Understanding Non-Linear Regression for Water Flow Analysis using Python
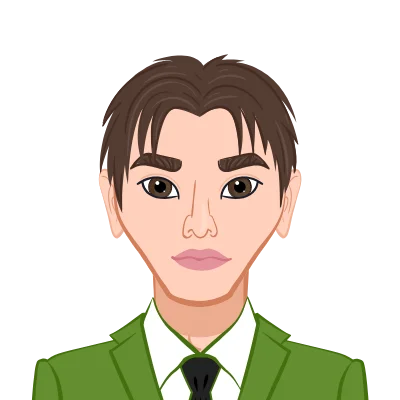
Non-linear regression, a robust statistical tool, finds applications across numerous domains, with hydrology standing out prominently. It proves indispensable for modeling the intricate interplay of variables within hydrology, enabling us to decipher complex relationships. Within the confines of this IPython notebook, we embark on a journey to explore the practical utility of non-linear regression in scrutinizing and forecasting water flow patterns, all designed to do my Non Linear Regression assignment using Python. This acquisition of knowledge presents an invaluable asset, meticulously tailored to aid students grappling with assignments encompassing hydrology, environmental science, or data analysis.
By mastering these non-linear regression techniques, students gain a profound comprehension of the dynamic and multifaceted nature inherent to water flow systems. They uncover the art of utilizing non-linear regression as a powerful tool for predicting future flow trends. In the process, students are poised to extract significant insights from data, empowering them to make well-informed decisions and contributions to the field of hydrology. As the world faces mounting environmental challenges, the ability to harness non-linear regression's potential is a skill that will not only prove advantageous in academia but also contribute to addressing real-world issues effectively.

Prerequisites
Before diving into non-linear regression, let's make sure we have the necessary tools and libraries installed in our Python environment. We'll be using:
- Python 3.x: Python is the linchpin of our analytical toolkit, providing the necessary foundation for our endeavors. We strongly recommend the use of Python 3.x for its compatibility with contemporary practices and access to the latest features. This ensures that we harness the full power of Python's robust ecosystem for our hydrology analysis.
- IPython Notebook: Our analytical journey unfolds within the confines of the IPython Notebook. This interactive and user-friendly environment facilitates seamless learning and experimentation. It empowers us to combine code, text, and visualizations in a coherent narrative, enhancing our understanding and communication of complex concepts.
- NumPy: NumPy assumes a pivotal role in our toolkit, serving as the backbone for numerical operations and array manipulation. Its array objects provide efficiency and versatility, making it an indispensable library for data analysis and scientific computing. NumPy underpins our ability to manipulate and explore our hydrology dataset effectively.
- Matplotlib: Matplotlib emerges as a visual storyteller in our analysis. It equips us with the tools to craft informative visualizations that convey our findings with clarity. By harnessing Matplotlib's capabilities, we can gain deeper insights into the patterns and trends within water flow data and effectively communicate our discoveries.
- SciPy: The scientific and statistical arsenal of SciPy plays a crucial role in our non-linear regression modeling and analysis. Its comprehensive suite of functions empowers us to optimize models, perform statistical tests, and conduct scientific investigations essential for robust hydrology analysis.
- Pandas: Pandas enters the scene as our data manipulation and analysis virtuoso. With its versatile data structures, such as DataFrames, Pandas simplifies the management and exploration of our hydrology dataset. This efficiency enables us to extract meaningful insights from our data while efficiently handling various data-related tasks.
Ensure you have these libraries installed. You can use pip or conda to install them if needed. Setting up the right environment with these tools ensures a smooth and productive journey through our exploration of non-linear regression in water flow analysis. By having these libraries in place, we'll be well-equipped to tackle the complexities of our hydrological data.
```python
!pip install numpy matplotlib scipy pandas
```
Data Preparation
Now that our environment is properly configured, let's focus on data preparation. This crucial step involves loading the water flow dataset, understanding its structure, and cleaning it if necessary. We'll also perform initial exploratory data analysis to gain insights into the data's characteristics.
To get started, let's load the sample water flow dataset using Pandas, one of the powerful libraries we've just installed. Once we have our data in place, we'll visualize it to better understand its patterns and trends. This initial data exploration is essential to inform our subsequent non-linear regression modeling. By taking the time to clean and explore our data, we ensure that our analysis is based on a solid foundation, leading to more accurate and meaningful results.
```python
import pandas as pd
# Load the water flow data
data = pd.read_csv('water_flow_data.csv')
# Explore the dataset
data.head()
```
Visualization
Visualizing the data is a crucial step in any data analysis project. It allows us to gain a deeper understanding of the dataset's characteristics and identify any potential trends or patterns. In our case, we'll create visualizations to represent the water flow data, making it easier to spot any non-linear relationships that may exist. Matplotlib, one of the libraries we've installed, will be our go-to tool for creating these visualizations.
By plotting the data, we'll be able to visualize the changes in water flow over time, helping us identify any potential non-linear trends. These visualizations will serve as a foundation for our non-linear regression modeling, allowing us to make informed decisions about the choice of model and the interpretation of our results.
```python
import matplotlib.pyplot as plt
# Plot the data
plt.figure(figsize=(10, 6))
plt.scatter(data['Time'], data['Flow'], label='Flow Data', color='blue')
plt.xlabel('Time')
plt.ylabel('Flow')
plt.title('Water Flow Data')
plt.legend()
plt.show()
```
Choosing a Non-Linear Model
In the realm of hydrology analysis, selecting an appropriate non-linear model is a pivotal step. The hydrological landscape often demands models that can capture complex relationships within water flow data. Common options include exponential, logarithmic, and power-law models. For our purposes, we opt for the power-law model due to its relevance in hydrological applications. This choice is informed by a fusion of domain expertise and insights gleaned from exploratory data analysis.
The Power-Law Model: The power-law model, a fundamental equation in non-linear regression, takes the form Y=aXb. Here's a breakdown of its components:
- Y - Dependent Variable (Flow): In the context of the power-law model, the variable "Y" represents the dependent variable, which, in our case, is the water flow. This is the phenomenon we are trying to understand and predict. The power-law model allows us to relate "Y" (Flow) to changes in "X" (Time) through a non-linear relationship defined by the parameters "a" and "b."
- X - Independent Variable (Time): "X" is the independent variable in our power-law model, typically representing time in our water flow analysis. This variable is under our control and serves as the input or predictor. As time progresses, we want to understand how it influences changes in water flow, and the power-law model helps us explore this relationship.
- a - Coefficient Scaling Magnitude: The parameter "a" within the power-law model acts as a coefficient that scales the magnitude of the relationship between time (X) and water flow (Y). It determines how changes in time are translated into changes in water flow. A larger "a" value indicates a stronger scaling effect, while a smaller value implies a weaker influence.
- b - Exponent Shaping Relationship: The exponent "b" plays a pivotal role in defining the shape of the relationship between time (X) and water flow (Y). It governs the curvature and direction of the curve in the power-law equation. A positive "b" value implies growth, while a negative value indicates decay. The magnitude of "b" determines the steepness of the curve, influencing the rapidity of change in water flow concerning time.
By embracing the power-law model, our objective is to unearth the underlying patterns concealed within the water flow data. This model accommodates situations where flow exhibits either exponential growth or decay. Its selection reflects our commitment to aligning our analysis with the anticipated behavior of water flow over time, laying a robust foundation for the subsequent steps in our investigation.
Model Fitting
Now that we've chosen the power-law model, the next step is to fit this model to our water flow data. This involves finding the optimal values of a and b that best represent the observed data. To do this, we'll use the powerful optimization capabilities of SciPy, one of the libraries in our toolkit.
The process involves iteratively adjusting the values of a and b to minimize the difference between our model's predictions and the actual flow data. Once we've successfully fit the model, we can extract the parameter values a and b from the optimization results. This step is crucial as it allows us to quantitatively describe the relationship between time and water flow using the power-law equation.
By fitting the model, we transform the raw data into a mathematical representation that can be used for prediction and analysis. This modeling step is the cornerstone of our non-linear regression approach, enabling us to make meaningful insights into water flow dynamics.
```python
from scipy.optimize import curve_fit
# Define the power-law function
def power_law(x, a, b):
return a * x**b
# Fit the model to the data
params, covariance = curve_fit(power_law, data['Time'], data['Flow'])
# Extract the parameters
a, b = params
```
Model Evaluation
To gauge the effectiveness of our non-linear model in capturing the underlying patterns in the water flow data, we turn to model evaluation. An essential metric for assessing the model's performance is the coefficient of determination, denoted as R2. This statistic provides a measure of how well the model fits the observed data. A high R2 value indicates a strong fit, while a lower value suggests that the model may not adequately capture the variation in the data. Alongside R2, visualizing the fitted curve is crucial. Plotting the model's predictions against the actual data points allows us to visually inspect the agreement between the model and the real-world water flow dynamics. This combined approach ensures a comprehensive evaluation of our non-linear regression results, helping us determine the model's reliability and accuracy in the context of hydrology analysis.
```python
import numpy as np
# Generate predicted values using the fitted model
predicted_flow = power_law(data['Time'], a, b)
# Calculate R-squared
residuals = data['Flow'] - predicted_flow
ss_res = np.sum(residuals**2)
ss_tot = np.sum((data['Flow'] - np.mean(data['Flow']))**2)
r_squared = 1 - (ss_res / ss_tot)
# Visualize the fitted curve
plt.figure(figsize=(10, 6))
plt.scatter(data['Time'], data['Flow'], label='Flow Data', color='blue')
plt.plot(data['Time'], predicted_flow, label='Fitted Curve', color='red')
plt.xlabel('Time')
plt.ylabel('Flow')
plt.title(f'Water Flow Data (R-squared: {r_squared:.2f})')
plt.legend()
plt.show()
```
Assignment Example
Now that we have successfully fitted our non-linear model to the water flow data, let's apply our newfound knowledge to a practical assignment example. Consider the following assignment question:
Assignment Question: Predict the water flow at t=100 using the fitted power-law model. Please provide your calculation steps.
This assignment question encapsulates the real-world applicability of non-linear regression in hydrology analysis. By leveraging the power-law model, students can make predictions about future water flow values, a task with significant implications in environmental planning and management. To solve this question, we will apply the parameters obtained from the model fitting process and walk through the step-by-step calculation. This example showcases how students can translate their theoretical understanding of non-linear regression into practical problem-solving skills, a valuable asset in their academic and professional journeys.
```python
# Predict the flow at t = 100
predicted_flow_at_100 = power_law(100, a, b)
print(f'Predicted flow at t=100: {predicted_flow_at_100:.2f}')
```
Conclusion
In summary, non-linear regression emerges as a powerful and indispensable tool for the analysis and prediction of water flow data. Through this comprehensive IPython notebook, we embarked on a journey that encompassed the fundamentals of non-linear regression, encompassing model selection, fitting, evaluation, and the practical application through an assignment example. These fundamental concepts empower students to navigate the intricate landscape of hydrology analysis and data-driven decision-making.
The versatility of non-linear regression transcends academia, finding relevance in real-world scenarios where understanding and forecasting water flow dynamics is paramount. Whether it's aiding environmental planning, managing water resources, or contributing to scientific research, the knowledge gleaned from this notebook equips students with the skills required to tackle complex hydrology challenges.
As a parting note, it's essential to adapt the code, explanations, and visualizations to the specific dataset and assignment requirements encountered in your academic journey. This notebook serves as a robust foundation, guiding students toward a deeper understanding of non-linear regression within the realm of water flow analysis. By applying these concepts and techniques, students can confidently contribute to the evolving field of hydrology and data analysis, making meaningful impacts in the world of environmental science and beyond.