Claim Your Offer
Unlock an amazing offer at www.programminghomeworkhelp.com with our latest promotion. Get an incredible 10% off on your all programming assignment, ensuring top-quality assistance at an affordable price. Our team of expert programmers is here to help you, making your academic journey smoother and more cost-effective. Don't miss this chance to improve your skills and save on your studies. Take advantage of our offer now and secure exceptional help for your programming assignments.
We Accept
Preview Top-Notch Data Structures and Algorithms Samples
Our Data Structures and Analysis of Algorithms Assignment Help service provides students with expertly crafted samples to guide their academic journey. These samples, created by our data Structures and Analysis of Algorithms Homework experts, showcase high-quality solutions and detailed analyses to help you understand complex concepts. Whether you need assistance with array implementations, binary trees, graph algorithms, or dynamic programming, our samples exemplify clarity, accuracy, and depth of analysis. Each sample reflects our commitment to delivering high-quality solutions tailored to academic requirements. Dive into our samples to see how we can help you excel in your coursework with precision and confidence.
Computer Science
Computer Science
Computer Science
Computer Science
Computer Science
Computer Science
Computer Science
Computer Science
Computer Science
Computer Science
Computer Science
Computer Science
Computer Science
Computer Science
Computer Science
Computer Science
Computer Science
Computer Science
Computer Science
Computer Science
Premium Data Structures and Analysis of Algorithms Assignment Help at Budget Prices
Our Data Structures and Analysis of Algorithms Assignment Help service offers affordable pricing to ensure every student can access expert assistance. When you seek help with Data Structures and Analysis of Algorithms Assignment, our team of experts provides high-quality solutions without straining your budget. Choose our service for exceptional support at prices that fit your financial needs.
Assignment Type | Turnaround Time | Price (USD) |
---|---|---|
Array and Linked List Implementation | 24 Hours | $30 |
Binary Trees and Binary Search Trees | 48 Hours | $40 |
Graph Algorithms and Traversals | 3 Days | $50 |
Sorting Algorithms (Quick Sort, Merge Sort, etc.) | 5 Days | $45 |
Dynamic Programming Problems | 1 Week | $60 |
Hash Tables and Hash Functions | 48 Hours | $35 |
Greedy Algorithms and Applications | 72 Hours | $55 |
- Key Role of Algorithms in Computer Science Curriculum
- Data Structures and Analysis of Algorithms Assignment Help
- Why Students Should Get Expert Help with Data Structures and Analysis of Algorithms Assignments?
- Implementation of Binary Search Tree (BST)
- Advantages Offered by Our Data Structures and Analysis of Algorithms Assignment Help Service
- Simple Steps to Submit Your Assignment to Our Data Structures and Analysis of Algorithms Assignment Experts
- Remarkable Offer at programminghomeworkhelp.com
Key Role of Algorithms in Computer Science Curriculum
Data Structures and Analysis of Algorithms play a crucial role in the education of college students across various disciplines, especially in computer science and related fields. By studying data structures in any programming languages, students learn to design and implement data storage solutions that optimize performance and memory usage. Analysis of algorithms, on the other hand, teaches students to evaluate the efficiency of different algorithms in solving computational problems, helping them choose the best approach for various tasks.
Moreover, proficiency in these areas equips students with problem-solving skills essential for tackling complex software development challenges. Understanding data structures and algorithms allows students to write more efficient and scalable code, which is crucial in modern software applications. It also prepares them for advanced topics in artificial intelligence, machine learning, database management, and more, where efficient data handling and algorithmic efficiency are paramount.
Data Structures and Analysis of Algorithms Assignment Help
At our Data Structures and Analysis of Algorithms Assignment Help service, we understand the challenges students face when navigating complex coursework. You may wonder, "Who will do my Computer Science Assignment?" Rest assured; our service is tailored to provide expert assistance from professionals well-versed in the intricacies of this field. Our team consists of dedicated subject matter expert with advanced degrees and extensive experience. They are committed to delivering high-quality data structure assignment that meet academic standards and exceed expectations.
When you choose our Computer Programming Assignment Help service, you gain access to comprehensive support tailored to your specific needs. Whether you're grappling with array implementations, graph algorithms, or dynamic programming, our experts is equipped to handle diverse topics with precision and clarity. We ensure thorough understanding and application of concepts, guaranteeing that your data structure homework is not only completed on time but also reflect deep insights and critical analysis.
Furthermore, our assignment help online service focuses on transparency, affordability, and confidentiality. We offer competitive prices with no hidden costs, making our Data Structures and Analysis of Algorithms Assignment Help accessible to students worldwide. Your privacy is protected with secure systems for payment and communication. With features like live chat, you can get instant support, ensuring a strong foundation for your future in computer science and related fields.
Why Students Should Get Expert Help with Data Structures and Analysis of Algorithms Assignments?
Understanding the intricacies of data structures and algorithms is essential for any computer science student, but the complexity of these topics often poses significant challenges. By seeking expert help with Data Structures and Analysis of Algorithms Assignment tasks, students can gain a clearer understanding of difficult concepts, such as trees, graphs, sorting algorithms, and complexity analysis. Experts provide tailored explanations and practical examples, enabling students to grasp the material more effectively than they might through self-study alone.
Moreover, professional guidance ensures that students receive high-quality, accurate solutions to their assignments. Data Structures and Analysis of Algorithms Homework experts have extensive knowledge and experience, which allows them to solve problems efficiently and correctly. This not only helps students complete their assignments on time but also teaches them the correct methodologies and best practices for approaching similar problems in the future. Consequently, students can improve their problem-solving skills and apply these techniques in their exams and future projects.
Lastly, getting expert help with Data Structures and Analysis of Algorithms Assignment from programming assignment help service can significantly reduce the stress and pressure associated with complex assignments. Balancing multiple courses, extracurricular activities, and personal commitments can be overwhelming. When students receive professional assistance, they can manage their workload more effectively and maintain a healthy academic-life balance. By entrusting their assignments to experts, students can focus on understanding the material rather than just completing tasks, leading to a more enriching and less stressful educational experience.
Implementation of Binary Search Tree (BST)
class TreeNode:
def __init__(self, key):
self.left = None
self.right = None
self.val = key
def insert(root, key):
if root is None:
return TreeNode(key)
else:
if root.val < key:
root.right = insert(root.right, key)
else:
root.left = insert(root.left, key)
return root
def inorder_traversal(root):
if root:
inorder_traversal(root.left)
print(root.val)
inorder_traversal(root.right)
# Example usage:
if __name__ == "__main__":
r = TreeNode(50)
r = insert(r, 30)
r = insert(r, 20)
r = insert(r, 40)
r = insert(r, 70)
r = insert(r, 60)
r = insert(r, 80)
print("Inorder traversal of the BST is:")
inorder_traversal(r)
Explanation:
- TreeNode Class: Defines the structure of a tree node with attributes left and right for left and right children, and val for the node's value.
- Insert Function: Recursively inserts a new key into the BST while maintaining the property that all nodes in the left subtree are less than the root, and all nodes in the right subtree are greater.
- Inorder Traversal Function: Performs an inorder traversal of the BST, which visits nodes in ascending order (left subtree, root, right subtree).
Advantages Offered by Our Data Structures and Analysis of Algorithms Assignment Help Service
Our Data Structures and Analysis of Algorithms Assignment Help service is designed to provide comprehensive support to students facing challenges in this critical area of computer science. We understand the complexities involved in mastering data structures and algorithms, and we are committed to helping students navigate through their coursework effectively. Here are the advantages offered by our service:
- Expert Assistance: Your assignments are handled by professionals with advanced degrees and extensive experience in Data Structures and Analysis of Algorithms.
- Customized Solutions: We tailor our solutions to meet your specific assignment requirements, ensuring accuracy and adherence to guidelines.
- Timely Delivery: We prioritize punctuality, ensuring that your online data structure assignments are delivered on time, allowing you to meet deadlines without stress.
- Quality Assurance: Every assignment undergoes rigorous quality checks to ensure it meets academic standards and reflects comprehensive understanding.
- 24/7 Support: Our customer support team is available round-the-clock to address any queries or concerns you may have, providing prompt assistance whenever needed.
- Plagiarism-Free Content: We guarantee originality in all our assignments, with thorough plagiarism checks conducted before delivery.
- Confidentiality: Your privacy is paramount to us. We ensure secure handling of your personal information and assignment details.
- Affordable Pricing: Our services are priced competitively to make them accessible to students, with transparent pricing and no hidden costs.
- Revision Policy: We offer unlimited revisions to ensure your satisfaction with the final assignment, accommodating any feedback or changes you may require.
We are committed to helping students navigate through their coursework effectively. Don't worry about "Who will do my Data Structures and Analysis of Algorithms Assignment?" Our dedicated team of experts ensures that every assignment receives personalized attention and adherence to academic standards. We provide top-notch programming homework help, ensuring that each student receives comprehensive support in mastering data structures and algorithms.
Simple Steps to Submit Your Assignment to Our Data Structures and Analysis of Algorithms Assignment Experts
We strive to simplify the process of submitting your assignments to our Data Structures and Analysis of Algorithms Assignment Experts at programminghomeworkhelp.com. Our Data Structures and Analysis of Algorithms Assignment Help service ensures that you can navigate this process smoothly, receiving expert assistance whenever you need it for your academic success. Here is the simple step by step process to submit your assignment:
- Access our website: Visit programminghomeworkhelp.com to begin the submission process.
- Fill Out Assignment Details: Provide comprehensive details about your Data Structures and Analysis of Algorithms assignment, including requirements, guidelines, and any specific instructions from your professor.
- Select Deadline: Choose your desired deadline for the assignment submission, ensuring we can deliver within your timeframe.
- Get a Quote: Receive a quote for the assignment based on its complexity, length, and deadline.
- Make Payment: Proceed to make payment securely through our trusted payment gateway, ensuring confidentiality and security of your financial information.
- Assignment: Once payment is confirmed, our experts will start working on your Data Structures and Analysis of Algorithms assignment.
- Track Progress: Stay updated on the progress of your assignment and communicate directly with our experts if needed.
- Receive Completed Assignment: Get your completed assignment delivered to you within the agreed timeframe.
- Review and Feedback: Review the assignment thoroughly and provide any feedback or additional instructions for revisions if necessary.
- Support and Assistance: Enjoy ongoing support from our customer service team and experts for any queries or concerns you may have.
- Revision Requests: If required, request revisions to ensure the assignment meets your expectations and academic standards.
- Final Submission: Submit the final assignment confidently to your professor and excel in your Data Structures and Analysis of Algorithms coursework.
This streamlined process ensures that you receive top-quality assistance from our Data Structures and Analysis of Algorithms Assignment Experts at programminghomeworkhelp.com, making your academic journey smoother and more successful.
Remarkable Offer at programminghomeworkhelp.com
We are thrilled to introduce a remarkable offer that enhances your experience with our Data Structures and Analysis of Algorithms assignment help service at programminghomeworkhelp.com. Through our Refer a Friend program, you can now enjoy a generous 50% discount on your next assignment when you refer a friend to our services. This offer is designed to reward our loyal customers while also extending our quality assistance to new students seeking help with their academic assignments.
Participating in our Refer a Friend offer is simple and beneficial. When you refer a friend to programminghomeworkhelp.com they receive expert guidance and support from our Data Structures and Analysis of Algorithms assignment helpers. Meanwhile, you earn the opportunity to save significantly on your next assignment with us. This initiative not only fosters a sense of community among our clients but also ensures that everyone benefits from our top-notch custom programming assignment help services.
Our commitment to excellence extends beyond providing high-quality solutions for Data Structures and Analysis of Algorithms assignments. With this remarkable offer, programminghomeworkhelp.com aims to create a rewarding experience for both existing and new customers, reinforcing our dedication to academic success and customer satisfaction. Don’t miss out on this opportunity to maximize your savings while receiving expert assistance from our trusted team of professionals.
Check out In-Depth Blog Articles on Algorithm Analysis by Experts
Explore dedicated blog articles on our Data Structures and Analysis of Algorithms Assignment Help service, where students can access expertly crafted content aimed at deepening their understanding of these essential topics. These blogs, written by our data Structures and Analysis of Algorithms Homework experts, cover a wide range of topics, offering valuable insights and practical tips. Whether you need help with Data Structures and Analysis of Algorithms Assignment challenges or are looking to deepen your knowledge, our professional blogs are designed to support your academic journey and boost your confidence. Explore our blog and benefit from the expertise of our seasoned professionals.
Find Genuine Reviews about Our Data Structures and Analysis of Algorithms Assignment Help service
Discover why students rave about our Data Structures and Analysis of Algorithms Assignment Help service at programminghomeworkhelp.com. Our dedicated Data Structures and Analysis of Algorithms Assignment helpers provide unparalleled help with Data Structures and Analysis of Algorithms Assignment, ensuring top-notch solutions tailored to your academic needs. Students praise our expertise in delivering comprehensive support, from intricate algorithmic problems to complex data structure implementations. With a track record of excellence, we've earned trust through exceptional service and timely deliveries. Read our reviews to see how we've helped students excel in their coursework, providing clarity and confidence in every assignment we handle.
Introducing Our Seasoned Data Structures and Analysis of Algorithms Assignment Experts
Meet our dedicated team of Data Structures and Analysis of Algorithms Assignment experts who are ready to provide you with top-notch help with Data Structures and Analysis of Algorithms Assignment. Whether you're worrying about "who will do my Data Structures and Analysis of Algorithms Assignment?" or need any programming homework help, our experts are here to assist. With years of experience and deep expertise in the field, they ensure thorough understanding and precise execution of assignments. Trust our professionals to deliver tailored solutions that meet your academic requirements and help you excel in your coursework effortlessly.
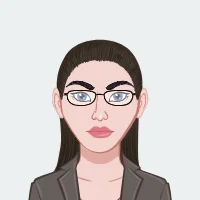
Eve Kemp
PhD in Programming
🇺🇸 United States
Eve Kemp is a computer science professional with extensive experience in software development and computational theory. Her expertise spans across pattern recognition, algorithm design, and software development, bringing a deep understanding to complex computational problems and practical solutions.
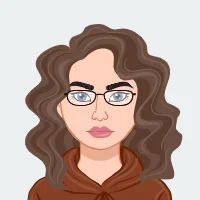
Sandra Alva
Masters in Programming
🇺🇸 United States
Sandra Alva is a software engineer with over 10 years of experience in reverse engineering and low-level programming, specializing in machine code analysis and instruction set architectures.
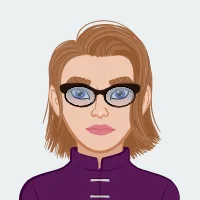
Josh Kaiser
Masters in Programming
🇺🇸 United States
Josh Kaiser is a computer architect with over 15 years of experience in optimizing CPU performance and pipeline design, specializing in data hazards, forwarding techniques, and advanced processor efficiency.
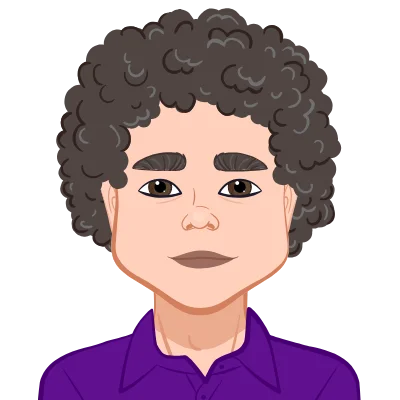
Andrew Bell
Ph.D. in Computer Science
🇨🇦 Canada
Andrew Bell, a seasoned Analog Electronics Assignment expert, holds a Ph.D. from the University of Ottawa, Canada. With 10 years of experience, he specializes in circuit analysis, transistor design, and amplifier optimization.
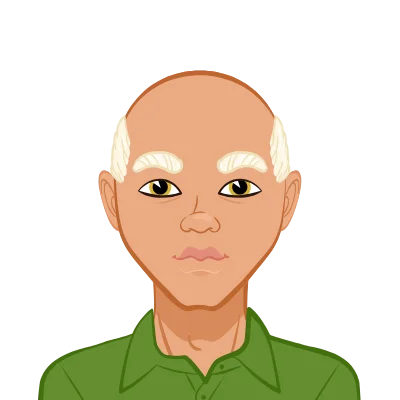
Adam Cook
Ph.D. in Computer Science
🇦🇺 Australia
Adam Cook, a seasoned Analog Electronics Assignment expert, holds a Ph.D. from Monash University, Australia. With 13 years of experience, he specializes in circuit analysis, transistor design, and amplifier optimization.
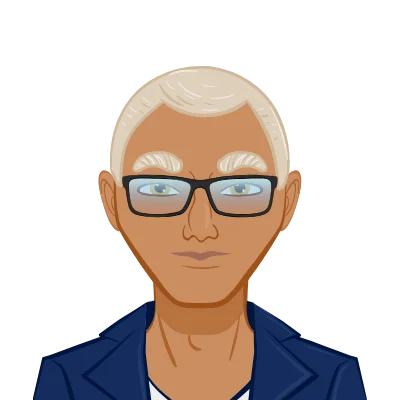
Zoe Carey
Ph.D. in Computer Science
🇬🇧 United Kingdom
Zoe Carey, a seasoned Analog Electronics Assignment expert, holds a Ph.D. from Imperial College London, UK. With 15 years of experience, she specializes in circuit analysis, transistor design, and amplifier optimization.
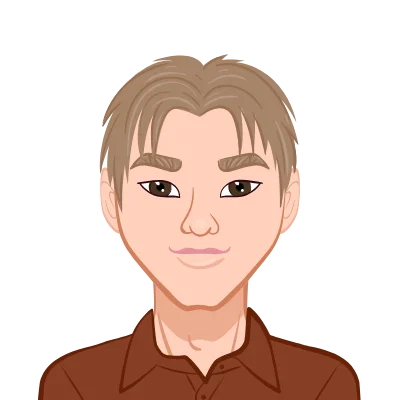
Noah Mellor
Ph.D. in Computer Science
🇺🇸 United States
Noah Mellor, a seasoned Analog Electronics Assignment expert, holds a Ph.D. from Columbia University, United States, and brings 18 years of comprehensive industry experience. His expertise spans circuit analysis, transistor design, and advanced amplifier configurations.
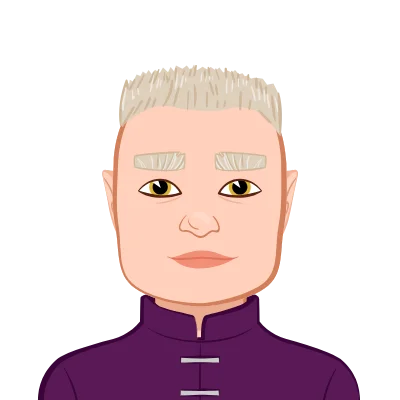
Lucas Chandler
Ph.D. in Computer Science
🇨🇦 Canada
Lucas Chandler, Ph.D., from the University of British Columbia, Canada, brings 10 years of expertise in Reverse-Echo-Server and Client Assignments. His academic rigor ensures high-quality solutions, reflecting his commitment to excellence and client satisfaction.
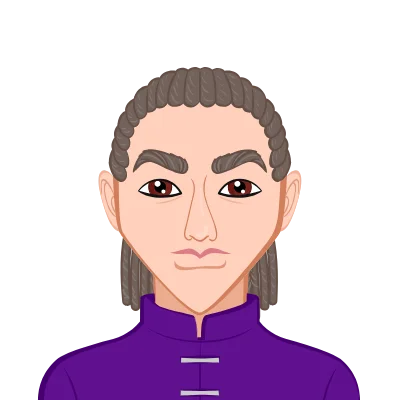
Aidan Dodd
Ph.D. in Computer Science
🇦🇺 Australia
Meet Aidan Dodd, a Ph.D. holder from the University of New South Wales, Australia, with 13 years of expertise in Reverse-Echo-Server and Client Assignments. Aidan delivers meticulous solutions, ensuring academic excellence and client satisfaction.
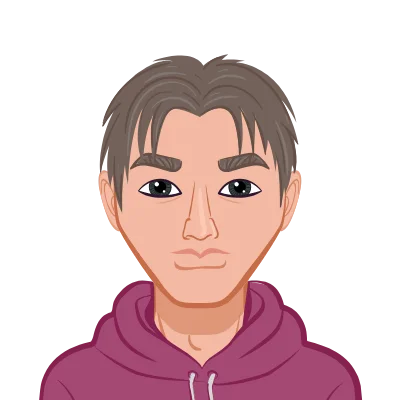
Ben Stone
Ph.D. in Computer Science
🇬🇧 United Kingdom
Meet Ben Stone, an esteemed expert in Reverse-Echo-Server and Client Assignments. Ben holds a Ph.D. from the University of Oxford, United Kingdom, and brings over 15 years of invaluable experience. His proficiency ensures top-tier solutions and academic excellence in every assignment.
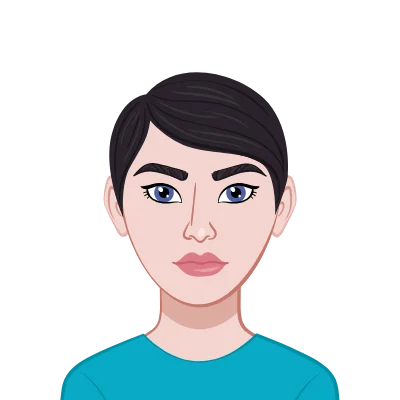
Courtney Sullivan
Ph.D. in Computer Science
🇺🇸 United States
Courtney Sullivan, Ph.D., brings 18 years of expertise in Reverse-Echo-Server and Client Assignments, graduating from Massachusetts Institute of Technology, United States. Her meticulous approach ensures impeccable solutions and academic success for students.
Deborah D. Noel
PhD in Computer Science
🇸🇬 Singapore
Deborah D. Noel, from Nanyang Technological University in Singapore, holds a PhD in computer science and 5 years of experience. Having completed 550 assignments, Noel's expertise lies at the intersection of data science and computational biology, where her research has yielded transformative insights into complex biological systems.
Jane C. Harris
PhD in Computer Science
🇺🇸 United States
Jane C. Harris, representing the University of Illinois in the United States, combines 9 years of experience with 756 completed assignments. Her focus on software engineering and human-computer interaction has led to groundbreaking research and innovation, setting new standards in the field of computer science.
Janice J. Seymore
PhD in Computer Science
🇬🇧 United Kingdom
Janice J. Seymore, a graduate of Carnegie Mellon University in Pittsburgh, Pennsylvania, brings 8 years of experience to the forefront of cybersecurity and data analytics. With 635 assignments under her belt, Seymore's expertise is unparalleled, making her a sought-after authority in digital security solutions.
Selma P. Davis
PhD in Computer Science
🇬🇧 United Kingdom
Selma P. Davis, a distinguished alumna of the University of Cambridge, England, holds a PhD in computer science and boasts 11 years of experience. With a remarkable track record of completing 900 assignments, her expertise lies in the realms of artificial intelligence and machine learning. Davis's contributions to the field have reshaped technological landscapes, earning her global recognition as a trailblazer in computational research.
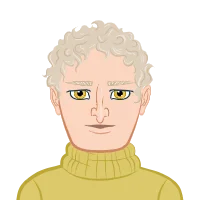
Dr. Arthur M. Garwood
PhD in Computer Science from the University of York
🇬🇧 United Kingdom
Dr. Arthur M. Garwood holds a PhD in Computer Science from the University of York and boasts over 8 years of experience in the field. With an impressive track record of completing over 600 Data Structures and Analysis of Algorithms Assignments, Dr. Garwood brings a wealth of expertise and academic rigor to his work. Known for his innovative approach and commitment to excellence, he ensures each assignment meets high standards of quality and clarity, empowering students to succeed in their studies.
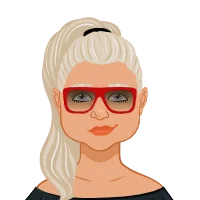
Dr. Carrie S. Benoit
PhD in Computer Science from the University of Texas
🇺🇸 United States
Dr. Carrie S. Benoit is a seasoned expert with a PhD in Computer Science from the University of Texas and more than 7 years of experience specializing in Data Structures and Analysis of Algorithms. Having successfully completed over 500 assignments, Dr. Benoit is known for her thorough approach and attention to detail. She leverages her academic background and practical experience to provide students with insightful solutions that foster learning and academic growth.
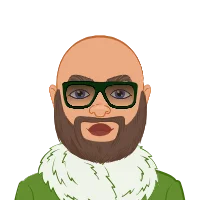
Dr. Wayne D
PhD in Computer Science from the University of Melbourne
🇺🇸 United States
Hedrick: Dr. Wayne D. Hedrick earned his PhD in Computer Science from the University of Melbourne and has over 6 years of experience in academia and industry. With a portfolio of over 400 completed Data Structures and Analysis of Algorithms Assignments, he combines theoretical knowledge with practical insights to deliver comprehensive solutions.
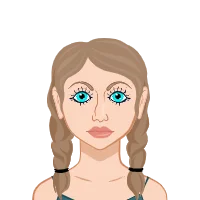
Dr. Crystal D. Hopkins
PhD in Computer Science from the University of Bristol
🇬🇧 United Kingdom
Dr. Crystal D. Hopkins holds a PhD in Computer Science from the University of Bristol and brings over 5 years of experience in the field. She has successfully completed over 300 Data Structures and Analysis of Algorithms Assignments, demonstrating her deep understanding and expertise in the subject. Driven by a passion for teaching and research, Dr. Hopkins ensures each assignment is meticulously crafted to meet academic standards and exceed student expectations.
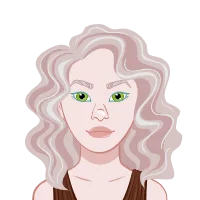
Dr. Annette J. Brown
PhD
🇬🇧 United Kingdom
Dr. Annette J. Brown holds a PhD from the University of Oxford and boasts over a decade of experience in Computer Science and AWS assignments. With a remarkable portfolio of over 1000 successfully completed assignments, Dr. Brown is renowned for her deep expertise, meticulous approach, and commitment to delivering exceptional Amazon Web Services solutions that empower student success.
Related Topics
Frequently Asked Questions
Explore our comprehensive Frequently Asked Questions (FAQs) to find answers to common queries about our Data Structures and Analysis of Algorithms Assignment Help service. Whether you're thinking "who will do my Data Structures and Analysis of Algorithms Assignment?" or seeking details about our Data Structures and Analysis of Algorithms Assignment helpers, we provide clear and informative responses. Our FAQs cover topics such as service details, assignment process, pricing, and more, ensuring you have all the information needed to make an informed decision. Dive into our FAQ to discover how we can assist you effectively and efficiently with your academic challenges.
We understand the importance of deadlines. Our team is equipped to handle urgent assignments and can deliver high-quality solutions within tight timelines without compromising on quality.
Yes, we prioritize confidentiality. Your personal information and assignment details are kept secure and confidential. We use encrypted communication and secure payment methods to protect your privacy.
We offer unlimited revisions to ensure your satisfaction. If you need any changes or additions to your assignment, simply let us know, and we'll revise it accordingly at no extra cost.
Our experts are highly qualified with advanced degrees and extensive experience in the field. We follow a rigorous quality assurance process, including plagiarism checks and thorough reviews before delivery.
Yes, you can! We provide the option to select an expert based on their profile and expertise. If you're unsure, our team can recommend the most suitable expert for your specific requirements.
Placing an order is simple! Visit our website, fill out the order form with your assignment details, and proceed with payment. Our team will assign the best-suited expert to work on your assignment promptly.