Claim Your Offer
Unlock an amazing offer at www.programminghomeworkhelp.com with our latest promotion. Get an incredible 10% off on your all programming assignment, ensuring top-quality assistance at an affordable price. Our team of expert programmers is here to help you, making your academic journey smoother and more cost-effective. Don't miss this chance to improve your skills and save on your studies. Take advantage of our offer now and secure exceptional help for your programming assignments.
We Accept
- Python Classes and Objects: Key Concepts for Outstanding Homework:
- Understanding Python Classes: The Blueprint
- Python Objects: Bringing Blueprints to Life
- Constructors: Creating Personalized Objects
- Relationships are strengthened by contrasting composition and inheritance.
- Static vs. instance methods for attributes
- Understanding Complex Object-Oriented Principles to Produce Outstanding Homework
- Conclusion:
Welcome to our comprehensive blog on "Understanding Classes and Objects in Python: Key Concepts for Homework Excellence." Python's object-oriented programming (OOP) paradigm is a crucial aspect of the language that every aspiring developer must grasp to build efficient and scalable applications. At the heart of OOP in Python lies the concept of classes and objects, which allow developers to model real-world entities and abstract concepts seamlessly.
In this blog, we will delve deep into the fundamentals of classes and objects in Python, exploring their significance in the programming landscape. Whether you are a beginner eager to expand your programming horizons or an experienced developer looking to reinforce your knowledge, this blog is designed to equip you with the essential skills required for handling Python Homework like a pro.
Get ready to unlock the power of Python classes and objects, and discover how they can be utilized to design modular, maintainable, and scalable code. From defining classes and creating objects to exploring inheritance and polymorphism, we leave no stone unturned in our pursuit of providing you with an in-depth understanding of this pivotal OOP concept.
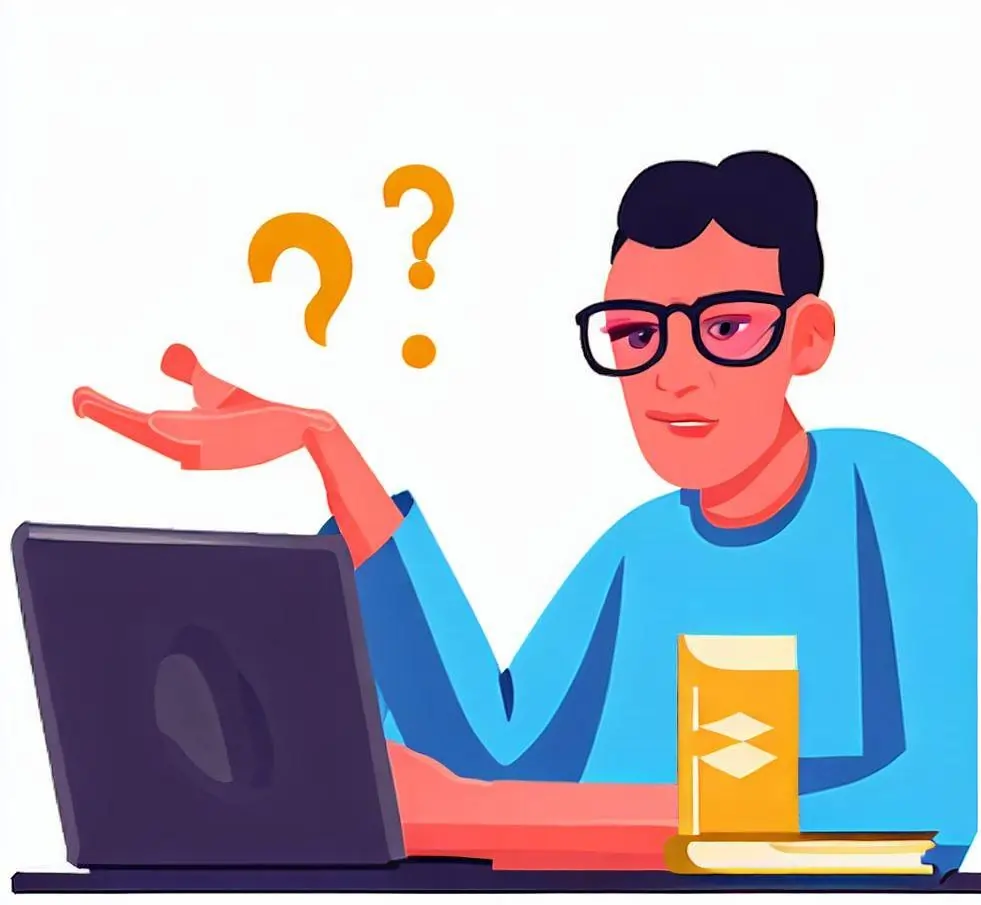
So, buckle up, as we embark on a journey that will transform your coding capabilities and elevate your Python Homework to the pinnacle of excellence.
Python Classes and Objects: Key Concepts for Outstanding Homework:
Both inexperienced developers and seasoned professionals are drawn to Python since it is one of the most widely used and flexible programming languages. The fundamental ideas of "classes" and "objects" are at the heart of object-oriented programming (OOP) in Python. Understanding these ideas may make the difference between turning in a programming Homework that is well-organized and effective and one that barely gets by. This deep dive into classes and objects will help you complete your programming homework in the direction of greatness whether you're a student trying to ace that forthcoming project or a curious person exploring Python.
It is essential to comprehend the underlying paradigm that underpins classes and objects, Object-Oriented Programming (OOP), before delving into the specifics of objects and classes. At its core, object-oriented programming (OOP) is a paradigm for computer programming that uses "objects" (instances of classes) to arrange and represent data and functions. OOP combines code and data instead of keeping them separate, creating a more organized and user-friendly programming environment. Since the world is seen in this paradigm as a collection of interconnected items, modeling complicated systems is easier to understand and more in line with how humans really see things.
Understanding Python Classes: The Blueprint
Think of yourself as an architect. A plan that specifies a building's design, measurements, and purpose is necessary before construction can begin. A "class" in the context of Python OOP is comparable to this design. A class describes the characteristics (data) and methods (functions) an object type may do in order to define that object type.
Take the traditional illustration of modeling a car:
class Car: # Attributes make = "" model = "" color = "" # Methods def drive(self): print(f"The {self.color} {self.make} {self.model} is now driving.") def park(self): print(f"The {self.color} {self.make} {self.model} is parked.")
The Car class in this crude representation contains two methods, drive and park, as well as three attributes: make, model, and color. However, this class is conceptual. It provides a theoretical foundation but is not a vehicle in and of itself. We would need to design objects in order to leverage this blueprint and produce distinct automobile instances.
Python Objects: Bringing Blueprints to Life
A concrete instance of a class is an object. Once the class (blueprint) has been established, we are free to construct as many objects (buildings) out of it as we see fit. Making use of the Car class from before, creating a car object would entail:
my_car = Car() my_car.make = "Toyota" my_car.model = "Camry" my_car.color = "red"
An instance of the Car class makes up this my_car object. It is a real thing with details like "Toyota" for the brand, "Camry" for the model, and "red" for the color. Utilize the class-defined methods to communicate with this car:
my_car.drive() # Output: The red Toyota Camry is now driving.
Constructors: Creating Personalized Objects
The way it is shown above is often not how we would want to generate objects in real-world programming. When an object is formed, Python classes often use the special function __init__ (sometimes referred to as a constructor) to initialize its attributes:
class Car: def __init__(self, make, model, color): self.make = make self.model = model self.color = color
With this configuration, establishing a new vehicle instance is simplified:
my_car = Car("Toyota", "Camry", "red")
This method guarantees attributes are set during object creation and improves the readability of the code.
1. Embedding: Protecting Internal Mechanisms
Encapsulation, which includes limiting access to certain areas of an object, is one of the fundamental concepts of OOP. This guards against outside intervention and data exploitation. Using the private and protected access modifiers in Python, this is accomplished. According to tradition, properties that begin with an underscore (_) are protected, whereas those that begin with double underscores (__) are private:
class Car: def __init__(self, make, model): self._color = "Red" # protected attribute self.__engine_status = "Off" # private attribute
Maintaining data integrity and making sure the object stays in a usable state need encapsulation above all else.
2. Polymorphism and Inheritance: Promoting the Evolution of Classes
Classes do not exist independently. One class often extends or borrows features from another, a process known as "inheritance". It permits the development of new classes that are modified iterations of existing classes. The new class is the "child" or "derived" class, while the previous class is the "parent" or "base" class.
On the other side, polymorphism allows for flexibility. Through inheritance, it is possible to consider distinct classes as instances of the same class. In situations when the precise kind of the item could be uncertain, it is particularly important since it allows for generic behaviour.
Your pursuit of perfection will unavoidably be fueled by the incorporation of a deep grasp of classes and objects into your Python Homework Help. You may deconstruct your Homework challenges into more manageable parts by looking at them through the OOP lens and identifying their links and hierarchies. Mastery of these ideas paves the way for more complex software development projects, whether it is via the creation of intuitive classes, the definition of clear objects, or the use of inheritance and polymorphism. Keep in mind that the essence of any sophisticated piece of software is a harmonious interaction between well defined classes and objects.
Relationships are strengthened by contrasting composition and inheritance.
One must recognize the nuances in how classes connect beyond the fundamental concept of classes and objects. "Composition" and "inheritance" are the two main ways classes come together. While heredity has been briefly discussed, contrasting it with composition provides more profound truths.
As was previously said, inheritance involves taking characteristics and traits from a parent class to create a new class. For instance, a Car class can derive from a generic Vehicle class, meaning that every car is a vehicle. But inheritance may have its limitations. Overusing inheritance may result in superclasses that are too big and difficult to manage, or in child classes gaining traits they don't require.
Contrarily, composition denotes a "has-a" connection. Rather of inheriting properties from a base class, it entails creating classes by mixing different parts. For instance, our Car class may include an Engine object, a Wheel object, and more in instead of deriving from a Vehicle class. Inferring that an automobile "has an" engine and "has" wheels is obvious.
In essence, while composition denotes a "has-a" connection (a car has an engine), inheritance denotes a "is-a" relationship (a car is a vehicle). Understanding the essence of the issue is necessary to choose amongst them. Composition often provides more flexibility by making it simple to switch out components and guaranteeing that each class is solely responsible for that obligation.
1. Increased Flexibility via Method Overriding and Overloading
As we go further into polymorphism, we come across overloading and method overriding. Method overriding refers to when a child class offers a customized implementation for a method already declared in its parent class. It enables the child class to independently specify distinct behaviors while also allowing it to inherit methods from the parent class. class Vehicle: def description(self): return "A generic vehicle" class Car(Vehicle): def description(self): # Overriding the parent method return "A four-wheeled vehicle"
However, method overloading refers to having many methods with the same name but different arguments in the same class. Unlike some other languages, Python does not natively enable method overloading, but it does provide means to implement a comparable capability using default parameters and variable-length argument lists.
Static vs. instance methods for attributes
Understanding the difference between static and instance members is essential for class design. Each object's instance properties are unique. On the other hand, all instances of the class share static characteristics. Similar to how instance methods use self to access instance data, static methods apply to the class as a whole and don't need to be called on an instance.
One may create static methods by using the @staticmethod decorator:
class Car: total_cars = 0 # static attribute def __init__(self, make): self.make = make # instance attribute Car.total_cars += 1 @staticmethod def total_car_count(): # static method return Car.total_cars
Understanding Complex Object-Oriented Principles to Produce Outstanding Homework
The path to grasping classes and objects in Python doesn't stop with only comprehending the fundamental definitions of these concepts. It involves understanding method dynamics, recognizing the depth of links between classes, deciding whether to utilize inheritance over composition, and making the most of both static and instance members.
Incorporating these smart ideas into your tasks demonstrates both your mastery of Python and your capacity to design complicated solutions. By consistently improving these abilities, you distinguish yourself and make sure that your Python Homework are not just accurate but also excellent in terms of design and organization.
Conclusion:
In conclusion, a solid grasp of classes and objects in Python is an indispensable skill for any programmer aiming to achieve Homework excellence. Object-oriented programming empowers developers to create clean, organized, and efficient code by encapsulating data and functionality into self-contained units.
Throughout this blog, we have explored the core concepts of Python's classes and objects, emphasizing their role in designing robust applications. From defining classes with attributes and methods to creating instances and understanding the principles of inheritance and polymorphism, each aspect of OOP in Python has been unraveled.
To truly excel in Python Homework, aspiring programmers should embrace the object-oriented paradigm wholeheartedly. Mastery over classes and objects empowers developers to craft elegant and elegant solutions, ultimately setting them apart as accomplished Python developers.