Claim Your Discount Today
Kick off the fall semester with a 20% discount on all programming assignments at www.programminghomeworkhelp.com! Our experts are here to support your coding journey with top-quality assistance. Seize this seasonal offer to enhance your programming skills and achieve academic success. Act now and save!
We Accept
See Our Free Samples of Threads and Synchronization Assignments
See our free samples of threads and synchronization assignments to get a glimpse of our expertise. Our threads and synchronization assignment experts provide valuable insights and solutions. Explore how our programming homework help can assist you with help with threads and synchronization assignments at ProgrammingHomeworkHelp.com.
Operating System
Operating System
Operating System
Operating System
Operating System
Operating System
Operating System
Operating System
Operating System
Operating System
Operating System
Operating System
Operating System
Operating System
Personalized Threads and Synchronization Assignment Help at Budget-Friendly Prices
Experience personalized threads and synchronization assignment help tailored to your needs at budget-friendly prices. Our threads and synchronization assignment experts deliver high-quality solutions for help with threads and synchronization assignments. Enjoy the benefits of our programming homework help service, including affordability and expert guidance. At ProgrammingHomeworkHelp.com, we ensure your assignments are handled by professionals who prioritize your academic success. Contact us today to get started on improving your understanding and grades in threads and synchronization topics.
Service | Price Range |
---|---|
Basic Threads & Synchronization Help | $15 - $30 |
Intermediate Threads Support | $35 - $60 |
Advanced Synchronization Assistance | $65 - $100 |
Complex Threads Assignment Solutions | $120 - $200+ |
Expedited Service (All Levels) | Additional 50% |
Consultation & Tips | $20 - $50 per hour |
- What are Threads and synchronization?
- Managing Concurrent Tasks with Synchronized Threads
- Threads and Synchronization Assignment Help
- Understanding the Demand for Online Threads and Synchronization Assignment Help Among Students
- The Benefits of Using Our Threads and Synchronization Assignment Help Service
- Dive into the Diverse Topics Offered in Our Threads and Synchronization Assignment Help Service
- Simple Steps to Obtain Expert Threads and Synchronization Assignment Help from Us
- Claim Your Special 20% Discount on Your Next Assignment with Us
What are Threads and synchronization?
Threads and synchronization are fundamental concepts in concurrent programming, essential for managing multiple tasks within a program. Threads and synchronization assignment help focuses on understanding how threads, lightweight processes within a program, interact and share resources. Expert guidance in help with threads and synchronization assignments ensures mastery of techniques like mutexes, semaphores, and synchronization primitives to prevent data conflicts and ensure orderly execution.
If you are concerned about who will do my threads and synchronization assignment? Our threads and synchronization assignment experts at ProgrammingHomeworkHelp.com provide comprehensive solutions that cover thread creation, synchronization mechanisms, deadlock prevention, and concurrent data structures.
Concurrency issues can be complex, requiring tailored programming homework help solutions to meet academic requirements and deadlines. By consulting with our threads and synchronization assignment expert team, students gain clarity and proficiency in handling concurrent operations effectively. Enhance your understanding and application of threads and synchronization with our specialized assistance, designed to elevate your programming skills and academic performance.
Managing Concurrent Tasks with Synchronized Threads
public class ThreadExample {
private static int counter = 0;
public static void main(String[] args) {
Runnable task = () -> {
for (int i = 0; i < 5; i++) {
incrementCounter();
System.out.println(Thread.currentThread().getName() + ": Counter = " + counter);
}
};
Thread thread1 = new Thread(task);
Thread thread2 = new Thread(task);
thread1.start();
thread2.start();
}
private static synchronized void incrementCounter() {
counter++;
}
}
Explanation:
- The ThreadExample class defines a static integer counter shared by two threads.
- The main method creates two threads (thread1 and thread2) that execute the task concurrently.
- The task is a lambda expression that increments the counter five times and prints its value, demonstrating concurrent access.
- The incrementCounter method is synchronized, ensuring that only one thread can execute it at a time, preventing simultaneous access and potential data corruption.
In this example, synchronization ensures that increments to the counter variable are atomic and prevents race conditions, demonstrating a basic use of threads and synchronization in a concurrent programming context.
Threads and Synchronization Assignment Help
Looking for threads and synchronization assignment help? Our team at ProgrammingHomeworkHelp.com offers expert guidance to ensure your understanding and mastery of these critical concepts. Whether you need help with threads and synchronization assignments or seek advice from threads and synchronization assignment experts, we provide tailored solutions to meet your academic needs.
Our service guarantees programming homework help that adheres to high academic standards, covering thread management, synchronization techniques, deadlock prevention, and more. With a focus on clarity and practical application, our experts ensure your assignments are not only completed on time but also enhance your comprehension of concurrent programming.
Benefit from our threads and synchronization assignment help service, designed for students seeking reliable assistance and aiming to excel in their programming coursework. If you are concerned about who will do my threads and synchronization assignment? Contact us today to explore how our expertise can support your academic success and deepen your understanding of threads and synchronization.
Understanding the Demand for Online Threads and Synchronization Assignment Help Among Students
Understanding the demand for online threads and synchronization assignment help among students reveals a growing need for expert guidance in mastering these complex concepts. Help with threads and synchronization assignments is sought after due to the intricacies involved in managing concurrent tasks and ensuring thread safety. If you are concerned about who will do my threads and synchronization assignment? Our threads and synchronization assignment experts at ProgrammingHomeworkHelp.com provide invaluable support, offering structured programming homework help that enhances understanding and boosts grades.
Students benefit from personalized assistance that clarifies challenging topics like synchronization techniques and deadlock prevention, ensuring they meet academic deadlines with confidence. Embracing online assistance allows students to excel in their programming coursework while building essential skills for future endeavors.
- Complexity: Threads and synchronization involve intricate concepts like race conditions and deadlock prevention, challenging students to grasp these advanced programming techniques.
- Time Constraints: Balancing multiple assignments and coursework deadlines can make it difficult for students to dedicate sufficient time to understand and complete threads and synchronization assignments effectively.
- Technical Challenges: Implementing synchronization mechanisms and managing concurrent processes in programming languages can be technically demanding without proper guidance.
- Risk of Errors: Without expert supervision, students may encounter errors such as data corruption or inconsistent results due to improper thread synchronization.
- Academic Pressure: The need to maintain high grades and meet academic standards in programming courses can create pressure, prompting students to seek professional assistance for threads and synchronization assignments.
The Benefits of Using Our Threads and Synchronization Assignment Help Service
Experience the numerous benefits of utilizing our threads and synchronization assignment help service at ProgrammingHomeworkHelp.com. Our threads and synchronization assignment experts offer specialized guidance to ensure clarity and mastery of complex concepts. Whether you need help with threads and synchronization assignments or seek reliable programming homework help, we provide tailored solutions that meet your academic requirements. Benefits include expert assistance in thread management, synchronization techniques, and deadlock prevention, ensuring error-free assignments and improved understanding.
Our service guarantees timely delivery, confidentiality, and a user-friendly platform that simplifies the process. Gain confidence in your programming skills and achieve higher grades with our dedicated support. If you are concerned about who will do my operating system assignment? Contact us today to leverage these advantages and excel in your threads and synchronization coursework. We handle concepts such as only one thread, attempts to acquire a single thread, threads blocked, created thread, locks mutexes, synchronization method, thread waiting, ensuring thorough understanding and implementation expertise.
- Expert Guidance: Benefit from the expertise of threads and synchronization assignment experts who provide in-depth understanding and solutions.
- Customized Solutions: Receive tailored help with threads and synchronization assignments that meet your specific academic requirements.
- Timely Delivery: Ensure your assignments are completed on schedule, meeting academic deadlines with our prompt programming homework help service.
- Confidentiality: Your information and assignments are kept secure and confidential throughout the process.
- Enhanced Understanding: Improve comprehension of thread management, synchronization techniques, and related concepts through clear explanations and examples provided by our experts.
Dive into the Diverse Topics Offered in Our Threads and Synchronization Assignment Help Service
Dive into the extensive range of topics covered by our threads and synchronization assignment help service at ProgrammingHomeworkHelp.com. Our threads and synchronization assignment experts are proficient in various areas, offering help with threads and synchronization assignments such as thread creation, synchronization techniques like mutexes and semaphores, deadlock avoidance strategies, and concurrent data structure implementations. Whether you're dealing with multiple threads, ensuring thread safety in multi-threaded programs, managing critical sections, handling attempts to acquiresingle thread access, or implementing mutual exclusion, our service provides the expertise you need for successful assignments.
Whether you're navigating basic thread management or tackling advanced synchronization challenges, our service provides programming homework help that ensures clarity and comprehensive understanding. Benefit from tailored solutions, timely delivery, and confidential assistance designed to enhance your academic performance. If you are concerned about who will do my threads and synchronization assignment? Contact us today to explore how our expertise can support your success in threads and synchronization coursework.
- Thread Creation: Learn to manage concurrent tasks with threads, understanding thread lifecycle, creation methods, and best practices for efficient execution in programming assignments.
- Mutexes and Semaphores: Explore synchronization techniques like mutexes (locks) and semaphores (counting mechanisms) to control access to shared resources and prevent data inconsistency.
- Deadlock Prevention: Study strategies to avoid deadlock situations in concurrent programming by understanding resource allocation, ordering, and deadlock detection algorithms.
- Concurrency in Data Structures: Implement concurrent data structures such as queues and hash tables to manage shared data efficiently while ensuring thread safety and consistency.
- Thread Synchronization Patterns: Discover common synchronization patterns like producer-consumer, reader-writer, and barrier synchronization for optimal resource management in multithreaded environments.
Simple Steps to Obtain Expert Threads and Synchronization Assignment Help from Us
Here are simple steps to obtain expert threads and synchronization assignment help from ProgrammingHomeworkHelp.com. Contact us with your assignment details and deadline. Our threads and synchronization assignment experts will assess your requirements and provide a competitive quote for help with threads and synchronization assignments.
Once confirmed, our team will promptly work on your assignment, ensuring programming homework help that meets high standards of quality and timeliness. Receive your completed assignment, reviewed and ready for submission, with the assurance of confidentiality and expert guidance throughout the process. If you are concerned about who will do my threads and synchronization assignment? Contact us today for expert assistance and support!
- Contact Us: Reach out to ProgrammingHomeworkHelp.com with your assignment details and deadline requirements for prompt assistance.
- Get a Quote: Receive a competitive quote from our threads and synchronization assignment experts tailored to your specific needs.
- Confirm Your Order: Once satisfied with the quote, confirm your order to initiate the assignment process with our team.
- Assignment Completion: Our experts will diligently work on your assignment, ensuring high-quality programming homework help and timely delivery.
- Receive Your Solution: Review and receive your completed assignment, ready for submission, backed by our commitment to confidentiality and academic excellence.
Claim Your Special 20% Discount on Your Next Assignment with Us
Claim your special 20% discount on your next assignment with ProgrammingHomeworkHelp.com. We offer expert threads and synchronization assignment help tailored to your academic needs. Whether you require help with threads and synchronization assignments or seek guidance from threads and synchronization assignment experts, our service ensures top-quality solutions.
To redeem your discount, simply contact us with your assignment details and mention the code provided. Our team of programming homework help specialists will handle your task with precision, adhering to deadlines and maintaining confidentiality.
Benefit from our commitment to excellence and receive your assignment at a discounted rate, enhancing both your academic performance and savings. Don't miss out on this opportunity to experience reliable and affordable assistance in threads and synchronization assignments. Claim your discount today and let us support your success in programming coursework!
Read Our Valuable Threads and Synchronization Assignment Blog Posts
Read our valuable blog posts on threads and synchronization assignment help. Gain insights and tips from our threads and synchronization assignment helpers to enhance your understanding and skills in handling complex programming tasks effectively. Whether you need help with threads and synchronization assignments or want to delve deeper into synchronization techniques, our blog provides educational content to support your academic journey. Stay informed and empowered with practical knowledge that complements your coursework and improves your programming capabilities.
Read Student Experiences with Our Threads and Synchronization Assignment Help
Read firsthand student experiences with our threads and synchronization assignment help. Discover how our dedicated threads and synchronization assignment helpers have assisted students in overcoming challenges and achieving academic success. Gain insights into our personalized approach to help with threads and synchronization assignments, ensuring comprehensive support and improvement in programming skills.
Learn About Our Dedicated Team for Threads and Synchronization Assignment Help
Learn about our dedicated team specializing in threads and synchronization assignment help. Our threads and synchronization assignment helpers are skilled professionals committed to providing expert guidance and support. With extensive experience in help with threads and synchronization assignments, they ensure tailored solutions that meet academic standards and enhance your understanding of complex programming concepts. Meet our team to discover how we can assist you in achieving academic success and mastering threads and synchronization topics effectively.
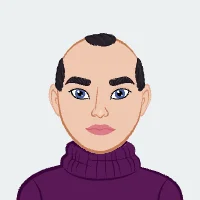
Andrew Tylor
PhD in Programming
🇺🇸 United States
Andrew Tylor is an experienced software engineer with expertise in Unix systems, process management, and shell programming.
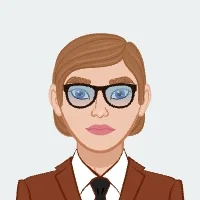
Scott Gray
PhD in Programming
🇬🇧 United Kingdom
Scott Gray is a seasoned operating systems expert with extensive experience in implementing system calls and process management environments.
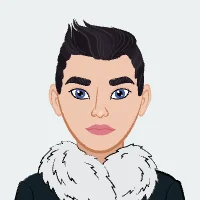
Robert Hampton
PhD in Programming
🇨🇦 Canada
Robert Hampton is an experienced software engineer specializing in operating systems and memory management. With extensive knowledge in virtual memory and algorithms.
.webp)
Madison Burgess
Ph.D. in Computer Science
🇸🇬 Singapore
Madison Burgess, holding a Ph.D. from the University of Strathclyde, United Kingdom, brings 13 years of expertise in UNIX Utility assignments. Her knowledge spans system administration, scripting, and network configurations, ensuring robust academic support.
Phoebe Davidson
Ph.D. in Computer Science
🇸🇬 Singapore
Phoebe Davidson, a Stanford University alumna with 13 years of experience and a Ph.D., excels in UNIX Utility assignments. Her expertise spans system administration, scripting, and network configurations, ensuring comprehensive support.
Benjamin Gibbons
Ph.D. in Computer Science
🇸🇬 Singapore
Benjamin Gibbons, a Ph.D. graduate from McGill University, Canada, offers 15 years of expertise in UNIX Utility assignments. His comprehensive knowledge spans system administration, scripting, and network configurations, ensuring top-tier academic support.
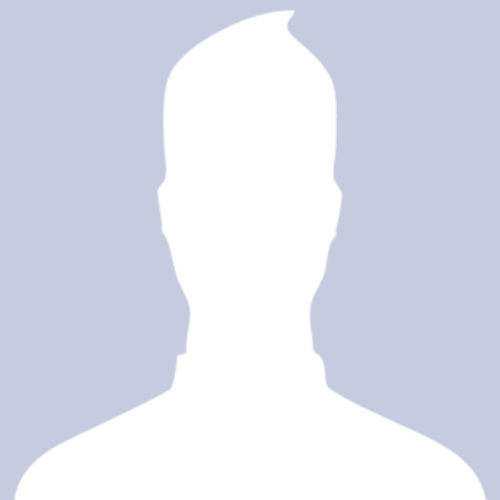
Sofia Martin
Ph.D. in Computer Science
🇨🇦 Canada
Sofia Martin, a Ph.D. graduate from the University of Waterloo, Canada, possesses 18 years of experience in UNIX Utility assignments. Her expertise includes system administration, scripting, and network configurations, ensuring comprehensive support.
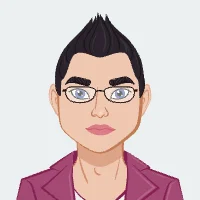
Brian Chase
Masters in Computer Science
🇨🇦 Canada
Brian Chase is a highly skilled Operating System Assignment Expert with a Master's in Computer Science. Proficient in OS design, C/C++, and assembly, he delivers top-notch solutions. With a structured approach and commitment to excellence, Brian ensures academic success and client satisfaction.
Nellie H. Parker
Ph.D. in Computer Science
🇸🇬 Singapore
Nellie H. Parker, a distinguished graduate from the prestigious University, holds a PhD in Computer Science and has amassed 9 years of experience in the field. Nellie's expertise in file handling assignments is reflected in her completion of 855 assignments, offering students meticulous and effective solutions that support their academic success.
Nita B. Russell
Ph.D. in Computer Science
🇨🇦 Canada
Nita B. Russell, an accomplished scholar from the University of Alberta, brings 8 years of experience in computer science and a PhD credential. With a focus on file handling assignments, Nita has successfully delivered 776 assignments, providing students with insightful solutions tailored to academic standards.
Sonja R. Newman
Ph.D. in Computer Science
🇨🇦 Canada
Sonja R. Newman, an esteemed graduate of Ghent University, is a seasoned professional with 7 years of experience in computer science. With a PhD in the field, Sonja has excelled in file handling assignments, completing 634 projects to date. Her comprehensive knowledge and meticulous approach ensure high-quality outcomes for students.
Casey T. Ford
Ph.D. in Computer Science
🇬🇧 United Kingdom
Casey T. Ford, a distinguished alum of Cardiff University, holds a PhD in Computer Science and boasts over 5 years of experience in the field. Specializing in file handling assignments, Casey has successfully completed 550 assignments, demonstrating expertise and dedication in delivering top-notch solutions.
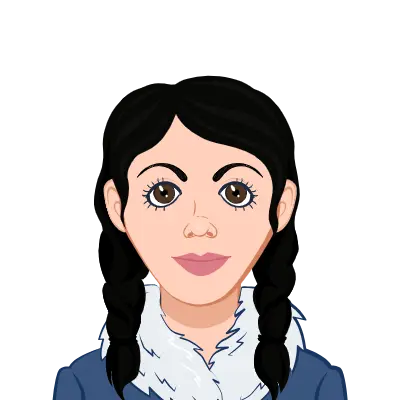
Kieran Gallagher
Ph.D. in Computer Science
🇦🇺 Australia
Kieran Gallagher, a Ph.D. graduate from Monash University, Australia, offers a decade of expertise in simulated file system assignments. Her proficiency ensures accurate solutions and in-depth knowledge of file system simulations.
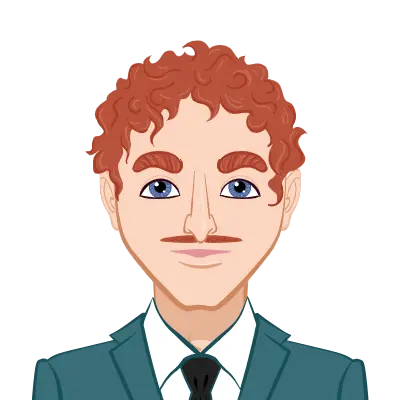
Thomas Buckley
Ph.D. in Computer Science
🇬🇧 United Kingdom
Thomas Buckley, a Ph.D. graduate from the University of Oxford, UK, boasts 13 years of experience. His expertise in simulated file system assignments ensures meticulous solutions and deep insights into file system dynamics.
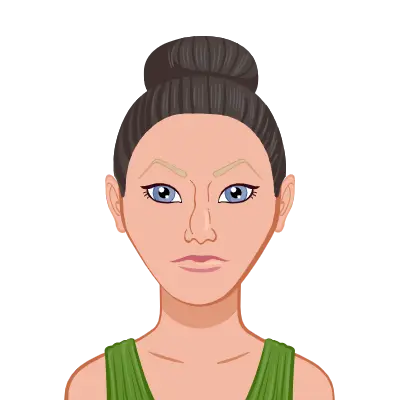
Georgia Myers
Ph.D. in Computer Science
🇨🇦 Canada
Georgia Myers, a Ph.D. graduate from the University of Ottawa, Canada, brings 15 years of experience in simulated file system assignments. Her expertise ensures meticulous solutions and deep insight into file system dynamics.
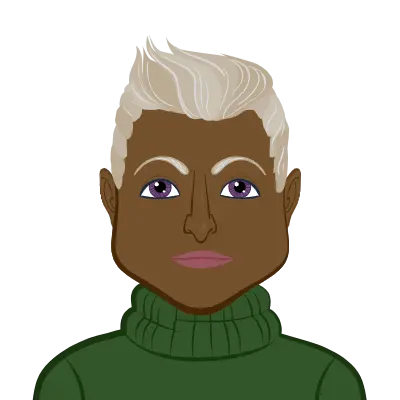
Patrick Austin
Ph.D. in Computer Science
🇦🇺 Australia
Patrick Austin, a Ph.D. graduate from the University of Queensland, Australia, offers 18 years of expertise in simulated file system assignments. His in-depth knowledge ensures meticulous solutions and academic excellence.
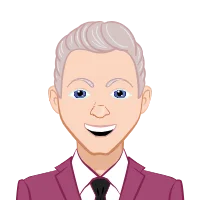
Dr. Michael C. Tomlinson
PhD in Computer Science
🇬🇧 United Kingdom
Dr. Michael C. Tomlinson graduated with a PhD in Computer Science from the University of Oxford. With more than 8 years of experience, he has completed over 1000+ Command Shell Assignments. Known for his meticulous approach and deep understanding of command line programming, Dr. Tomlinson excels in guiding students through challenging shell scripting tasks with clarity and expertise.
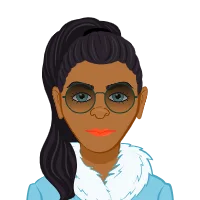
Dr. Michelle D. Brown
PhD in Computer Science
🇺🇸 United States
Dr. Michelle D. Brown received her PhD in Computer Science from the University of Wisconsin-Madison. With a solid background and over 7 years of experience, she has successfully delivered 900+ Command Shell Assignments. Her areas of expertise include shell variables, loops, and script optimization techniques, ensuring high-quality outputs for students.
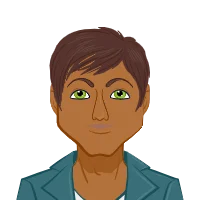
Dr. Jason C. Mellor
PhD in Computer Science
🇺🇸 United States
Dr. Jason C. Mellor earned his PhD in Computer Science from the University of Alberta. He brings over 6 years of experience in the industry, having completed 800+ Command Shell Assignments with precision. His specialization includes advanced script debugging and innovative solutions tailored to complex programming challenges.
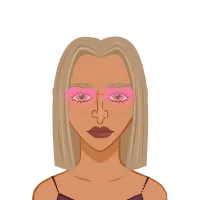
Dr. Mary G. Meigs
PhD in Computer Science
🇮🇪 Ireland
Dr. Mary G. Meigs holds a PhD in Computer Science from Trinity College Dublin. With over 5 years of experience, she has successfully completed 700+ Command Shell Assignments. Her expertise lies in optimizing shell scripts and ensuring efficient command execution, making her a trusted mentor in the field.
Related Topics
Frequently Asked Questions (FAQs)
Explore our frequently asked questions (FAQs) about threads and synchronization assignment help. Find answers regarding help with threads and synchronization assignments, our threads and synchronization assignment helpers, and how we ensure top-notch support for your programming needs. Get clarity on our services, turnaround times, and how to get started on your assignments with confidence.