Claim Your Offer
Unlock an amazing offer at www.programminghomeworkhelp.com with our latest promotion. Get an incredible 10% off on your all programming assignment, ensuring top-quality assistance at an affordable price. Our team of expert programmers is here to help you, making your academic journey smoother and more cost-effective. Don't miss this chance to improve your skills and save on your studies. Take advantage of our offer now and secure exceptional help for your programming assignments.
We Accept
Explore Sample Array Class Interface Assignments Solved by Our Experts
In the sample section, you can browse through examples of Array class interface assignments completed by our experts. These samples provide a glimpse into the quality and depth of our work, showcasing our expertise in tackling various programming challenges.
C++
C++
C++
Java
C++
Java
Java
C++
Java
Java
Java
Java
Java
Java
Java
Java
Java
C++
Java
Java
Affordable Array Class Interface Assignment Help Is Just One Click Away
Ensuring affordability is paramount in our Array class interface assignment help online service. We customize our rates to cater to the diverse needs and budgets of students, ensuring accessibility without compromising on quality. Our pricing structure is transparent and flexible, allowing students to choose the pricing plan that best suits their requirements and financial constraints. To provide a clear overview of our prices, we offer a detailed table showcasing sample price ranges based on the complexity and urgency of assignments. This approach empowers students to make informed decisions while availing our services, ensuring that they receive high-quality assistance at competitive rates.
Complexity Level | Urgency | Price Range |
---|---|---|
Basic | Low | $20 - $30 |
Intermediate | Medium | $30 - $50 |
Advanced | High | $50 - $100 |
Expert | Urgent | $100 - $150 |
Customized | Custom | Customized |
- A One-Stop Solution for All Your Array Class Interface Assignment Writing Needs
- Subject-Areas Covered by Our Array Class Interface Assignment Writing Services
- Why Should I Pay Your Experts to Do My Array Class Interface Homework?
- How Our Seasoned Array Class Interface Assignment Helpers Approach Your Homework?
A One-Stop Solution for All Your Array Class Interface Assignment Writing Needs
An Array class interface represents a blueprint for creating and manipulating arrays in programming languages such as Java or C++. It typically includes key components such as methods for accessing elements, inserting and deleting elements, resizing the array, and searching for specific values. Additionally, it may encompass functionalities like sorting, iterating through elements, and performing various operations on array elements. The interface serves as a contract defining how arrays can be interacted with, providing a structured way for programmers to work with array data structures in their code.
Students often struggle to write assignments on the Array class interface due to several reasons. Firstly, mastering array manipulation requires a solid understanding of fundamental programming concepts such as loops, conditionals, and data structures. Additionally, grasping the nuances of array indexing, memory management, and algorithmic complexities can be challenging. Moreover, students may face difficulties in implementing various array operations efficiently while adhering to the specific requirements of their assignments.
Thousands of college Students opt for our help with java homework because we offer comprehensive assistance in understanding and mastering the Array class interface. With our expertise, we simplify complex concepts, provide step-by-step guidance, and offer personalized support tailored to individual learning needs. Whether struggling with basic array operations or seeking advanced techniques, our service ensures students receive the clarity and guidance necessary to excel in their programming assignments.
Subject-Areas Covered by Our Array Class Interface Assignment Writing Services
At Programminghomeworkhelp.com, we take pride in our proficiency in tackling a wide range of Array class interface assignment topics. Our team of experienced programmers and educators excels in crafting high-quality homework solutions tailored to meet your specific needs. Below are eight key topics in which we excel, ensuring that you receive top-notch assistance every step of the way.
- Array Initialization and Declaration: Crafting homework on array initialization and declaration involves understanding the syntax and semantics of defining arrays in programming languages. Our array class interface assignment writing service ensures that students grasp the intricacies of declaring arrays with precision and clarity, enabling them to implement this fundamental concept effectively in their assignments.
- Array Operations (Insertion, Deletion, Searching): Our expertise extends to guiding students through the implementation of essential array operations such as insertion, deletion, and searching. With our array class interface assignment help online, students gain a deep understanding of the algorithms and techniques required to perform these operations efficiently, empowering them to tackle complex array manipulation tasks with confidence in their homework.
- Array Sorting Algorithms: When it comes to array sorting algorithms, our programming help service shines. We offer comprehensive assistance in understanding and implementing popular sorting algorithms like bubble sort, insertion sort, merge sort, and quicksort. With our guidance, students learn not only how to apply these algorithms but also how to analyze their efficiency and choose the most suitable approach for their homework assignments.
- Multi-dimensional Arrays: Crafting homework on multi-dimensional arrays requires a solid understanding of array indexing and memory layout. Our array class interface assignment writing service provides students with clear explanations and practical examples to master the complexities of working with multi-dimensional arrays. From basic array traversal to advanced matrix operations, we ensure students have the necessary skills to excel in their assignments.
- Dynamic Arrays and Resizing: Dynamic arrays and resizing present unique challenges in array manipulation. With our array class interface assignment help online, students learn how to implement dynamic arrays using concepts such as dynamic memory allocation and reallocation. We guide students through the process of resizing arrays dynamically to accommodate changing data requirements, empowering them to handle dynamic array scenarios effectively in their homework.
- Array Searching Techniques: Our programming help service specializes in teaching students various array searching techniques, including linear search, binary search, and hashing. Through comprehensive explanations and hands-on examples, students gain a deep understanding of how these techniques work and when to apply them in their array class interface assignments. We ensure that students are equipped with the knowledge and skills to tackle searching-related tasks with confidence.
- Array Iteration and Traversal: Mastering array iteration and traversal is essential for effectively processing array elements in programming assignments. Our array class interface assignment writing service equips students with the necessary skills to iterate through arrays using loops and iterators. We teach students how to access and manipulate array elements efficiently, enabling them to implement iterative algorithms and solve array traversal problems effectively in their homework.
- Error Handling and Exception Management: Error handling and exception management play a crucial role in writing robust and reliable code involving arrays. With our programming homework help, students learn best practices for handling errors and exceptions that may arise during array manipulation. We provide guidance on implementing error-checking mechanisms and exception handling strategies, ensuring that students deliver error-free and resilient solutions in their array class interface assignments.
Why Should I Pay Your Experts to Do My Array Class Interface Homework?
Programminghomeworkhelp.com offers a multitude of benefits that ensure the success and satisfaction of students wondering, “Why should I pay someone to do my programming homework?” Whether you're struggling with complex algorithms or seeking clarity on fundamental concepts, our team is dedicated to providing unparalleled assistance tailored to your needs. Below are the key benefits of availing our help with Array class interface homework:
- Expert Guidance from Programming Professionals: Our team comprises seasoned programmers and educators with extensive experience in the field of programming. When you entrust us with your Array class interface homework, you gain access to expert guidance and support every step of the way. Our professionals offer personalized assistance, helping you navigate through challenging concepts and ensuring a thorough understanding of array manipulation techniques.
- Customized Solutions for Your Unique Needs: We understand that every student's learning journey is unique, which is why we prioritize offering customized solutions for your Array class interface homework. Whether you need help with specific assignments or require comprehensive tutoring sessions, our services are tailored to meet your individual requirements. With our personalized approach, you can rest assured that your homework needs will be addressed effectively and efficiently.
- Timely Delivery to Meet Deadlines: Deadlines are a crucial aspect of academic life, and we recognize the importance of timely submission when it comes to Array class interface homework. Our team is committed to delivering high-quality solutions within the stipulated time frame, ensuring that you never have to worry about missing deadlines again. With our prompt delivery service, you can focus on other academic priorities while we take care of your programming assignments with precision and efficiency.
- Enhanced Understanding and Concept Clarity: Beyond simply completing your Array class interface homework, our goal is to foster a deeper understanding of programming concepts and techniques. Through detailed explanations, examples, and interactive sessions, we strive to enhance your understanding and clarity on array manipulation, algorithms, and data structures. Our approach empowers you to tackle similar programming challenges with confidence in the future.
- Round-the-Clock Support for Your Convenience: We understand that programming assignments can sometimes pose challenges outside traditional office hours. That's why our support team is available 24/7 to address any queries or concerns you may have regarding your Array class interface homework. Whether you need clarification on a concept or assistance with technical issues, our dedicated support staff is just a message away, ensuring that you receive the assistance you need whenever you need it.
Choosing our services for your Array class interface homework guarantees not only top-notch solutions but also a rewarding learning experience that equips you with the skills and confidence to excel in your programming endeavors. Let us handle your homework while you focus on unleashing your full potential in the world of programming.
How Our Seasoned Array Class Interface Assignment Helpers Approach Your Homework?
Our team of dedicated experts at programminghomeworkhelp.com follows a systematic approach to ensure precision, accuracy, and efficiency. Leveraging their extensive knowledge and experience, our programming assignment helpers employ proven strategies and methodologies to deliver high-quality solutions tailored to your specific requirements:
- Understanding Requirements: Our programming experts begin by thoroughly understanding the requirements and specifications of your Array class interface assignment. They analyze the assignment prompt, identify key objectives, and clarify any doubts or ambiguities to ensure a clear understanding of the task at hand.
- Research and Planning: Once the requirements are understood, our experts conduct comprehensive research to gather relevant resources, documentation, and examples related to the Array class interface. They formulate a detailed plan outlining the steps and approach to be followed in completing the assignment, ensuring a structured and organized workflow.
- Implementation and Coding: With a solid plan in place, our programming experts proceed to implement the necessary algorithms, data structures, and code logic required for the Array class interface assignment. They leverage their expertise in programming languages such as Java, C++, Python, etc., to write clean, efficient, and well-documented code that adheres to best practices and industry standards.
- Testing and Debugging: Rigorous testing and debugging are integral parts of our process to ensure the correctness and functionality of the Array class interface assignment solutions. Our experts meticulously test the code against various test cases, identify and rectify any errors or bugs, and fine-tune the implementation to achieve optimal performance and reliability.
- Documentation and Explanation: In addition to providing the code solution, our Array class interface assignment helpers prioritize documenting the implementation process and providing detailed explanations for key components and functionalities. They ensure that the solution is accompanied by clear and concise documentation, including comments, annotations, and explanations, to facilitate easy understanding and future reference.
- Quality Assurance and Review: Before delivering the final solution, our experts conduct a thorough quality assurance review to validate the correctness, completeness, and adherence to requirements of the Array class interface assignment.
Stay Updated with Our Informative Blogs on Array Class Interface
In our blog section, we provide valuable insights, tips, and tutorials related to Array class interface and programming in general. Our blog posts cover a wide range of topics, from basic concepts to advanced techniques, catering to students at all skill levels. Whether you're looking for troubleshooting advice or want to stay updated on the latest trends in programming, our blog is your go-to resource.
Discover What Our Clients Say About Our Online Array Class Interface Assignment Help
In the review section, you can explore testimonials from our satisfied clients who have benefited from our Array class interface assignment help online. Our clients share their experiences and feedback, highlighting the quality of our services and the expertise of our team. From timely deliveries to personalized assistance, our reviews showcase the positive impact our services have had on students' academic journeys.
Hire from Our Pool of Highly Qualified Array Class Interface Assignment Experts
Our team of Array class interface assignment helpers comprises seasoned programmers and educators who bring years of experience and expertise to the table. With a deep understanding of programming concepts and a passion for teaching, our experts are dedicated to providing top-notch assistance tailored to your learning needs. From guiding you through complex algorithms to offering personalized tutoring sessions, our team is committed to helping you excel in your Array class interface assignments.
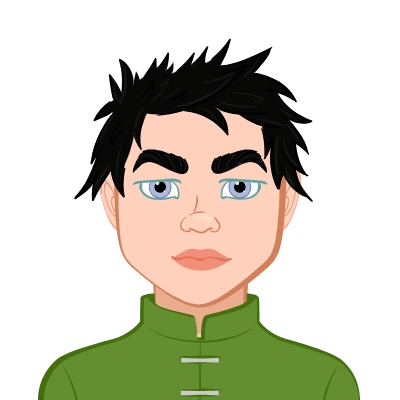
John Anderson
Ph.D. in Computer Science
🇬🇧 United Kingdom
I am John Anderson, a skilled Java programmer with 8+ years of experience in software development and academic assistance, frameworks, and academic assistance. With over 8 years of expertise, he specializes in crafting tailored solutions for students, ensuring top-notch quality, timely delivery, and enhanced understanding of Java concepts. John is committed to students' success and excellence.
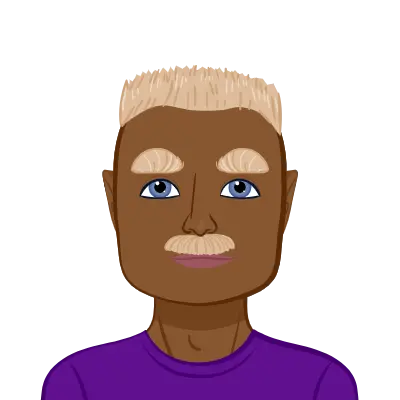
Patrick Railey
Ph.D. Computer Science
🇬🇧 United Kingdom
Patrick Railey is an experienced Java programming expert with over 7 years in academic assistance and software development. He specializes in Java assignments, multithreading, and frameworks like Spring and Hibernate. With a focus on quality, deadlines, and personalized support, John helps students excel in Java programming projects and coursework.
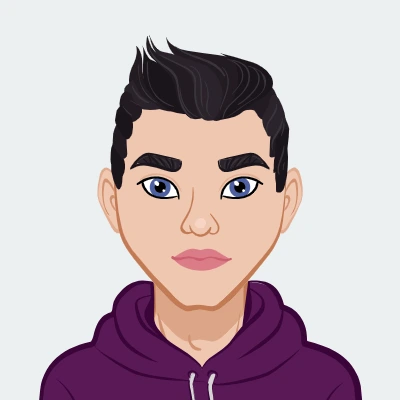
Dennis Posner
PhD in Programming
🇳🇿 New Zealand
Dennis Posner, a seasoned Programming Assignment Expert, specializes in Java, Python, and C++. With 7 years of experience, Dennis provides affordable, plagiarism-free solutions tailored to students' needs. Known for meeting tight deadlines and simplifying complex coding challenges, Dennis ensures academic success for programming enthusiasts worldwide.
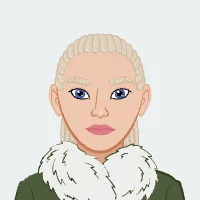
Mark Grimmer
PhD in Programming
🇺🇸 United States
Mark Grimmer, a software engineer with 10+ years in C++ development, specializes in data structures and algorithm design.
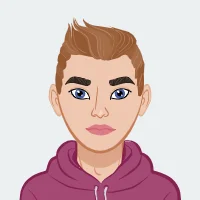
Elena Guillen
PhD in Programming
🇺🇸 United States
Elena Guillen is a software engineer with over a decade of experience in data structures and algorithms. Specializing in optimizing hash table implementations, Elena brings extensive expertise in improving data management and performance for complex challenges.
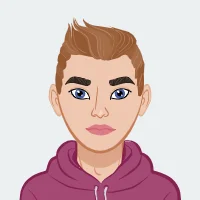
Daniel Clark
PhD in Programming
🇺🇸 United States
Daniel Clark is a software developer with over a decade of experience in JavaFX and data structures. He specializes in creating interactive visualizations and user-friendly applications
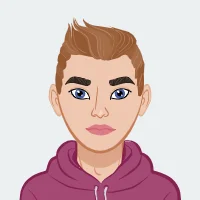
James Nickerson
PhD in Programming
🇺🇸 United States
James Nickerson, a software engineer with extensive experience in system design and development, offers in-depth insights into building modular, scalable applications. His expertise in Java and UML ensures robust solutions for complex software challenges.
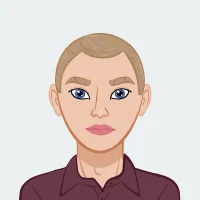
George Paden
Masters in Computer Science
🇺🇸 United States
George Paden holds a master's degree in computer science from the University of California, Irvine. With extensive experience in software development and data structures, he specializes in advanced programming techniques and dynamic memory management.
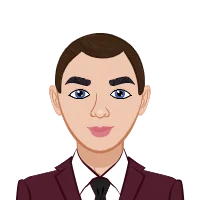
Alexander Gough
PhD in Programming
🇺🇸 United States
Alexander Gough is a seasoned C++ programmer with over a decade of experience in developing advanced algorithms and data structures. Specializing in recursive problem-solving and binary search trees, Alexander excels in providing tailored solutions for complex programming assignments. His expertise ensures clear, efficient code and insightful guidance, making him a top choice for C++ assignment help.
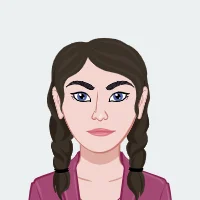
Ann Leff
PhD in Programming
🇺🇸 United States
Ann Leff is an experienced software engineer with a strong background in algorithm design and programming languages. With over a decade of expertise in developing efficient solutions, she specializes in expression parsing, data structures, and optimizing computational processes for various applications.
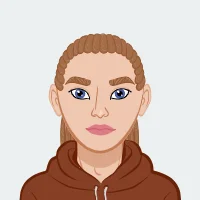
Howard Zuniga
PhD in Programming
🇺🇸 United States
Howard Zuniga is a seasoned Java Assignment Expert with over a decade of experience in Java programming and teaching. Holding advanced degrees and certifications, Howard excels in guiding students through complex assignments, optimizing code, and integrating Java with databases. Dedicated to clear, personalized instruction, Howard ensures student success in Java.
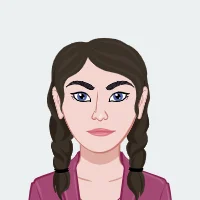
Neven Bell
Masters in Programming
🇺🇸 United States
Neven Bell is an experienced C++ developer with a strong background in data structures and algorithm design. He specializes in text processing, efficient data storage, and software optimization. With over 10 years of industry experience, Bell has a proven track record of solving complex programming challenges and helping students excel in their C++ assignments.
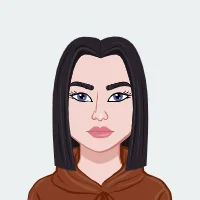
Debra Cortez
Masters in Programming
🇺🇸 United States
Debra Cortez is a seasoned software developer with over eight years of experience in C++ programming. Passionate about combining theoretical knowledge with practical applications, she enjoys creating engaging simulations and enhancing coding skills through innovative projects in computer science.
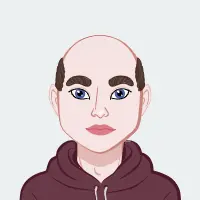
Matthew Newcomb
Masters in Computer Science
🇺🇸 United States
Matthew Newcomb is a seasoned C++ Assignment Expert with a master's degree in computer science from Dokata University. With extensive experience in modern C++, object-oriented programming, and data structures, Alex excels in providing tailored support for assignments and tutoring students to master C++ concepts effectively.
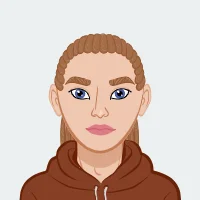
Martin Hyatt
Ph.D. in Programming
🇺🇸 United States
Martin Hyatt, a software engineer with 10 years of experience, specializes in algorithm design and data analysis, with extensive experience in optimizing complex systems.
Dr. Hayden Buckley
Ph.D. in Computer Science
🇨🇦 Canada
Dr. Hayden Buckley, with a Ph.D. from Duke University, has 8 years of experience in the field of Computer Science. Having completed over 700 Active Template Library assignments, Dr. Buckley is renowned for his expertise and dedication. His extensive experience and exceptional problem-solving skills make him an invaluable resource for students seeking high-quality ATL assignment help. Trust Dr. Buckley to provide comprehensive support and deliver outstanding results.
Dr. Morgan Griffin
Ph.D. in Computer Science
🇺🇸 United States
Dr. Morgan Griffin, a Ph.D. graduate from Technische Universität Wien, brings 7 years of experience to our Active Template Library Assignment Help service. Having completed over 600 ATL assignments, Dr. Griffin combines advanced technical skills with a deep understanding of assignment complexities. Students can rely on Dr. Griffin for thorough and insightful assistance, aimed at achieving superior academic results.
Dr. Evan Cartwright
Ph.D. in Computer Science
🇦🇺 Australia
Dr. Evan Cartwright earned his Ph.D. in Computer Science from The University of Warwick. With 6 years of professional experience, he has completed more than 400 Active Template Library assignments. Dr. Cartwright’s extensive knowledge and hands-on approach provide students with exceptional guidance and innovative solutions. His dedication to academic excellence ensures that every assignment is handled with the utmost care and expertise.
Dr. Hollie Bennett
Ph.D. in Computer Science
🇺🇸 United States
Dr. Hollie Bennett holds a Ph.D. in Computer Science from City University of Hong Kong. With 5 years of experience in the field, Dr. Bennett has completed over 300 Active Template Library assignments. Her deep expertise in ATL and commitment to delivering high-quality solutions make her a valuable asset to our team. Students benefit from her precise and reliable assistance, ensuring top-notch support for all their ATL assignment needs.
Owen Phillips
Ph.D. in Computer Science
🇺🇸 United States
Owen Phillips, a distinguished Java Swing assignment expert, earned his Ph.D. from Princeton University, United States, and possesses over 10 years of invaluable experience. His proficiency in Java Swing includes advanced GUI design and innovative custom widget development.
Related Topics
Frequently Asked Questions
In the FAQs section, we address common queries and concerns related to our Array class interface assignment help service. From inquiries about pricing and payment options to questions about our team and process, we cover all the essential information you need to know. If you can't find the answer you're looking for, our 24/7 customer support team is available via live chat to assist you.
We offer a free consultation service where you can discuss your specific requirements and concerns with our experts. During the consultation, we'll assess your needs and provide personalized recommendations on how our services can best assist you with your Array class interface homework. Additionally, our 24/7 customer support team is available via live chat to address any further inquiries you may have.
Yes, error handling and exception management are crucial aspects of writing robust code involving arrays. Our experts are proficient in explaining error-checking mechanisms, exception handling strategies, and best practices specifically tailored to Array class interface assignments. We ensure that you understand how to effectively handle errors and exceptions to deliver resilient solutions.
Dynamic arrays and resizing operations can be challenging, but our experts are here to help. We offer personalized assistance in understanding dynamic memory allocation, reallocation, and resizing techniques within the context of Array class interface assignments. With our guidance, you'll gain the confidence to tackle dynamic array scenarios with ease.
Absolutely! Our team specializes in explaining and implementing sorting algorithms such as bubble sort, insertion sort, merge sort, and quicksort specifically tailored to Array class interface assignments. We provide step-by-step guidance and code examples to help you grasp the concepts and apply them effectively in your assignments.
We offer comprehensive assistance with various topics related to the Array class interface, including array initialization, insertion, deletion, searching, sorting algorithms, multi-dimensional arrays, dynamic arrays, and error handling. Our experts are proficient in addressing any specific challenges or queries you may have related to array manipulation and programming tasks.