Claim Your Offer
Unlock an amazing offer at www.programminghomeworkhelp.com with our latest promotion. Get an incredible 10% off on your all programming assignment, ensuring top-quality assistance at an affordable price. Our team of expert programmers is here to help you, making your academic journey smoother and more cost-effective. Don't miss this chance to improve your skills and save on your studies. Take advantage of our offer now and secure exceptional help for your programming assignments.
We Accept
- The Python Language's Basic Exceptions
- Handling Exceptions
- Techniques for Debugging Homework Exceptions:
- Record Your Error Handling Strategy:
- Advanced Techniques for Handling Errors:
- Error Handling Techniques for Particular Situations:
- Conclusion:
Welcome to our comprehensive guide on "Error Handling in Python: A Guide to Resolving Homework Exceptions." As a Python programmer, you might have encountered various errors during your coding journey. Understanding how to handle these errors effectively is crucial to ensuring the smooth execution of your code and delivering robust applications.
It is essential to develop a thorough understanding of common exceptions in Python, including NameError, TypeError, and ValueError, among others. By mastering these exceptions, you'll be better equipped to troubleshoot and resolve errors effectively, leading to more efficient and reliable code.
In this guide, we have compiled valuable insights, best practices, and real-world examples to enhance your error handling skills. Whether you're a beginner looking to grasp the basics or an experienced Python developer seeking to fine-tune your error resolution techniques, this guide is tailored to cater to all skill levels.
Let's embark on this journey to explore the art of error handling in Python and equip ourselves with the necessary knowledge to become adept problem solvers in the realm of programming.
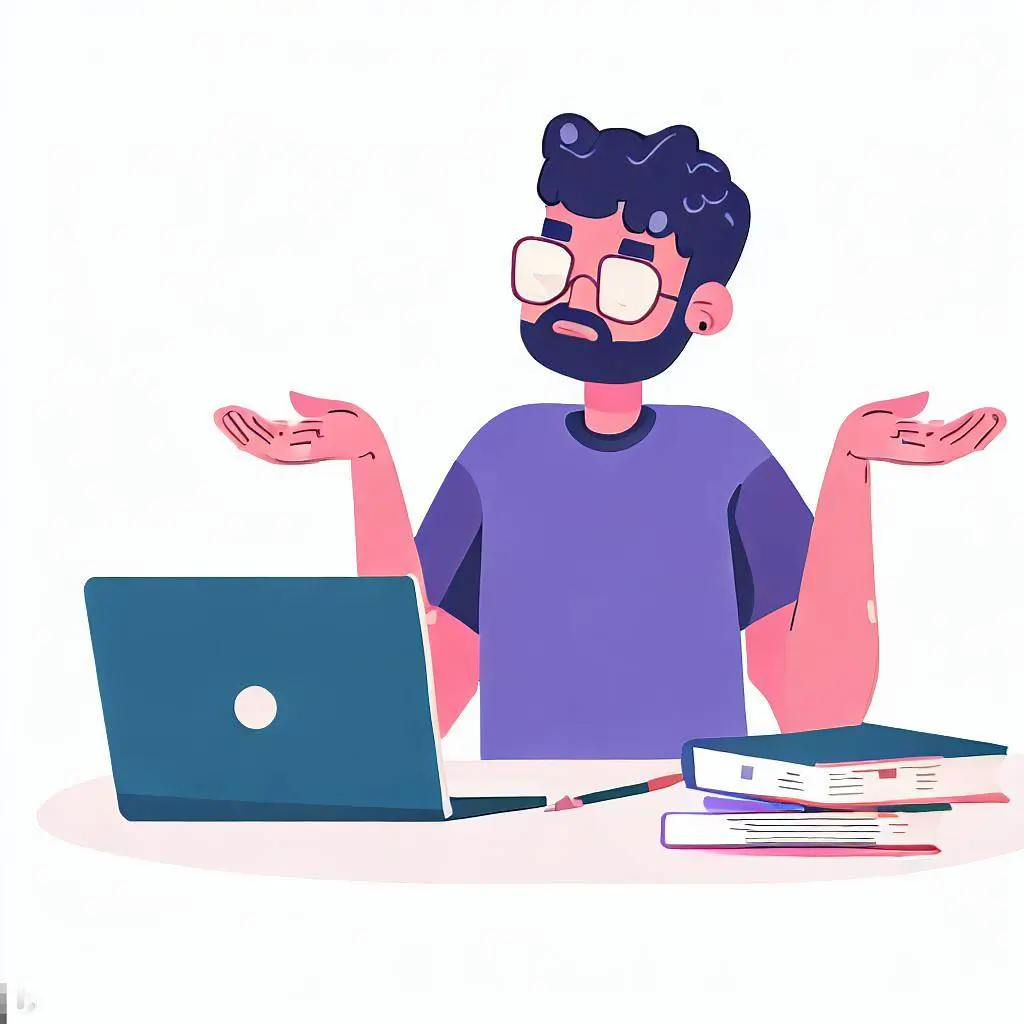
The Python Language's Basic Exceptions
It's crucial to understand the fundamentals of Python exceptions before delving into error handling strategies. An exception in Python is a circumstance that modifies the usual course of a program's execution. Python raises an exception when an error occurs, and if it is not handled correctly, the program ends with an error message that identifies the problem's root cause.
The base class BaseException, which comprises system-exiting exceptions like SystemExit, KeyboardInterrupt, and GeneratorExit, is the first level in Python's hierarchy of exceptions. There are other exception classes underneath this basic class that represent different types of errors, including Exception, TypeError, ValueError, and many more.
We will go through some of the most typical Python exception types that students could run across when working on projects in this section:
SyntaxError:
When the Python interpreter runs across code that doesn't follow the grammar conventions of the language, a SyntaxError is raised. This may be the result of omitted colons, parentheses that are mismatched, or erroneous indentation. When a SyntaxError occurs, thoroughly examine the offending code portion and fix any syntactical errors.
NameError:
When an undefined variable or function is utilized, a NameError occurs. Before using, make sure the variable or function is correctly specified by checking its spelling and definition twice.
TypeError:
When an operation or function is applied to an object of the wrong type, a TypeError occurs. Verify the compatibility of the data types used in the operation.
ValueError:
When a function gets an argument of the right type but with the wrong value, a ValueError occurs. Verify input data thoroughly and deal with edge situations to prevent ValueError exceptions from being raised.
KeyError and IndexError:
When trying to access items in sequences (lists, tuples, strings, etc.) or dictionaries with an erroneous index or key, respectively, IndexError and KeyError occur. Prior to accessing a sequence, always verify its boundaries and the presence of its keys.
Handling Exceptions
After exploring some typical sorts of Python exceptions, let's study some useful methods for managing these problems in Homeworks:
Exception Block using Try:
The essential building element for managing exceptions in Python is the try-except block. It's possible to get a better understanding of how to use your phone's camera to capture pictures. The following generic syntax is used:
try: # Code that may raise an exception except SomeException: # Code to handle the exception
Handling Multiple Exceptions: Multiple Exceptions may be handled by using numerous except blocks or by specifying various exception types inside a single except block. This enables you to modify your error-handling logic for various exception kinds.
try: # Code that may raise an exception except ValueError: # Code to handle ValueError except IndexError: # Code to handle IndexError except (TypeA, TypeB, TypeC): # Code to handle multiple exceptions
The else Clause: The else clause may be used with a try-except block to designate a code segment that only executes in the absence of an exception. It is helpful for running programs when there are no errors.
try: # Code that may raise an exception except SomeException: # Code to handle the exception else: # Code to execute if no exception occurs
The finally clause: The finally clause designates a block of code that always runs, whether or not an exception is triggered. This is often used to carry out cleaning procedures, such shutting files or releasing resources.
try: # Code that may raise an exception except SomeException: # Code to handle the exception finally: # Code that always executes
Techniques for Debugging Homework Exceptions:
You can run across challenging exceptions as students working on tasks that are difficult to diagnose. Here are several debugging strategies to effectively fix certain problems:
1. Debug Statements Printing:
Place print statements carefully to keep track of variable flow and values. This may assist you in determining the moment at which the exception is raised and in comprehending the program's current state.
print("Reached checkpoint A") # Code that may raise an exception print("Reached checkpoint B")
You may interactively debug your code with the pdb module. You may examine variables and the status of the program at various points during execution by establishing breakpoints and stepping through the code. import pdb def some_function(): pdb.set_trace() # Code to debug
2. Optimal Procedures for Efficient Error Handling
Let's review some recommended practices for efficient error handling in Python Homeworks to wrap up this guide:
Be Particular When Handling Exceptions:
Always be explicit when handling exceptions, capturing only those you can manage. Use caution when utilizing general exceptions like except Exception: since they may conceal faults or unforeseen problems.
Utilize Custom Exceptions When Necessary:
Making your own exceptions might be useful in certain circumstances, particularly if you wish to distinguish between distinct mistake conditions in your code.
Record Your Error Handling Strategy:
Document your strategy to addressing errors in projects or tasks that are complicated. This will make it easier for you and others to understand why certain decisions were made and will make future maintenance easier.
The ability to handle errors in a timely manner is a key component of the Python programming language, and it is something that must be done in order to succeed. You may confidently resolve Homework errors and make your code dependable and robust by grasping the principles of Python exceptions, implementing efficient error handling strategies, and following best practices. Gaining proficiency as a Python developer will surely result from embracing the learning process, using debugging tools, and adopting a proactive approach to problem resolution.
Advanced Techniques for Handling Errors:
Python includes a number of sophisticated methods that may improve your mistake management skills beyond the fundamentals of exception handling. Let's examine these methods in further detail:
1.Raising Exceptions:
On sometimes, you may wish to explicitly cause an exception depending on certain circumstances in your code. The raise statement may be used to do this. In order to propagate captured exceptions to higher levels of the application, you may do this or design your own custom exceptions.
def divide(x, y): if y == 0: raise ValueError("Division by zero is not allowed") return x / y
2.Chaining Exceptions:
The ability to link many occurrences of a given event with a single entity is known as "citizenship." When debugging, this might be helpful to comprehend the whole context of a mistake.
try: # Code that may raise an exception except SomeException as e: # Code to handle the exception raise NewException("Additional information", e) from e
3.Context Managers and the with Statement:
Context managers and the with statement provide a clear method for managing resources that must be appropriately obtained and released. Context managers may take care of cleaning tasks and exception handling automatically, strengthening your code.
with open("myfile.txt", "r") as file: # Code to work with the file # File automatically closed after leaving the 'with' block
Error Handling Techniques for Particular Situations:
Different programming contexts could call for different methods to error handling. Let's look at some tactics for certain situations:
The following is a list of all the ways in which we may help you.
Always be careful to gracefully handle file-related errors while interacting with files. When reading or writing files, use try-except blocks to handle probable problems like file not found or permission difficulties.
try: with open("myfile.txt", "r") as file: # Code to read from the file except FileNotFoundError: # Handle the case when the file is not found except IOError as e: # Handle any other input/output error
Conclusion:
In conclusion, mastering error handling in Python is an indispensable skill for any programmer aiming to create robust and reliable applications. Throughout this comprehensive guide on Python error handling, including understanding exceptions, utilizing the try-except block, and effectively using the else and finally clauses, you will find all the necessary knowledge to complete your Python homework successfully. By applying the concepts and techniques presented here, you'll be able to handle errors and exceptions in your code with confidence, ensuring your programs run smoothly and handle unexpected situations gracefully. So go ahead and put your newfound skills to the test, and complete your Python homework with ease and excellence. Happy coding!
By employing proper error handling techniques, Programming homework help developers can ensure their programs handle unexpected situations gracefully, provide informative feedback to users, and maintain smooth execution under adverse conditions. Moreover, proper error handling enhances the overall user experience by reducing application crashes and unhandled exceptions.
We hope this guide has provided valuable insights and practical examples that will empower you to tackle Homework exceptions confidently. Remember, proficiency in error handling comes with practice and experience, so keep coding and exploring. By continuously honing your skills, you can become a proficient Python developer, capable of crafting resilient and efficient solutions for any coding challenge that comes your way.
Now, go forth and apply your newfound knowledge to build remarkable Python applications with robust error handling! Happy coding!