Claim Your Offer
Unlock an amazing offer at www.programminghomeworkhelp.com with our latest promotion. Get an incredible 20% off on your second programming assignment, ensuring top-quality assistance at an affordable price. Our team of expert programmers is here to help you, making your academic journey smoother and more cost-effective. Don't miss this chance to improve your skills and save on your studies. Take advantage of our offer now and secure exceptional help for your programming assignments.
We Accept
Discover Java Arithmetic Assignment Samples
Explore the quality and depth of our Java Arithmetic Operators assignment help with our carefully curated samples. Each sample, crafted by our experienced programming experts, showcases our ability to tackle various topics from basic arithmetic to bitwise operations. Review these examples to see how our Programming Homework Help service can answer the question, "Who will do my Java Arithmetic Operators assignment?", and elevate your understanding and grades.
Java
Affordable Pricing for Top-Quality Java Arithmetic Operators Assignment Help
At ProgrammingHomeworkHelp.com, we offer competitive and transparent pricing for expert help with Java Arithmetic Operators assignments. Our affordable plans ensure you receive quality assistance without breaking the bank. Get personalized support tailored to your budget and academic needs. Contact us today to learn more about our pricing options and start your journey towards academic success!
Topics Covered | Price (USD) | Expected Completion Time |
---|---|---|
Basic Arithmetic Operations | $20 | 1-2 days |
Mixed Arithmetic Operations | $30 | 2-3 days |
Modulus and Exponential Operations | $40 | 2-3 days |
Compound Assignment Operators | $45 | 2-4 days |
Bitwise Arithmetic Operations | $50 | 3-5 days |
Integration with Java Control Structures | $60 | 4-6 days |
Customized Assignment Requirements | Contact for Quote | Varies based on complexity |
- Java Arithmetic Operators Assignment Help
- Illustrating Java Arithmetic Operations
- What Sets Our Java Arithmetic Operators Help Service Apart?
- Comprehensive Coverage of Topics by Our Java Arithmetic Operators Assignments Experts
- Plagiarism-Free Java Arithmetic Operators Assignment Help
- Claim 20% Discount on Our Java Arithmetic Operators Assignments Help Service
Java Arithmetic Operators Assignment Help
Struggling with your Java Arithmetic assignments? At ProgrammingHomeworkHelp.com, we specialize in providing help with Java Arithmetic Operators assignments. Our team of experienced Java developers and tutors is dedicated to helping you master every facet of coding assignment. Whether you're tackling basic arithmetic tasks or delving into more complex topics like modulus and bitwise operations, count on our Java Arithmetic Operators assignment help service to guide you towards academic excellence.
When you choose our Java Arithmetic Operators assignment help service, you gain access to dedicated experts who are well-versed in Java programming concepts. Whether you need help with basic arithmetic operations like addition, subtraction, multiplication, and division, or more complex tasks involving modulus and bitwise operations, our skilled professionals are ready to assist you.
Why struggle with simple assignments operator alone when you can have the support of our qualified team? Our commitment is to deliver accurate solutions tailored to your specific requirements, ensuring you not only complete your assignments on time but also gain a deeper understanding of Java arithmetic concepts. Don’t hesitate to reach out—let our Java Arithmetic Operators assignment experts guide you towards academic success!
Illustrating Java Arithmetic Operations
public class ArithmeticOperationsExample {
public static void main(String[] args) {
int num1 = 20;
int num2 = 5;
// Addition
int sum = num1 + num2;
System.out.println("Sum: " + sum);
// Subtraction
int difference = num1 - num2;
System.out.println("Difference: " + difference);
// Multiplication
int product = num1 * num2;
System.out.println("Product: " + product);
// Division
int quotient = num1 / num2;
System.out.println("Quotient: " + quotient);
// Modulus
int remainder = num1 % num2;
System.out.println("Remainder: " + remainder);
}
}
In this example:
- We declare two integers num1 and num2.
- We demonstrate basic arithmetic operations such as addition (+), subtraction (-), multiplication (*), division (/), and modulus (%).
- Each operation result is printed to the console using System.out.println().
What Sets Our Java Arithmetic Operators Help Service Apart?
Are you wondering, who will do my Java Arithmetic Operators assignment effectively? At ProgrammingHomeworkHelp.com, we are your trusted assignment experts dedicated to delivering top-notch Java Arithmetic Operators assignment help. Here are the key features of our service:
- Experienced Tutors: Our team comprises seasoned Java Arithmetic Operators assignment experts with extensive knowledge and practical skills in Java programming. They are adept at handling assignments of varying complexities.
- Customized Solutions: We offer tailored solutions that cater to your specific requirements for Java assignments. Whether its basic arithmetic operations or advanced concepts like modulus and bitwise operations, we ensure thorough understanding and precise solutions.
- Timely Delivery: We understand the importance of deadlines. Our experts work diligently to deliver your assignments on time, ensuring you meet academic deadlines without compromise.
- Comprehensive Guidance: Beyond solving assignments, we provide comprehensive guidance to enhance your understanding of Java arithmetic concepts. Our tutors offer detailed explanations and step-by-step solutions to foster learning.
- 24/7 Availability: Need urgent help? We're available round-the-clock to assist you. Contact us anytime for immediate help with Java Arithmetic Operators assignments.
- Affordable Pricing: Our services come at competitive rates designed to fit student budgets. Get value for your money with transparent pricing and no hidden costs.
Comprehensive Coverage of Topics by Our Java Arithmetic Operators Assignments Experts
We pride ourselves on delivering complete and thorough assistance at ProgrammingHomeworkHelp.com you need to excel. Our Java Arithmetic Operators assignments experts handle every aspect of your assignment, ensuring detailed solutions and explanations for all topics involved. Here’s how our team supports you across various topics:
- Basic Arithmetic Operations: Our team completes the entire assignment by performing addition, subtraction using the subtraction operator, multiplication, and division operations precisely, ensuring you grasp the foundational concepts effortlessly.
- Mixed Arithmetic Operations: Our experts handle assignments that involve combined arithmetic operations, such as solving complex expressions that use multiple operators, including the increment operator, part of a larger expression, delivering a comprehensive solution tailored to your needs.
- Modulus Operations: We handle assignments involving modulus operations, providing clear explanations and accurate results to help you understand how to determine remainders in division problems.
- Exponential Operations: Our helpers complete the entire assignment by managing tasks that include exponential calculations, guiding you through the process of handling power and exponential functions in Java.
- Compound Assignment Operators: We tackle assignments involving compound operators like +=, -=, *=, /=, and %=, offering step-by-step solutions to ensure you understand how these operators work in simplifying expressions.
- Bitwise Arithmetic Operations: Our experts manage bitwise operations, including AND, OR, XOR, and bit shifts, delivering clear and concise solutions that help you master these advanced arithmetic operations.
- Integration with Java Control Structures: We complete assignments that integrate arithmetic operations within loops, conditionals, and other control structures, ensuring you can apply arithmetic operations effectively within broader Java programming contexts.
Plagiarism-Free Java Arithmetic Operators Assignment Help
Concerned about originality and academic integrity while seeking programming homework help? In our Java Arithmetic Operators Assignment Help service, we ensure that every solution we provide is 100% plagiarism-free. Our dedicated Java Arithmetic Operators assignment experts craft each assignment from scratch, adhering to strict guidelines to maintain academic integrity. When you ask, "Who will do my Java Arithmetic Operators assignment?” rest assured that our experts will deliver unique and original content, tailored specifically to your requirements.
We use advanced plagiarism detection tools to verify the originality of every assignment before delivery. This commitment guarantees that you receive authentic help with Java Arithmetic assignment without any risk of academic misconduct. Trust our expertise for high-quality; plagiarism-free solutions that not only meet your academic standards but also contribute to your learning and success.
Claim 20% Discount on Our Java Arithmetic Operators Assignments Help Service
We appreciate our returning students and are thrilled to offer a 20% discount on our Java Arithmetic Operators assignment help service. If you’ve used our services before, you can now enjoy this special offer on your next assignment. Whether you're working on a new topic or revisiting a familiar one, our programming assignment experts will handle your entire assignment, delivering comprehensive solutions tailored to your needs. Simply provide your previous assignment or account details when you contact us and the discount will be applied to your new order.
Our Java Arithmetic Operators assignment experts are dedicated to providing you with comprehensive assistance on all topics, including basic arithmetic operations, bitwise arithmetic, and compound assignments. If you’ve ever wondered, “Who will do my programming assignment? now is the perfect time to benefit from our Programming Homework Help service while saving on costs. Don’t miss out on this opportunity to excel in your assignments with the guidance of our support team and enjoy the exclusive discount tailored just for you!
Stay Updated with Our Blog
Dive into a wealth of resources, insights, and tips designed to enhance your understanding of Java Arithmetic Operators and programming concepts. Our expert-authored articles cover a range of topics, from basic arithmetic operations to advanced Java programming techniques. Whether you're a student seeking assignment tips or a programming enthusiast looking to expand your knowledge, our blog is here to support your learning journey. Stays tuned for regular updates and enrich your programming skills with us!
Student Testimonials on Our Java Arithmetic Operators Assignment Help
Our student’s feedback speaks volumes about the quality of our Java Arithmetic Operators assignment help service. From precise solutions to timely delivery, our experts consistently receive high praise for their exceptional support. Check out the reviews below to see how we’ve helped students excel in their assignments and gain a better understanding of Java arithmetic concepts. Visit ProgrammingHomeworkHelp.com to learn more.
Introducing Our Java Arithmetic Operators Assignment Experts
Our team of Java Arithmetic Operators assignment experts is the backbone of our service. With extensive experience in Java programming and a deep understanding of arithmetic operations, our experts are equipped to handle any assignment with precision and clarity. Trust our experts to deliver exceptional results, and see the difference in your understanding and grades.
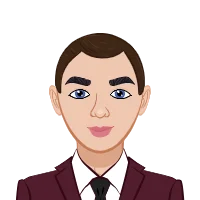
Nathan Patel
Masters in Java
🇦🇺 Australia
Nathan Patel is an experienced Java programming tutor with over 8 years of expertise. He holds a Master's degree from the University of Queensland, Australia, specializing in guiding students through advanced programming concepts and assignments.
Related Topics
Frequently Asked Questions
Find answers to common queries about our Java Arithmetic Operators assignment help service at ProgrammingHomeworkHelp.com. Our FAQs cover everything from how to place an order and our pricing structure to how our experts handle assignments. Get clarity on your concerns and make informed decisions with our comprehensive FAQs.