Tip of the day
News
Instructions
Objective
Write a C++ assignment program to implement riders of fortune game.
Requirements and Specifications
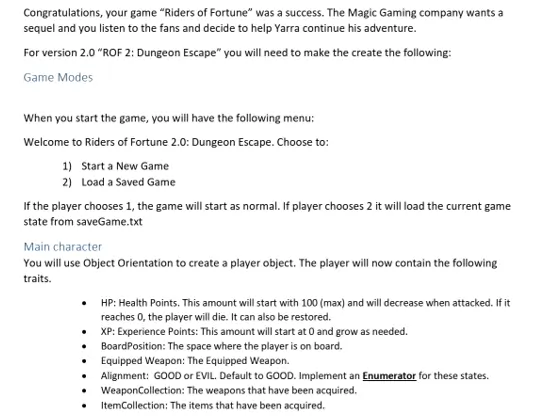
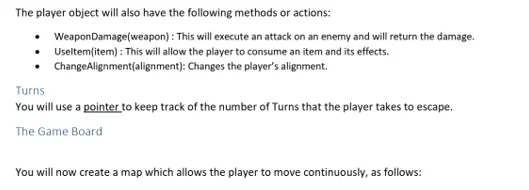
Source Code
#ifndef board_h
#define board_h
#include
#include
#include
#include "Location.h"
using namespace std;
class Board
{
public:
// Create the board
Board()
{
for (int row = 0; row < 8; row++)
for (int col = 0; col < 8; col++)
if (col == 0 || col == 7 || row == 0 || row == 7)
_grid[row][col] = '*';
else
_grid[row][col] = ' ';
// Put the graveyards
put('G', Location(getHeight() / 2 - 1, getWidth() - 1));
put('G', Location(getHeight() - 1, 0));
// Put the cathedrals
put('C', Location(0, getWidth() - 1));
put('C', Location(getHeight() - 1, getWidth() - 1));
// Put the wells
put('W', Location(0, getWidth() / 2));
put('W', Location(getHeight() - 1, getWidth() / 2));
// Put the exit
put('E', Location(getHeight() / 2 - 1, 0));
}
// Return what's in the location
char explore(const Location &location)
{
if (location.getRow() < 0 || location.getRow() >= 8
|| location.getColumn() < 0 || location.getColumn() >= 8)
return '\0';
return _grid[location.getRow()][location.getColumn()];
}
// Getter methods
int getWidth() const
{
return 8;
}
int getHeight() const
{
return 8;
}
// Put an object into the grid
void put(char c, const Location &loc)
{
if (loc.getRow() < 0 || loc.getRow() >= 8
|| loc.getColumn() < 0 || loc.getColumn() >= 8)
return;
_grid[loc.getRow()][loc.getColumn()] = c;
}
// Return a string representation of a board
string toString() const
{
stringstream ss;
for (int r = 0; r < 8; r++)
{
for (int c = 0; c < 8; c++)
ss << _grid[r][c] << " ";
ss << endl;
}
return ss.str();
}
private:
char _grid[8][8];
};
#endif
CHARACTER
#ifndef character_h
#define character_h
#include
#include
#include
#include
#include "Location.h"
#include "Weapon.h"
using namespace std;
enum Alignment
{
GOOD,
EVIL
};
class Character
{
public:
// Construct the character
Character()
: _hp(100), _xp(0), _bagCapacity(5), _bagSize(0), _turns(0)
{
_bag = new string[_bagCapacity];
}
// Clear used pointers
~Character()
{
delete[] _bag;
}
// Setter methods
void setTurns(int turns)
{
_turns = turns;
}
void setHP(int hp)
{
if (hp > 100)
hp = 100;
else if (hp < 0)
hp = 0;
_hp = hp;
}
void setAlignment(Alignment alignment)
{
_alignment = alignment;
}
void setXP(int xp)
{
_xp = xp;
}
void setLocation(const Location &location)
{
_location = location;
}
// Getter methods
int getTurns() const
{
return _turns;
}
int getHP() const
{
return _hp;
}
int getXP() const
{
return _xp;
}
vector getWeapons() const
{
return _weapons;
}
Location getLocation() const
{
return _location;
}
Alignment getAlignment() const
{
return _alignment;
}
// Return items in bag
vector getBag() const
{
vector theItems;
for (int i = 0; i < _bagSize; i++)
theItems.push_back(_bag[i]);
return theItems;
}
// Put item to pag
void addToBag(const string &item)
{
// Extend the bag's capacity if out of sace
if (_bagSize >= _bagCapacity)
{
int capacity = _bagCapacity + 5;
string *bag = new string[capacity];
for (int i = 0; i < _bagSize; i++)
bag[i] = _bag[i];
delete[] _bag;
_bag = bag;
_bagCapacity = capacity;
}
_bag[_bagSize++] = item;
}
// Put in a weapon
void addWeapon(const Weapon &weapon)
{
_weapons.push_back(weapon);
}
// Atempt to apply item to character
string applyItem(const string &item)
{
int position = -1;
for (int i = 0; i < _bagSize; i++)
{
if (_bag[i] == item)
{
position = i;
break;
}
}
stringstream ss;
if (position == -1)
{
ss << item << " is not in your bag.";
}
else if (item == "Potion 1")
{
_hp += 5;
ss << "Used " << item << " 5 HP recovered.";
}
else if (item == "Potion 2")
{
_hp += 10;
ss << "Used " << item << " 10 HP recovered.";
}
return ss.str();
}
// Remove an item from bag
void deleteItem(const string &item)
{
int position = -1;
for (int i = 0; i < _bagSize; i++)
{
if (_bag[i] == item)
{
position = i;
break;
}
}
if (position == -1)
return;
for (int i = position; i < _bagSize - 1; i++)
_bag[i] = _bag[i + 1];
_bagSize--;
}
// Find if character has item
bool hasItem(const string &item) const
{
for (int i = 0; i < _bagSize; i++)
if (_bag[i] == item)
return true;
return false;
}
// Find the weapon in the player's inventory
bool hasWeapon(const Weapon &weapon) const
{
for (unsigned i = 0; i < _weapons.size(); i++)
if (_weapons[i] == weapon)
return true;
return false;
}
// Return a string representation
string toString() const
{
stringstream ss;
ss << "HP : " << _hp << endl;
ss << "XP : " << _xp << endl;
ss << "Turns : " << _turns << endl;
ss << "Weapons: " << "Stick";
for (int i = 0; i < (int)_weapons.size(); i++)
ss << ", " << _weapons[i].name;
ss << endl;
ss << "Bag : ";
for (int i = 0; i < _bagSize; i++)
{
ss << _bag[i];
if (i + 1 < _bagSize)
ss << ", ";
}
return ss.str();
}
private:
int _hp;
int _xp;
int _bagCapacity;
int _bagSize;
int _turns;
Location _location;
Alignment _alignment;
vector _weapons;
string *_bag;
};
#endif
ENEMY
#ifndef enemy_h
#define enemy_h
#include
#include
#include
#include "Weapon.h"
using namespace std;
class Enemy
{
public:
// Create the enemy
Enemy()
{
_xp = (rand() % 10) + 1;
_hp = (rand() % (_xp * 5)) + 1;
switch (rand() % 5)
{
case 0:
_weapon = Weapon("Crossbow", 3);
break;
case 1:
_weapon = Weapon("Flail", 5);
break;
case 2:
_weapon = Weapon("Broad Sword", 8);
break;
case 3:
_weapon = Weapon("Morning Star", 13);
break;
case 4:
_weapon = Weapon("Holy Staff", 21);
break;
}
}
// Setter methods
void setHP(int hp)
{
_hp = hp;
}
// Getter methods
int getHP() const
{
return _hp;
}
int getXP() const
{
return _xp;
}
Weapon getWeapon() const
{
return _weapon;
}
// Return a string representation
string toString() const
{
stringstream ss;
ss << "HP: " << _hp;
return ss.str();
}
private:
int _hp;
int _xp;
Weapon _weapon;
};
#endif
LOCATION
#ifndef location_h
#define location_h
class Location
{
public:
// Create a location
Location()
: _row(0), _col(0)
{
}
// Create a location
Location(int row, int col)
: _row(row), _col(col)
{
}
// Setter methods
void setColumn(int col)
{
_col = col;
}
void setRow(int row)
{
_row = row;
}
// Getter methods
int getColumn() const
{
return _col;
}
int getRow() const
{
return _row;
}
Location getLeft() const
{
return Location(_row, _col - 1);
}
Location getRight() const
{
return Location(_row, _col + 1);
}
Location getUp() const
{
return Location(_row - 1, _col);
}
Location getDown() const
{
return Location(_row + 1, _col);
}
// Compare if locations are the same
bool operator == (const Location &location) const
{
return _row == location._row
&& _col == location._col;
}
private:
int _row;
int _col;
};
#endif
Similar Sample
Explore our C++ assignment samples to witness firsthand the quality and expertise we bring to programming education. Our curated examples cover diverse topics, showcasing our commitment to clarity, precision, and practical application in C++ programming. Whether you're a student or professional, these samples illustrate our proficiency in delivering top-notch solutions tailored to your learning needs.
C++
Word Count
6780 Words
Writer Name:Alexander Gough
Total Orders:2435
Satisfaction rate:
C++
Word Count
6185 Words
Writer Name:Neven Bell
Total Orders:2376
Satisfaction rate:
C++
Word Count
5749 Words
Writer Name:Professor Kevin Tan
Total Orders:569
Satisfaction rate:
C++
Word Count
4786 Words
Writer Name:Dr. Isabelle Taylor
Total Orders:580
Satisfaction rate:
C++
Word Count
3997 Words
Writer Name:Professor Oliver Bennett
Total Orders:304
Satisfaction rate:
C++
Word Count
6830 Words
Writer Name:Dr. Maddison Todd
Total Orders:865
Satisfaction rate:
C++
Word Count
4424 Words
Writer Name:Dr. Alexandra Mitchell
Total Orders:589
Satisfaction rate:
C++
Word Count
7250 Words
Writer Name:Mr. Harrison Yu
Total Orders:311
Satisfaction rate:
C++
Word Count
4894 Words
Writer Name:James Miller
Total Orders:824
Satisfaction rate:
C++
Word Count
5544 Words
Writer Name:Callum Chambers
Total Orders:932
Satisfaction rate:
C++
Word Count
3533 Words
Writer Name:Aaliyah Armstrong
Total Orders:658
Satisfaction rate:
C++
Word Count
3645 Words
Writer Name:Len E. Villasenor
Total Orders:489
Satisfaction rate:
C++
Word Count
4033 Words
Writer Name:Daniel M. Harrison
Total Orders:700
Satisfaction rate:
C++
Word Count
3576 Words
Writer Name:Dr. Isabella Wong
Total Orders:652
Satisfaction rate:
C++
Word Count
3600 Words
Writer Name:Prof. Liam Wilson
Total Orders:554
Satisfaction rate:
C++
Word Count
1035 Words
Writer Name:Professor Marcus Tan
Total Orders:544
Satisfaction rate:
C++
Word Count
6288 Words
Writer Name:Dr. Stephanie Kim
Total Orders:812
Satisfaction rate:
C++
Word Count
1586 Words
Writer Name:Ethan Patel
Total Orders:556
Satisfaction rate:
C++
Word Count
3852 Words
Writer Name:Sarah Rodriguez
Total Orders:689
Satisfaction rate:
C++
Word Count
4701 Words
Writer Name:Harry F. Grimmett
Total Orders:342
Satisfaction rate: