Claim Your Offer
Unlock an amazing offer at www.programminghomeworkhelp.com with our latest promotion. Get an incredible 10% off on your all programming assignment, ensuring top-quality assistance at an affordable price. Our team of expert programmers is here to help you, making your academic journey smoother and more cost-effective. Don't miss this chance to improve your skills and save on your studies. Take advantage of our offer now and secure exceptional help for your programming assignments.
We Accept
Access Our Repository of Sample sorting Array Values in C Assignments
We provide sample assignments to give you a clear idea of the quality and comprehensiveness of our work. These samples showcase various sorting algorithms implemented in C, complete with detailed explanations and comments.
C
Data Structures and Algorithms
C
C
C
C
C
C
C
C
C
C
C
C
C
C
C
C
C
C
Get Comprehensive Online Sorting Array Values in C Assignment Help at Affordable Price
At ProgrammingHomeworkHelp.com, we understand the financial constraints that students often face, which is why we are committed to providing affordable Sorting Array Values in C assignment help. Our pricing model is designed to be flexible and transparent, ensuring that you receive top-quality assistance without breaking the bank. By customizing our rates based on the complexity, urgency, and length of the assignment, we make sure our services are accessible to all students. Below is a table showcasing sample price ranges for different types of assignments to give you an idea of how we keep our services budget-friendly.
Assignment Type | Price Range (USD) |
---|---|
Simple Sorting Algorithm (Bubble Sort) | $20 - $40 |
Intermediate Sorting Algorithm (Selection Sort) | $30 - $50 |
Advanced Sorting Algorithm (Quick Sort, Merge Sort) | $40 - $70 |
Large Dataset Sorting and Optimization | $50 - $90 |
Comprehensive Project with Multiple Sorting Algorithms | $70 - $120 |
- Can I Pay Your Experts to Do My Sorting Array Values Assignment in C on Any Topic?
- Why Programming Students Need Help with Sorting Array Values Homework in C?
- Benefits of Availing Our Sorting Array Values in C Assignment Help Service
- How Our Sorting Array Values in C Assignment Helpers Approach Your Homework?
Can I Pay Your Experts to Do My Sorting Array Values Assignment in C on Any Topic?
At Programming Homework Help, we take pride in our expertise in a wide range of topics related to sorting array values in C. Whether you're a beginner or an advanced student, our team is equipped to handle assignments of any complexity. So, if you have decided, “I want to pay someone to do my data structures and algorithms assignments,” expect your homework to be completed accurately and on time. Below are the top eight assignment topics in Sorting Array Values in C where we excel:
- Bubble Sort: Bubble Sort is one of the simplest sorting algorithms, but it can be tricky to implement efficiently. Our experts can craft detailed homework solutions that not only sort your data but also explain the underlying principles and optimization techniques.
- Selection Sort: Selection Sort involves finding the minimum element and placing it at the beginning. We specialize in providing homework help that breaks down each step, ensuring you understand the logic and can apply it to future assignments.
- Insertion Sort: Insertion Sort is excellent for small datasets and nearly sorted arrays. Our programming professionals will deliver homework solutions that illustrate how to insert elements correctly and maintain the sorted order efficiently.
- Merge Sort: Merge Sort is a divide-and-conquer algorithm that can handle large datasets efficiently. We excel in delivering homework that includes comprehensive explanations of the recursive process and the merging of sorted subarrays.
- Quick Sort: Quick Sort is one of the fastest sorting algorithms for large datasets. Our expertise ensures that your homework demonstrates how to choose pivots effectively and handle partitioning to optimize performance.
- Heap Sort: Heap Sort involves building a heap and then sorting it. Our team provides detailed homework solutions that explain how to construct the heap and perform the sort, ensuring you understand each step of the process.
- Radix Sort: Radix Sort is used for sorting numbers or strings. Our experts can deliver homework that clearly shows how to sort using different digit places, making complex sorting tasks manageable and understandable.
- Shell Sort: Shell Sort is an optimization of Insertion Sort that handles larger gaps. We offer homework solutions that explain the gap sequence and the sorting process, helping you grasp how this algorithm improves efficiency.
By choosing ProgrammingHomeworkHelp.com, you are assured of receiving high-quality, well-explained solutions tailored to your academic needs. Let us help you excel in your programming coursework with our specialized knowledge and experience.
Why Programming Students Need Help with Sorting Array Values Homework in C?
Sorting array values in C can be a challenging task for many programming students, particularly those new to coding or working with complex algorithms for the first time. Understanding the nuances of different sorting techniques and their implementations requires a strong grasp of both theoretical concepts and practical coding skills. Below, we discuss some common reasons why students seek help with Sorting Array Values homework in C and how professional assistance can make a difference.
- Understanding Complex Algorithms: Many sorting algorithms, such as Quick Sort and Merge Sort, involve intricate steps and recursive processes that can be difficult to grasp without proper guidance. Professional help with Sorting Array Values homework in C can provide clear explanations and examples to make these algorithms more understandable.
- Debugging and Error Handling: C programming is known for its strict syntax and potential for subtle bugs. Students often struggle with debugging their sorting algorithms. Expert assistance can help identify and fix errors, ensuring the code runs correctly and efficiently.
- Time Management: Balancing multiple assignments and deadlines can be overwhelming. Getting help with Sorting Array Values homework in C allows students to manage their time better and focus on other important academic or personal responsibilities.
- Optimizing Code Performance: Writing efficient code is crucial, especially for large datasets. Professionals can offer insights into optimizing sorting algorithms, making them run faster and use fewer resources, which is a valuable skill in both academics and real-world applications.
- Lack of Practical Experience: Students may understand the theory behind sorting algorithms but struggle with actual implementation. Practical examples and step-by-step guidance from experts can bridge this gap, enhancing their coding skills and confidence.
Hiring our C programmers ensures you eliminate all the aforementioned challenges and receive error-free and running codes that can impress the strictest of professors. Here’s an example of a clean code written by our experts of a Bubble Sort algorithm in C:
Example Code: Bubble Sort in C
#include <stdio.h>
// Function to perform Bubble Sort
void bubbleSort(int arr[], int n) {
int i, j, temp;
for (i = 0; i < n-1; i++) {
for (j = 0; j < n-i-1; j++) {
if (arr[j] > arr[j+1]) {
// Swap the elements
temp = arr[j];
arr[j] = arr[j+1];
arr[j+1] = temp;
}
}
}
}
// Function to print the array
void printArray(int arr[], int size) {
int i;
for (i = 0; i < size; i++)
printf("%d ", arr[i]);
printf("\n");
}
// Main function
int main() {
int arr[] = {64, 34, 25, 12, 22, 11, 90};
int n = sizeof(arr)/sizeof(arr[0]);
bubbleSort(arr, n);
printf("Sorted array: \n");
printArray(arr, n);
return 0;
}
Benefits of Availing Our Sorting Array Values in C Assignment Help Service
Choosing the right assistance for your programming assignments can make a significant difference in your academic performance and overall learning experience. Our Sorting Array Values in C assignment help service offers numerous advantages that can enhance your understanding and ensure you succeed in your coursework. Below, we outline the key benefits of availing our specialized services.
- Expert Guidance from Professionals: Our team comprises seasoned programming experts who have extensive experience in C programming and sorting algorithms. By opting for our Sorting Array Values in C assignment help, you gain access to professionals who can provide in-depth explanations and insights, making complex topics easier to understand.
- Customized Solutions Tailored to Your Needs: Every assignment is unique, and our service ensures that the solutions we provide are customized to meet your specific requirements. Whether you need help with a specific algorithm or a comprehensive project, our tailored approach guarantees that your homework is handled with precision and care.
- Timely Delivery and Reliable Support: We understand the importance of deadlines in academic settings. Our team is committed to delivering your Sorting Array Values in C assignment help on time, allowing you to review the work and seek any necessary clarifications. Our reliable support system ensures that you can reach out to us with any questions or concerns.
- Improved Academic Performance: By availing our expert assistance, you can significantly improve your academic performance. Our well-crafted solutions not only help you complete your assignments but also enhance your understanding of key concepts, leading to better grades and a stronger grasp of programming principles.
- Stress Reduction and Better Time Management: Handling multiple programming assignments can be stressful and time-consuming. Our Sorting Array Values in C assignment help service allows you to delegate some of the workload, reducing stress and freeing up time to focus on other important tasks or simply relax and recharge.
- Access to Quality samples for Free: Our service provides you with access to high-quality samples that can further aid your studies. From detailed explanations to practical examples, these free samples are designed to complement your coursework and deepen your understanding of sorting algorithms in C.
- Enhanced Practical Skills: Working with our experts on your assignments helps you develop practical coding skills that are essential for real-world applications. The hands-on experience you gain through our guidance ensures you are well-prepared for future programming challenges.
- Confidence Boost: Receiving professional assistance and successfully completing your assignments can significantly boost your confidence. Our support helps you tackle even the most challenging tasks with assurance, knowing you have the expertise and knowledge to succeed.
By choosing our Sorting Array Values in C assignment writing services, you are investing in a reliable partner dedicated to your academic success and overall development in programming. Let us help you excel in your coursework and build a strong foundation for your future career.
How Our Sorting Array Values in C Assignment Helpers Approach Your Homework?
When you hire our eminent Sorting Array Values in C assignment helpers, you can be assured of a meticulous and thorough approach. Our programming experts follow a well-defined process to ensure your assignments are completed accurately and efficiently. Below, we detail how our sorting array values in C assignment writers work to provide top-quality solutions for your programming needs.
- Understanding the Assignment Requirements: Our process begins with a comprehensive review of your assignment requirements. Our tutors carefully analyze the problem statement, any specific instructions, and desired outcomes. This initial step ensures that we fully understand what is expected and can tailor our approach accordingly.
- Selecting the Appropriate Algorithm: Based on the assignment requirements, our experts choose the most suitable sorting algorithm. Whether it's Bubble Sort, Quick Sort, Merge Sort, or any other algorithm, our programming experts are adept at selecting and implementing the one that best fits the task at hand.
- Writing and Documenting the Code: Our experts then proceed to write the code, ensuring it is clean, efficient, and well-documented. Clear comments and documentation are added to help you understand the logic and flow of the program. This step is crucial for both readability and educational purposes, allowing you to learn from the provided solution.
- Testing and Debugging: After writing the initial code, our experts rigorously test it with various inputs to ensure accuracy and robustness. Any bugs or issues are identified and resolved during this phase, ensuring that the final solution is error-free and performs optimally.
- Optimizing the Code: Efficiency is a key aspect of programming, especially with sorting algorithms. Our sorting array values in C assignment helpers optimize the code to enhance performance. This may involve refining the algorithm, reducing time complexity, or improving resource utilization.
- Providing Explanations and Support: Once the assignment is complete, we provide detailed explanations of the code and the chosen algorithm. This helps you understand the solution thoroughly and prepares you for similar tasks in the future. Additionally, our programming experts are available to answer any questions and provide further support if needed.
- Delivering the Final Solution: The final step is delivering the completed assignment to you well before the deadline. We ensure that you have ample time to review the work and seek any necessary clarifications. Our commitment to timely delivery and quality is a cornerstone of our service.
By following this comprehensive process, our programming assignment help doers guarantee a high-quality, reliable, and educational experience. Let our programming experts assist you in mastering sorting algorithms and achieving academic success.
Latest Blog Posts on Sorting Array Values in C to Sharpen Your Assignment Writing Skills
Stay updated with the latest trends and insights in C programming by exploring our blog section. Our blog features articles on a wide range of topics, from basic sorting techniques to advanced algorithm optimizations. Written by our in-house experts, these posts offer valuable tips, tutorials, and industry news to help you stay ahead in your studies. Regularly reading our blog can provide you with additional knowledge and practical skills that complement your coursework. Visit our blog often to make the most of the resources we offer and enhance your programming expertise.
Honest Reviews & Testimonials Shared by Our Esteemed Customers
We take pride in the positive feedback we receive from our students, which reflects our commitment to quality and customer satisfaction. Our clients consistently praise our timely delivery, comprehensive solutions, and the clarity of our explanations. We believe that listening to our students' experiences is crucial for continuous improvement. By maintaining high standards and focusing on individual needs, we ensure that each student receives the best possible service. Join the many satisfied students who have benefited from our expert assistance and see the difference for yourself.
Meet Our 100+ Proficient & Skilled Sorting Array Values in C Assignment Experts
Our team of experts is comprised of highly skilled and experienced professionals in the field of C programming. Each member holds advanced degrees and has extensive experience in teaching and solving complex programming problems. They are well-versed in various sorting algorithms and can provide in-depth explanations and solutions tailored to your specific needs. By working with our experts, you gain access to a wealth of knowledge and practical insights that can significantly enhance your understanding and performance. Our dedication to excellence ensures that you receive top-quality assistance for your Sorting Array Values in C assignments.
.webp)
Carl Mitchel
PhD in Programming
🇨🇦 Canada
Carl Mitchel is a seasoned software engineer with over 10 years of experience in data structures and algorithms, specializing in Python and efficient data management techniques.
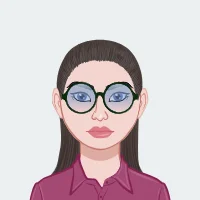
Helen Stevenson
PhD in Programming
🇺🇸 United States
Helen Stevenson, an expert in computer science, offers deep insights into data structures and algorithms. With extensive experience in C programming and software development efficient problem-solving and robust software design, offering valuable perspectives for advanced and aspiring developers alike.
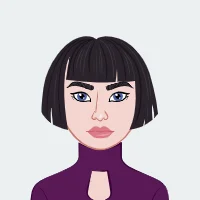
Camila Perry
PhD in Programming
🇺🇸 United States
Camila Perry, an experienced programmer with a degree from the Princeton University, specializes in teaching efficient memory management and advanced C programming techniques.
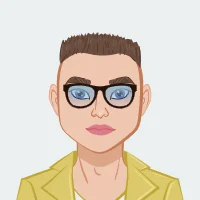
Andrew Reisinger
PhD in Programming
🇺🇸 United States
Andrew Reisinger is a seasoned C Programming Assignment Expert with over a decade of experience. Specializing in data structures, algorithms, and embedded systems, he helps students and professionals tackle complex C programming challenges. John is known for his clear explanations, strong problem-solving skills, and dedication to student success.
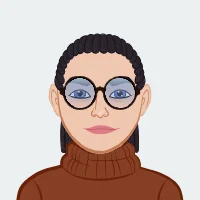
Philip Dudley
PhD in Programming
🇺🇸 United States
Philip Dudley is a seasoned software engineer with over 10 years of experience specializing in C programming and systems-level development. He excels in file descriptor management, memory allocation, and performance optimization. Philip has a strong track record of mentoring students and professionals, helping them master complex programming concepts and improve their coding practices for robust software solutions.
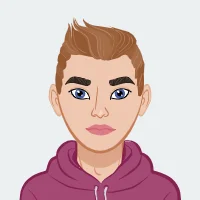
Fredrick Smith
Masters in Programming
🇺🇸 United States
Fredrick Smith is a seasoned software engineer specializing in data structures, algorithms, and C programming. With over 10 years of experience, he excels in designing efficient linked list operations and implementing robust file handling systems. His expertise lies in translating complex concepts into practical solutions, ensuring high performance and reliability in every project.
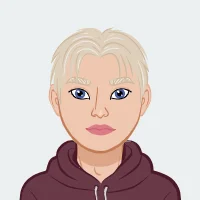
Jeremy Kramer
Masters in Programming
🇺🇸 United States
Jeremy Kramer is a software engineer with over 15 years of experience in algorithm design and performance optimization. He specializes in time complexity analysis and efficient code development.
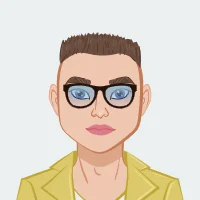
James Maker
Masters in Programming
🇺🇸 United States
James Maker is a seasoned C Programming Assignment Expert with over a decade of experience. Specializing in linked lists, file I/O, and bitwise operations, Alex provides expert guidance and solutions. With a strong background in computer science, Alex helps students master complex assignments and optimize their coding skills.
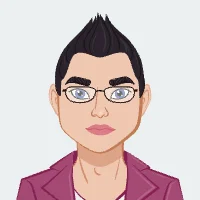
Jimmy Young
Masters in Programming
🇺🇸 United States
Jimmy Young, with 10+ years of experience, specializes in operating systems, covering process scheduling, memory management, concurrency, and file systems. He has successfully guided over 1,000 students, provided tailored solutions and ensured top grades in OS assignments with his in-depth knowledge and practical expertise.
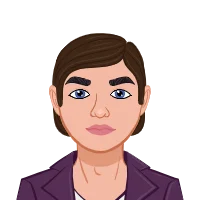
Richard Hayman
Masters in Programming
🇺🇸 United States
Richard Hayman is a seasoned software engineer with over 15 years of experience in algorithm design and implementation. He specializes in graph theory and optimization techniques, bringing a deep understanding of algorithms and their practical applications.
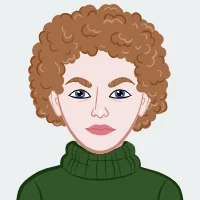
Andrew Hicks
Masters in Computer Science
🇺🇸 United States
Andrew Hicks, a seasoned software engineer, specializes in high-performance systems and memory management with over 15 years of experience.

Rafael Barner
Ph.D. in Programming
🇺🇸 United States
Rafael Barner, an expert in multi-threaded programming with extensive experience in synchronization mechanisms and POSIX threads implementation.
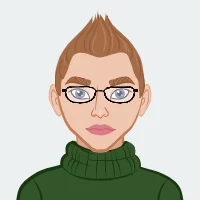
Nathan Carter
Ph.D. in Programming
🇺🇸 United States
Nathan Carter is a seasoned software engineer with 10+ years of experience in algorithm design and performance analysis.
.webp)
John Osborn
Masters in Computer Science
🇬🇧 United Kingdom
John Osborn is a seasoned algorithm expert with over a decade of experience. Specializing in algorithm design and analysis, he offers personalized tutoring, assignment assistance, and guidance on best practices. Proficient in multiple programming languages, John is dedicated to helping students master algorithms and excel academically.
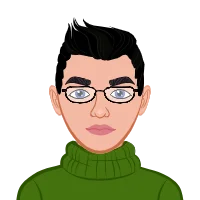
Johnny Houlihan
Masters in Computer Science
🇬🇧 United Kingdom
I’m Johnny Houlihan, a Data Structure Expert with a Master’s in Computer Science from Stanford University. I offer detailed assistance for data structure and algorithm assignments in Java, C++, Python, and more. With years of experience in teaching and problem-solving, I’m here to help you excel.
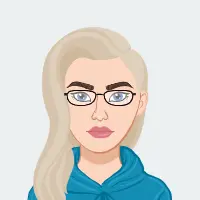
Dr. Amanda King
Ph.D. in Computer Science
🇬🇧 United Kingdom
Dr. Amanda King, a Ph.D. in Computer Science from MIT, is a seasoned Algorithm Assignment Expert with 15+ years of experience. Specializing in algorithm design, data structures, and machine learning, she excels in simplifying complex concepts and mentoring students.
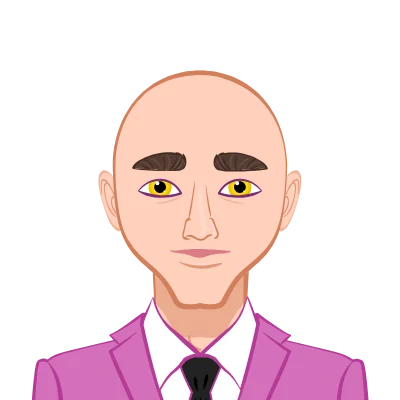
Hayden Ball
Ph.D. in Computer Science
🇬🇧 United Kingdom
Hayden Ball, Ph.D., brings 10 years of expertise in Linked List Assignments. Educated at the University of Warwick, UK, Hayden excels in data structures and algorithmic implementations.
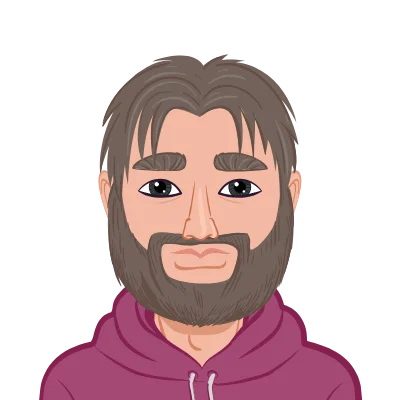
Charles Higgins
Ph.D. in Computer Science
🇺🇸 United States
Introducing Charles Higgins, a seasoned expert in Linked List Assignments with 13 years of experience. Charles earned his Ph.D. from Columbia University, specializing in advanced data structures and algorithmic analysis.
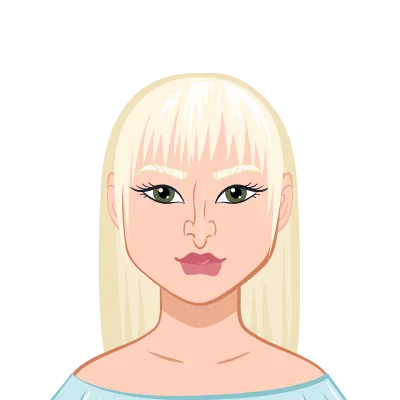
Maya Riley
Ph.D. in Computer Science
🇬🇧 United Kingdom
Maya Riley is an accomplished expert in Linked List Assignments with a Ph.D. from the University of Oxford, UK. With 15 years of experience, she excels in advanced data structures and algorithm implementations.
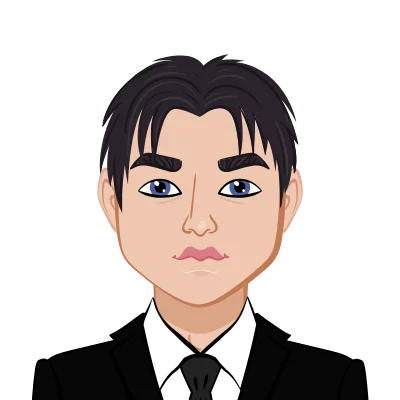
James Patterson
Ph.D. in Computer Science
🇨🇦 Canada
James Patterson, Ph.D., brings 18 years of expertise in Linked List Assignments. Educated at the University of Waterloo, Canada, he specializes in advanced data structures and algorithms.
Related Topics
Frequently Asked Questions
Have questions about our Sorting Array Values in C assignment help service? Our FAQs section has you covered. Here, we address common queries about our process, pricing, and the types of assignments we handle. If you need further clarification, our live chat support is always available to assist you in real-time.
We offer expedited services for urgent assignments. If you have a tight deadline, our team will prioritize your work and ensure it is completed accurately and delivered on time. You can use our live chat support to discuss your urgent needs and get immediate assistance.
Yes, we specialize in handling large datasets. Our experts can implement and optimize sorting algorithms like Quick Sort, Merge Sort, and others to efficiently sort large amounts of data, ensuring your solution is both effective and scalable.
Absolutely. Our solutions come with step-by-step explanations of the sorting algorithms used, including how they work, why they were chosen, and how each part of the code contributes to the overall algorithm. This ensures you not only complete your homework but also understand the underlying concepts.
Yes, we can help optimize your existing sorting code. Our experts can analyze your code for inefficiencies and suggest improvements to enhance performance, whether it’s reducing time complexity or improving resource utilization.
Our experts thoroughly test each sorting algorithm with a variety of input cases to ensure accuracy and robustness. We also provide detailed explanations and comments in the code, which help verify the correctness and facilitate your understanding of the solution.