive web design. In this blog post, we delve into the practical applications of JavaScript event handling, offering students insights and examples that go beyond the basics. From form validation to real-time data updates, join us on a journey to discover how mastering event handling can elevate your web programming assignments and set you on the path to creating immersive and user-centric web applications. For assistance with your JavaScript assignment, let's explore the art and science of JavaScript event handling together!
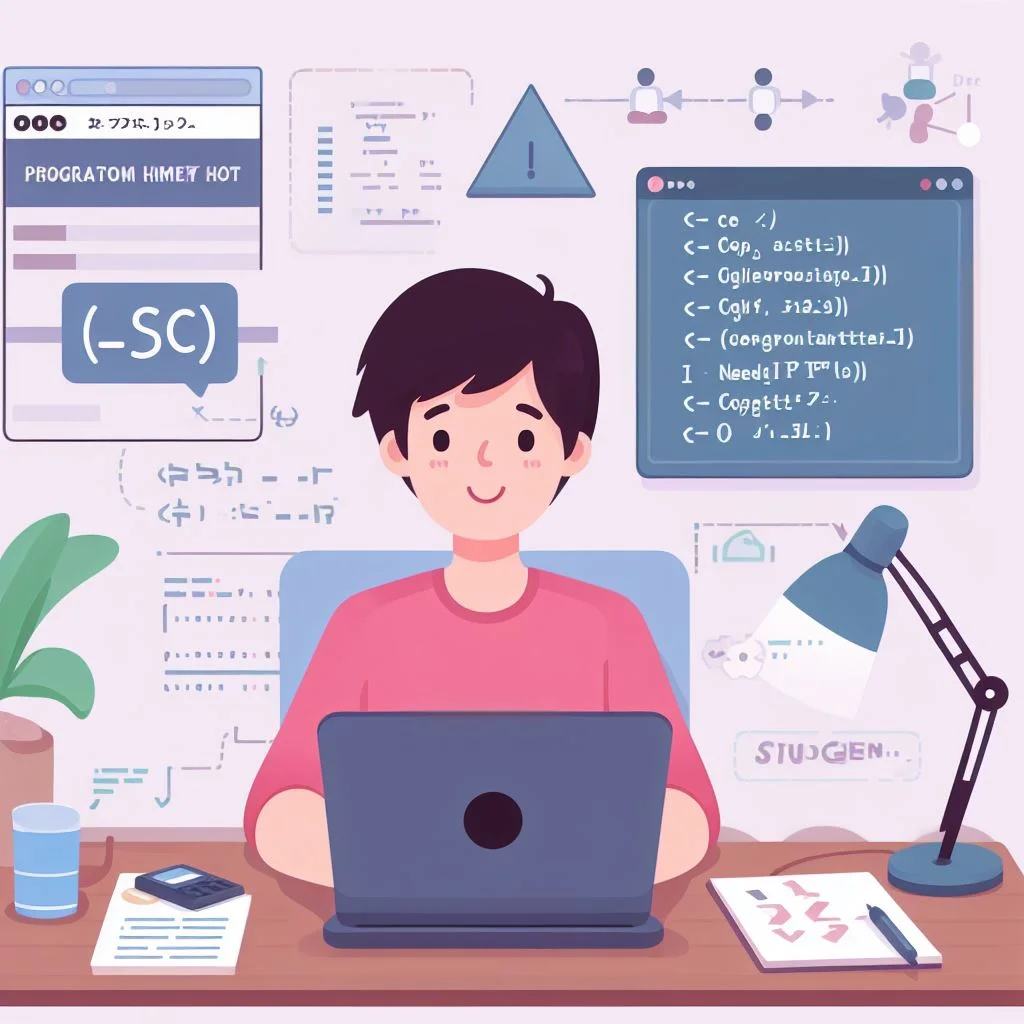
JavaScript serves as the linchpin for transforming static websites into immersive, interactive experiences. The heartbeat of this metamorphosis is event handling—a powerful mechanism that responds to user actions and browser dynamics. In this blog, we embark on an exploration of the pragmatic applications of JavaScript event handling, offering students not just a glimpse, but a deep dive into its myriad possibilities. From refining form validation to crafting real-time data updates, our journey extends into the intricate world of image sliders, dynamic content loading, and drag-and-drop interfaces. This blog is your passport to mastering the nuanced art of event handling, transcending the ordinary to create web applications that captivate and engage. Join us as we unravel the intricacies, explore real-world applications, and empower your web programming assignments with the finesse of JavaScript event handling. Let the coding adventure begin!
Step into the dynamic universe of web development, where JavaScript acts as the orchestrator, conducting symphonies of interactivity on otherwise static web pages. At the core of this orchestration is the elegant dance of event handling—a choreography of responsiveness triggered by users' every click, hover, or input. In this blog, we embark on a journey beyond the syntax, into the practical and creative realms of JavaScript event handling. From validating forms with finesse to seamlessly loading dynamic content, we unravel the intricate threads that weave together real-time data updates, captivating image sliders, and intuitive drag-and-drop interfaces. This blog is not just a guide; it's an invitation to elevate your web programming assignments from mundane to masterful. Join us as we explore the untapped potential of JavaScript event handling, turning lines of code into captivating user experiences. Let your programming prowess shine as we venture into the captivating world of web development's secret sauce—JavaScript events. Ready to code a masterpiece? Let's dive in!
Understanding JavaScript Events
In the realm of web development, events can range from simple actions like clicking a button or submitting a form to more complex interactions such as mouse movements, keyboard inputs, and page load events. JavaScript's event handling mechanism enables developers to capture and respond to these events, making web pages come alive with user-driven interactivity.
Understanding JavaScript events is akin to unlocking the gateway to dynamic and interactive web development. At its core, JavaScript events are the triggers that respond to user interactions or browser actions, allowing developers to infuse life into otherwise static web pages. Beyond the basic syntax lies a rich tapestry of possibilities, where a nuanced understanding of event handling empowers developers to craft seamless, user-centric experiences. In this comprehensive exploration, we'll delve into the intricacies of JavaScript events, unraveling the underlying concepts, and navigating through practical applications that extend far beyond the conventional. Brace yourself for an 800-word journey into the heart of event handling, where every line of code becomes a conduit for captivating user engagement.
At the foundation of JavaScript event handling lies the concept of events as tangible occurrences that happen during the lifecycle of a web page. These occurrences could range from user-initiated actions like clicks and keystrokes to browser-triggered events such as page load or unload. To harness these events, developers employ the addEventListener method, a versatile tool that binds a specific function to a designated event on a selected HTML element. This fundamental syntax, though succinct, serves as the gateway to a myriad of possibilities in creating responsive and dynamic web applications.
Let's dissect this process further. Consider a scenario where a button with the ID "myButton" needs to respond to a user click. The JavaScript code would look like this:
var myButton = document.getElementById("myButton"); myButton.addEventListener("click", function() { // Your event handling code goes here alert("Button clicked!"); });
In this snippet, the addEventListener method is employed to attach a function to the "click" event of the button. When the button is clicked, the associated function executes, triggering an alert with the message "Button clicked!".
Moving beyond the basics, event handling in JavaScript becomes a linchpin for enhancing user experience and interactivity. One practical application lies in the realm of form validation—a critical aspect of web development. Imagine a scenario where a form with the ID "myForm" needs to undergo validation before submission. By utilizing event handling, developers can intercept the form submission, validate the input, and prevent submission if the validation fails.
In this snippet, the addEventListener method is employed to attach a function to the "click" event of the button. When the button is clicked, the associated function executes, triggering an alert with the message "Button clicked!".
var myForm = document.getElementById("myForm"); myForm.addEventListener("submit", function(event) { // Your form validation code goes here if (!validateForm()) { event.preventDefault(); // Prevents form submission if validation fails } }); function validateForm() { // Implement your form validation logic // Return true if validation passes, false otherwise }
In this example, the submit event of the form triggers the validation function. If the validation fails, the event.preventDefault() method is called, preventing the form from being submitted. This showcases how event handling becomes an integral part of ensuring data integrity and user input accuracy.
Dynamic content loading represents another facet of event handling's practical applications. In scenarios where users expect seamless content updates without reloading the entire page, events like "click" can be harnessed to trigger content retrieval and display.
Basic Event Handling Syntax
At the foundation of modern web development lies the intricate dance of interactivity driven by JavaScript events. These events, triggered by user actions or browser dynamics, form the bedrock of creating dynamic, responsive, and engaging web applications. In this exploration, we will immerse ourselves in the fundamental yet profound realm of basic event handling syntax in JavaScript—an initiation into the language's power to seamlessly respond to user input and breathe life into static web pages.
At its essence, JavaScript events are akin to the conduits that bridge the gap between user interactions and a website's response. Be it the click of a button, the submission of a form, or the navigation through a gallery of images, events orchestrate the symphony of user experience. Understanding the basic syntax for handling these events is the initial step in mastering the art of dynamic web development.
The primary method that facilitates event handling in JavaScript is addEventListener. This method serves as a versatile tool that enables developers to associate a specific function with a particular event on a chosen HTML element. The syntax is concise yet powerful, laying the groundwork for a multitude of possibilities.
Consider a scenario where a button in your web page, identified by the ID "myButton," needs to respond to a user's click. The JavaScript code for this event handling would look like the following:
var myButton = document.getElementById("myButton"); myButton.addEventListener("click", function() { // Your event handling code goes here alert("Button clicked!"); });
In this succinct code snippet, we first retrieve the button element from the HTML using document.getElementById("myButton"). Subsequently, the addEventListener method is employed to bind an anonymous function to the "click" event of the button. When the button is clicked, the associated function is executed, triggering an alert with the message "Button clicked!".
This basic syntax provides the foundation for a plethora of interactive elements within a webpage. As students and developers delve into the intricacies of event handling, this foundational structure becomes a canvas on which they can paint a variety of user experiences.
Beyond the simplicity of a button click, the real power of JavaScript event handling unfolds when it comes to form validation—a cornerstone of web development. Consider a scenario where a form, represented by the ID "myForm," requires validation before submission. JavaScript event handling can intercept the form submission, validate the input, and prevent submission if the validation fails.
var myForm = document.getElementById("myForm"); myForm.addEventListener("submit", function(event) { // Your form validation code goes here if (!validateForm()) { event.preventDefault(); // Prevents form submission if validation fails } }); function validateForm() { // Implement your form validation logic // Return true if validation passes, false otherwise }
Dynamic content loading is another practical application of JavaScript event handling. In scenarios where users expect seamless content updates without reloading the entire page, events like "click" can be harnessed to trigger content retrieval and display.
var loadMoreButton = document.getElementById("loadMoreButton"); loadMoreButton.addEventListener("click", function() { // Your content loading code goes here loadMoreContent(); });
Moving beyond singular interactions, the world of image sliders and carousels showcases the prowess of JavaScript event handling in crafting visually appealing and interactive galleries. By utilizing events like "click" on navigation buttons, developers can seamlessly transition between images.
var nextButton = document.getElementById("nextButton"); var prevButton = document.getElementById("prevButton"); nextButton.addEventListener("click", function() { // Your code to show the next image }); prevButton.addEventListener("click", function() { // Your code to show the previous image });
The basic event handling syntax in JavaScript serves as the gateway to a world of interactive possibilities. From the simplicity of a button click to the sophistication of form validation, dynamic content loading, and image sliders, understanding and mastering this syntax is pivotal for anyone venturing into web development. It lays the foundation upon which developers can build immersive user experiences and responsive web applications. As students and aspiring developers navigate the complexities of event handling, they unlock the door to a realm where every user action can be met with a thoughtful and engaging response. This foundational understanding sets the stage for a journey into the broader landscape of JavaScript, where events become the threads that weave together the fabric of a dynamic and user-centric web. Happy coding!
Practical Applications in Web Programming Assignments
Practical applications of JavaScript in web programming assignments encompass a diverse array of scenarios, ranging from enhancing user experience to creating dynamic, data-driven interfaces. In this comprehensive exploration, we'll delve into practical applications that students and developers can leverage to elevate their web programming assignments, showcasing the versatility and power of JavaScript. Embarking on the dynamic journey of web development, the mastery of JavaScript event handling becomes the linchpin for crafting immersive and interactive user experiences. Beyond the foundational syntax lies a realm of practical applications that can elevate web programming assignments to new heights. From ensuring seamless form interactions to dynamically loading content, creating engaging image sliders, facilitating real-time data updates, and implementing intuitive drag-and-drop interfaces, JavaScript's event handling capabilities form the backbone of modern web applications. In this exploration, we unravel the potential of JavaScript events, inviting students and developers to delve into a world where every user action becomes an opportunity to create a more responsive and captivating online experience. Let's dive into the intricate yet immensely rewarding landscape of JavaScript event handling and unleash the full potential of dynamic web development.
In the dynamic landscape of web development, the mastery of JavaScript event handling is akin to wielding a powerful tool that breathes life into static web pages. As developers, students, and enthusiasts embark on the intricate journey of crafting interactive user interfaces, understanding the nuanced syntax of event handling serves as the gateway to a realm of limitless possibilities. Beyond the syntax, it is the practical applications that truly illuminate the prowess of JavaScript events. These applications extend far beyond the conventional, allowing for the creation of seamless form interactions, the dynamic loading of content, visually engaging image sliders, real-time data updates, and the implementation of intuitive drag-and-drop interfaces. JavaScript's event handling capabilities not only respond to user actions but also shape the very essence of user experience, transforming the digital landscape into a canvas where creativity meets functionality. In this exploration, we invite developers to unlock the potential within every click, hover, and keystroke, unleashing the full force of event-driven development. The journey begins where the syntax ends, as we delve into the diverse applications that make JavaScript event handling an indispensable skill in the ever-evolving world of web development. Get ready to harness the power of events and propel your web programming assignments into a realm of innovation and user-centric design.
Form Validation: Ensuring Data Integrity
Form validation is a fundamental aspect of web development, and JavaScript plays a pivotal role in enhancing the user experience by providing real-time feedback on user input. In a form validation scenario, JavaScript event handling intercepts the form submission, allowing developers to validate user inputs before they are sent to the server.
Consider the following example:
var myForm = document.getElementById("myForm"); myForm.addEventListener("submit", function(event) { // Your form validation code goes here if (!validateForm()) { event.preventDefault(); // Prevents form submission if validation fails } }); function validateForm() { // Implement your form validation logic // Return true if validation passes, false otherwise
Real-time Data Updates: Keeping Content Fresh
For web applications that require live updates, JavaScript can facilitate real-time data retrieval and presentation. Technologies like WebSockets or Server-Sent Events can be employed, and JavaScript event handling captures incoming data, enabling dynamic updates without manual page refresh.
// Example using WebSocket
var socket = new WebSocket("ws://example.com"); socket.addEventListener("message", function(event) { // Your code to handle incoming data and update the UI });
In this example, the "message" event from a WebSocket triggers a function to handle incoming data, allowing developers to update the user interface in real-time. This application is particularly relevant for chat applications, live feeds, or any scenario where up-to-the-moment data presentation is essential.
Drag-and-Drop Interfaces: Intuitive User Interactions
Implementing drag-and-drop interfaces is a sophisticated yet rewarding web programming assignment. JavaScript event handling, with events like "dragstart," "dragover," and "drop," enables developers to create interfaces where users can interact with elements in an intuitive and engaging manner.
var draggableElement = document.getElementById("draggableElement"); draggableElement.addEventListener("dragstart", function(event) { // Your code to handle the start of the drag operation }); // Additional event listeners for dragover, dragenter, and drop // Implement the necessary code to handle these events
In this snippet, the "dragstart" event triggers a function to initiate the drag operation, while additional events like "dragover" and "drop" can be employed to manage the ongoing drag-and-drop process. This application demonstrates how event handling can be leveraged to create sophisticated and interactive user interfaces.
Conclusion
Mastering JavaScript event handling is essential for students and developers aiming to create dynamic, interactive, and user-friendly web applications. These practical applications, from form validation to real-time data updates, provide a glimpse into the versatility of JavaScript in web programming assignments. As students delve into these scenarios, they not only solidify their understanding of event handling but also acquire skills that are directly applicable in real-world development scenarios.
By incorporating these practical examples into web programming assignments, students can elevate their coding proficiency and lay the foundation for building modern, dynamic web applications. As the web development landscape continues to evolve, event handling remains a timeless and crucial aspect of creating engaging user experiences. Encouraging students to experiment with different scenarios, explore additional events, and continually enhance their skills will position them at the forefront of web development innovation. Happy coding!
JavaScript event handling is not just a technical necessity; it is an art that transforms lines of code into interactive symphonies. The applications discussed are not isolated silos; rather, they intertwine seamlessly to construct web applications that resonate with users. As students navigate through these practical scenarios, they embark on a journey that goes beyond syntax – a journey that challenges them to think creatively, consider user experience intricacies, and design interfaces that not only function but captivate.
The collaborative nature of these applications also mirrors the reality of modern web development, where functionalities often overlap and blend to create seamless user experiences. For instance, a real-world e-commerce website might incorporate form validation during the checkout process, dynamically load product suggestions based on user behavior, and utilize drag-and-drop interfaces for personalized wish lists. As students delve into these integrative scenarios, they not only hone their technical skills but also develop a holistic understanding of how different aspects of web development converge to deliver comprehensive solutions.
The importance of accessibility cannot be overstated in contemporary web development. JavaScript event handling, when thoughtfully implemented, contributes to creating accessible and inclusive web applications. For instance, ensuring that drag-and-drop interfaces are navigable using keyboard controls or providing alternative mechanisms for users who may not rely on traditional input methods are essential considerations. By instilling these principles early in their education, students can contribute to the development of web applications that prioritize inclusivity and user diversity.