Claim Your Offer
Unlock an amazing offer at www.programminghomeworkhelp.com with our latest promotion. Get an incredible 20% off on your second programming assignment, ensuring top-quality assistance at an affordable price. Our team of expert programmers is here to help you, making your academic journey smoother and more cost-effective. Don't miss this chance to improve your skills and save on your studies. Take advantage of our offer now and secure exceptional help for your programming assignments.
We Accept
- Understanding HTTP and Requests
- Installation and Getting Started
- Making Basic GET Requests
- Handling Parameters and Headers
- Handling POST Requests
- Dealing with Authentication
- Advanced Features: Session Management
- Error Handling and Status Codes
- Taking Control of Errors
- Conclusion
Navigating the realm of Python programming frequently involves managing HTTP requests, a ubiquitous task whether constructing web scrapers, engaging with APIs, or simply retrieving data through HTTP. Streamlining this process is crucial, demanding a dependable and user-friendly library to enhance workflow efficiency. Enter the Requests library – a pivotal player simplifying HTTP intricacies in Python projects. This blog post delves into the multifaceted ways in which the Requests library proves invaluable, elucidating its role in facilitating seamless interactions with web services, APIs, and data retrieval. Whether you're a novice or seasoned developer, integrating the Requests library into your coding repertoire promises heightened efficiency and code readability. Join us on an exploration of how this library empowers Python developers to navigate the intricacies of HTTP with ease. Whether you're exploring HTTP interactions in Python or need help with your Python assignment, incorporating the Requests library into your toolkit can significantly enhance your ability to work with web services and APIs efficiently.
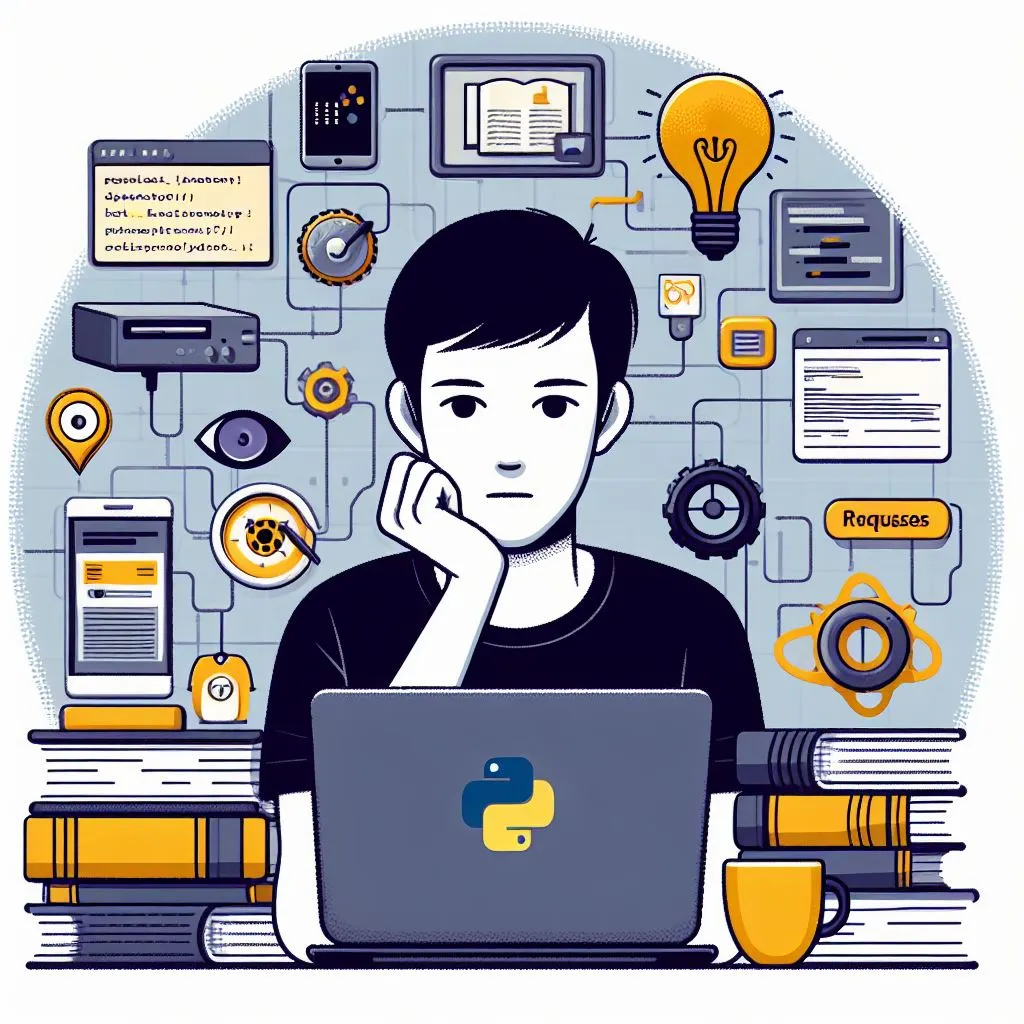
Understanding HTTP and Requests
Delving into the intricacies of Python's Requests library necessitates a foundational grasp of HTTP (Hypertext Transfer Protocol). Serving as the cornerstone for data exchange on the Web, HTTP facilitates the transmission of hypermedia documents like HTML. Whether initiating a web page view or executing an API call, these actions entail sending an HTTP request to a server, triggering the retrieval of desired information. Python's Requests library emerges as a potent instrument in this landscape, adept at abstracting the complexities inherent in crafting HTTP requests. Distinguished by its straightforward and refined API, the library significantly simplifies the interaction between developers and web services, offering an elegant solution for data retrieval within Python projects.
Installation and Getting Started
Getting started with the Requests library is a breeze. If you haven't installed it yet, you can do so using the following command:
```bash pip install requests ```
Once the installation process is complete, you'll find that incorporating the Requests library into your Python projects is seamless. Begin by importing the library into your scripts:
```python import requests ```
This straightforward installation process ensures that developers, whether novices or seasoned professionals, can swiftly integrate the library into their projects. With just a single command, you gain access to a powerful toolset for managing HTTP requests in Python. This simplicity aligns with the Pythonic philosophy of keeping things clear and concise, making it an excellent choice for enhancing your workflow.
Moreover, the Requests library seamlessly integrates with virtual environments, allowing for a clean and isolated development environment. This ensures that dependencies are managed efficiently, and projects maintain consistency across different systems and collaborators. The straightforward installation process coupled with compatibility features makes the Requests library an essential component in the toolkit of any Python developer aiming for efficiency and maintainability.
Making Basic GET Requests
The Requests library simplifies the process of making basic GET requests, a fundamental operation in web development. Consider the following example:
```python import requests response = requests.get('https://api.example.com/data') print(response.text) ```
Here, the GET request is directed to a hypothetical API endpoint, showcasing the simplicity of the Requests library. The response object, a key component, provides a wealth of information, encompassing the status code, headers, and the response body. This feature-rich response object empowers developers to gain insights into the server's acknowledgment, understand the data structure, and implement tailored logic based on the specific use case. Whether you're a beginner exploring HTTP interactions or an experienced developer seeking efficiency, the clarity and versatility of the Requests library in handling basic GET requests make it an essential tool in your Python toolkit. Additionally, the library's seamless integration with various response formats, such as JSON or XML, further enhances its utility, offering flexibility in data processing.
Handling Parameters and Headers
In the realm of web development, interacting with APIs often requires the inclusion of parameters and headers in your requests, a task made remarkably straightforward by the Requests library. Consider this illustrative example:
```python import requests url = 'https://api.example.com/search' params = {'query': 'python', 'type': 'programming'} headers = {'Authorization': 'Bearer YOUR_API_KEY'} response = requests.get(url, params=params, headers=headers) print(response.json()) ```
Here, a GET request is dispatched to a search endpoint, equipped with query parameters and an authorization header. This scenario mirrors the common use case of querying specific data while ensuring secure access to the API. The Requests library excels in abstracting the intricacies of parameter and header management, enabling developers to construct elegant and readable code. The inclusion of an authorization header in this example demonstrates the library's support for authentication mechanisms, a critical aspect of secure API interactions. Furthermore, the ability to parse the response as JSON showcases the library's versatility in handling different data formats, providing a solid foundation for subsequent data processing and analysis in your Python projects.
Handling POST Requests
Beyond the realm of GET requests, the Requests library extends its capabilities to support various HTTP methods, prominently including POST. This becomes invaluable when interacting with servers to submit data, create resources, or engage in other write operations. Here's an example to illustrate:
```python import requests url = 'https://api.example.com/create' data = {'name': 'John Doe', 'email': 'john@example.com'} response = requests.post(url, data=data) print(response.status_code) ```
In this instance, a POST request is initiated to create a resource using the specified data. The Requests library simplifies the process of sending data payloads, be it form submissions or resource creation, with its intuitive post method. The response object, as always, provides essential feedback, including the status code, allowing developers to ascertain the success of the operation. Leveraging POST requests with the Requests library not only enhances the versatility of your Python projects but also ensures a seamless integration of data-intensive functionalities. Whether you're building an application that captures user inputs or interacting with APIs to modify resources, the Requests library remains a robust companion in facilitating these operations with clarity and efficiency.
Dealing with Authentication
In the dynamic landscape of web development, securing API requests stands as a paramount concern, and the Requests library addresses this by providing support for various authentication methods. Let's explore an example that employs basic authentication:
```python import requests from requests.auth import HTTPBasicAuth url = 'https://api.example.com/private' username = 'your_username' password = 'your_password' response = requests.get(url, auth=HTTPBasicAuth(username, password)) print(response.text) ```
This example showcases the implementation of basic authentication, a fundamental approach to secure API interactions. The Requests library seamlessly integrates authentication mechanisms, allowing developers to fortify their requests with credentials effortlessly. Basic authentication, as depicted here, involves sending a GET request to a private endpoint with a specified username and password. The extensibility of the Requests library in accommodating various authentication methods, such as OAuth or API tokens, empowers developers to tailor security measures according to the requirements of their projects. Whether you're accessing sensitive data or performing privileged operations, the ability to seamlessly implement authentication ensures the integrity and confidentiality of your interactions within Python projects.
Advanced Features: Session Management
In navigating the intricacies of web interactions, the Requests library goes beyond the basics and offers advanced features such as session management. This proves invaluable in handling more complex scenarios where persistent parameters need to span multiple requests – a common need when dealing with authentication tokens or cookies. Let's delve into an illustrative example:
```python import requests url = 'https://api.example.com/login' login_data = {'username': 'your_username', 'password': 'your_password'} # Create a session session = requests.Session() # Login and persist the session session.post(url, data=login_data) # Make subsequent requests using the same session response = session.get('https://api.example.com/dashboard') print(response.text) ```
In this scenario, a session is initiated using requests.Session(), providing a container to persist data across requests. The example illustrates the creation of a session, followed by a login operation, showcasing how the library manages and retains information like authentication tokens. Subsequent requests, such as accessing a dashboard, can then leverage the existing session without the need for re-authentication. This powerful feature not only simplifies code structure but also optimizes performance, especially in scenarios involving multiple sequential requests within a single user session. Incorporating session management in your Python projects using the Requests library enhances your ability to handle complex workflows seamlessly.
Error Handling and Status Codes
Ensuring robust error handling is a crucial aspect of working with HTTP requests, and the Requests library simplifies this process with its intuitive error handling mechanisms. Consider the following example:
```python import requests url = 'https://api.example.com/data' try: response = requests.get(url) response.raise_for_status() # Raises an HTTPError for bad responses print(response.text) except requests.exceptions.HTTPError as err: print(f"HTTP error occurred: {err}") except Exception as err: print(f"An unexpected error occurred: {err}") ```
In this demonstration, the raise_for_status() method is employed to raise an exception in the event of HTTP errors, allowing for a graceful and context-aware error handling approach. This ensures that developers can tailor their responses based on the specific error encountered, distinguishing between client and server-side issues. Furthermore, the use of a broad Exception catch-all block provides a safety net for unexpected errors, enhancing the robustness of error handling in Python projects. By incorporating these error handling practices, developers can create resilient applications that gracefully handle unforeseen circumstances, contributing to the reliability and stability of their HTTP interactions.
Taking Control of Errors
In the dynamic landscape of web development, understanding and managing errors is paramount. The provided Python example showcases the Requests library's prowess in handling HTTP errors with finesse. The raise_for_status() method emerges as a key player, triggering an exception when encountering undesirable HTTP responses.
In the specific context of requests.exceptions.HTTPError, developers gain insight into the nature of the HTTP error, enabling tailored responses. Whether it's a client-side misstep or a server-side hiccup, the granular control over error types facilitates a more nuanced and user-friendly approach.
Beyond HTTP-specific errors, the broad Exception catch-all block serves as a safety net for unexpected issues. This catch-all approach enhances the resilience of the error-handling mechanism, ensuring that unforeseen circumstances are addressed gracefully.
By incorporating such robust error-handling practices into Python projects, developers not only fortify their applications against potential pitfalls but also contribute to a more reliable and stable user experience. The Requests library's commitment to effective error management empowers developers to build applications that not only thrive under optimal conditions but gracefully navigate the challenges presented by real-world scenarios.
Conclusion
In conclusion, the Requests library emerges as an indispensable asset for streamlining HTTP processes within Python projects. Renowned for its user-friendly API and comprehensive features, it stands out as the preferred option for developers engaged in tasks ranging from managing web services and APIs to retrieving crucial data. Whether you are a novice navigating the intricacies of Python programming or an experienced developer seeking to optimize your workflow, integrating the Requests library proves transformative. By doing so, you not only bolster the efficiency of your code but also enhance its readability, making it more accessible and comprehensible. Embrace the opportunity to delve into the library's versatile capabilities and unlock the full potential of HTTP in your Python projects. As you embark on this coding journey, may your experience be filled with productive and enjoyable moments. Happy coding!