Claim Your Offer
Unlock an amazing offer at www.programminghomeworkhelp.com with our latest promotion. Get an incredible 10% off on your all programming assignment, ensuring top-quality assistance at an affordable price. Our team of expert programmers is here to help you, making your academic journey smoother and more cost-effective. Don't miss this chance to improve your skills and save on your studies. Take advantage of our offer now and secure exceptional help for your programming assignments.
We Accept
Browse Our Repository of Free Simulating a Game Using C Assignment Samples
Browse through our sample section to see examples of the high-quality work our experts produce. These samples showcase our expertise in Simulating a Game Using C and provide valuable insights into our approach to assignments.
C
C
C
C
C
C
C
C
C
C
C
C
C
C
C
C
C
C
C
C
Comprehensive Simulating a Game Using C Assignment Writing Help at Affordable Price
At ProgrammingHomeworkHelp.com, we understand the importance of affordability when it comes to academic assistance. That's why we offer customized rates tailored to meet the budgetary needs of our clients. We ensure that our pricing remains competitive while maintaining the highest standards of quality and expertise. By carefully evaluating the complexity and scope of each assignment, we provide transparent pricing that reflects the value of our services. With flexible pricing options and transparent communication, we make it easy for students to access the help they need without breaking the bank.
Service | Price Range |
---|---|
Basic Assignment | $30 - $50 |
Intermediate Project | $50 - $100 |
Advanced Assignment | $100 - $200 |
Complex Project | $200 - $300 |
Customized Solutions | Varies based on requirements |
- Benefits of Availing Our Help with Simulating a Game Assignment Using C
- The Best Practices of Simulating a Game Using C
- Subject Areas Our Brilliant Simulating a Game Using C Homework Helpers Excel In
- Pay Someone to Do My Simulating a Game Homework Using C: Projects Our Experts Have Completed for Students
- How Our Simulating a Game Assignment Using C Works in?
Benefits of Availing Our Help with Simulating a Game Assignment Using C
When you seek help with simulating a game assignment using C from ProgrammingHomeworkHelp.com, you gain access to a wealth of expertise and resources tailored to ensure your success. Our services are designed to provide comprehensive support, from the initial stages of game development to the final touches. Here are the key benefits you can expect when you choose our assistance.
- Certified C Programmers Skilled in Game Simulation: Our team comprises certified C programmers who specialize in game simulation. They possess deep knowledge and practical experience, ensuring that your game is built on a solid foundation and adheres to best practices.
- Perfectly Running and Error-Free Games: Quality is our top priority. We guarantee that the games we help you simulate will run smoothly and be free from errors. Our meticulous testing process ensures that every aspect of the game functions as intended.
- On-Time Deliveries: We understand the importance of deadlines. Our team is committed to delivering your game simulation assignments on time, allowing you to meet your submission dates without any stress.
- 24/7 Customer Support: Our customer support team is available around the clock to address any questions or concerns you may have. Whether you need clarification on an assignment or assistance with a new request, we are here to help at any time.
- Free Unlimited Revisions: Your satisfaction is paramount. We offer free unlimited revisions to ensure that the final product meets your expectations and requirements. Our goal is to provide you with a game simulation that you are completely happy with.
- Free Preview of Solution Before Final Payment: To build trust and confidence, we provide a free preview of the solution before you make the final payment. This allows you to review the work and ensure it meets your standards before completing the transaction.
By availing our help with simulating a game assignment using C, you benefit from expert guidance, high-quality results, timely deliveries, and exceptional customer support, all designed to enhance your learning experience and academic performance.
The Best Practices of Simulating a Game Using C
Simulating a game using the C programming language requires a blend of creativity and technical skill. C's performance and low-level capabilities make it an excellent choice for game development, allowing for fine control over system resources. However, to leverage these benefits effectively, adhering to best practices is crucial. These practices ensure your code is efficient, maintainable, and scalable, making the development process smoother and more enjoyable:
- Modular Design: Break down your game into smaller, manageable components. Use functions and modules to separate different parts of the game, such as game logic, input handling, and rendering. This makes the code more organized and easier to debug.
- Use Structs: Utilize C structs to represent game entities and their attributes. This helps in managing the data efficiently and keeps the code clean.
- Consistent Coding Style: Maintain a consistent coding style with clear naming conventions and comments. This improves readability and makes it easier for others (or yourself) to understand the code later.
- Memory Management: Be mindful of memory allocation and deallocation. Use dynamic memory allocation for entities that change in size and always free allocated memory to avoid leaks.
- Error Handling: Implement robust error handling to manage unexpected situations without crashing the game.
- Optimize Performance: Optimize performance by profiling your code and identifying bottlenecks. Use efficient algorithms and data structures to improve the game's responsiveness.
Sample Code for a Simple Game Simulation in C
Here’s a basic example of a simple game simulation in C. This code simulates a player moving around in a 2D grid.
#include <stdio.h>
#include <stdlib.h>
// Define a struct for the player
typedef struct {
int x;
int y;
int score;
} Player;
// Function to initialize the player
void initializePlayer(Player* player, int startX, int startY) {
player->x = startX;
player->y = startY;
player->score = 0;
}
// Function to move the player
void movePlayer(Player* player, char direction) {
switch(direction) {
case 'w': player->y -= 1; break; // move up
case 's': player->y += 1; break; // move down
case 'a': player->x -= 1; break; // move left
case 'd': player->x += 1; break; // move right
default: printf("Invalid move!\n"); break;
}
}
// Function to print the player's position
void printPlayerPosition(Player* player) {
printf("Player Position: (%d, %d)\n", player->x, player->y);
}
int main() {
Player player;
initializePlayer(&player, 0, 0);
char input;
while (1) {
printf("Enter move (w/a/s/d) or q to quit: ");
scanf(" %c", &input);
if (input == 'q') break;
movePlayer(&player, input);
printPlayerPosition(&player);
}
printf("Game Over. Final Position: (%d, %d)\n", player.x, player.y);
return 0;
}
Subject Areas Our Brilliant Simulating a Game Using C Homework Helpers Excel In
At ProgrammingHomeworkHelp.com, our Simulating a game using C assignment helpers are experts in a wide range of topics. Our programming experts provide detailed and customized assistance for each topic, ensuring that your homework is completed to the highest standard. Here are eight specific areas where we excel:
- Game Loop Implementation: Our Simulating a game using C assignment help experts are proficient in designing and implementing efficient game loops. We ensure your homework includes a robust loop that manages game updates, rendering, and user input seamlessly.
- Physics and Collision Detection: Understanding physics and collision detection is crucial for realistic game simulations. Our programming experts can help you create homework assignments that accurately simulate physical interactions and handle collision detection effectively.
- AI and NPC Behavior: Creating intelligent non-player characters (NPCs) can be challenging. Our experts excel in crafting homework that includes sophisticated AI algorithms, making your NPCs behave in a lifelike and engaging manner.
- User Input Handling: Handling user inputs accurately is vital for any interactive game. We provide assistance in creating homework assignments that ensure smooth and responsive input handling, enhancing the overall gaming experience.
- Graphics and Rendering: Our programming experts are skilled in the intricacies of graphics and rendering. We help you with homework assignments that involve drawing and animating game objects, ensuring they look polished and run efficiently.
- Game State Management: Managing different game states (e.g., menu, playing, paused) is essential for a smooth user experience. Our Simulating a game using C assignment helpers can guide you in creating homework that effectively transitions between these states.
- Sound Integration: Sound adds another layer of immersion to games. Our experts assist in incorporating sound effects and music into your game simulations, making sure your homework includes a complete and engaging audio experience.
- Data Structures and Algorithms: Efficient data structures and algorithms are the backbone of any game. We provide help with homework that involves choosing and implementing the best data structures and algorithms to optimize your game's performance.
With our expertise in these areas, our Simulating a game using C assignment helpers ensure that your homework not only meets academic standards but also stands out for its quality and thoroughness.
Pay Someone to Do My Simulating a Game Homework Using C: Projects Our Experts Have Completed for Students
At ProgrammingHomeworkHelp.com, we take pride in the diverse range of Simulating a game using C homework our experts have successfully completed for students. With our programming homework help, students wondering, “where can I pay someone to do my simulating a game homework using C” receive top-notch assistance in crafting engaging and functional projects. Here are eight exemplary projects that showcase our expertise:
- Number Guessing Game: Our experts have crafted homework assignments for the classic Number Guessing Game, where players attempt to guess a randomly generated number within a specified range. We ensure that the game logic is implemented accurately, providing an enjoyable and interactive experience for players.
- Dice Rolling Simulator: Students seeking programming help can rely on us to create a Dice Rolling Simulator homework project. Our experts ensure that the simulation accurately emulates the rolling of dice, allowing users to specify the number of dice and the number of sides on each die.
- Rock-Paper-Scissors: For homework assignments involving the Rock-Paper-Scissors game, our experts deliver projects that implement the game's rules and logic flawlessly. Players can engage in matches against the computer or against each other, with intuitive user interfaces and smooth gameplay.
- Text-Based Adventure Game: Our programming help extends to crafting Text-Based Adventure Game projects, where players navigate through a story-driven narrative by making choices at various decision points. We ensure that the game offers multiple paths and endings, providing an immersive experience for players.
- Tic-Tac-Toe: Students can pay someone to do their Tic-Tac-Toe homework project using C programming help from us. Our experts create well-designed implementations of the classic game, complete with intelligent AI opponents and options for single-player or multiplayer gameplay.
- Hangman: Homework assignments for the Hangman game are expertly handled by our team. We develop projects that challenge players to guess words within a limited number of attempts, with customizable word lists and appealing graphical interfaces.
- Snake: Our experts excel in creating homework projects for the Snake game, where players control a snake to eat food and grow in length while avoiding collisions with walls and the snake's own body. We ensure smooth movement and responsive controls for an enjoyable gaming experience.
- Text-Based RPG (Role-Playing Game): Students can rely on our programming help for crafting Text-Based RPG projects that immerse players in rich, interactive worlds. Our experts design homework assignments that feature diverse characters, engaging storylines, and strategic gameplay elements.
With our expertise in these projects, students can rest assured that their Simulating a game using C homework and assignments will be completed with precision and creativity, meeting both academic requirements and their own expectations.
How Our Simulating a Game Assignment Using C Works in?
At ProgrammingHomeworkHelp.com, we've streamlined the process of getting expert assistance with your Simulating a game assignment using C. Follow these four simple steps to receive high-quality guidance and support:
- Submit Your Assignment Details
- Get Matched with a Programming Expert
- Collaborate on Your Assignment
- Receive Your Completed Assignment
By following these four steps, you can access expert assistance with your Simulating a game assignment using C, ensuring that you receive personalized support and guidance every step of the way.
Well-Researched Blogs to Keep You Updated on Simulating a Game Using C
Explore our blog section for insightful articles, tips, and tutorials on Simulating a Game Using C and other programming topics. Written by our team of experts, these blog posts cover a wide range of subjects, from beginner tutorials to advanced techniques. Stay updated with the latest trends and developments in game development and programming through our informative blog posts.
More than 1.2K Reviews & Testimonials Shared by Our Esteemed Customers
In our review section, you'll find feedback from satisfied clients who have benefited from our Simulating a Game Using C assignment help. These reviews highlight the quality of our services, the expertise of our experts, and our commitment to customer satisfaction. We take pride in our reputation for delivering exceptional results and strive to exceed our clients' expectations with every project.
Hire Certified Simulating a Game Using C Assignment Experts with 10+ Years of Experience
Our team of experts consists of seasoned professionals with extensive experience in game development and programming using C. Each expert undergoes a rigorous selection process to ensure they possess the necessary skills and expertise to provide top-notch assistance to our clients. With their in-depth knowledge and dedication to excellence, our experts are committed to delivering outstanding results for every Simulating a Game Using C assignment.
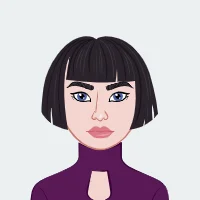
Camila Perry
PhD in Programming
🇺🇸 United States
Camila Perry, an experienced programmer with a degree from the Princeton University, specializes in teaching efficient memory management and advanced C programming techniques.
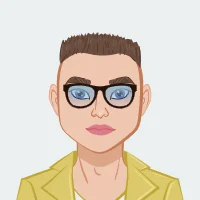
Andrew Reisinger
PhD in Programming
🇺🇸 United States
Andrew Reisinger is a seasoned C Programming Assignment Expert with over a decade of experience. Specializing in data structures, algorithms, and embedded systems, he helps students and professionals tackle complex C programming challenges. John is known for his clear explanations, strong problem-solving skills, and dedication to student success.
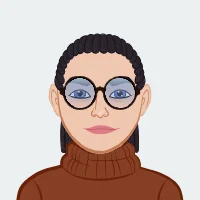
Philip Dudley
PhD in Programming
🇺🇸 United States
Philip Dudley is a seasoned software engineer with over 10 years of experience specializing in C programming and systems-level development. He excels in file descriptor management, memory allocation, and performance optimization. Philip has a strong track record of mentoring students and professionals, helping them master complex programming concepts and improve their coding practices for robust software solutions.
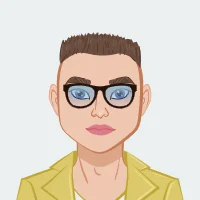
James Maker
Masters in Programming
🇺🇸 United States
James Maker is a seasoned C Programming Assignment Expert with over a decade of experience. Specializing in linked lists, file I/O, and bitwise operations, Alex provides expert guidance and solutions. With a strong background in computer science, Alex helps students master complex assignments and optimize their coding skills.
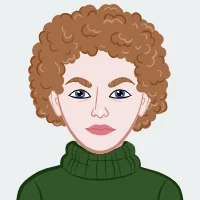
Andrew Hicks
Masters in Computer Science
🇺🇸 United States
Andrew Hicks, a seasoned software engineer, specializes in high-performance systems and memory management with over 15 years of experience.
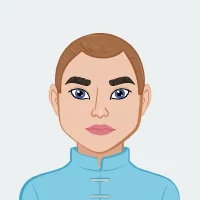
Robert Horta
Masters in C
🇬🇧 United Kingdom
Alex Johnson is a seasoned C programming expert with over a decade of experience. He has helped over 1,000 students excel in their C assignments through personalized tutoring and clear, efficient code solutions. Alex holds advanced degrees in computer science and has received multiple awards for his exceptional teaching and programming skills.
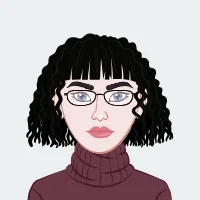
Elijah Patel
Masters in C
🇦🇺 Australia
Elijah Patel is a seasoned C assignment help Expert with 12 years of experience. Holding a Master's degree from the University of New South Wales, Australia.
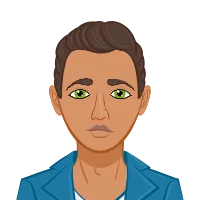
Damien Carrick
Bachelor of Science degree in Computer Science
🇬🇧 United Kingdom
Damien holds a Bachelor of Science degree in Computer Science and specializes in data structures and algorithms for game programming in C. With over 900 completed orders, he is proficient in designing efficient and scalable solutions for game simulations.

Isabella Ruiz
Bachelor of Engineering
🇺🇸 United States
Isabella Ruiz is a Bachelor of Engineering graduate. She is skilled in physics simulation and collision detection for game development in C. With over 600 completed orders, she specializes in creating realistic and immersive gaming experiences.
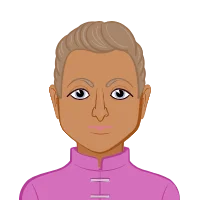
Jake Walker
Master's degree in Software Engineering
🇬🇧 United Kingdom
Jake Walker, with a Master's degree in Software Engineering, is adept at implementing efficient game loops and handling user inputs in C. Having completed over 700 orders, he excels in optimizing performance and debugging C game simulations.
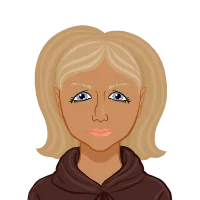
Dr. Miriam Goldbridge
Ph.D. in Computer Science
🇬🇧 United Kingdom
Dr. Miriam Goldbridge holds a Ph.D. in Computer Science and specializes in game development using C programming. With over 800 completed orders, she has extensive experience in crafting complex game simulations with a focus on AI algorithms and graphics rendering.
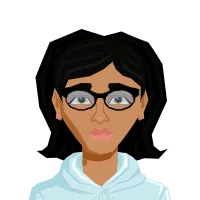
Prof. Rachel Anderson
M.Sc. in Software Engineering
🇨🇦 Canada
Prof. Rachel Anderson holds an M.Sc. in Software Engineering from the University of Toronto and has completed over 600 assignments on Strings. With a focus on user interface development and human-computer interaction, Prof. Anderson excels in formatting strings for output and implementing user-friendly string handling features. Her expertise includes integrating string operations with graphical user interfaces (GUI) and optimizing string formatting for performance.
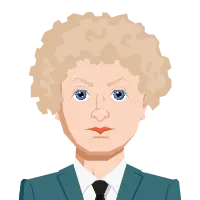
Dr. Oliver Mitchell
Ph.D. in Computer Science
🇦🇺 Australia
Dr. Oliver Mitchell holds a Ph.D. in Computer Science from the University of Melbourne and has successfully completed over 900 assignments on Strings in C. Dr. Mitchell specializes in Unicode handling, multibyte character sets, and internationalization in programming. His expertise extends to implementing secure string manipulation functions and optimizing string search algorithms for diverse applications.
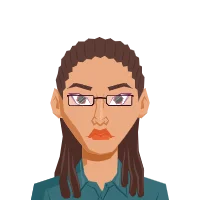
Prof. Elizabeth Taylor
Master's in Computer Engineering
🇬🇧 United Kingdom
Prof. Elizabeth Taylor is a seasoned professional with a Master's degree in Computer Engineering from MIT and has completed over 700 C programming assignments related to Strings. Prof. Taylor's expertise lies in software development methodologies and debugging techniques. She excels in crafting robust solutions for string parsing, tokenization, and implementing custom string functions. Prof. Taylor's dedication to precision and clarity ensures that students receive well-documented and efficient solutions.
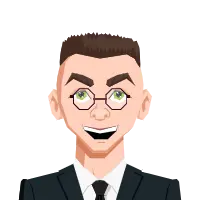
Dr. Benjamin White
Ph.D. in Computer Science
🇺🇸 United States
Dr. Benjamin White holds a Ph.D. in Computer Science from Stanford University and has completed over 800 C programming assignments on Strings. With a specialization in algorithm design and analysis, Dr. White excels in developing efficient solutions for complex programming tasks. His expertise includes string manipulation, dynamic memory allocation, and optimization techniques. Dr. White is committed to delivering high-quality solutions that meet academic standards and exceed expectations.
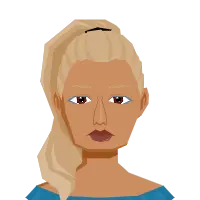
Prof. Isabella Storey
MEd in Computer Science
🇨🇦 Canada
Prof. Isabella Storey, an accomplished educator with an MEd in Computer Science from McGill University, has completed over 600 assignments on Pointers in C. With a teaching tenure at the University of Toronto, Prof. Storey specializes in pointer and array manipulation, pointer arithmetic, and error handling in pointer operations. Her assignments focus on foundational concepts that lay a strong groundwork for advanced C programming skills.
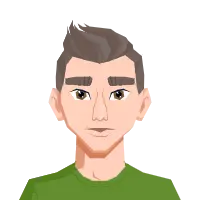
Dr. Liam Davies
PhD in Software Engineering
🇦🇺 Australia
Dr. Liam Davies, with a PhD in Software Engineering from the University of Sydney, has successfully completed more than 900 assignments on Pointers in C. As a seasoned industry practitioner in Melbourne, Dr. Davies specializes in pointer to functions, pointer-based data structures, and real-time applications of pointers. His assignments emphasize practical implementations that bridge theoretical knowledge with industry demands.
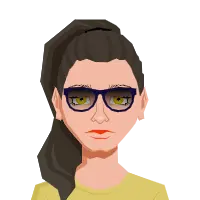
Prof. Rachel Reynolds
MSc in Computer Engineering
🇺🇸 United States
Prof. Rachel Reynolds brings her expertise as a former professor at Harvard University with over 700 completed C assignments on Pointers. Holding an MSc in Computer Engineering from MIT, Prof. Reynolds excels in pointer basics, pointer casting, and the application of pointers in data structures. Her assignments often focus on enhancing pointer efficiency in algorithmic solutions and integrating pointers with object-oriented programming concepts.
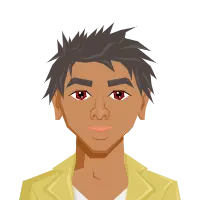
Dr. James Mitchell
Ph.D in Computer Science
🇬🇧 United Kingdom
Dr. James Mitchell holds a PhD in Computer Science from Oxford University and has completed over 800 C programming assignments on Pointers. With a background as a former lecturer at University College London, Dr. Mitchell specializes in dynamic memory allocation, pointer arithmetic, and file handling with pointers. His expertise extends to optimizing memory usage in complex data structures and implementing efficient algorithms using pointers.
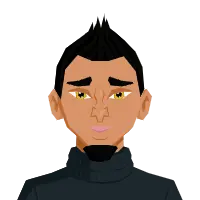
Prof. Ethan Lee
Master's in Computer Science
🇨🇦 Canada
Professor Ethan Lee, with a Master's degree in Computer Science from a prominent university in Canada, has successfully handled over 600 assignments on memory allocation using C. Prof. Lee's expertise spans across teaching fundamental programming concepts, implementing efficient memory allocation strategies, and providing practical solutions to challenging programming assignments.
Related Topics
Frequently Asked Questions
Have questions about our Simulating a Game Using C assignment help? Check out our FAQs section for answers to common queries. If you can't find the information you're looking for, don't hesitate to reach out to us through our live chat support. Our friendly customer service team is here to assist you with any questions or concerns you may have.
Yes, we offer free unlimited revisions to ensure that the final solution meets your expectations. If you need any changes or enhancements to your C game simulation assignment, simply let us know, and we'll make the necessary adjustments until you're satisfied.
Certainly! Debugging is an essential part of the development process, and our experts can assist you in identifying and fixing errors in your C game simulation code. We provide step-by-step guidance to help you overcome any challenges you encounter.
Yes, optimization is a key aspect of game development, and our experts are skilled in identifying and addressing performance bottlenecks in C code. We can help optimize algorithms, data structures, and memory usage to ensure your game runs smoothly.
Absolutely! Our team includes experts in graphics programming who can help with rendering game objects, creating animations, and optimizing performance for your C game simulation assignments.
Yes, our experts are proficient in implementing AI algorithms for game simulations using C. Whether you need simple decision-making logic or more complex behavior trees, we can tailor the solution to meet your specific requirements.