Claim Your Offer
Unlock an amazing offer at www.programminghomeworkhelp.com with our latest promotion. Get an incredible 20% off on your second programming assignment, ensuring top-quality assistance at an affordable price. Our team of expert programmers is here to help you, making your academic journey smoother and more cost-effective. Don't miss this chance to improve your skills and save on your studies. Take advantage of our offer now and secure exceptional help for your programming assignments.
We Accept
Access Our Free Linked List Assignment Samples
Access Our Free Linked List Assignment Samples at programminghomeworkhelp.com. Get help with Linked List assignment concepts from Linked List assignment experts. Our programming homework help includes original and A+ grade assurance. Explore examples to understand Linked List assignments better and excel in your studies.
Data Structures and Algorithms
Our Affordable Pricing for Expert Linked List Assignment Help
Explore Our Affordable Pricing for Expert Linked List Assignment Help at programminghomeworkhelp.com. We offer competitive rates for help with Linked List assignment tasks, ensuring top-notch solutions from Linked List assignment experts. Our programming homework help service guarantees affordability without compromising on quality. Whether you need assistance with Linked List assignments or other programming tasks, our transparent pricing ensures you receive value for your investment. Contact us today to discuss your Linked List assignment needs and benefit from our cost-effective solutions.
Description | Price Range |
Basic Linked List Operations | $20 - $40 |
Intermediate Linked List Algorithms | $45 - $70 |
Advanced Linked List Implementations | $75 - $120 |
Comprehensive Linked List Projects | $150 - $250+ |
Expedited Assignment Service (Additional 50%) | Variable |
Technical Support, Optimization, and Consultation | $25 - $60 per hour |
- What is Linked List?
- Creating and Traversing a Linked List
- Linked List Assignment Help
- Why Online Linked List Assignment Help is Ideal for Students?
- Unique Features of Our Linked List Assignment Help
- Comprehensive Coverage of Topics in Our Linked List Assignment Help
- Simple Steps to Accessing Our Linked List Assignment Help Service
- 50% Discount Available on Linked List Assignment Help through Our Referral Program
What is Linked List?
A linked list is a fundamental data structure in computer science used to store a sequence of elements called nodes. Each node contains two main parts: data and a reference (or link) to the next node in the sequence. This structure allows for dynamic memory allocation, unlike arrays which have fixed sizes.
In linked list assignment help contexts, understanding linked lists is crucial for mastering data structures and algorithms. A linked list assignment expert can provide help with linked list assignment tasks, guiding students through concepts like insertion, deletion, traversal, and sorting within linked lists. These assignments often require applying theoretical knowledge to practical programming scenarios, ensuring students grasp the complexities and nuances of data manipulation.
If you are worried about who will do my linked list assignment? At programminghomeworkhelp.com, our experts specialize in offering comprehensive programming homework help services, including dedicated support for understanding and implementing linked lists. Whether you're learning the basics or tackling advanced topics, our resources and assistance ensure clarity and proficiency in handling linked list assignments effectively.
Creating and Traversing a Linked List
# Node class to define nodes of the linked list
class Node:
def __init__(self, data):
self.data = data
self.next = None
# LinkedList class for operations on the linked list
class LinkedList:
def __init__(self):
self.head = None
# Method to traverse and print the linked list
def print_list(self):
current = self.head
while current:
print(current.data, end=" ")
current = current.next
print()
# Example usage
if __name__ == "__main__":
# Creating a linked list with three nodes
linked_list = LinkedList()
linked_list.head = Node(1)
second_node = Node(2)
third_node = Node(3)
linked_list.head.next = second_node
second_node.next = third_node
# Printing the linked list
linked_list.print_list()
In this example:
- We define a Node class to create nodes with data and a next pointer.
- The LinkedList class manages operations such as creating the list and printing its elements.
- We create a linked list with nodes containing integers 1, 2, and 3, and then print the list using the print_list() method.
This code demonstrates basic operations of creating and traversing a singly linked list in Python.
Linked List Assignment Help
Looking for Linked List Assignment Help? Our platform, programminghomeworkhelp.com, offers expert assistance for students needing help with linked list assignments. Our linked list assignment experts are proficient in guiding you through various aspects of linked lists, from basic operations to advanced algorithms. Whether you're struggling with insertion, deletion, traversal, or sorting within linked lists, our team ensures comprehensive support tailored to your academic needs. With programming homework help services designed to enhance your understanding and proficiency, we guarantee clarity and excellence in every assignment.
If you are worried about who will do my linked list assignment? Trust our linked list assignment experts to deliver accurate solutions and clear explanations, helping you achieve academic success. Our programming expert team is adept at handling various operations on linked lists, from inserting a node in the list that points to the next node to efficiently removing elements and deleting nodes. We ensure you grasp the concept of each pointer to the next node and its applications in real world scenarios. Contact us today to explore how we can assist you in mastering linked lists and advancing your programming skills effectively.
Why Online Linked List Assignment Help is Ideal for Students?
Online Linked List Assignment Help is ideal for students due to the complexity of linked list assignments. Our platform, programminghomeworkhelp.com, connects students with experienced linked list assignment experts who simplify intricate concepts like insertion, deletion, and traversal. These assignments often require understanding algorithms and data structures, where our experts excel. By offering help with linked list assignments online, we ensure convenience and accessibility, allowing students to receive guidance tailored to their learning pace and schedule.
Students benefit from clear explanations and step-by-step solutions, enhancing their grasp of programming homework concepts. If you are worried about who will do my linked list assignment? Trust our platform to provide reliable programming homework help that supports your academic goals effectively.
- Complex Concepts: Understanding intricate concepts like insertion, deletion, and traversal in linked list assignments can be daunting without expert guidance.
- Algorithmic Challenges: Solving algorithms related to linked lists requires deep understanding and proficiency, which may overwhelm students.
- Time Constraints: Balancing programming homework deadlines with other academic commitments can be stressful and challenging.
- Lack of Clarity: Without clear explanations and examples, students may struggle to grasp fundamental linked list assignment concepts.
- Pressure to Perform: The pressure to excel academically while mastering complex programming tasks like linked lists can be overwhelming for students.
Unique Features of Our Linked List Assignment Help
Discover the unique features of our Linked List Assignment Help at programminghomeworkhelp.com. We offer personalized help with linked list assignment tasks delivered by experienced linked list assignment experts. Our service stands out with tailored solutions for insertion, deletion, traversal, and other complexities of programming homework related to linked lists. Students benefit from clear explanations, step-by-step guidance, and adherence to academic standards.
Whether you're facing algorithmic challenges or time constraints, our linked list assignment experts ensure comprehensive support to enhance your understanding and academic performance. Experience the difference with our specialized approach to programming homework help that prioritizes your success. If you are worried about who will do my linked list assignment? We are here to help you every step of the way!
- Expert Guidance: Receive assistance from experienced linked list assignment experts who provide thorough explanations and solutions tailored to your needs.
- Timely Delivery: Benefit from prompt delivery of solutions, meeting deadlines and ensuring you stay on track with your academic schedule.
- 24/7 Availability: Access support anytime with our round-the-clock customer service, ready to address your queries and provide immediate assistance.
- Affordable Pricing:Enjoy competitive and transparent pricing with no hidden costs, making our service accessible to all students.
- Plagiarism-Free Solutions: Receive original and unique solutions for linked list assignments, ensuring academic integrity.
Comprehensive Coverage of Topics in Our Linked List Assignment Help
Our Linked List Assignment Help at programminghomeworkhelp.com ensures comprehensive coverage of all essential linked list assignment topics. From basic operations like insertion and deletion to advanced concepts such as traversal methods and sorting algorithms, our linked list assignment experts offer meticulous guidance. We cater to diverse academic needs with a focus on clarity and understanding, helping students navigate complexities in programming homework effectively. Whether it's understanding the singly linked list, doubly linked list, or how each node in the listpoints to the next node and to the previous node, our experts are here to help.
We delve into programming languages to create a linked list and explore the nuances of this linear data structure through each coding assignment. Whether you need help with linked list assignment tasks or seek expertise in handling algorithmic challenges, our service guarantees thorough assistance and timely delivery of solutions. If you are worried about who will do my linked list assignment? Trust us to enhance your knowledge and academic performance in linked lists and related programming concepts.
- Insertion in Linked Lists: Learn methods for adding new elements efficiently into different positions within a linked list, ensuring proper pointer adjustments.
- Deletion Operations: Understand techniques for removing nodes from linked lists, including strategies for handling edge cases and updating pointers.
- Traversal Methods: Explore algorithms for accessing and navigating through linked list elements, such as iterative and recursive approaches for optimal efficiency.
- Sorting Algorithms: Study various sorting techniques applicable to linked lists, such as merge sort and insertion sort, tailored for non-contiguous data structures.
- Circular Linked Lists: Discover the implementation and advantages of circular linked lists, including applications in managing circular data structures and algorithms.
Simple Steps to Accessing Our Linked List Assignment Help Service
Accessing our Linked List Assignment Help service at programminghomeworkhelp.com is straightforward. Simply submit your help with linked list assignment request outlining your requirements. Our dedicated linked list assignment experts will promptly review your needs and provide tailored solutions. We ensure clarity and efficiency in every step, from initial inquiry to final delivery.
Experience seamless assistance for all aspects of programming homework, including insertion, deletion, and traversal in linked lists. If you are worried about who will do my linked list assignment? Trust our platform to enhance your understanding and achieve academic success with comprehensive programming homework help.
- Submit Your Assignment Details: Fill out our straightforward form at programminghomeworkhelp.com, detailing your linked list assignment help requirements, such as tasks and deadlines.
- Receive a Quote: Our system promptly generates a competitive quote based on the complexity and urgency of your help with linked list assignment needs.
- Make Payment: Securely pay using our encrypted platform, ensuring confidentiality and peace of mind for your transaction.
- Assignment Processing: Our linked list assignment experts begin working on your task, employing their expertise in programming concepts and algorithms.
- Receive Completed Assignment: Get notified once your assignment is ready, reviewed for accuracy, and delivered promptly to meet your academic deadlines.
50% Discount Available on Linked List Assignment Help through Our Referral Program
Enjoy an exclusive opportunity to save big on Linked List Assignment Help through our referral program at programminghomeworkhelp.com. When you refer a friend or classmate to our service for help with linked list assignment, both you and your referral will receive a generous 50% discount on your next assignments. Our linked list assignment experts ensure top-notch quality and timely delivery, handling all aspects of linked lists with precision. Whether you need assistance with insertion, deletion, traversal methods, or other programming homework challenges, our team is here to support you.
To avail of this offer, simply share your referral link or code with others in your network. Once they sign up and use our services, both parties will automatically qualify for the discount. This program not only helps you save on academic assistance but also rewards you for recommending our trusted programming homework help service to peers. Don’t miss out on this chance to excel academically while enjoying significant savings with our referral program for Linked List Assignment Help.
Learn More with Our Informative Linked List Assignment Help Blogs
Learn more with our informative Linked List Assignment Help blogs. Our Linked List assignment help articles provide valuable insights and tips from experienced linked list assignment helpers. Whether you need help with linked list assignment concepts, detailed guides, or problem-solving techniques, our blogs cover it all. Stay updated with the latest trends and best practices in linked list assignment help to enhance your understanding and academic performance. Dive into our resources today and boost your proficiency in Linked List assignments.
Read Student Reviews on Our Linked List Assignment Help
Read student reviews on our Linked List Assignment Help services. Discover how our linked list assignment helpers have assisted students with help with linked list assignment tasks. Gain insights into their experiences and successes, and learn why students trust us for linked list assignment help. Our testimonials reflect the quality, reliability, and dedication we bring to every linked list assignment we handle. Join satisfied students who have achieved academic excellence with our expert assistance in Linked List assignments.
Connect with Expert Linked List Assignment Help Professionals
Connect with expert Linked List assignment help professionals for comprehensive assistance. Our linked list assignment helpers offer personalized help with linked list assignment tasks, ensuring clarity and understanding. Whether you need guidance on concepts or detailed solutions, our linked list assignment help professionals are here to support your academic journey. Benefit from their experience and expertise to excel in your programming studies. Contact us today to get started with our dedicated team of linked list assignment helpers.
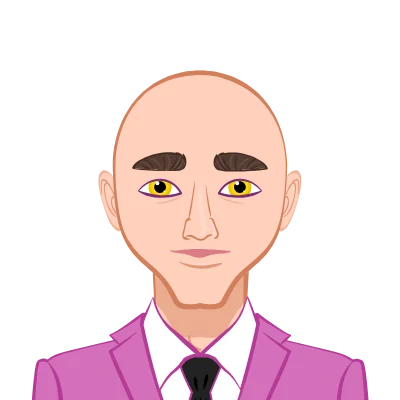
Hayden Ball
Ph.D. in Computer Science
🇬🇧 United Kingdom
Hayden Ball, Ph.D., brings 10 years of expertise in Linked List Assignments. Educated at the University of Warwick, UK, Hayden excels in data structures and algorithmic implementations.
Related Topics
Frequently Asked Questions (FAQs)
Explore our Frequently Asked Questions (FAQs) to learn more about our linked list assignment help services. Find answers regarding help with linked list assignment, our linked list assignment helpers, and how we ensure quality and satisfaction. Our FAQs cover common queries to provide clarity on our processes, pricing, and guarantees. If you have questions about linked list assignment help, chances are, you'll find the answers here. Dive into our FAQs to understand how we can assist you with your programming needs effectively and reliably.
Experienced professionals and programmers who specialize in data structures and algorithms offer Linked List Assignment Help.
Simply contact us via our website, provide details of your assignment, and our team will assist you promptly.
Yes, our helpers have extensive experience, advanced degrees, and proven track records in handling linked list assignments.