Claim Your Offer
Unlock an amazing offer at www.programminghomeworkhelp.com with our latest promotion. Get an incredible 20% off on your second programming assignment, ensuring top-quality assistance at an affordable price. Our team of expert programmers is here to help you, making your academic journey smoother and more cost-effective. Don't miss this chance to improve your skills and save on your studies. Take advantage of our offer now and secure exceptional help for your programming assignments.
We Accept
- XNA Overview
- Sprite Animation Basics
- Creating a C# Class for 2D Animated Sprites
- Step 1: Setting Up Your XNA Project
- Step 2: Create a Sprite Class
- Step 3: Using the AnimatedSprite Class
- Step 4: Implementing Sprite Animation States
- Conclusion
Game development is a thrilling domain that merges the realms of creativity and programming prowess, yielding interactive and captivating digital experiences. An indispensable facet within this multifaceted field revolves around the art of animating game elements, encompassing characters, and in-game items. Within the confines of this blog, we will embark on an enlightening journey, delving into the intricacies of crafting a C# class tailored explicitly to bolster the animation of 2D sprites. Our chosen tool for this endeavor is XNA, a widely acclaimed game development framework that empowers developers to bring their imaginative visions to life. The insights and skills gleaned from this exploration are poised to become a priceless asset for students keen on not just tackling but excelling in their game development assignments and ambitious projects. So, if you're ready to immerse yourself in the world of game development, learn how to write your C# assignment, and embark on the creation of enthralling animations, this blog is your stepping stone towards that exciting journey.
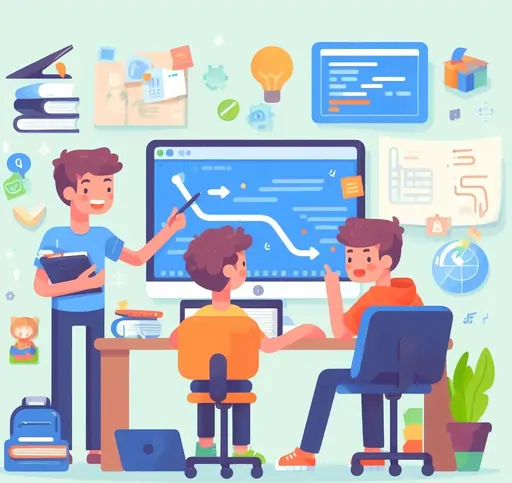
XNA Overview
XNA, short for "XNA's Not Acronymed," stands as a robust game development framework crafted by the brilliant minds at Microsoft. Its primary mission is to furnish game creators with an arsenal of tools and libraries, tailored to fashion immersive gaming experiences on both Windows and Xbox platforms. At its core, XNA is a developer's ally, simplifying the intricate landscape of game development. It shines particularly in rendering graphics, orchestrating audio, and deftly handling user input.
What sets XNA apart is its affinity for the C# programming language, making it an ideal choice for budding game developers and students alike. C#, known for its readability and approachability, provides an accessible entry point for those eager to delve into the world of game development. XNA marries the power of C# with game development wizardry, granting individuals the ability to bring their gaming visions to life with relative ease.
Whether you're a student embarking on a game development journey or an aspiring game creator, XNA serves as a bridge between your creative aspirations and the captivating world of interactive digital experiences.
Sprite Animation Basics
Before we embark on the creation of our 2D animated sprite class, it's crucial to lay a foundation by understanding the fundamentals of sprite animation. Within the realm of gaming, a sprite serves as a fundamental building block—a 2D image that assumes the role of a game entity, be it a hero, a foe, or an in-game item. These sprites form the visual essence of the gaming world.
Sprite animation, a pivotal technique, revolves around orchestrating these 2D images in a harmonious dance. It's akin to flipping through a flipbook's pages in rapid succession, where each page is a slightly altered depiction of the scene. Similarly, in sprite animation, a sequence of these sprites is displayed swiftly, creating the mesmerizing illusion of movement. It breathes life into the game, endowing characters with the ability to walk, monsters to roar, and projectiles to soar through the digital realm.
Mastering sprite animation is akin to wielding a magician's wand, transforming static images into dynamic, pulsating elements that immerse players in the captivating tapestry of the gaming universe.
Key components of sprite animation include:
- Spritesheet:At the heart of sprite animation lies the spritesheet, a singular image housing a multitude of animation frames organized meticulously in a grid. Each frame within this visual compendium captures a distinct state or pose of the animated object. This resource-efficient approach ensures that all necessary frames are contained within a single image, ready to be summoned when the animation cues.
- Frame Rate:The frame rate governs the pace at which these frames come to life. It dictates how swiftly the individual frames are displayed in succession. For 2D sprite animation, a typical and visually pleasing frame rate hovers around 60 frames per second (FPS). This harmonious rhythm creates the illusion of fluid motion, immersing players in the game world.
- Animation State: The animation state serves as the narrative thread weaving through the frames. It represents the present condition or action of the animated entity, whether it's in an "idle" stance, "walking" with purpose, or "jumping" into the unknown. Each animation state triggers a specific sequence of frames, choreographing the character's behavior and interactions within the game's dynamic tapestry.
Creating a C# Class for 2D Animated Sprites
In the journey to bring 2D animated sprites to life within the XNA framework, we embark on the pivotal step of crafting a specialized C# class. This class will serve as the bridge between the static image of a sprite and its dynamic, animated counterpart. As we venture forward, we'll dissect the process into comprehensible steps, ensuring that students, even those new to game development, can confidently follow along. With each step, we'll unlock the secrets of initializing, updating, and rendering sprites, propelling students towards the realm of game development excellence. This foundational knowledge forms the cornerstone upon which captivating games are built, empowering students to infuse their creative visions with the magic of animation. So, prepare to delve into the world of code and creativity as we embark on the journey of crafting a C# class that breathes life into 2D animated sprites.
Step 1: Setting Up Your XNA Project
Before we can dive into crafting our sprite class, establishing the XNA project environment is paramount.
Follow these initial steps:
- Create a new Windows Game (4.0) project in Visual Studio. Begin by launching Visual Studio and selecting the "New Project" option. Choose the "Windows Game (4.0)" template to lay the foundation for your game development venture.
- Name your project and choose a location to save it. Naming your project is the first step in defining its identity. Select a meaningful and memorable name, and specify the location where your project files will be stored.
- Ensure that you have the necessary XNA libraries referenced. Building a game within the XNA framework requires access to its essential libraries. To do this, navigate to the Solution Explorer, right-click on "References," and select "Add Reference." This action will grant your project the power it needs to harness the capabilities of XNA.
With these initial setup steps, you're ready to embark on your game development journey within the XNA environment.
Step 2: Create a Sprite Class
With your XNA project established, the next crucial step is the creation of a specialized C# class named "AnimatedSprite." This class will serve as the heart of your sprite animation system, responsible for key operations such as loading, updating, and rendering animated sprites.
Below is a simplified representation of the class structure:
```csharp
using Microsoft.Xna.Framework;
using Microsoft.Xna.Framework.Graphics;
public class AnimatedSprite
{
private Texture2D spriteSheet;
private Rectangle[] frames;
private int currentFrame;
private float frameTime;
private float timeElapsed;
public Vector2 Position { get; set; }
public Vector2 Velocity { get; set; }
public float Rotation { get; set; }
public Color Color { get; set; }
public float Scale { get; set; }
public AnimatedSprite(Texture2D spriteSheet, int frameCount, float frameTime)
{
this.spriteSheet = spriteSheet;
this.frameTime = frameTime;
this.Scale = 1f;
int frameWidth = spriteSheet.Width / frameCount;
frames = new Rectangle[frameCount];
for (int i = 0; i < frameCount; i++)
{
frames[i] = new Rectangle(i * frameWidth, 0, frameWidth, spriteSheet.Height);
}
}
public void Update(GameTime gameTime)
{
timeElapsed += (float)gameTime.ElapsedGameTime.TotalSeconds;
if (timeElapsed > frameTime)
{
timeElapsed -= frameTime;
currentFrame = (currentFrame + 1) % frames.Length;
}
Position += Velocity;
}
public void Draw(SpriteBatch spriteBatch)
{
spriteBatch.Draw(spriteSheet, Position, frames[currentFrame], Color, Rotation, Vector2.Zero, Scale, SpriteEffects.None, 0);
}
}
```
- Loading a Spritesheet: The "AnimatedSprite" class, at its core, handles the loading of a spritesheet—a single image containing multiple frames arranged in a grid. These frames capture different states or poses of your animated object.
- Updating the Animation: Animation in games thrives on the orchestration of these frames, and the class efficiently manages this process. It calculates the passage of time and ensures that frames are updated according to your specified frame rate.
- Drawing the Sprite: Finally, the "AnimatedSprite" class possesses the capability to draw the sprite on the game screen. It leverages the graphics capabilities of XNA to bring your animated character or object to life within the game world.
By creating this essential "AnimatedSprite" class, you're laying the foundation for dynamic, visually captivating game experiences that will engage players and enhance your game development skills.
Step 3: Using the AnimatedSprite Class
Now that you've crafted your powerful "AnimatedSprite" class, it's time to put it to work within your game. Here's how to seamlessly integrate it into your game project:
- Loading the Spritesheet Image:Begin by importing your spritesheet image into your project's content folder. Simply right-click on the "Content" folder, select "Add," and then choose "Existing Item" to import your meticulously designed spritesheet. This step ensures that your animated sprites have the visual essence they need to come to life.
- Creating an AnimatedSprite Instance: In your game's "LoadContent" method, initiate an instance of the "AnimatedSprite" class and load your spritesheet into it. This pivotal step initializes the core of your animated character, providing it with the frames it needs to animate.
```csharp
Texture2D spriteSheet = Content.Load("spritesheet");
AnimatedSprite playerSprite = new AnimatedSprite(spriteSheet, frameCount, frameTime);
```
- Updating the AnimatedSprite:Within your game's "Update" method, make sure to regularly update your "playerSprite" instance. By doing so, you ensure that the animation sequence progresses as intended, creating a seamless and engaging visual experience for your players.
```csharp
playerSprite.Update(gameTime);
```
- Drawing the AnimatedSprite: Finally, in your game's "Draw" method, use the "SpriteBatch" to draw the "playerSprite" onto the game screen. This action brings your animated character to the forefront, allowing players to interact with and enjoy the fruits of your game development labor.
```csharp
spriteBatch.Begin();
playerSprite.Draw(spriteBatch);
spriteBatch.End();
```
Step 4: Implementing Sprite Animation States
To elevate your game's animation to more sophisticated levels, it's essential to implement different animation states within the "AnimatedSprite" class. This approach allows for the seamless transition between various character behaviors or in-game actions. Here's how you can expand the functionality of your "AnimatedSprite" class:
```csharp
public void ChangeAnimation(string state, int frameCount, float frameTime)
{
// Set the frames, frame count, and frame time based on the animation state.
}
```
- Adding Animation States:Begin by defining different animation states, such as "idle," "walking," "jumping," or any specific actions your game requires. Each state represents a unique sequence of frames, encapsulating the distinct visual aspects of the character or object during that state.
- Changing Animation States: Create methods within your "AnimatedSprite" class that enable you to switch between these animation states dynamically. By invoking these methods in response to in-game events or conditions, you can seamlessly transition your character's appearance and behavior.
- Customizing Frame Counts and Frame Times: Depending on the animation state, you can adjust the frame count and frame time. This level of customization empowers you to control the speed and fluidity of animations, ensuring that they align perfectly with your game's pacing and aesthetics.
With the implementation of animation states, you unlock the ability to imbue your characters and objects with a rich array of behaviors and movements, enhancing the overall depth and immersion of your game.
Conclusion
In this blog, we've explored how to create a C# class to support 2D animated sprites using XNA, a valuable skill for students interested in game development. Understanding sprite animation and the basics of XNA is crucial for building interactive and visually appealing games. With the provided `AnimatedSprite` class, students can jumpstart their game development assignments and projects, creating exciting games that showcase their programming skills and creativity.
By mastering the fundamentals of game development, students can unlock their potential to create immersive and entertaining gaming experiences that captivate players around the world. Happy coding!