Claim Your Offer
Unlock an amazing offer at www.programminghomeworkhelp.com with our latest promotion. Get an incredible 20% off on your second programming assignment, ensuring top-quality assistance at an affordable price. Our team of expert programmers is here to help you, making your academic journey smoother and more cost-effective. Don't miss this chance to improve your skills and save on your studies. Take advantage of our offer now and secure exceptional help for your programming assignments.
We Accept
Object-oriented programming (OOP) is not merely a programming paradigm; it's a mindset, a methodology, and a powerful tool for software development. In the realm of programming languages, C++ stands tall as a stalwart supporter of OOP principles, offering students a robust platform to grasp the intricacies of this paradigm. As students embark on their journey into the world of programming, understanding OOP in C++ is akin to unlocking the door to a treasure trove of possibilities. This comprehensive guide serves as a beacon, illuminating the path for students seeking assistance to solve their C++ Assignment.
From the fundamental concepts to advanced techniques, this guide aims to demystify OOP and provide students with the knowledge and skills necessary to navigate the complexities of modern software development. Whether you're a novice programmer taking your first steps into the world of coding or a seasoned developer looking to broaden your horizons, this guide offers invaluable insights and practical examples to help you master OOP in C++. By delving into the core principles of encapsulation, inheritance, and polymorphism, students will gain a deeper understanding of how to design modular, maintainable, and scalable software solutions. With real-world examples and hands-on exercises, this guide empowers students to apply OOP concepts in their projects with confidence and competence. So, let's embark on this journey together, unraveling the mysteries of object-oriented programming in C++ and unlocking the door to a world of endless possibilities in software development.
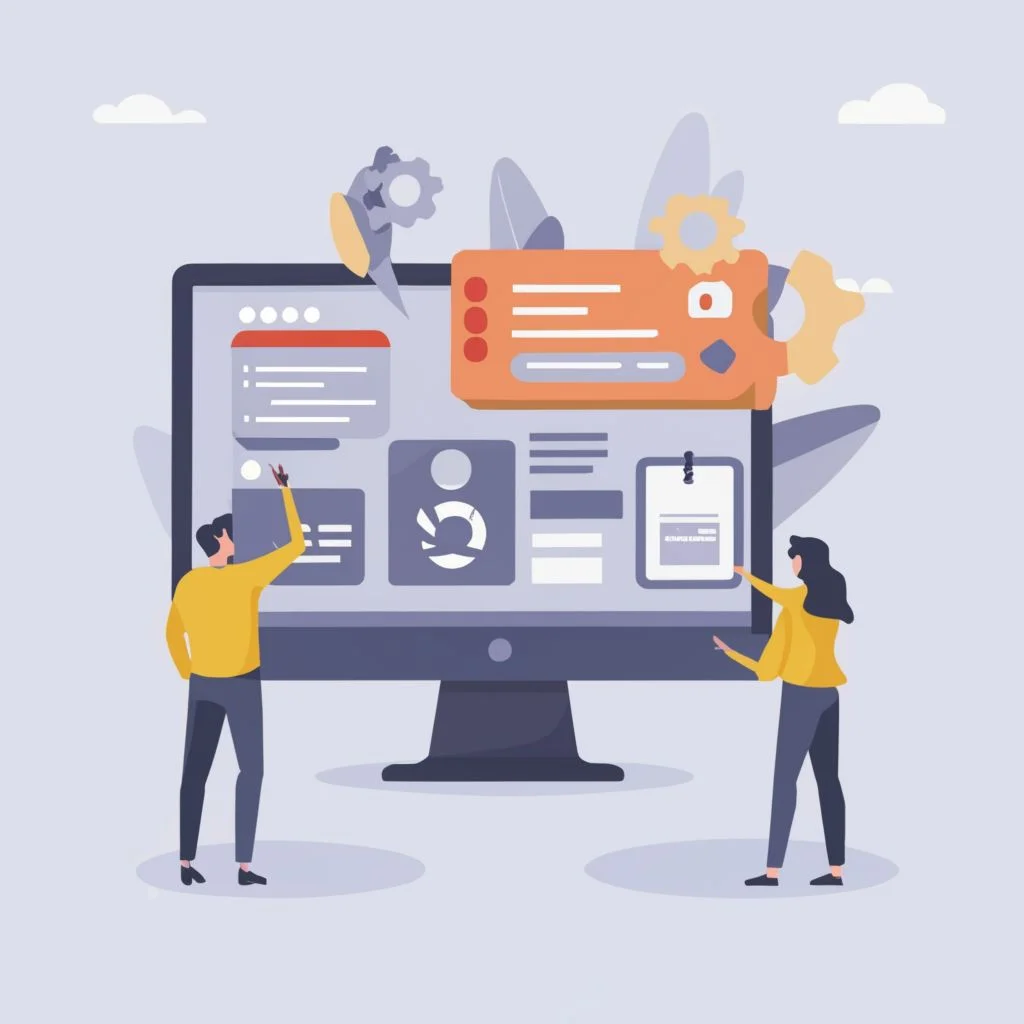
As we delve deeper into the realm of object-oriented programming (OOP) in C++, it's crucial to understand the underlying principles that form the foundation of this paradigm. At its core, OOP revolves around the concept of objects, which are instances of classes encapsulating data and behaviors. C++, with its rich set of features, provides a fertile ground for implementing OOP concepts effectively. By embracing OOP, students gain the ability to design modular, reusable, and extensible code, which is essential for tackling complex software projects. Moreover, OOP promotes code organization, enhances code readability, and facilitates collaboration among developers.
As students embark on their journey to master OOP in C++, they'll encounter a myriad of concepts, ranging from classes and objects to inheritance and polymorphism. Each concept serves as a building block, contributing to the overarching goal of writing efficient and maintainable code. Through hands-on practice and experimentation, students will not only sharpen their programming skills but also cultivate a deeper understanding of software design principles. This guide serves as a roadmap, guiding students through the intricate landscape of OOP in C++ and equipping them with the tools and knowledge needed to succeed in today's competitive software industry. So, let's dive in and explore the fascinating world of object-oriented programming in C++, where creativity meets functionality, and innovation knows no bounds.
Introduction to Object-Oriented Programming
Object-oriented programming (OOP) is a paradigm that has profoundly influenced the landscape of software development, offering a powerful methodology for organizing and managing code. At its core, OOP revolves around the concept of objects, which are instances of classes representing tangible entities or abstract concepts within a program. These objects encapsulate both data, in the form of attributes or member variables, and behaviors, encapsulated within methods or member functions. By encapsulating data and methods within objects, OOP promotes modularity, reusability, and scalability, fostering a more intuitive and organized approach to software design.
The cornerstone of OOP is the class, which serves as a blueprint or template for creating objects. Classes encapsulate the structure and behavior of objects, defining their properties and methods. Through the process of instantiation, objects are created based on these class definitions, inheriting their attributes and behaviors. This concept of instantiation enables developers to create multiple instances of the same class, each with its unique state and behavior, facilitating code reuse and promoting efficiency in software development.
One of the key principles of OOP is encapsulation, which entails bundling data and methods within objects and restricting access to them from external entities. Encapsulation fosters data hiding and abstraction, shielding the internal implementation details of a class from external manipulation. By enforcing access controls through access specifiers such as public, private, and protected, developers can regulate the visibility of class members, enhancing code security and maintainability.
Inheritance is another fundamental concept in OOP, enabling classes to inherit properties and behaviors from their parent classes. Through inheritance, developers can create hierarchical relationships between classes, with derived classes inheriting attributes and methods from their base classes. This promotes code reuse, as common functionalities can be defined in base classes and inherited by subclasses. Moreover, inheritance facilitates the creation of specialized classes that extend the functionality of their parent classes, allowing for flexible and extensible code designs.
Polymorphism, the third pillar of OOP, empowers objects to exhibit different forms or behaviors based on their underlying types or contexts. In C++, polymorphism is achieved through virtual functions and function overriding, allowing for dynamic dispatch and runtime determination of function implementations. This enables developers to write code that operates on objects of different types in a uniform manner, promoting flexibility and adaptability in code design.
Object-oriented programming in C++ provides a robust framework for developing complex and scalable software solutions. By embracing the principles of encapsulation, inheritance, and polymorphism, developers can create modular, maintainable, and extensible codebases. Through the use of classes, objects, and inheritance hierarchies, developers can model real-world entities and abstract concepts in a clear and intuitive manner. As students delve into the world of OOP in C++, they will gain invaluable insights into software design principles and practices, empowering them to tackle a wide range of programming challenges with confidence and proficiency.
Understanding Classes and Objects in C++
In the realm of C++ programming, classes and objects serve as fundamental building blocks, laying the groundwork for the implementation of object-oriented principles. A class in C++ is a user-defined data type that encapsulates both data members (attributes) and member functions (methods), embodying the essence of abstraction and encapsulation. With the class keyword, developers can declare a class, specifying its properties and behaviors within the curly braces that define its scope. Each instance of a class is referred to as an object, representing a unique entity with its own set of attributes and behaviors. Objects are instantiated using the new keyword or by directly declaring them, allowing developers to create multiple instances of a class within their programs.
One of the key advantages of using classes and objects in C++ is the concept of encapsulation, which promotes data hiding and abstraction. Encapsulation involves bundling the data members and member functions of a class together, thus restricting access to the internal implementation details of the class. By declaring certain members as private within the class definition, developers can prevent external entities from directly accessing or modifying the underlying data, ensuring data integrity and security. This encapsulation mechanism fosters modular and maintainable code, as changes to the internal implementation of a class do not affect its external interface.
C++ provides access specifiers such as public, private, and protected, which control the visibility of class members within the class hierarchy. Public members are accessible from outside the class and are often used to define the interface through which external entities interact with the class. Private members, on the other hand, are accessible only within the class itself and are hidden from external access, thereby enforcing encapsulation. Protected members, a hybrid between public and private, are accessible within the class and its derived classes, facilitating the implementation of inheritance.
Inheritance, another fundamental concept in object-oriented programming, allows classes to inherit properties and behaviors from their parent classes. In C++, inheritance is achieved using the extends keyword, with derived classes inheriting the attributes and methods of their base classes. This facilitates code reuse and promotes a hierarchical structure in class relationships, as derived classes can build upon the functionality provided by their base classes. Furthermore, C++ supports both single and multiple inheritance, enabling a derived class to inherit from multiple base classes. While inheritance promotes code reuse, developers must carefully consider the design and implementation of inheritance hierarchies to avoid issues such as the diamond problem and tight coupling.
C++ supports the concept of constructors and destructors, which are special member functions responsible for initializing and destroying objects, respectively. Constructors are invoked automatically when an object is created, allowing developers to initialize the object's data members and perform any necessary setup tasks. Destructors, on the other hand, are called when an object is destroyed or goes out of scope, enabling cleanup operations such as releasing resources or deallocating memory. By leveraging constructors and destructors, developers can ensure the proper initialization and cleanup of objects within their programs.
Understanding classes and objects in C++ is essential for mastering object-oriented programming principles and designing robust and scalable software solutions. By harnessing the power of encapsulation, inheritance, constructors, and destructors, developers can create modular, maintainable, and extensible code that meets the demands of complex real-world projects. From encapsulating data and behaviors within classes to leveraging inheritance hierarchies and constructor/destructor semantics, the concepts of classes and objects form the cornerstone of effective C++ programming practices. As students continue to explore and apply these concepts in their programming endeavors, they will unlock new levels of efficiency, flexibility, and innovation in software development.
Exploring Inheritance and Polymorphism
Inheritance and polymorphism are two fundamental pillars of object-oriented programming that empower developers to create flexible, reusable, and extensible code. Inheritance, often described as an "is-a" relationship, allows classes to inherit properties and behaviors from their parent classes, fostering code reusability and promoting a hierarchical structure in class relationships. In C++, inheritance is implemented using the extends keyword, where derived classes inherit from base classes, gaining access to their member variables and functions. This inheritance hierarchy enables developers to model real-world relationships and hierarchies effectively, facilitating the organization and maintenance of complex codebases. C++ supports multiple inheritance, where a class can inherit from multiple base classes, although this feature requires careful consideration to avoid ambiguity and maintain code clarity.
Polymorphism, on the other hand, enables objects to exhibit different forms based on their underlying types or contexts, promoting flexibility and adaptability in code design. There are two main types of polymorphism in C++: compile-time polymorphism (also known as static polymorphism) and runtime polymorphism (dynamic polymorphism). Compile-time polymorphism is achieved through function overloading and operator overloading, where multiple functions or operators share the same name but differ in their parameter types or number. This allows developers to write concise and expressive code by providing multiple implementations of a function or operator for different data types or argument combinations. Runtime polymorphism, on the other hand, is facilitated through virtual functions and function overriding, enabling dynamic dispatch of function calls based on the runtime type of objects. By declaring a function as virtual in a base class and overriding it in derived classes, developers can achieve runtime polymorphism, where the appropriate function implementation is determined dynamically at runtime based on the actual type of the object being referenced. This powerful feature enables developers to write generic code that can work with objects of different types, promoting code reuse, extensibility, and maintainability.
In practice, inheritance and polymorphism often work hand in hand to enable sophisticated code designs and implementations. By leveraging inheritance, developers can create a hierarchy of classes that share common properties and behaviors, while polymorphism allows them to write code that operates on objects of the base class type but behaves differently depending on the actual derived class type. This enables developers to write code that is more flexible, adaptable, and scalable, as it can accommodate changes and additions to the codebase without requiring extensive modifications. Moreover, inheritance and polymorphism facilitate the implementation of design patterns such as the factory pattern, adapter pattern, and strategy pattern, which encapsulate common solutions to recurring design problems and promote code reuse and maintainability.
Inheritance and polymorphism are indispensable tools in the toolkit of any C++ programmer, enabling them to create elegant, modular, and maintainable code. By understanding the principles of inheritance and polymorphism and their implementation in C++, developers can unlock the full potential of object-oriented programming and tackle complex software projects with confidence and efficiency. Whether designing class hierarchies, implementing generic algorithms, or applying design patterns, inheritance and polymorphism offer a powerful combination of features that empower developers to write code that is not only functional and efficient but also flexible, extensible, and easy to maintain.
Applying OOP Principles in C++ Projects
Applying object-oriented programming (OOP) principles in C++ projects is not just about understanding the theory; it's about applying these principles effectively to solve real-world problems and create robust, maintainable software solutions. One of the key principles, encapsulation, serves as a cornerstone for building reliable software systems. By encapsulating data within class structures and controlling access to it through well-defined interfaces, developers can ensure data integrity and prevent unintended modifications, thus enhancing the robustness and reliability of their codebases. Moreover, encapsulation fosters modularity, allowing developers to isolate changes within specific components of the system without affecting other parts—a crucial aspect of large-scale software development.
Inheritance, another fundamental concept in OOP, plays a pivotal role in code reuse and organization. In C++, developers can leverage inheritance to create hierarchical relationships between classes, where derived classes inherit properties and behaviors from their parent classes. This promotes code reuse by eliminating redundant implementations and fosters a structured approach to software design. By defining common functionalities in base classes and extending them in derived classes, developers can streamline the development process and ensure consistency across the codebase. However, it's important to exercise caution when using inheritance to avoid tight coupling and maintain flexibility in the design.
Polymorphism, the third pillar of OOP, empowers developers to write flexible and adaptable code that can handle varying object types and behaviors seamlessly. In C++, polymorphism is typically achieved through virtual functions and function overriding. By declaring functions as virtual in base classes and providing different implementations in derived classes, developers can enable dynamic dispatch, where the appropriate function implementation is determined at runtime based on the object's type. This facilitates code extensibility and promotes code reuse by allowing developers to write generic algorithms that can operate on objects of different types without modification.
Beyond these core principles, effective application of OOP in C++ projects involves careful design considerations and architectural decisions. Design patterns, such as the Factory Method, Singleton, and Observer patterns, offer standardized solutions to common design problems and promote code maintainability and scalability. By understanding and applying these design patterns, developers can streamline the development process, improve code readability, and facilitate future modifications and enhancements. Additionally, adopting agile development methodologies, such as Scrum or Kanban, can help teams iterate quickly, gather feedback, and adapt to changing requirements, ensuring that OOP principles are applied iteratively and effectively throughout the project lifecycle.
Applying OOP principles in C++ projects requires more than just theoretical knowledge—it demands practical application and thoughtful consideration of design choices. By embracing encapsulation, inheritance, and polymorphism, developers can build robust, maintainable, and scalable software solutions that meet the evolving needs of users and stakeholders. Moreover, by incorporating design patterns and agile methodologies into their development process, teams can streamline their workflows, enhance collaboration, and deliver high-quality software that stands the test of time. As students and aspiring programmers continue to refine their skills in OOP and C++, they will unlock new opportunities for innovation and creativity in software development.
Conclusion
In the vast landscape of programming, object-oriented programming (OOP) stands as a cornerstone principle, offering a paradigm shift in software development methodologies. Throughout this guide, we've delved into the intricate world of OOP in C++, unraveling its fundamental concepts and exploring their practical applications. From the foundational understanding of classes and objects to the advanced principles of inheritance and polymorphism, students have gained valuable insights into the power and versatility of OOP in C++.
As students journey through their exploration of OOP in C++, they'll encounter myriad challenges and opportunities for growth. Each concept covered in this guide serves as a building block, paving the way for deeper comprehension and mastery of C++ programming. Encapsulation, with its emphasis on data hiding and abstraction, fosters the creation of robust and secure software systems. Inheritance enables the efficient reuse of code and promotes code organization, laying the groundwork for scalable and maintainable projects. Polymorphism, with its ability to adapt and evolve, empowers developers to create flexible and extensible solutions, capable of meeting the dynamic demands of modern software development.
Beyond the theoretical understanding of OOP principles, students must embrace a hands-on approach, applying their knowledge to real-world projects. Through experimentation and practice, students refine their problem-solving skills and cultivate a deeper intuition for effective software design. By immersing themselves in C++ projects that leverage OOP, students gain practical experience in crafting elegant and efficient solutions to complex problems.
The journey of learning OOP in C++ extends far beyond the confines of individual projects. It is a journey of continuous growth and exploration, fueled by curiosity and a passion for programming. As students encounter new challenges and technologies, they must remain adaptable and open-minded, embracing the evolving landscape of software development. Whether it's mastering the intricacies of template metaprogramming or exploring the emerging paradigms of machine learning and artificial intelligence, the principles of OOP serve as a guiding light, empowering students to navigate the ever-changing currents of technology with confidence and resilience.
In essence, mastering object-oriented programming in C++ is not merely about acquiring technical proficiency; it's about cultivating a mindset of creativity, innovation, and lifelong learning. It's about embracing the journey of becoming a skilled craftsman in the art of software development, continually honing one's skills and pushing the boundaries of what's possible. As students embark on this journey, they join a vibrant community of developers, educators, and innovators, united by a shared passion for harnessing the power of technology to shape the world around us.
In conclusion, the path to mastery in object-oriented programming in C++ is challenging yet immensely rewarding. With dedication, perseverance, and a thirst for knowledge, students can unlock endless opportunities for personal and professional growth. As they navigate the complexities of OOP principles and apply them in their projects, they lay the foundation for a fulfilling and impactful career in software development. So, let us embrace this journey wholeheartedly, embracing the transformative power of OOP in C++ to create a brighter and more connected future for generations to come.