Simulating Wormhole Travel with Java Multithreading and Synchronization in Java

Wormholes, those captivating theoretical shortcuts through the fabric of spacetime, have long held the fascination of both scientific minds and science fiction enthusiasts. Despite their intriguing potential, the actualization of wormhole travel remains firmly entrenched within the realm of scientific theory. However, within the realm of computer science, we can embark on an exciting journey of simulation. In the following discussion, we will delve into the intricacies of simulating individuals as they traverse these cosmic conduits using the power of multiple threads and synchronization in the Java programming language.
This immersive undertaking promises to be a valuable educational experience for students seeking to deepen their understanding of multithreading and synchronization in the context of Java. It also offers a practical outlet for honing their programming skills and tackling complex assignments. As parallel computing and simulations become increasingly essential in various fields, this project serves as a bridge between theory and application, offering hands-on experience that can prove invaluable in a student's academic journey. So, join us as we embark on this journey through the digital wormhole, where you'll not only explore the mysteries of space-time but also sharpen your Java programming skills, all while preparing to excel in writing your Multithreading and Synchronization assignment using Java assignment in the realm of parallel computing and simulations.
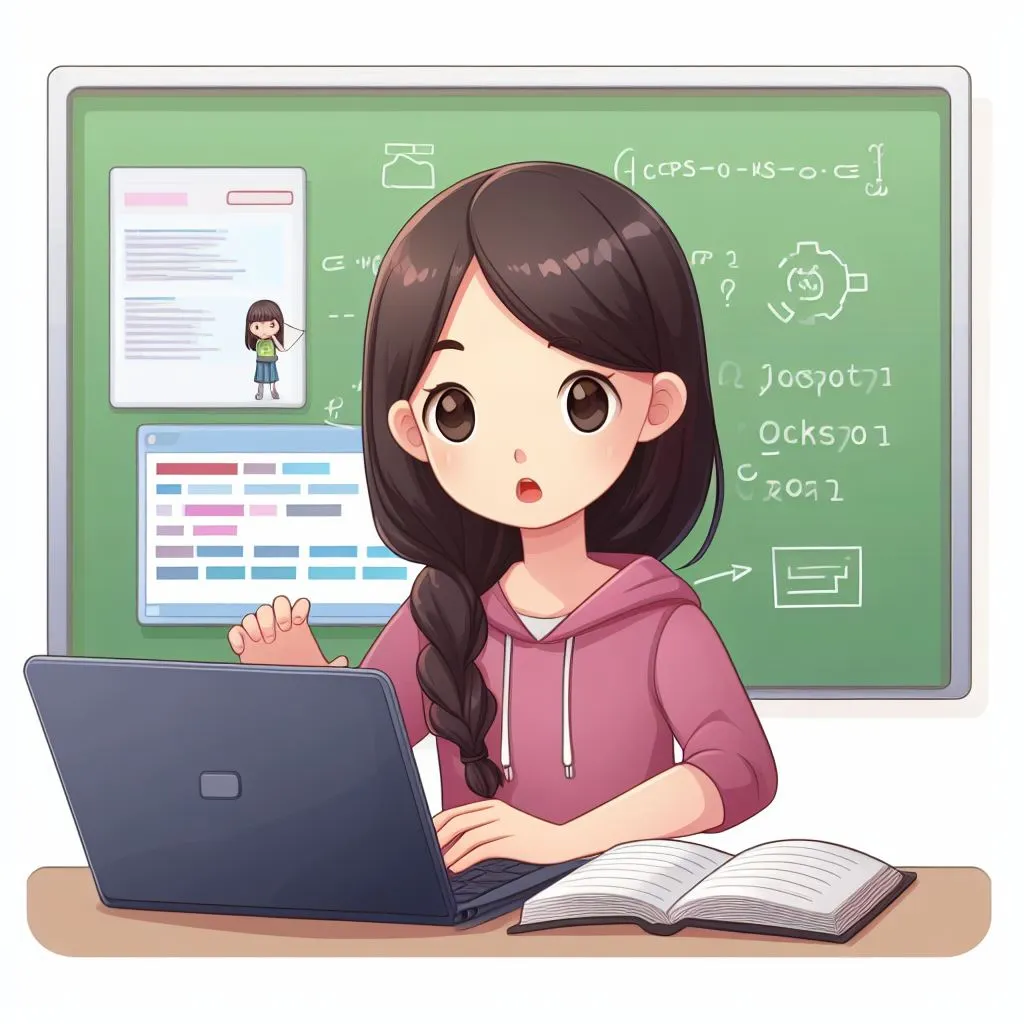
Understanding Multithreading and Synchronization
Before embarking on our fascinating journey of simulating wormhole travel, it's essential to grasp the fundamental principles of multithreading and synchronization in Java. These concepts form the bedrock of our simulation, allowing us to orchestrate the smooth and concurrent movement of travelers through the intricate fabric of our wormhole. Multithreading, the art of executing multiple threads simultaneously, enables us to simulate the presence of numerous travelers within the same space. On the other hand, synchronization steps in as the conductor of this intricate symphony, ensuring that each traveler enters and exits the wormhole in an orderly and synchronized manner. By delving into the nuances of multithreading and synchronization, we lay the groundwork for a simulation that not only captures our imagination but also instills practical knowledge that can be applied to a wide array of parallel computing and simulation scenarios.
- Multithreading: Multithreading is a powerful concept that revolutionizes program execution. By permitting the concurrent execution of multiple threads, it unleashes the true potential of modern computing, particularly on multi-core processors. Threads, essentially sub-processes within a program, share the same memory space. While this sharing enhances efficiency, it also presents challenges, particularly in scenarios where multiple threads access and modify shared data concurrently. This phenomenon, known as a race condition, can lead to unpredictable and erroneous program behavior. Fortunately, this is where synchronization emerges as a crucial player in multithreading. It introduces mechanisms to orchestrate thread execution, ensuring that multiple threads can work harmoniously without stepping on each other's toes. By understanding and skillfully implementing synchronization, developers can eliminate race conditions, thereby enhancing program reliability and performance.
- Synchronization: Synchronization is the linchpin of multithreaded programming. It serves as a guardian, ensuring that only one thread can access a specific block of code or a shared resource at any given time. In the world of Java, synchronization is facilitated through the synchronized keyword and various synchronization constructs like locks. These mechanisms grant exclusive access to the synchronized code, preventing multiple threads from entering simultaneously and potentially corrupting shared data. In essence, synchronization acts as a traffic cop, directing the flow of thread execution in an orderly and controlled manner. Without it, multithreaded programs could spiral into chaos, leading to data inconsistencies, crashes, and a nightmare of debugging challenges. Therefore, a solid grasp of synchronization is imperative for any developer venturing into the realm of multithreaded programming in Java, ensuring the safe and predictable execution of concurrent tasks.
Now, let's apply these foundational concepts to our exciting wormhole simulation. As we embark on this journey, we'll bring the theory of multithreading and synchronization to life in a tangible and engaging manner.
Designing the Wormhole Simulation
Our objective is to simulate the fascinating experience of individuals venturing through a wormhole. To achieve this, we'll craft a straightforward yet comprehensive Java program that models three essential elements: the wormhole itself, the intrepid travelers who will navigate its depths, and a simulation engine to oversee and coordinate this intriguing journey.
- Wormhole: This element represents the entry and exit points of our wormhole, serving as the gateway to another dimension. It's the focal point of our simulation, and its proper management is vital to ensure the safety and orderliness of the travelers' passage.
- Travelers: These brave souls are the heart of our simulation, representing the people who will enter and exit the wormhole. Each traveler operates independently, and their interactions with the wormhole must be meticulously orchestrated to create a realistic and immersive experience.
- Simulation: Serving as the control center, the simulation component is responsible for managing the overall flow of our wormhole journey. It coordinates the activities of travelers, ensuring that they enter and exit the wormhole in a synchronized manner, all while maintaining the integrity of our simulation.
To accomplish this, we'll harness the robust multithreading and synchronization features offered by Java. These tools will enable us to create a seamless and safe passage for our travelers, making their adventure through the wormhole not only captivating but also a valuable learning experience for students exploring the world of concurrent programming and simulations.
Setting Up the Project
To embark on our wormhole simulation adventure, the first step is to establish a Java project with all the essential components in place. This foundation is crucial for a smooth and organized development process. Below, we outline the key classes required for our project:
- Wormhole: This class will serve as the core representation of the wormhole, encompassing its entry and exit points. To enable the orderly flow of travelers through the wormhole, this class should feature methods that allow travelers to enter and exit the wormhole safely.
- Traveler: The Traveler class is instrumental in our simulation. Each traveler represents an individual who will journey through the wormhole. To create a realistic and dynamic experience, we'll design each traveler as a separate thread. This class should include methods that facilitate a traveler's entry into and exit from the wormhole, ensuring that their interactions are synchronized and coherent.
- Simulation: Serving as the command center of our project, the Simulation class plays a pivotal role in orchestrating the entire simulation. It's responsible for creating and managing travelers, starting their threads, and overseeing the overall flow of the simulation. This class acts as the glue that binds our project together, ensuring a seamless and captivating wormhole travel experience for both developers and users alike.
By organizing our project in this manner, we set the stage for a well-structured and efficient development process. These foundational classes will not only bring our wormhole simulation to life but also provide an excellent learning opportunity for students exploring the intricate world of multithreading and synchronization in Java.
Coding the Wormhole Class
```java
public class Wormhole {
private boolean entryOccupied = false;
private boolean exitOccupied = false;
public synchronized void enterWormhole() {
while (entryOccupied || exitOccupied) {
try {
wait(); // Wait until the wormhole is clear
} catch (InterruptedException e) {
Thread.currentThread().interrupt();
}
}
entryOccupied = true;
}
public synchronized void exitWormhole() {
entryOccupied = false;
exitOccupied = false;
notifyAll(); // Notify waiting travelers that the wormhole is clear
}
}
```
Within the Wormhole class, our primary objective is to implement synchronization mechanisms that guarantee the orderly passage of travelers through the wormhole. This is achieved by utilizing Java's synchronization capabilities. Specifically, we ensure that only one traveler can enter or exit the wormhole at any given time. To accomplish this, we employ the wait() method, which temporarily halts travelers if the wormhole is already occupied. This synchronization technique is pivotal in maintaining the integrity and safety of our simulation, preventing clashes and ensuring that each traveler's journey proceeds smoothly.
```java
public class Traveler extends Thread {
private String name;
private Wormhole wormhole;
public Traveler(String name, Wormhole wormhole) {
this.name = name;
this.wormhole = wormhole;
}
@Override
public void run() {
System.out.println(name + " is ready to enter the wormhole.");
wormhole.enterWormhole();
System.out.println(name + " has entered the wormhole.");
try {
Thread.sleep(1000); // Simulate some time spent in the wormhole
} catch (InterruptedException e) {
Thread.currentThread().interrupt();
}
wormhole.exitWormhole();
System.out.println(name + " has exited the wormhole.");
}
}
```
The Traveler class takes center stage in our simulation. It extends the Thread class, allowing each traveler to operate as an independent thread. Every traveler possesses a reference to the wormhole, granting them access to the cosmic portal. Inside the run method, travelers undertake their journey: they enter the wormhole, experience a simulated passage of time within its enigmatic depths, and eventually emerge on the other side. Crucially, both the enterWormhole and exitWormhole methods are synchronized. This synchronization ensures that access to the wormhole remains secure and that only one traveler occupies it at any given moment. This careful orchestration of thread interactions guarantees the realism and reliability of our wormhole travel experience.
```java
public class Simulation {
public static void main(String[] args) {
Wormhole wormhole = new Wormhole();
for (int i = 1; i <= 5; i++) {
Traveler traveler = new Traveler("Traveler " + i, wormhole);
traveler.start();
}
}
}
```
In the Simulation class, we bring together all the elements we've meticulously designed and coded. Its primary role is to serve as the conductor of our wormhole travel symphony. This class orchestrates the entire simulation by creating a wormhole and generating multiple travelers, each representing an intrepid explorer. Subsequently, it kicks off their threads, setting the stage for the grand journey through the wormhole's enigmatic depths. The flexibility of this class allows you to adapt the simulation to your specific needs, enabling you to adjust the number of travelers according to your requirements and desired level of complexity.
Running the Simulation
With our Java program and simulation classes in place, it's time to bring our wormhole travel simulation to life. The next step involves compiling and running the program. When you execute the simulation, you'll witness the simulated travelers embarking on their extraordinary journeys into the wormhole's cosmic depths. The beauty of this exercise lies in the observable synchronization achieved through the Wormhole class. The output will reveal the order in which travelers enter and exit the wormhole, providing tangible evidence of the synchronization mechanisms at work. This visual representation not only showcases the success of our simulation but also serves as a valuable learning tool for comprehending the intricate world of multithreading and synchronization in Java.
Conclusion
In this blog, we've embarked on an intriguing journey into the realm of simulating wormhole travel using the power of multiple threads and synchronization in Java. This hands-on exercise not only ignites the imagination of students but also equips them with invaluable skills in multithreading and synchronization, the very foundations of parallel computing and simulations.
As students dive deeper into the world of concurrent programming, they can further enrich this simulation by introducing advanced features. These may include time tracking mechanisms to monitor how long travelers spend within the enigmatic wormhole, implementing constraints to limit the number of simultaneous travelers, or even designing a user-friendly interface for a more immersive experience.
This project serves as a remarkable Launchpad for students, propelling them into the captivating universe of parallel computing and simulations. It not only fosters a deeper understanding of these intricate concepts but also instills a sense of curiosity and fun in the world of wormhole travel, making learning a truly engaging adventure.