Claim Your Offer
Unlock an amazing offer at www.programminghomeworkhelp.com with our latest promotion. Get an incredible 20% off on your second programming assignment, ensuring top-quality assistance at an affordable price. Our team of expert programmers is here to help you, making your academic journey smoother and more cost-effective. Don't miss this chance to improve your skills and save on your studies. Take advantage of our offer now and secure exceptional help for your programming assignments.
We Accept
- Understanding the Fundamentals of Software Design Patterns
- Introduction to C++: A Brief Overview
- Creational Design Patterns in C++
- 1. Singleton Pattern
- 2. Factory Method Pattern
- 3. Abstract Factory Pattern
- Structural Design Patterns in C++
- 1. Adapter Pattern
- 2. Decorator Pattern
- 3. Composite Pattern
- Behavioral Design Patterns in C++
- 1. Observer Pattern
- 2. Strategy Pattern
- 3. Command Pattern
- Integrating Software Design Patterns into an Academic Curriculum
- 1. Design Pattern Fundamentals in Programming Courses
- 2. Advanced Topics: Design Patterns in Software Engineering Courses
- 3. Design Patterns in Group Projects
- Conclusion
In the ever-evolving landscape of software development, mastering design patterns is paramount for crafting code that is robust, maintainable, and scalable. This blog delves into the realm of software design patterns, focusing specifically on their application in C++. Adopting an academic approach, we explore how educators and students can integrate the study of design patterns into a structured curriculum. Design patterns provide proven solutions to recurring problems in software design, offering a systematic and efficient way to address common challenges. By emphasizing their significance in creating adaptable and high-quality code, this exploration aims to empower developers and learners alike in navigating the complexities of software development. The academic lens ensures a comprehensive understanding, guiding readers through the fundamentals of design patterns and their practical implementations in the C++ programming language. Whether you're an educator shaping the curriculum of future developers or a student eager to enhance your coding skills, this blog serves as a valuable resource for embracing design patterns as a cornerstone of effective software engineering practices. If you need help with your C++ assignment, consider seeking assistance from your instructors, fellow students, or exploring online resources tailored to C++ programming.
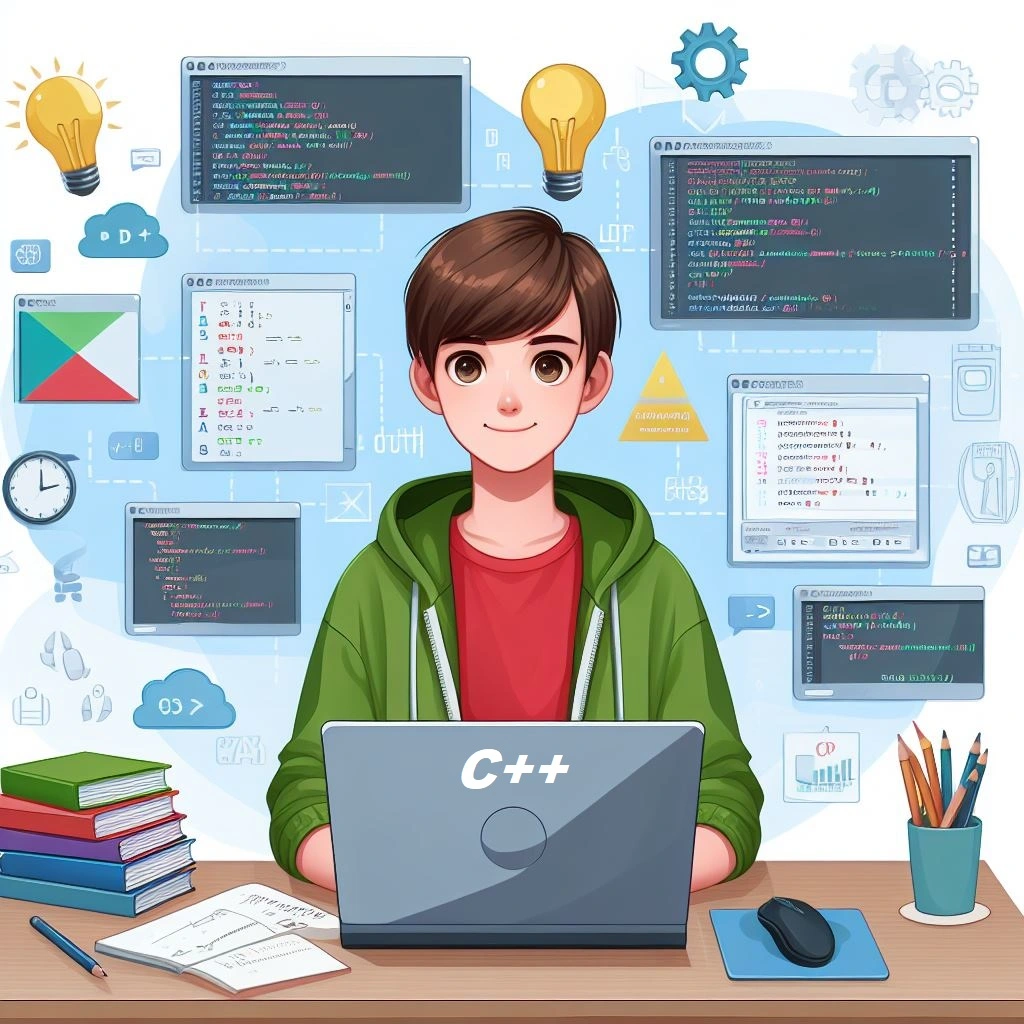
Understanding the Fundamentals of Software Design Patterns
Embarking on the journey into C++ design patterns necessitates a firm grasp of their foundational principles. This section lays the groundwork by exploring the very essence of design patterns — their definition, purpose, and the inherent advantages they bestow upon software development. Delve into the significance of adopting a standardized approach to problem-solving, shedding light on how design patterns become a cornerstone in achieving code reusability. Through a comprehensive exploration of these fundamentals, readers will gain a profound understanding of the theoretical underpinnings that set the stage for the subsequent practical implementations of design patterns in C++.
Introduction to C++: A Brief Overview
This section offers a succinct exploration of the C++ programming language, offering readers a compact yet comprehensive understanding of its essential features. Emphasizing its object-oriented nature, the overview highlights why C++ stands as a popular choice in the realm of software development. Providing insights into the language's strengths, this segment aims to familiarize both novice and experienced developers with the key attributes that contribute to its widespread adoption. For those less acquainted with C++, a brief touch upon its syntax ensures a foundational understanding, setting the stage for a deeper exploration of design patterns within the language's context. Through this concise overview, readers gain valuable insights into the language that serves as the canvas for the subsequent in-depth discussion on design patterns.
Creational Design Patterns in C++
This section delves into the realm of Creational Design Patterns within the context of C++. Creational patterns are instrumental in providing flexible mechanisms for object creation, and in this exploration, readers will navigate through various patterns tailored to address specific challenges in instantiation. From the Singleton Pattern, ensuring a single, globally accessible instance, to the Factory Method Pattern facilitating object creation within a superclass, and the Abstract Factory Pattern handling the creation of related objects without specifying their classes — this segment unravels the intricacies of creational design patterns. With practical examples and insights, readers gain a nuanced understanding of how these patterns contribute to the efficient and effective creation of objects in C++ applications.
1. Singleton Pattern
In the realm of C++, the Singleton pattern stands as a fundamental design pattern with wide-ranging applications. This pattern ensures the creation of a single, globally accessible instance of a class, promoting efficiency and resource management. Beyond its foundational principles, the Singleton pattern finds utility in scenarios where a sole point of control or coordination is imperative. Consider a scenario in a gaming application where a GameManager class controls the state of the game. Implementing the Singleton pattern ensures that there's only one instance of the GameManager, preventing inconsistent states and enabling centralized management.
2. Factory Method Pattern
The Factory Method pattern, a stalwart in object-oriented design, extends its influence in the C++ landscape. This pattern provides a structured approach to creating objects within a superclass while empowering subclasses to alter the types of objects created. The flexibility offered by the Factory Method pattern is particularly valuable in scenarios where a class cannot anticipate the class of objects it needs to create. Imagine a document editing application where various document types are handled. The Factory Method pattern allows each document type to have its own creator, enabling seamless extensibility as new document types are introduced.
3. Abstract Factory Pattern
The Abstract Factory pattern, renowned for its ability to create families of related or dependent objects, finds a rich tapestry of applications within C++ development. Serving as an interface for creating object families without specifying their concrete classes, the Abstract Factory pattern enhances flexibility and scalability. Consider a scenario in an e-commerce system where product families, such as smartphones and laptops, need to be created. The Abstract Factory pattern allows the system to produce products with consistent interfaces while accommodating variations in product types. This dynamic capability ensures adaptability to evolving product lines and market demands, exemplifying the versatility of the Abstract Factory pattern in real-world applications.
Structural Design Patterns in C++
This section navigates through the landscape of Structural Design Patterns in the realm of C++. Structural patterns focus on the composition of classes and objects, providing solutions to form larger structures while maintaining flexibility. Within this exploration, readers will encounter patterns such as the Adapter Pattern, facilitating the collaboration of incompatible interfaces, the Decorator Pattern for dynamically adding new functionalities to objects, and the Composite Pattern for treating individual objects and compositions uniformly. Each pattern is intricately woven into the fabric of C++ programming, showcasing their application in crafting code structures that are both scalable and adaptable. With real-world examples and practical insights, this segment sheds light on how structural design patterns empower developers to architect robust systems in the C++ programming language.
1. Adapter Pattern
The Adapter pattern emerges as a key player in the harmonization of disparate interfaces within C++ development. Its significance lies in bridging the gap between incompatible interfaces, fostering collaboration between components that would otherwise remain isolated. In the intricate dance of software integration, the Adapter pattern acts as a mediator, enabling seamless communication between modules with divergent interfaces. To illustrate its practicality, imagine a legacy system with a well-established interface structure and a new module with a modern, yet incompatible, interface. The Adapter pattern acts as an intermediary, facilitating interaction and ensuring the coexistence of these disparate components. Through code examples and detailed explanations, this section will illuminate the Adapter pattern's transformative role in promoting interoperability within C++ projects.
2. Decorator Pattern
The Decorator pattern, an elegant embellishment in the realm of design patterns, takes center stage in dynamically enhancing object functionalities within C++ applications. Its role extends beyond static configurations, allowing developers to augment object behaviors at runtime. Delving into its intricacies, this section explores scenarios where the Decorator pattern shines with unparalleled brilliance. Picture a graphic design application where users can dynamically apply filters to images. The Decorator pattern empowers developers to seamlessly add new filters without altering the original image classes. As readers traverse through this section, they will not only grasp the theoretical underpinnings of the Decorator pattern but also be guided through practical C++ implementations, unlocking the potential for dynamic functionality enhancement in their own coding endeavors.
3. Composite Pattern
The Composite pattern, a masterful orchestration of individual and composite objects, unveils its prowess in treating diverse elements uniformly within C++ structures. This pattern is a cornerstone in the creation of hierarchies where both individual objects and compositions are seamlessly interchangeable. To exemplify its application, consider a graphic design software where shapes, both simple and complex, need to be manipulated uniformly. The Composite pattern allows the software to treat individual shapes and complex compositions of shapes uniformly, simplifying the codebase and promoting flexibility. This section provides a window into the practicalities of the Composite pattern, guiding readers through a tangible example that vividly illustrates the pattern's ability to unify disparate elements within the fabric of a C++ application.
Behavioral Design Patterns in C++
This section immerses readers in the dynamic realm of Behavioral Design Patterns within the context of C++. Behavioral patterns focus on the interaction and responsibility distribution among objects, offering solutions to effectively manage algorithms, relationships, and communication. Within this exploration, readers will encounter patterns such as the Observer Pattern, facilitating a one-to-many dependency between objects, the Strategy Pattern for defining families of algorithms interchangeably, and the Command Pattern for encapsulating requests as objects. Each pattern in this behavioral tapestry is meticulously tailored to enhance the flexibility and collaboration of objects in C++ applications. Through practical examples and insightful discussions, this segment illuminates the ways in which behavioral design patterns elevate the quality and adaptability of software solutions, providing readers with a nuanced understanding of their application in the C++ programming language.
1. Observer Pattern
The Observer pattern, a linchpin in fostering dynamic relationships between objects, beckons exploration into its intricate workings within the C++ landscape. Serving as the architect of a one-to-many dependency framework, the Observer pattern orchestrates communication among objects in a synchronized dance. To demystify its application, this section weaves through real-world scenarios where dynamic updates and notifications are paramount. C++ code snippets will act as our guide, revealing the Observer pattern's code-symphonies that harmonize disparate objects. By navigating through tangible examples, readers will not only comprehend the theoretical foundation but also gain a practical understanding of how the Observer pattern elevates the connectivity and responsiveness of objects within the C++ ecosystem.
2. Strategy Pattern
The Strategy pattern, a maestro in the symphony of algorithmic design, beckons exploration into its strategic role in defining algorithm families within C++. This section delves into the strategy's artistry, encapsulating each algorithm and allowing for seamless interchangeability. As we traverse through the nuanced landscapes of C++ development, real-world examples will illuminate scenarios where the Strategy pattern orchestrates efficient algorithmic collaborations. Whether optimizing sorting algorithms in a data analytics application or fine-tuning gameplay strategies in a simulation, the Strategy pattern becomes an invaluable ally. Through illustrative narratives and practical examples, this segment unveils the transformative power of the Strategy pattern, showcasing its adaptability and efficiency in enhancing codebase flexibility within the realm of C++.
3. Command Pattern
The Command pattern, a director in orchestrating request encapsulation within C++, unfurls its purpose in this exploration. Beyond the encapsulation of requests as objects, the Command pattern allows for parameterization of clients with varying requests, queuing, and systematic logging. This section delves into real-world scenarios where the Command pattern emerges as a virtuoso conductor, orchestrating complex sequences of actions. Through vivid examples, readers will witness the elegance of the Command pattern, providing clarity in scenarios ranging from user interface interactions to complex systems requiring precise command execution. With detailed explanations and practical implementations in C++, this segment elucidates the role of the Command pattern as a strategic tool in facilitating flexibility, modularity, and comprehensive request management within diverse software architectures.
Integrating Software Design Patterns into an Academic Curriculum
This section delves into the crucial intersection of academia and practical application by exploring the integration of Software Design Patterns into an academic curriculum. As educators shape the learning journey of aspiring developers, the discussion centers on the strategic inclusion of design patterns at various levels of programming courses. From foundational programming courses to advanced software engineering classes, this segment examines the seamless infusion of design pattern fundamentals. Real-world case studies and practical applications underscore the value of hands-on experience in grasping the complexities of design patterns. Additionally, insights are provided on incorporating design patterns into group projects, fostering collaborative learning environments where students can apply theoretical knowledge in a practical context. Through this exploration, educators and students alike gain a roadmap for embracing design patterns as an integral component of academic programming, ensuring a holistic and applicable understanding for the next generation of software developers.
1. Design Pattern Fundamentals in Programming Courses
The integration of design pattern fundamentals into introductory programming courses marks a paradigm shift in shaping the programming mindset of aspiring developers. This section unravels the importance of instilling design pattern fundamentals from the outset, fostering a proactive problem-solving approach. As students embark on their programming journey, understanding and applying design patterns become pillars of proficiency. Early exposure not only nurtures a design-centric mindset but also equips learners with a robust toolkit for tackling real-world coding challenges. By delving into the significance of this foundational knowledge, educators can sculpt a generation of developers poised to architect efficient and scalable solutions, laying the groundwork for a lifetime of strategic coding excellence.
2. Advanced Topics: Design Patterns in Software Engineering Courses
Transitioning from fundamentals to the advanced echelons of software engineering courses, this segment embarks on a nuanced exploration of design patterns. As students ascend the academic ladder, design patterns cease to be mere coding techniques; they become architectural keystones in building complex and scalable systems. Real-world applications and case studies take center stage, offering students insights into the pragmatic deployment of design patterns in industry settings. By immersing students in these advanced topics, educators bridge the gap between theoretical knowledge and practical application, nurturing a cohort of software engineers adept at leveraging design patterns as indispensable tools in their professional arsenal.
3. Design Patterns in Group Projects
Guiding the educational journey into collaborative terrain, this section advocates for the integration of design patterns in group projects. As educators sculpt multifaceted learning experiences, group projects focused on design patterns emerge as crucibles for practical application. Students traverse beyond theoretical realms, applying their acquired knowledge in a hands-on, collaborative environment. The synthesis of diverse perspectives in group projects not only enriches the educational experience but also mirrors the collaborative nature of real-world software development. Through insightful guidance, educators empower students to seamlessly integrate design patterns into project architectures, fostering a holistic understanding that transcends individual prowess, ultimately sculpting agile and collaborative software engineers ready to navigate the complexities of the professional arena.
Conclusion
In conclusion, this blog has navigated through the intricacies of software design patterns, underscoring their pivotal role in the realm of C++ programming. The discussion has spotlighted the significance of adopting an academic curriculum-based approach to studying these patterns, providing a structured foundation for educators and students alike. By summarizing the key takeaways, it becomes evident that design patterns are not mere coding techniques but rather essential tools for creating robust, maintainable, and scalable software solutions. The academic approach advocated in this exploration ensures a systematic understanding, enabling the next generation of software developers to tackle real-world challenges with confidence. As we wrap up, it's crucial to recognize that the journey of mastering design patterns is ongoing. Encouraging readers to persist in their exploration and practical application of design patterns, this conclusion serves as a call to action. Embrace the knowledge gained here, continue delving into the intricacies of C++ design patterns, and witness the transformative impact these principles can have on elevating your coding practices to new heights.