Claim Your Offer
Unlock an amazing offer at www.programminghomeworkhelp.com with our latest promotion. Get an incredible 10% off on your all programming assignment, ensuring top-quality assistance at an affordable price. Our team of expert programmers is here to help you, making your academic journey smoother and more cost-effective. Don't miss this chance to improve your skills and save on your studies. Take advantage of our offer now and secure exceptional help for your programming assignments.
We Accept
Browse Our Free Makefile & Modular Design Assignment Samples
In our sample section, you can view examples of Makefile & Modular Design assignments completed by our experts. These samples demonstrate the quality of our work and the level of expertise we bring to each task.
C
C
C
C
C
C
C
C
C
C
C
C
C
C
C
C
C
C
C
C
Get Affordable Makefile & Modular Design Assignment Writing Help with One Click
At ProgrammingHomeworkHelp.com, we prioritize affordability by offering customized rates for our Makefile & Modular Design assignment writing help. We understand the financial constraints students face, and our flexible pricing structure reflects our commitment to providing accessible assistance. Our prices are tailored to accommodate various budgets, with options to suit both simple and complex assignments. We ensure transparency by providing a detailed breakdown of our rates, allowing students to choose the option that best fits their needs. Additionally, our discounts and special offers further enhance affordability, making it easier for students to access top-quality assistance without breaking the bank.
Service Level | Price Range (USD) |
---|---|
Basic | $20 - $50 |
Standard | $40 - $80 |
Advanced | $60 - $120 |
Premium | $80 - $150 |
Customized Solutions | Custom Quote |
- Why Students Need Help with Makefile & Modular Design Assignments
- Hire Our Skilled Programmers Skilled in Makefile & Modular Design
- Can I Pay You to Do My Makefile & Modular Design Assignment On Advanced Topics?
- Exclusive Discounts that Comes with Our Online Makefile & Modular Design Homework Help Service
- How Our Makefile & Modular Design Assignment Helpers Complete Your Project with Excellence
- Benefits of Availing Our Makefile & Modular Design Assignment Help Online
Why Students Need Help with Makefile & Modular Design Assignments
Makefile and modular design are crucial aspects of software development, but many students find them challenging. The complexity of understanding how to automate build processes and break down a project into manageable modules can be overwhelming. This often leads to difficulties in completing assignments effectively and on time. Here are some common reasons why students seek help with Makefile & Modular Design assignments.
- Lack of Understanding
Many students struggle with the foundational concepts of Makefile and modular design. They may not fully grasp how Makefiles work to automate the build process or how to properly structure code into modules. This lack of understanding can result in incomplete or incorrect assignments.
- Time Constraints
Balancing coursework with other responsibilities can be difficult, leaving students with limited time to dedicate to complex homework tasks. Makefile and modular design assignments often require significant time and effort, making it hard for students to complete them without assistance.
- Debugging Issues
Makefiles and modular code can introduce various bugs and errors that are difficult to identify and fix. Debugging these issues requires a deep understanding of the build process and the relationships between different modules, which many students lack. This can lead to frustration and a need for expert help.
- Practical Application
Applying theoretical knowledge to practical projects is a common hurdle. Students may understand the concepts of Makefile and modular design in a classroom setting but struggle to apply them to real-world scenarios. This gap between theory and practice often necessitates external help to bridge.
- Achieving High Grades
Students aiming for high grades often seek help to ensure their assignments meet the highest standards. Professional assistance can provide expertly crafted solutions, detailed explanations, and a polished final product, which can significantly boost a student's grades.
By seeking help with Makefile & Modular Design assignments, students can overcome these challenges, enhance their understanding, and achieve better academic outcomes.
Hire Our Skilled Programmers Skilled in Makefile & Modular Design
Makefile and modular design are essential components in the development of complex software projects. Makefiles automate the build process, ensuring that only the necessary parts of a project are recompiled, while modular design breaks a project into smaller, manageable, and reusable modules. This combination not only makes the development process more efficient but also enhances the maintainability and scalability of the codebase. Understanding the process involved can significantly improve your programming skills and project outcomes. Our programming homework helpers are familiar with the process of Makefile & modular design:
- Identify Modules: Start by breaking down your project into smaller, logical modules. Each module should represent a distinct functionality or a component of the project.
- Define Dependencies: Determine the dependencies between the modules. This involves identifying which modules rely on others to function correctly.
- Create Source Files: Write the source code for each module. Ensure that each module is contained within its own set of files to maintain a clean and organized structure.
- Write a Makefile: Create a Makefile that defines how to compile and link the modules. The Makefile should include rules that specify the dependencies and the commands to build each module.
- Set Up Targets: Define targets in the Makefile for building the complete project and for individual modules. Targets can include actions like compiling source files, linking object files, and cleaning up build artifacts.
- Specify Rules and Macros: Use rules to specify how to build each target, including the source files, object files, and the commands to compile them. Utilize macros to simplify the Makefile and make it more readable and maintainable.
- Test and Debug: Run the Makefile to compile the project. Test each module individually and the complete project to ensure everything works as expected. Debug any issues that arise during the build process.
- Optimize: Optimize the Makefile and modular design for efficiency. This can include improving compilation times, reducing dependencies, and refactoring code to enhance modularity.
Example Code
Below is an example demonstrating a simple modular project with two modules: math and main. The math module contains basic mathematical operations, and the main module uses these operations.
Directory Structure:
project/
├── Makefile
├── main.c
├── math.c
└── math.h
Math.h
#ifndef MATH_H
#define MATH_H
int add(int a, int b);
int subtract(int a, int b);
#endif // MATH_H
Math.C
#include "math.h"
int add(int a, int b) {
return a + b;
}
int subtract(int a, int b) {
return a - b;
}
Main.C
#include <stdio.h>
#include "math.h"
int main() {
int x = 5, y = 3;
printf("Addition: %d\n", add(x, y));
printf("Subtraction: %d\n", subtract(x, y));
return 0;
}
Makefile
# Compiler and flags
CC = gcc
CFLAGS = -Wall -Werror -g
# Source files
SRCS = main.c math.c
# Object files
OBJS = $(SRCS:.c=.o)
# Executable name
EXEC = project
# Default target
all: $(EXEC)
# Link object files to create executable
$(EXEC): $(OBJS)
$(CC) $(CFLAGS) -o $@ $^
# Compile source files to object files
%.o: %.c
$(CC) $(CFLAGS) -c $< -o $@
# Clean target
clean:
rm -f $(OBJS) $(EXEC)
.PHONY: all clean
In this example:
- The math module (math.c and math.h) contains functions for addition and subtraction.
- The main module (main.c) uses these functions to perform calculations and print the results.
- The Makefile defines how to compile the project, specifying dependencies and rules for building the executable and cleaning up.
Can I Pay You to Do My Makefile & Modular Design Assignment On Advanced Topics?
At ProgrammingHomeworkHelp.com, we specialize in a wide range of topics within Makefile and modular design. Our expertise ensures that students receive high-quality, tailored assistance that meets their academic needs. If you’ve decided, “I want to pay someone to do my Makefile & Modular Design assignments,” here are some of the topics we excel in:
- Basic Makefile Syntax and Structure
Understanding the basic syntax and structure of a Makefile is crucial for automating the build process. We craft homework solutions that clearly explain the components of a Makefile, including targets, dependencies, and rules, ensuring students grasp the fundamentals effectively.
- Managing Dependencies
Handling dependencies correctly is essential to avoid unnecessary recompilation. Our experts excel in creating homework assignments that demonstrate how to manage dependencies efficiently using Makefile, ensuring that students learn to streamline their build processes.
- Modular Programming Concepts
Breaking a project into reusable modules can be complex. We offer comprehensive homework help that illustrates modular programming concepts, showing students how to design and implement modular code effectively in their assignments.
- Advanced Makefile Features
Makefile supports various advanced features like pattern rules, automatic variables, and functions. Our team provides detailed homework solutions that explore these advanced features, helping students leverage them to optimize their build systems.
- Debugging Makefiles
Debugging Makefiles can be challenging due to the complexity of the build process. We provide expert guidance on homework assignments focused on identifying and resolving common issues in Makefiles, enhancing students' debugging skills.
- Cross-Platform Makefile Creation
Creating Makefiles that work across different operating systems requires careful consideration. Our homework help covers cross-platform Makefile creation, ensuring students can develop portable build scripts suitable for various environments.
- Integrating External Libraries
Incorporating external libraries into projects using Makefiles can be tricky. We excel in crafting homework assignments that guide students through the process of integrating and managing external libraries, ensuring their projects are robust and functional.
- Optimizing Build Performance
Improving the performance of the build process is key to efficient software development. Our experts provide homework solutions that teach students how to optimize their Makefiles for faster and more efficient builds, enhancing their overall programming skills.
By choosing to trust us with their “do my Makefile & Modular Design homework”, students receive top-notch assistance across these critical topics, ensuring their academic success and a deeper understanding of these essential programming concepts.
Exclusive Discounts that Comes with Our Online Makefile & Modular Design Homework Help Service
At Programming Homework Help, we understand the financial constraints students often face. To make our top-notch Makefile & Modular Design homework assistance even more accessible, we offer a variety of exclusive discounts. Here are some of the ways you can save when you choose our services:
- 20% Discount on Your Second Order
We appreciate your loyalty. To thank you for returning to us, we offer a 20% discount on your second Makefile & Modular Design homework order. Enjoy significant savings as you continue to benefit from our expert assistance.
- Bulk Orders Discount
Have multiple assignments or a large project? We offer special bulk order discounts. The more homework you submit at once, the more you save. This is ideal for end-of-semester rushes or large-scale programming projects.
- Seasonal Discounts
Keep an eye out for our seasonal discounts. During certain times of the year, such as back-to-school season or holidays, we offer additional discounts on our Makefile & Modular Design homework help. Take advantage of these deals to get top-quality assistance at reduced rates.
By availing our help with Makefile & Modular Design programming homework, not only do you receive expert guidance and high-quality solutions, but you also benefit from these fantastic discounts, making it easier to achieve academic success without breaking the bank.
How Our Makefile & Modular Design Assignment Helpers Complete Your Project with Excellence
At Programming Homework Help, our Makefile & Modular Design assignment helpers are dedicated to delivering exceptional, customized solutions for your assignments. Our programming experts follow a meticulous process to ensure that each task is completed accurately and efficiently. Here’s an overview of how our experts handle your Makefile & Modular Design assignments:
- Understanding Assignment Requirements
The first step in our process is to thoroughly understand the assignment requirements. Our Makefile & Modular Design homework helpers carefully review the assignment brief, instructions, and any additional materials provided. This ensures that they grasp the objectives and specific needs of the task.
- Planning and Research
Once the requirements are clear, our programming experts conduct detailed planning and research. They outline the structure of the Makefile and the modular design, identifying the key components and dependencies. This phase involves gathering relevant information and determining the best approach to solve the problem.
- Writing Custom Code
With a solid plan in place, our experts begin writing the code from scratch. They implement the Makefile and modular design according to the assignment specifications, ensuring that the code is clean, efficient, and well-documented. Our experts use best practices in coding to create robust and maintainable solutions.
- Testing and Debugging
After the initial code is written, it undergoes rigorous testing and debugging. Our Makefile & Modular Design homework helpers test the code to ensure it works as intended and meets all the requirements. They identify and fix any bugs or issues, refining the solution for optimal performance.
- Reviewing and Proofreading
The final step involves a thorough review and proofreading of the entire assignment. Our programming experts check for accuracy, completeness, and adherence to the assignment criteria. They also ensure that the code is free of errors and is easy to understand.
- Delivering the Solution
Once the assignment is finalized, we deliver the completed solution to the student. Our Makefile & Modular Design homework helpers provide detailed explanations and documentation to help students understand the solution. We also offer support for any follow-up questions or clarifications.
By following this comprehensive process, our programming experts ensure that your Makefile & Modular Design homework is completed to the highest standards, providing you with a thorough and well-crafted solution.
Benefits of Availing Our Makefile & Modular Design Assignment Help Online
When you choose our Makefile & Modular Design assignment help online, you gain access to a range of benefits that can enhance your learning experience and academic success. Our programming experts are dedicated to providing top-notch assistance tailored to your specific needs. Here are some of the key benefits of availing our services:
- Get help from highly qualified experts
- Well-commented and running codes
- Prompt completion of all orders
- 24x7 customer support
- Affordable rates
Tips & Insights into the Best Practices of Makefile & Modular Design
Explore our blog section for insightful articles, tutorials, and tips related to Makefile & Modular Design and programming in general. Our blog features informative content curated by our experts to help you deepen your understanding of key concepts, stay updated on industry trends, and enhance your programming skills. Whether you're a beginner or an experienced programmer, our blog has something for everyone.
Reviews & Testimonials Shared by Students Who Have Used Our Reliable Service
In our review section, you'll find feedback from students who have availed our Makefile & Modular Design assignment help. These authentic reviews reflect the satisfaction and success of our clients, highlighting our commitment to excellence and customer satisfaction. We take pride in the positive feedback we receive and continuously strive to exceed expectations.
Meet Our Experienced & Highly Qualified Makefile & Modular Design Assignment Experts
Our team of experts comprises seasoned programmers and professionals with extensive experience in Makefile & Modular Design. With a deep understanding of programming concepts and practical application, our experts are well-equipped to provide top-notch assistance tailored to your specific needs. They are committed to delivering high-quality solutions and ensuring your academic success in Makefile & Modular Design assignments.
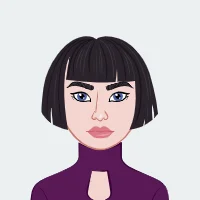
Camila Perry
PhD in Programming
🇺🇸 United States
Camila Perry, an experienced programmer with a degree from the Princeton University, specializes in teaching efficient memory management and advanced C programming techniques.
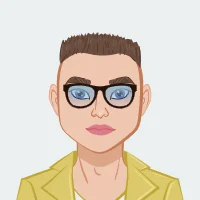
Andrew Reisinger
PhD in Programming
🇺🇸 United States
Andrew Reisinger is a seasoned C Programming Assignment Expert with over a decade of experience. Specializing in data structures, algorithms, and embedded systems, he helps students and professionals tackle complex C programming challenges. John is known for his clear explanations, strong problem-solving skills, and dedication to student success.
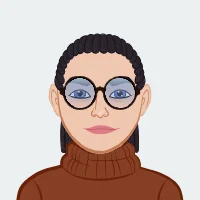
Philip Dudley
PhD in Programming
🇺🇸 United States
Philip Dudley is a seasoned software engineer with over 10 years of experience specializing in C programming and systems-level development. He excels in file descriptor management, memory allocation, and performance optimization. Philip has a strong track record of mentoring students and professionals, helping them master complex programming concepts and improve their coding practices for robust software solutions.
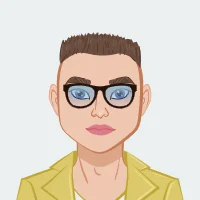
James Maker
Masters in Programming
🇺🇸 United States
James Maker is a seasoned C Programming Assignment Expert with over a decade of experience. Specializing in linked lists, file I/O, and bitwise operations, Alex provides expert guidance and solutions. With a strong background in computer science, Alex helps students master complex assignments and optimize their coding skills.
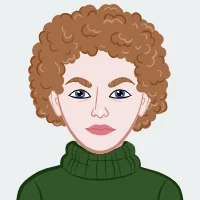
Andrew Hicks
Masters in Computer Science
🇺🇸 United States
Andrew Hicks, a seasoned software engineer, specializes in high-performance systems and memory management with over 15 years of experience.
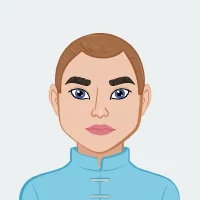
Robert Horta
Masters in C
🇬🇧 United Kingdom
Alex Johnson is a seasoned C programming expert with over a decade of experience. He has helped over 1,000 students excel in their C assignments through personalized tutoring and clear, efficient code solutions. Alex holds advanced degrees in computer science and has received multiple awards for his exceptional teaching and programming skills.
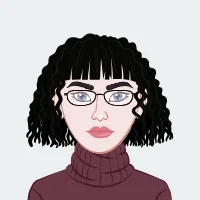
Elijah Patel
Masters in C
🇦🇺 Australia
Elijah Patel is a seasoned C assignment help Expert with 12 years of experience. Holding a Master's degree from the University of New South Wales, Australia.
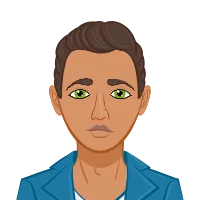
Damien Carrick
Bachelor of Science degree in Computer Science
🇬🇧 United Kingdom
Damien holds a Bachelor of Science degree in Computer Science and specializes in data structures and algorithms for game programming in C. With over 900 completed orders, he is proficient in designing efficient and scalable solutions for game simulations.

Isabella Ruiz
Bachelor of Engineering
🇺🇸 United States
Isabella Ruiz is a Bachelor of Engineering graduate. She is skilled in physics simulation and collision detection for game development in C. With over 600 completed orders, she specializes in creating realistic and immersive gaming experiences.
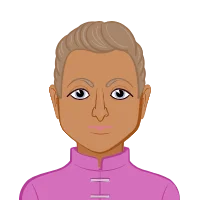
Jake Walker
Master's degree in Software Engineering
🇬🇧 United Kingdom
Jake Walker, with a Master's degree in Software Engineering, is adept at implementing efficient game loops and handling user inputs in C. Having completed over 700 orders, he excels in optimizing performance and debugging C game simulations.
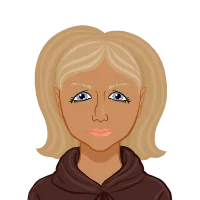
Dr. Miriam Goldbridge
Ph.D. in Computer Science
🇬🇧 United Kingdom
Dr. Miriam Goldbridge holds a Ph.D. in Computer Science and specializes in game development using C programming. With over 800 completed orders, she has extensive experience in crafting complex game simulations with a focus on AI algorithms and graphics rendering.
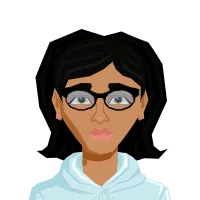
Prof. Rachel Anderson
M.Sc. in Software Engineering
🇨🇦 Canada
Prof. Rachel Anderson holds an M.Sc. in Software Engineering from the University of Toronto and has completed over 600 assignments on Strings. With a focus on user interface development and human-computer interaction, Prof. Anderson excels in formatting strings for output and implementing user-friendly string handling features. Her expertise includes integrating string operations with graphical user interfaces (GUI) and optimizing string formatting for performance.
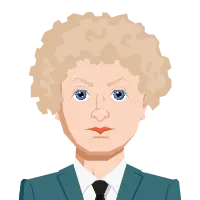
Dr. Oliver Mitchell
Ph.D. in Computer Science
🇦🇺 Australia
Dr. Oliver Mitchell holds a Ph.D. in Computer Science from the University of Melbourne and has successfully completed over 900 assignments on Strings in C. Dr. Mitchell specializes in Unicode handling, multibyte character sets, and internationalization in programming. His expertise extends to implementing secure string manipulation functions and optimizing string search algorithms for diverse applications.
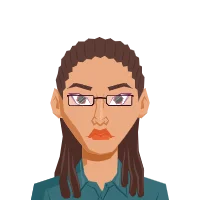
Prof. Elizabeth Taylor
Master's in Computer Engineering
🇬🇧 United Kingdom
Prof. Elizabeth Taylor is a seasoned professional with a Master's degree in Computer Engineering from MIT and has completed over 700 C programming assignments related to Strings. Prof. Taylor's expertise lies in software development methodologies and debugging techniques. She excels in crafting robust solutions for string parsing, tokenization, and implementing custom string functions. Prof. Taylor's dedication to precision and clarity ensures that students receive well-documented and efficient solutions.
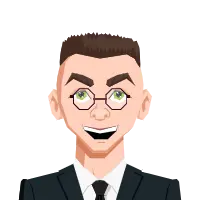
Dr. Benjamin White
Ph.D. in Computer Science
🇺🇸 United States
Dr. Benjamin White holds a Ph.D. in Computer Science from Stanford University and has completed over 800 C programming assignments on Strings. With a specialization in algorithm design and analysis, Dr. White excels in developing efficient solutions for complex programming tasks. His expertise includes string manipulation, dynamic memory allocation, and optimization techniques. Dr. White is committed to delivering high-quality solutions that meet academic standards and exceed expectations.
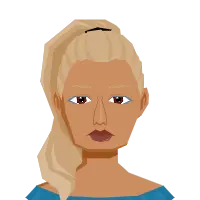
Prof. Isabella Storey
MEd in Computer Science
🇨🇦 Canada
Prof. Isabella Storey, an accomplished educator with an MEd in Computer Science from McGill University, has completed over 600 assignments on Pointers in C. With a teaching tenure at the University of Toronto, Prof. Storey specializes in pointer and array manipulation, pointer arithmetic, and error handling in pointer operations. Her assignments focus on foundational concepts that lay a strong groundwork for advanced C programming skills.
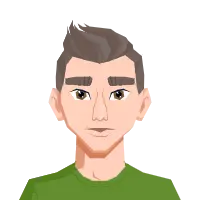
Dr. Liam Davies
PhD in Software Engineering
🇦🇺 Australia
Dr. Liam Davies, with a PhD in Software Engineering from the University of Sydney, has successfully completed more than 900 assignments on Pointers in C. As a seasoned industry practitioner in Melbourne, Dr. Davies specializes in pointer to functions, pointer-based data structures, and real-time applications of pointers. His assignments emphasize practical implementations that bridge theoretical knowledge with industry demands.
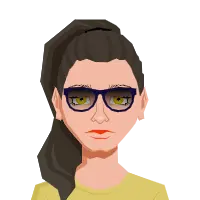
Prof. Rachel Reynolds
MSc in Computer Engineering
🇺🇸 United States
Prof. Rachel Reynolds brings her expertise as a former professor at Harvard University with over 700 completed C assignments on Pointers. Holding an MSc in Computer Engineering from MIT, Prof. Reynolds excels in pointer basics, pointer casting, and the application of pointers in data structures. Her assignments often focus on enhancing pointer efficiency in algorithmic solutions and integrating pointers with object-oriented programming concepts.
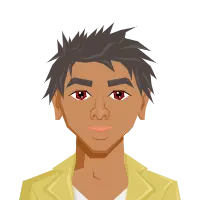
Dr. James Mitchell
Ph.D in Computer Science
🇬🇧 United Kingdom
Dr. James Mitchell holds a PhD in Computer Science from Oxford University and has completed over 800 C programming assignments on Pointers. With a background as a former lecturer at University College London, Dr. Mitchell specializes in dynamic memory allocation, pointer arithmetic, and file handling with pointers. His expertise extends to optimizing memory usage in complex data structures and implementing efficient algorithms using pointers.
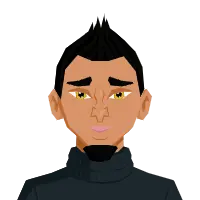
Prof. Ethan Lee
Master's in Computer Science
🇨🇦 Canada
Professor Ethan Lee, with a Master's degree in Computer Science from a prominent university in Canada, has successfully handled over 600 assignments on memory allocation using C. Prof. Lee's expertise spans across teaching fundamental programming concepts, implementing efficient memory allocation strategies, and providing practical solutions to challenging programming assignments.
Related Topics
Frequently Asked Questions
Have questions about our Makefile & Modular Design assignment help? Check out our FAQs section for answers to commonly asked questions. If you can't find the information you're looking for, feel free to reach out to our 24/7 customer support team via live chat. We're here to assist you with any inquiries you may have.