Claim Your Offer
Unlock an amazing offer at www.programminghomeworkhelp.com with our latest promotion. Get an incredible 10% off on your all programming assignment, ensuring top-quality assistance at an affordable price. Our team of expert programmers is here to help you, making your academic journey smoother and more cost-effective. Don't miss this chance to improve your skills and save on your studies. Take advantage of our offer now and secure exceptional help for your programming assignments.
We Accept
View Our Free Assignment Samples for a Complete Perspective
View our free assignment samples at ProgrammingHomeworkHelp.com for a comprehensive understanding of Reverse-Echo-Server and Client assignment help. Gain insights from expertly crafted solutions and see how our Reverse-Echo-Server and Client assignment experts tackle complex tasks. For top-notch programming homework help, explore our samples to ensure clarity and originality in your assignments.
Computer Science
Computer Science
Computer Science
Computer Science
Computer Science
Computer Science
Computer Science
Computer Science
Computer Science
Computer Science
Computer Science
Computer Science
Computer Science
Computer Science
Computer Science
Computer Science
Computer Science
Computer Science
Computer Science
Computer Science
Pocket-Friendly Reverse-Echo-Server and Client Assignment Help Tailored Just for You
Get pocket-friendly Reverse-Echo-Server and Client assignment help at ProgrammingHomeworkHelp.com, tailored specifically to your requirements. Our Reverse-Echo-Server and Client assignment experts ensure clarity and originality in every solution, providing expert guidance for all your programming homework help needs. Benefit from cost-effective solutions without compromising on quality, backed by years of experience and a commitment to excellence. Explore how we can assist you in achieving academic success with our customized services.
Description | Price Range |
Basic Reverse-Echo-Server and Client Tasks | $15 - $30 |
Intermediate Challenges with Server-Client Models | $35 - $60 |
Advanced Configuration in Reverse-Echo-Server and Client | $65 - $100 |
Complex Server-Client Projects and Scalable Solutions | $120 - $200+ |
Priority Service for Any Level of Assignment | Additional 50% |
Consultation on Reverse-Echo-Server and Client Assignment | $20 - $50 per hour |
- Understanding Reverse-Echo-Server and Client
- Socket Communication: Reverse Echo Server and Client
- Reverse-Echo-Server and Client Assignment Help
- Reasons to Invest in Reverse-Echo-Server and Client Assignment Help for Students
- Exclusive Student Offer: Save 20% on Your Next Assignment
- Discover the Benefits of Our Reverse-Echo-Server and Client Assignment Help Service
- Easy Steps to Connect with Our Reverse-Echo-Server and Client Assignment Help Experts
- Varied Topics Covered in Our Reverse-Echo-Server and Client Assignment Help Service
Understanding Reverse-Echo-Server and Client
Reverse-Echo-Server and Client refers to a network communication setup where a server and client interact in a specific manner. In this context, the Reverse-Echo-Server and Client assignment help focuses on understanding and implementing this interaction effectively. A reverse-echo-server is designed to receive data from a client, process it, and then send it back to the client, functioning opposite to a typical echo server. This assignment delves into building a simple reverse mechanism where the server receives a message from the client, processes it, and sends the source in reverse order back to the client, ensuring a comprehensive grasp of network programming concepts.
This setup often involves intricate programming tasks that require expertise in network protocols and socket programming. If you are wondering who will do my Reverse-Echo-Server and Client assignment? Our reverse-echo-server and client assignment experts at ProgrammingHomeworkHelp.com specialize in providing programming homework help tailored to these assignments. Whether it's setting up a simple reverse echo server, managing how the reverse echo server receives and replies the same message, or ensuring the client program stops and waits while the user types and sends the message, our team is here to support you.
Socket Communication: Reverse Echo Server and Client
# Reverse Echo Server
import socket
HOST = 'localhost'
PORT = 12345
with socket.socket(socket.AF_INET, socket.SOCK_STREAM) as server_socket:
server_socket.bind((HOST, PORT))
server_socket.listen()
print(f'Server listening on {HOST}:{PORT}')
conn, addr = server_socket.accept()
with conn:
print(f'Connected by {addr}')
while True:
data = conn.recv(1024)
if not data:
break
conn.sendall(data[::-1])
print('Connection closed')
# Reverse Echo Client
import socket
HOST = 'localhost'
PORT = 12345
MESSAGE = 'Hello, server!'
with socket.socket(socket.AF_INET, socket.SOCK_STREAM) as client_socket:
client_socket.connect((HOST, PORT))
client_socket.sendall(MESSAGE.encode())
data = client_socket.recv(1024)
print(f'Received from server: {data.decode()}')
In this example:
- The server listens for incoming connections and reverses the message sent by the client.
- The client sends a message ('Hello, server!') to the server and receives the reversed message back.
Make sure to adjust the HOST and PORT values based on your network setup. This code demonstrates a basic implementation and can be expanded for more complex functionalities as needed in Reverse-Echo-Server and Client assignments.
Reverse-Echo-Server and Client Assignment Help
Looking for Reverse-Echo-Server and Client assignment help? Our platform, ProgrammingHomeworkHelp.com, offers expert assistance tailored to your needs. Whether you need help with reverse-echo-server and client assignments or seek guidance from a seasoned reverse-echo-server and client assignment expert, we provide comprehensive support. Our team specializes in programming homework help, ensuring clarity in concepts like network protocols and socket programming.
By choosing our services, students gain practical insights into server-client interactions, error handling, and data transmission. We prioritize delivering original solutions that meet academic standards, helping you excel in your programming coursework. If you are wondering who will do my Reverse-Echo-Server and Client assignment? Explore our platform for reliable support and enhance your understanding of reverse-echo-server and client assignments with our expert guidance.
Reasons to Invest in Reverse-Echo-Server and Client Assignment Help for Students
Investing in Reverse-Echo-Server and Client assignment help is essential due to the complexity involved in understanding server-client interactions. Our reverse-echo-server and client assignment experts at ProgrammingHomeworkHelp.com provide expert help with reverse-echo-server and client assignments, simplifying concepts like network protocols and socket programming.
This programming homework help ensures students grasp intricate details such as error handling and data transmission, paving the way for clearer understanding and academic success in programming fields. If you are wondering who will do my Reverse-Echo-Server and Client assignment? Rest assured, we are here to help you succeed!
- Complex Concepts: Understanding server-client interactions and socket programming can be challenging without expert guidance.
- Technical Requirements: Assignments often require precise implementation of network protocols and error handling, which can be daunting without proper expertise.
- Time Constraints: Balancing coursework with other responsibilities can make it difficult to devote sufficient time to mastering these concepts.
- Accuracy Demands: Assignments must adhere to strict academic standards, requiring precise solutions and clear explanations.
- Future Impact: Mastery of these concepts is crucial for future programming tasks and career prospects, making thorough understanding essential.
Exclusive Student Offer: Save 20% on Your Next Assignment
Unlock an exclusive opportunity with ProgrammingHomeworkHelp.com! As a student, you can now save 20% on your next assignment with our specialized reverse-echo-server and client assignment help service. Whether you need help with reverse-echo-server and client assignments or seek guidance from reverse-echo-server and client assignment experts, we're here to support your academic journey. Our platform is dedicated to providing best programming homework help, ensuring that you receive expertly crafted solutions tailored to your needs.
At ProgrammingHomeworkHelp.com, we understand the challenges students face with complex programming tasks. That's why we offer this exclusive discount to ease your academic burden while maintaining the highest standards of quality and reliability. Take advantage of this opportunity to excel in your studies with confidence. Trust our team of experienced professionals to deliver exceptional results and help you achieve your academic goals. Don't miss out—claim your discount today and experience the difference with ProgrammingHomeworkHelp.com!
Discover the Benefits of Our Reverse-Echo-Server and Client Assignment Help Service
Discover the comprehensive benefits of our Reverse-Echo-Server and Client assignment help service at ProgrammingHomeworkHelp.com. Our dedicated reverse-echo-server and client assignment experts provide tailored help with reverse-echo-server and client assignments, ensuring mastery of network protocols and socket programming. With our programming homework help, students gain clarity in complex concepts like error handling and data transmission, crucial for academic success. Whether it's handling messages from a user, ensuring the client sends the message and receives it after it replies, or handling coding assignments where the program should be terminated when data arrives from the server, we offer expert guidance every step of the way.
We guarantee original solutions that meet rigorous academic standards, empowering students to excel in their programming coursework confidently. Partner with us to unlock exclusive features and enhance your understanding of reverse-echo-server and client assignments effectively. If you are wondering who will do my Reverse-Echo-Server and Client assignment? Trust our reliable service for expert guidance and support!
- Expert Guidance: Access dedicated reverse-echo-server and client assignment experts who specialize in network protocols and socket programming.
- Clarity in Concepts: Gain thorough understanding of complex topics such as error handling and data transmission crucial for academic success.
- Original Solutions: Receive original solutions that adhere to rigorous academic standards, ensuring high-quality submissions.
- Enhanced Learning: Partner with us to access exclusive features that facilitate effective learning and mastery of reverse-echo-server and client assignments.
- Reliable Support: Trust our reliable service for expert guidance and support throughout your academic journey in programming.
Easy Steps to Connect with Our Reverse-Echo-Server and Client Assignment Help Experts
Connecting with our Reverse-Echo-Server and Client assignment help experts at ProgrammingHomeworkHelp.com is simple. Begin by exploring our programming homework help services tailored for help with reverse-echo-server and client assignments. Engage with our dedicated reverse-echo-server and client assignment experts, who specialize in network protocols and socket programming.
Our efficient process ensures quick access to expert guidance, enabling clarity in reverse-echo-server and client assignments and fostering academic success. If you are wondering who will do my Reverse-Echo-Server and Client assignment? Join us for a seamless journey toward mastering these complex programming concepts effectively.
- Navigate to Our Platform: Access ProgrammingHomeworkHelp.com to discover our tailored programming homework help services.
- Choose Your Assignment: Select "Reverse-Echo-Server and Client assignment help" from our comprehensive list of specialized assistance.
- Place Your Order: Complete a simple order form, providing assignment details and specific requirements.
- Connect with Our Experts: Our team will match you with dedicated reverse-echo-server and client assignment specialists skilled in network protocols and socket programming.
- Receive Expert Guidance: Expect timely delivery of a meticulously crafted solution that enhances your grasp of complex programming concepts and boosts your academic performance.
Varied Topics Covered in Our Reverse-Echo-Server and Client Assignment Help Service
Explore the breadth of topics addressed through our Reverse-Echo-Server and Client assignment help service at ProgrammingHomeworkHelp.com. Our reverse-echo-server and client assignment experts cover a wide array of subjects related to network protocols and socket programming. Whether you need help with reverse-echo-server and client assignments involving error handling, data transmission, or advanced concepts, our service ensures comprehensive support.
If you're grappling with questions like who will do my Reverse-Echo-Server and Client assignment, look no further than our programming homework help service. We specialize in delivering comprehensive explanations and expert solutions that not only clarify complex topics but also boost your academic performance. Whether you need to stop the client program, program user inputs for the echo server and waits, or ensure the client program the user sends the message, our team is dedicated to helping you achieve success in your programming coursework. Partner with us today to navigate through diverse programming challenges effectively and excel in your studies.
- Socket Programming Basics: Learn fundamental concepts for establishing communication channels between networked devices in reverse-echo-server and client assignments.
- Error Handling Strategies: Explore techniques to manage and resolve errors encountered during reverse-echo-server and client operations effectively.
- Data Transmission Protocols: Understand protocols governing the orderly and efficient exchange of data between servers and clients in network programming tasks.
- Network Security Measures: Discover methods to ensure data integrity and confidentiality in reverse-echo-server and client applications.
- Concurrency in Servers: Master techniques for managing simultaneous client connections and data processing in server-side programming environments.
Expand Your Knowledge with Our Reverse-Echo-Server and Client Assignment Blogs
Expand your understanding with our Reverse-Echo-Server and Client assignment blogs. Designed to provide valuable insights and practical tips, our blogs help you navigate through challenges in reverse-echo-server and client assignments. Whether you need help with reverse-echo-server and client assignment concepts or seek guidance from a dedicated reverse-echo-server and client assignment helper, our informative content ensures clarity and enhances your skills. Stay updated and informed with our educational resources to excel in your programming journey.
Real Student Feedback on Our Reverse-Echo-Server and Client Assignment Help
Read genuine student feedback on how our Reverse-Echo-Server and Client assignment help has made a difference. Students appreciate our dedicated help with reverse-echo-server and client assignment tasks, highlighting the expertise of our reverse-echo-server and client assignment helper team. Discover firsthand experiences that showcase our commitment to clarity, reliability, and excellence in assisting students with their programming challenges.
Introducing Our Expert Reverse-Echo-Server and Client Assignment Help Team
Introducing our dedicated Reverse-Echo-Server and Client assignment help team—a group of experts ready to provide exceptional help with reverse-echo-server and client assignment tasks. Our team of reverse-echo-server and client assignment helpers brings extensive experience and deep knowledge to ensure your assignments are handled with precision and expertise. Count on us for reliable support, comprehensive understanding, and solutions that meet your academic needs effectively and efficiently.
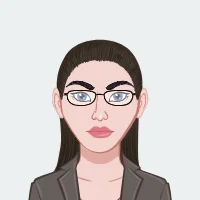
Eve Kemp
PhD in Programming
🇺🇸 United States
Eve Kemp is a computer science professional with extensive experience in software development and computational theory. Her expertise spans across pattern recognition, algorithm design, and software development, bringing a deep understanding to complex computational problems and practical solutions.
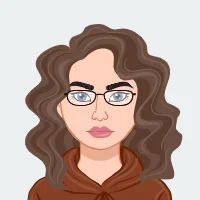
Sandra Alva
Masters in Programming
🇺🇸 United States
Sandra Alva is a software engineer with over 10 years of experience in reverse engineering and low-level programming, specializing in machine code analysis and instruction set architectures.
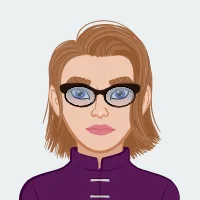
Josh Kaiser
Masters in Programming
🇺🇸 United States
Josh Kaiser is a computer architect with over 15 years of experience in optimizing CPU performance and pipeline design, specializing in data hazards, forwarding techniques, and advanced processor efficiency.
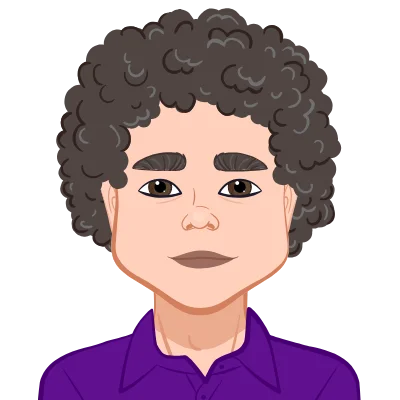
Andrew Bell
Ph.D. in Computer Science
🇨🇦 Canada
Andrew Bell, a seasoned Analog Electronics Assignment expert, holds a Ph.D. from the University of Ottawa, Canada. With 10 years of experience, he specializes in circuit analysis, transistor design, and amplifier optimization.
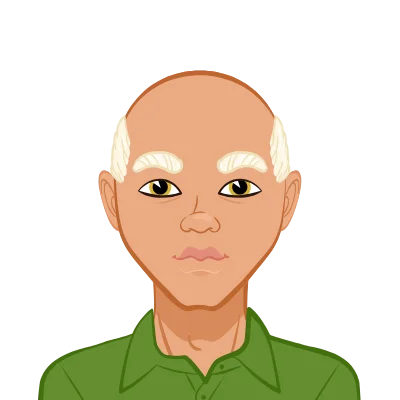
Adam Cook
Ph.D. in Computer Science
🇦🇺 Australia
Adam Cook, a seasoned Analog Electronics Assignment expert, holds a Ph.D. from Monash University, Australia. With 13 years of experience, he specializes in circuit analysis, transistor design, and amplifier optimization.
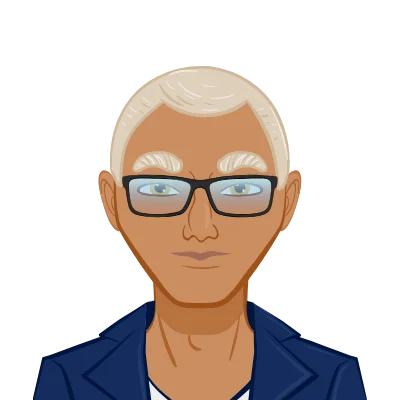
Zoe Carey
Ph.D. in Computer Science
🇬🇧 United Kingdom
Zoe Carey, a seasoned Analog Electronics Assignment expert, holds a Ph.D. from Imperial College London, UK. With 15 years of experience, she specializes in circuit analysis, transistor design, and amplifier optimization.
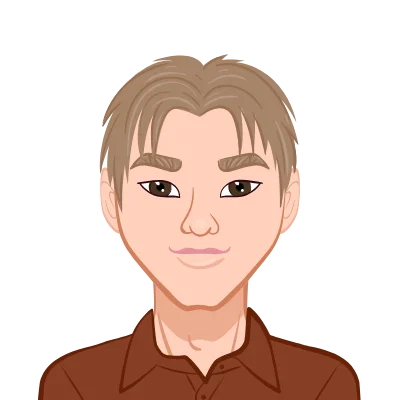
Noah Mellor
Ph.D. in Computer Science
🇺🇸 United States
Noah Mellor, a seasoned Analog Electronics Assignment expert, holds a Ph.D. from Columbia University, United States, and brings 18 years of comprehensive industry experience. His expertise spans circuit analysis, transistor design, and advanced amplifier configurations.
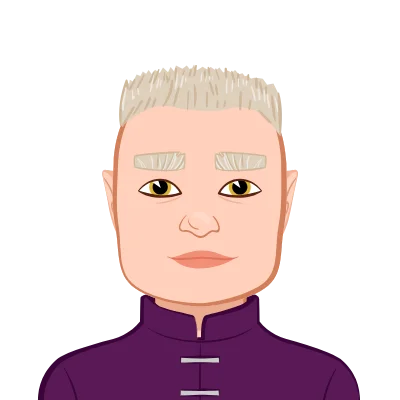
Lucas Chandler
Ph.D. in Computer Science
🇨🇦 Canada
Lucas Chandler, Ph.D., from the University of British Columbia, Canada, brings 10 years of expertise in Reverse-Echo-Server and Client Assignments. His academic rigor ensures high-quality solutions, reflecting his commitment to excellence and client satisfaction.
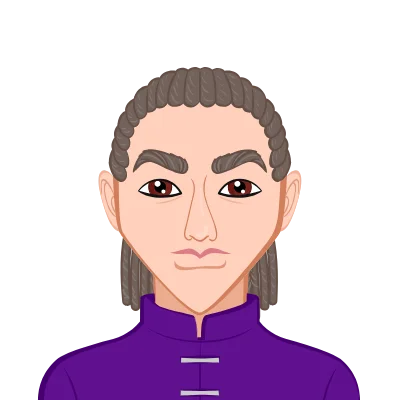
Aidan Dodd
Ph.D. in Computer Science
🇦🇺 Australia
Meet Aidan Dodd, a Ph.D. holder from the University of New South Wales, Australia, with 13 years of expertise in Reverse-Echo-Server and Client Assignments. Aidan delivers meticulous solutions, ensuring academic excellence and client satisfaction.
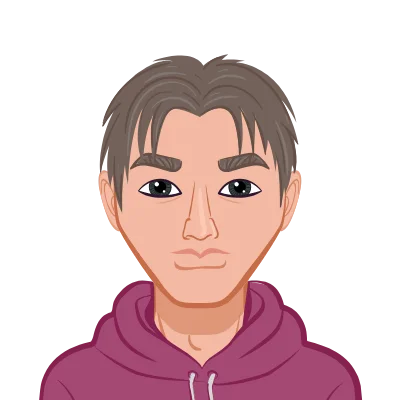
Ben Stone
Ph.D. in Computer Science
🇬🇧 United Kingdom
Meet Ben Stone, an esteemed expert in Reverse-Echo-Server and Client Assignments. Ben holds a Ph.D. from the University of Oxford, United Kingdom, and brings over 15 years of invaluable experience. His proficiency ensures top-tier solutions and academic excellence in every assignment.
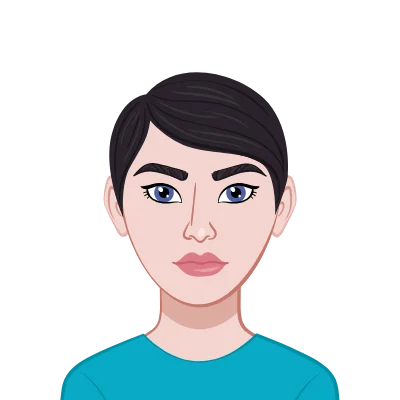
Courtney Sullivan
Ph.D. in Computer Science
🇺🇸 United States
Courtney Sullivan, Ph.D., brings 18 years of expertise in Reverse-Echo-Server and Client Assignments, graduating from Massachusetts Institute of Technology, United States. Her meticulous approach ensures impeccable solutions and academic success for students.
Deborah D. Noel
PhD in Computer Science
🇸🇬 Singapore
Deborah D. Noel, from Nanyang Technological University in Singapore, holds a PhD in computer science and 5 years of experience. Having completed 550 assignments, Noel's expertise lies at the intersection of data science and computational biology, where her research has yielded transformative insights into complex biological systems.
Jane C. Harris
PhD in Computer Science
🇺🇸 United States
Jane C. Harris, representing the University of Illinois in the United States, combines 9 years of experience with 756 completed assignments. Her focus on software engineering and human-computer interaction has led to groundbreaking research and innovation, setting new standards in the field of computer science.
Janice J. Seymore
PhD in Computer Science
🇬🇧 United Kingdom
Janice J. Seymore, a graduate of Carnegie Mellon University in Pittsburgh, Pennsylvania, brings 8 years of experience to the forefront of cybersecurity and data analytics. With 635 assignments under her belt, Seymore's expertise is unparalleled, making her a sought-after authority in digital security solutions.
Selma P. Davis
PhD in Computer Science
🇬🇧 United Kingdom
Selma P. Davis, a distinguished alumna of the University of Cambridge, England, holds a PhD in computer science and boasts 11 years of experience. With a remarkable track record of completing 900 assignments, her expertise lies in the realms of artificial intelligence and machine learning. Davis's contributions to the field have reshaped technological landscapes, earning her global recognition as a trailblazer in computational research.
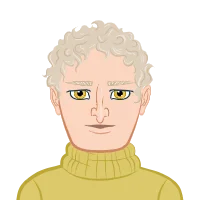
Dr. Arthur M. Garwood
PhD in Computer Science from the University of York
🇬🇧 United Kingdom
Dr. Arthur M. Garwood holds a PhD in Computer Science from the University of York and boasts over 8 years of experience in the field. With an impressive track record of completing over 600 Data Structures and Analysis of Algorithms Assignments, Dr. Garwood brings a wealth of expertise and academic rigor to his work. Known for his innovative approach and commitment to excellence, he ensures each assignment meets high standards of quality and clarity, empowering students to succeed in their studies.
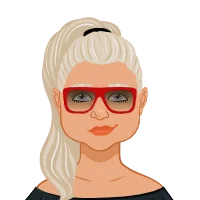
Dr. Carrie S. Benoit
PhD in Computer Science from the University of Texas
🇺🇸 United States
Dr. Carrie S. Benoit is a seasoned expert with a PhD in Computer Science from the University of Texas and more than 7 years of experience specializing in Data Structures and Analysis of Algorithms. Having successfully completed over 500 assignments, Dr. Benoit is known for her thorough approach and attention to detail. She leverages her academic background and practical experience to provide students with insightful solutions that foster learning and academic growth.
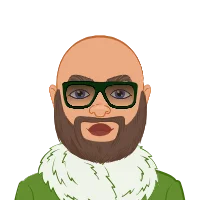
Dr. Wayne D
PhD in Computer Science from the University of Melbourne
🇺🇸 United States
Hedrick: Dr. Wayne D. Hedrick earned his PhD in Computer Science from the University of Melbourne and has over 6 years of experience in academia and industry. With a portfolio of over 400 completed Data Structures and Analysis of Algorithms Assignments, he combines theoretical knowledge with practical insights to deliver comprehensive solutions.
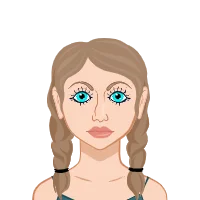
Dr. Crystal D. Hopkins
PhD in Computer Science from the University of Bristol
🇬🇧 United Kingdom
Dr. Crystal D. Hopkins holds a PhD in Computer Science from the University of Bristol and brings over 5 years of experience in the field. She has successfully completed over 300 Data Structures and Analysis of Algorithms Assignments, demonstrating her deep understanding and expertise in the subject. Driven by a passion for teaching and research, Dr. Hopkins ensures each assignment is meticulously crafted to meet academic standards and exceed student expectations.
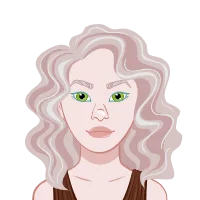
Dr. Annette J. Brown
PhD
🇬🇧 United Kingdom
Dr. Annette J. Brown holds a PhD from the University of Oxford and boasts over a decade of experience in Computer Science and AWS assignments. With a remarkable portfolio of over 1000 successfully completed assignments, Dr. Brown is renowned for her deep expertise, meticulous approach, and commitment to delivering exceptional Amazon Web Services solutions that empower student success.
Related Topics
Frequently Asked Questions (FAQs)
Explore our Frequently Asked Questions (FAQs) to get quick answers about our Reverse-Echo-Server and Client assignment help services. Find detailed information on how we provide help with reverse-echo-server and client assignment tasks, the expertise of our reverse-echo-server and client assignment helpers, and what sets us apart in delivering reliable academic support. Whether you're curious about our process, pricing, or service guarantees, our FAQs are designed to address your queries comprehensively and efficiently.
We provide revisions to ensure your satisfaction. Simply let us know the changes you require, and our experts will address them promptly.
The turnaround time depends on the complexity of the assignment. We strive to deliver solutions promptly while ensuring quality and accuracy.
Absolutely! Our experts are proficient in both server-side and client-side programming, ensuring comprehensive assistance for your assignments.
Yes, we guarantee originality in all solutions. Our experts ensure that each assignment is crafted from scratch to meet your specific requirements.
Our helpers have advanced degrees in computer science from reputable universities and years of experience handling complex server-client assignments.
You can get help by contacting our team of experienced Reverse-Echo-Server and Client assignment helpers who provide personalized assistance tailored to your assignment requirements.