Claim Your Offer
Unlock an amazing offer at www.programminghomeworkhelp.com with our latest promotion. Get an incredible 10% off on your all programming assignment, ensuring top-quality assistance at an affordable price. Our team of expert programmers is here to help you, making your academic journey smoother and more cost-effective. Don't miss this chance to improve your skills and save on your studies. Take advantage of our offer now and secure exceptional help for your programming assignments.
We Accept
Access Our Sample Mapping Module Assignments with Solutions
Get a glimpse of the quality of our work by exploring our sample solutions for Mapping module assignments. These carefully crafted solutions showcase our expertise in C programming and demonstrate our ability to tackle various mapping challenges effectively.
C
C
C
C
C
C
C
C
C
C
C
C
C
C
C
C
C
C
C
C
Get Comprehensive Mapping Module Assignment Help Using C at Affordable Price
At ProgrammingHomeworkHelp.com, we understand the financial constraints students often face, which is why we're committed to providing Mapping module assignment help using C at affordable rates. We customize our prices to ensure accessibility without compromising on quality. Our transparent pricing model is designed to accommodate varying needs and budgets, with options for both basic and more complex assignments. Check out our sample price ranges below to see how we tailor our rates to suit your requirements:
Service Type | Price Range |
---|---|
Basic Mapping Algorithm | $30 - $50 |
Data Structure Mapping | $40 - $60 |
Complex Algorithm Design | $50 - $80 |
Class Project | $80 - $120 |
Performance Optimization | $60 - $100 |
- What Is Mapping Module in C Programming?
- Explore the Popular Topics Covered by Our Reliable Mapping Module in C Homework Writing Help
- Advantages of Availing Our Premium Quality Help with Mapping Module in C Assignments
- Pay Us to Do Your Mapping Module Assignments Using C
- How to Hire Our Mapping Module Assignment Helpers to Do Your Homework Using C?
What Is Mapping Module in C Programming?
In C programming, the term "mapping module" typically refers to a component or functionality within a program that involves mapping data from one form to another. This could involve various operations such as transforming data structures, converting between data types, or associating keys with corresponding values (commonly known as key-value mapping). Essentially, the mapping module facilitates the manipulation and organization of data in a way that is efficient and meaningful for the specific requirements of a program or application. It's commonly used in areas such as database management, algorithm design, and system development to handle and process data effectively.
Here's a simple example of a mapping module in C programming that demonstrates a basic key-value mapping using a structure:
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
// Define a structure for key-value pair
struct KeyValue {
char key[50];
int value;
};
// Function to insert a key-value pair into the map
void insert(struct KeyValue map[], char key[], int value, int *size) {
strcpy(map[*size].key, key);
map[*size].value = value;
(*size)++;
}
// Function to get the value corresponding to a given key
int get(struct KeyValue map[], char key[], int size) {
for (int i = 0; i < size; i++) {
if (strcmp(map[i].key, key) == 0) {
return map[i].value;
}
}
return -1; // Return -1 if key is not found
}
int main() {
struct KeyValue map[100];
int size = 0;
// Inserting key-value pairs
insert(map, "apple", 10, &size);
insert(map, "banana", 20, &size);
insert(map, "orange", 15, &size);
// Getting value for a given key
printf("Value for key 'banana': %d\n", get(map, "banana", size));
printf("Value for key 'grape': %d\n", get(map, "grape", size));
return 0;
}
Explore the Popular Topics Covered by Our Reliable Mapping Module in C Homework Writing Help
Mapping module coursework in C programming covers a plethora of exciting topics. At ProgrammingHomeworkHelp.com, we specialize in crafting exceptional homework solutions tailored to these eight key areas and more:
- Data Structure Implementation: Our expertise shines in implementing various data structures crucial for mapping operations, including arrays, linked lists, trees, and hash tables. With our Mapping module homework writing help by C assignment help experts, students grasp the intricacies of data structure design and manipulation.
- Algorithm Design: Crafting efficient algorithms for mapping tasks is our forte. Whether it's implementing key-value mapping, searching, sorting, or traversing algorithms, our C programming help ensures students receive comprehensive guidance in algorithmic problem-solving for their Mapping module homework.
- Memory Management: Memory optimization is essential in C programming, especially when dealing with large datasets. We excel in teaching students memory management techniques like dynamic memory allocation and deallocation, ensuring their Mapping module homework solutions are not only correct but also optimized.
- File Handling: Manipulating files and streams is a fundamental aspect of many mapping applications. Our experts provide detailed guidance on file handling concepts and help students integrate file operations seamlessly into their Mapping module homework assignments.
- Pointer Manipulation: Pointers are powerful tools in C programming, often used extensively in mapping tasks. We offer specialized assistance in mastering pointer manipulation techniques, enabling students to leverage pointers effectively in their Mapping module homework projects.
- Error Handling: Robust error handling mechanisms are crucial for reliable mapping applications. Our C programming help emphasizes error detection and handling strategies, equipping students with the skills to create resilient Mapping module homework solutions that gracefully handle unexpected scenarios.
- Optimization Techniques: Efficiency is key in mapping applications, and we specialize in teaching students optimization techniques to enhance the performance of their C programs. From algorithmic optimizations to memory and time complexity analysis, our experts ensure students deliver top-notch Mapping module homework solutions.
- Application Development: Applying mapping concepts in real-world scenarios is where the true learning experience lies. Our experts guide students in developing practical Mapping module homework projects, ranging from database management systems to geographic information systems, fostering creativity and problem-solving skills.
With our programming homework writing help, students receive comprehensive support and guidance to conquer the challenges of C programming and excel in their academic endeavors.
Advantages of Availing Our Premium Quality Help with Mapping Module in C Assignments
Navigating the complexities of Mapping module assignments in C programming can be daunting, but at ProgrammingHomeworkHelp.com, we're here to make it easier for you. Our premier help with mapping module assignments come with a plethora of benefits tailored to ensure your academic success:
- Student-Friendly Rates: We understand the financial constraints students often face, which is why we offer our help with Mapping module in C programming assignments at affordable rates, making academic assistance accessible to all.
- Personalized Services by Skilled C Programmers: When you choose us, you're not just getting generic solutions. Our team of skilled C programmers provides personalized assistance, catering to your specific requirements and ensuring that your Mapping module assignments are tailored to your needs.
- Prompt Deliveries of All Orders: Time is of the essence, especially when deadlines are looming. With our help, you can rest assured that your Mapping module assignments will be delivered promptly, allowing you ample time for review and submission.
- Free Multiple Revisions: Your satisfaction is our priority. That's why we offer free multiple revisions on all Mapping module assignments to ensure they meet your expectations and academic standards.
- Well-Commented Codes Worthy of an A+ Grade: We don't just focus on correctness; we also emphasize clarity and understanding. Our experts provide well-commented codes for your Mapping module assignments, enabling you to grasp the concepts thoroughly and earn top grades.
- Money-Back Guarantee: Your trust is invaluable to us. In the rare event that you're not satisfied with our services, we offer a money-back guarantee, ensuring that you get the quality assistance you deserve or your money back.
With our help with Mapping module in C programming assignments, you're not just investing in completing tasks; you're investing in your academic growth and success. Let us be your partners in achieving excellence in C programming.
Pay Us to Do Your Mapping Module Assignments Using C
The Mapping module in C programming presents students with a diverse range of assignments that test their understanding of data structures, algorithms, and programming principles. At ProgrammingHomeworkHelp.com, we excel in providing exceptional coursework assistance to students saying, “I want to pay someone to do my mapping module assignments using C,” ensuring they achieve academic success effortlessly:
- Writing Codes: From basic mapping algorithms to complex data structure implementations, our experts are adept at writing codes for Mapping module assignments that meet your requirements and exceed expectations. Pay someone to do my Mapping module assignment using C, and witness code excellence.
- Class Projects: Class projects often require comprehensive mapping functionalities integrated into larger software systems. Our skilled programmers are well-equipped to handle such projects, ensuring seamless integration of mapping modules and adherence to project specifications. Let us handle your Mapping module homework while you focus on other aspects of your coursework.
- Debugging: Debugging is an inevitable part of programming, and our team excels in identifying and fixing errors in Mapping module assignments. Whether it's runtime errors, logical bugs, or compilation issues, we'll ensure your code runs smoothly and efficiently.
- Performance Optimization: Mapping module assignments may involve handling large datasets or complex operations, requiring optimization for efficiency. Our experts specialize in optimizing code and algorithms, ensuring your Mapping module homework solutions are both correct and performant.
- Algorithmic Analysis: Understanding the time and space complexity of algorithms is crucial in Mapping module coursework. Our team provides in-depth analysis and optimization recommendations, helping you develop efficient algorithms and earn top grades in your assignments.
- Documentation and Presentation: Clear documentation and presentation of Mapping module assignments are essential for conveying your ideas and methodologies effectively. Our experts ensure that your homework is well-documented and presented professionally, enhancing its readability and comprehension.
How to Hire Our Mapping Module Assignment Helpers to Do Your Homework Using C?
With our straightforward process, hiring our mapping module assignment helpers is just a few clicks away. Here's how our service works:
- Submit Your Requirements: The first step is to submit your Mapping module homework requirements through our user-friendly platform. Simply provide details such as the assignment prompt, any specific instructions, and your deadline.
- Get Matched with an Expert: Once we receive your requirements, our team carefully assesses the task and assigns it to a suitable C programming expert. We match you with a knowledgeable professional who has the skills and experience to handle your Mapping module assignment effectively.
- Receive Your Solution: Sit back and relax as our expert works diligently on your Mapping module homework. We ensure prompt deliveries, so you'll receive your completed assignment well before your deadline. Once you receive the solution, review it thoroughly.
Enhance Your Knowledge of Mapping Module in C Programming Through Our Blog
Stay updated with the latest trends, tips, and insights in C programming and Mapping module assignments by exploring our blog. Our blog features informative articles, tutorials, and guides written by our expert team to help you enhance your programming skills and excel in your coursework. Whether you're looking for programming tricks or in-depth explanations of mapping algorithms, our blog has something for everyone. Dive into our blog section to expand your knowledge and stay ahead in your studies.
More than 1.2K Genuine Customer Reviews with an Average Rating of 4.8/5
Our satisfied customers speak volumes about the quality of our service. Check out what students have to say about their experience with our Mapping module assignment help using C programming. We take pride in delivering exceptional solutions that exceed expectations and help students achieve academic success. Our reviews reflect our commitment to excellence and our dedication to providing top-notch assistance to students worldwide. Read through our customer testimonials to see why we're the preferred choice for Mapping module assignments.
Hire Our In-House Mapping Module Assignment Experts Certified in C Programming
Our team of experts comprises seasoned C programmers with years of experience in tackling Mapping module assignments. Each expert undergoes a rigorous selection process to ensure they possess the necessary skills and knowledge to deliver high-quality solutions. With their proficiency in C programming and dedication to academic excellence, our experts are committed to providing personalized assistance tailored to your specific needs.
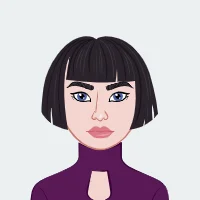
Camila Perry
PhD in Programming
🇺🇸 United States
Camila Perry, an experienced programmer with a degree from the Princeton University, specializes in teaching efficient memory management and advanced C programming techniques.
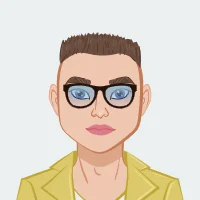
Andrew Reisinger
PhD in Programming
🇺🇸 United States
Andrew Reisinger is a seasoned C Programming Assignment Expert with over a decade of experience. Specializing in data structures, algorithms, and embedded systems, he helps students and professionals tackle complex C programming challenges. John is known for his clear explanations, strong problem-solving skills, and dedication to student success.
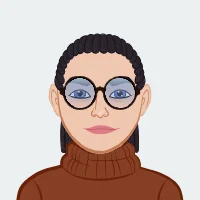
Philip Dudley
PhD in Programming
🇺🇸 United States
Philip Dudley is a seasoned software engineer with over 10 years of experience specializing in C programming and systems-level development. He excels in file descriptor management, memory allocation, and performance optimization. Philip has a strong track record of mentoring students and professionals, helping them master complex programming concepts and improve their coding practices for robust software solutions.
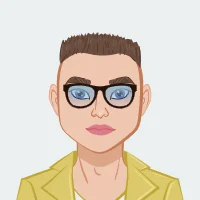
James Maker
Masters in Programming
🇺🇸 United States
James Maker is a seasoned C Programming Assignment Expert with over a decade of experience. Specializing in linked lists, file I/O, and bitwise operations, Alex provides expert guidance and solutions. With a strong background in computer science, Alex helps students master complex assignments and optimize their coding skills.
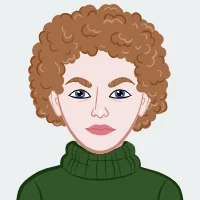
Andrew Hicks
Masters in Computer Science
🇺🇸 United States
Andrew Hicks, a seasoned software engineer, specializes in high-performance systems and memory management with over 15 years of experience.
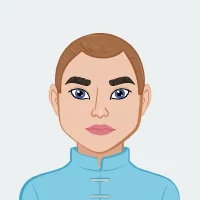
Robert Horta
Masters in C
🇬🇧 United Kingdom
Alex Johnson is a seasoned C programming expert with over a decade of experience. He has helped over 1,000 students excel in their C assignments through personalized tutoring and clear, efficient code solutions. Alex holds advanced degrees in computer science and has received multiple awards for his exceptional teaching and programming skills.
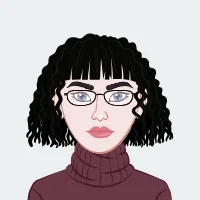
Elijah Patel
Masters in C
🇦🇺 Australia
Elijah Patel is a seasoned C assignment help Expert with 12 years of experience. Holding a Master's degree from the University of New South Wales, Australia.
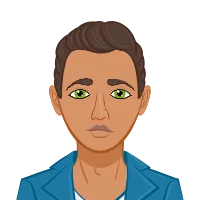
Damien Carrick
Bachelor of Science degree in Computer Science
🇬🇧 United Kingdom
Damien holds a Bachelor of Science degree in Computer Science and specializes in data structures and algorithms for game programming in C. With over 900 completed orders, he is proficient in designing efficient and scalable solutions for game simulations.

Isabella Ruiz
Bachelor of Engineering
🇺🇸 United States
Isabella Ruiz is a Bachelor of Engineering graduate. She is skilled in physics simulation and collision detection for game development in C. With over 600 completed orders, she specializes in creating realistic and immersive gaming experiences.
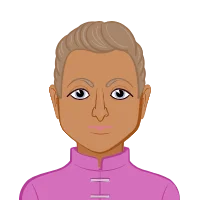
Jake Walker
Master's degree in Software Engineering
🇬🇧 United Kingdom
Jake Walker, with a Master's degree in Software Engineering, is adept at implementing efficient game loops and handling user inputs in C. Having completed over 700 orders, he excels in optimizing performance and debugging C game simulations.
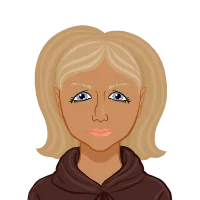
Dr. Miriam Goldbridge
Ph.D. in Computer Science
🇬🇧 United Kingdom
Dr. Miriam Goldbridge holds a Ph.D. in Computer Science and specializes in game development using C programming. With over 800 completed orders, she has extensive experience in crafting complex game simulations with a focus on AI algorithms and graphics rendering.
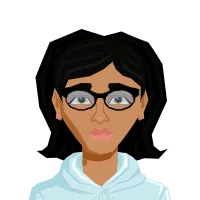
Prof. Rachel Anderson
M.Sc. in Software Engineering
🇨🇦 Canada
Prof. Rachel Anderson holds an M.Sc. in Software Engineering from the University of Toronto and has completed over 600 assignments on Strings. With a focus on user interface development and human-computer interaction, Prof. Anderson excels in formatting strings for output and implementing user-friendly string handling features. Her expertise includes integrating string operations with graphical user interfaces (GUI) and optimizing string formatting for performance.
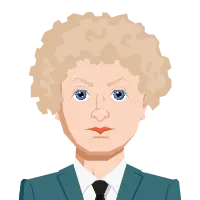
Dr. Oliver Mitchell
Ph.D. in Computer Science
🇦🇺 Australia
Dr. Oliver Mitchell holds a Ph.D. in Computer Science from the University of Melbourne and has successfully completed over 900 assignments on Strings in C. Dr. Mitchell specializes in Unicode handling, multibyte character sets, and internationalization in programming. His expertise extends to implementing secure string manipulation functions and optimizing string search algorithms for diverse applications.
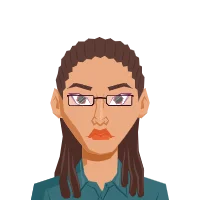
Prof. Elizabeth Taylor
Master's in Computer Engineering
🇬🇧 United Kingdom
Prof. Elizabeth Taylor is a seasoned professional with a Master's degree in Computer Engineering from MIT and has completed over 700 C programming assignments related to Strings. Prof. Taylor's expertise lies in software development methodologies and debugging techniques. She excels in crafting robust solutions for string parsing, tokenization, and implementing custom string functions. Prof. Taylor's dedication to precision and clarity ensures that students receive well-documented and efficient solutions.
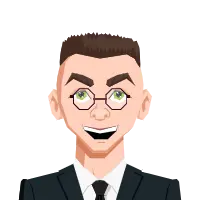
Dr. Benjamin White
Ph.D. in Computer Science
🇺🇸 United States
Dr. Benjamin White holds a Ph.D. in Computer Science from Stanford University and has completed over 800 C programming assignments on Strings. With a specialization in algorithm design and analysis, Dr. White excels in developing efficient solutions for complex programming tasks. His expertise includes string manipulation, dynamic memory allocation, and optimization techniques. Dr. White is committed to delivering high-quality solutions that meet academic standards and exceed expectations.
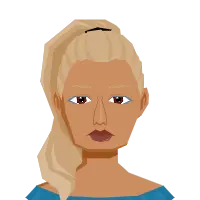
Prof. Isabella Storey
MEd in Computer Science
🇨🇦 Canada
Prof. Isabella Storey, an accomplished educator with an MEd in Computer Science from McGill University, has completed over 600 assignments on Pointers in C. With a teaching tenure at the University of Toronto, Prof. Storey specializes in pointer and array manipulation, pointer arithmetic, and error handling in pointer operations. Her assignments focus on foundational concepts that lay a strong groundwork for advanced C programming skills.
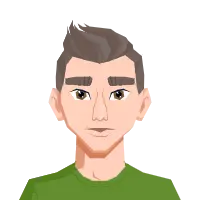
Dr. Liam Davies
PhD in Software Engineering
🇦🇺 Australia
Dr. Liam Davies, with a PhD in Software Engineering from the University of Sydney, has successfully completed more than 900 assignments on Pointers in C. As a seasoned industry practitioner in Melbourne, Dr. Davies specializes in pointer to functions, pointer-based data structures, and real-time applications of pointers. His assignments emphasize practical implementations that bridge theoretical knowledge with industry demands.
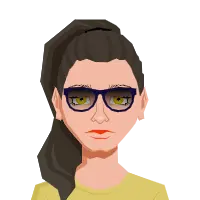
Prof. Rachel Reynolds
MSc in Computer Engineering
🇺🇸 United States
Prof. Rachel Reynolds brings her expertise as a former professor at Harvard University with over 700 completed C assignments on Pointers. Holding an MSc in Computer Engineering from MIT, Prof. Reynolds excels in pointer basics, pointer casting, and the application of pointers in data structures. Her assignments often focus on enhancing pointer efficiency in algorithmic solutions and integrating pointers with object-oriented programming concepts.
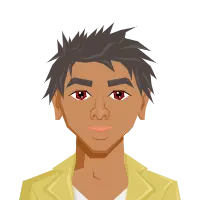
Dr. James Mitchell
Ph.D in Computer Science
🇬🇧 United Kingdom
Dr. James Mitchell holds a PhD in Computer Science from Oxford University and has completed over 800 C programming assignments on Pointers. With a background as a former lecturer at University College London, Dr. Mitchell specializes in dynamic memory allocation, pointer arithmetic, and file handling with pointers. His expertise extends to optimizing memory usage in complex data structures and implementing efficient algorithms using pointers.
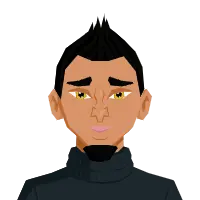
Prof. Ethan Lee
Master's in Computer Science
🇨🇦 Canada
Professor Ethan Lee, with a Master's degree in Computer Science from a prominent university in Canada, has successfully handled over 600 assignments on memory allocation using C. Prof. Lee's expertise spans across teaching fundamental programming concepts, implementing efficient memory allocation strategies, and providing practical solutions to challenging programming assignments.
Related Topics
Frequently Asked Questions
Have questions about our Mapping module assignment help using C programming? Check out our FAQs section for answers to commonly asked questions. Can't find what you're looking for? Our 24/7 customer support team is available via live chat to assist you with any inquiries or concerns you may have. Explore our FAQs to learn more about our services, pricing, and how we can help you succeed in your academic endeavors.
If you encounter any errors or bugs in the code we provide, don't worry. Simply reach out to our support team via live chat, and we'll promptly address and fix any issues you encounter. Your satisfaction is our priority, and we're committed to ensuring that you receive high-quality solutions for your Mapping module homework.
Absolutely. Memory management is a crucial aspect of programming, especially in mapping applications. Our C programmers are proficient in optimizing memory usage through techniques like dynamic memory allocation and deallocation, ensuring efficient memory utilization in your assignment.
Yes, our solutions always come with detailed explanations. We ensure that each step of the solution is clearly explained, enabling you to understand the logic and implementation behind the code for your Mapping module assignment.
Our C programmers specialize in algorithm design for mapping tasks. Whether it's implementing key-value mapping, sorting algorithms, or data structure optimizations, we can help you with your specific algorithmic requirements.