Claim Your Offer
Unlock an amazing offer at www.programminghomeworkhelp.com with our latest promotion. Get an incredible 10% off on your all programming assignment, ensuring top-quality assistance at an affordable price. Our team of expert programmers is here to help you, making your academic journey smoother and more cost-effective. Don't miss this chance to improve your skills and save on your studies. Take advantage of our offer now and secure exceptional help for your programming assignments.
We Accept
Examine Top-Quality Algorithm Assignment Samples
Browse our samples to gain insights into expertly crafted solutions for Design and Analysis of Algorithms assignments. Our curated examples, prepared by Design and Analysis of Algorithms experts, offer a clear understanding of complex algorithms and their applications. Whether you need help with dynamic programming, graph algorithms, or computational complexity, our samples provide valuable guidance to enhance your learning and academic performance. Discover practical solutions and refine your algorithm analysis skills with our comprehensive samples.
Data Structures and Algorithms
Data Structures and Algorithms
Data Structures and Algorithms
Data Structures and Algorithms
Data Structures and Algorithms
Data Structures and Algorithms
Data Structures and Algorithms
Java
C++
Data Structures and Algorithms
Data Structures and Algorithms
Data Structures and Algorithms
Data Structures and Algorithms
Data Structures and Algorithms
Data Structures and Algorithms
Get Quality Design and Analysis of Algorithms Assignment Help at a Fair Price
Our Design and Analysis of Algorithms Assignment Help service offers competitive pricing tailored to fit student budgets without compromising on quality. Get the assistance you need with your Design and Analysis of Algorithms Assignment from seasoned experts. If you are asking yourself, "Who will do my Design and Analysis of Algorithms Assignment?" Rest assured, our team of Design and Analysis of Algorithms experts is here to deliver top-notch solutions, ensuring your academic success.
Assignment Type | Turnaround Time | Price (USD) |
---|---|---|
Algorithm Analysis | 2 days | $50 |
Sorting Algorithms | 3 days | $70 |
Dynamic Programming | 4 days | $90 |
Graph Algorithms | 5 days | $110 |
Greedy Algorithms | 3 days | $70 |
Complexity Theory | 4 days | $90 |
Recursion and Backtracking | 2 days | $50 |
- Design and Analysis of Algorithms Assignment Help
- Implementation of Merge Sort Algorithm
- Unmatched Benefits of Our Design and Analysis of Algorithms Assignment Help Service
- Extensive Coverage of Topics by Our Design and Analysis of Algorithms Assignment Experts
- Flexible Revision Policy from Our Design and Analysis of Algorithms Assignment Helpers
- Students around the World Count on Our Design and Analysis of Algorithms Assignment Help
Design and Analysis of Algorithms Assignment Help
At programminghomeworkhelp.com, our Design and Analysis of Algorithms Assignment Help service is tailored to assist students in mastering intricate algorithmic concepts and achieving academic success. Whether you're grappling with Sorting Algorithms, Dynamic Programming, or Graph Algorithms, data structure in any programming languages our team of seasoned experts is here to provide comprehensive support. We understand the challenges students face in navigating these complex coding assignment, which is why we offer personalized assistance to clarify concepts, solve problems, and deliver high-quality assignments.
Our approach emphasizes not just completing assignments but also ensuring that students understand the underlying principles of algorithm design. Each assignment is handled by subject matter experts who hold advanced degrees in Computer Science from prestigious universities worldwide. They bring years of experience and a deep understanding of algorithmic techniques to every task, ensuring that your assignments are not only completed on time but also meet the highest academic standards.
Moreover, our Design and Analysis of Algorithms Assignment Help service is dedicated to providing confidential, reliable, and affordable assistance. We focus on student satisfaction with clear communication, unlimited revisions, and 24/7 support. Our experts offer plagiarism-free solutions, guiding you step-by-step through running time and other aspects of algorithms on a computer system. Trust programminghomeworkhelp.com for your academic success.
Implementation of Merge Sort Algorithm
One popular algorithm often encountered in Design and Analysis of Algorithms assignments is the Merge Sort. Merge Sort is a classic divide-and-conquer algorithm known for its efficiency in sorting arrays or lists. Here's how it works:
def merge_sort(arr):
if len(arr) > 1:
mid = len(arr) // 2 # Finding the mid of the array
left_half = arr[:mid] # Dividing the array elements into 2 halves
right_half = arr[mid:]
merge_sort(left_half) # Sorting the first half
merge_sort(right_half) # Sorting the second half
i = j = k = 0
# Merge the temporary arrays back into arr
while i < len(left_half) and j < len(right_half):
if left_half[i] < right_half[j]:
arr[k] = left_half[i]
i += 1
else:
arr[k] = right_half[j]
j += 1
k += 1
# Checking if any element was left
while i < len(left_half):
arr[k] = left_half[i]
i += 1
k += 1
while j < len(right_half):
arr[k] = right_half[j]
j += 1
k += 1
return arr
Unmatched Benefits of Our Design and Analysis of Algorithms Assignment Help Service
We empathize with students facing common concerns such as "Who will do my Data Structures Assignment?" in our Design and Analysis of Algorithms Assignment Help Service. Our commitment is to deliver exceptional benefits that ensure your academic success in tackling complex challenges. Here's why you should choose us:
- Expertise of Top Professionals: Our service boasts a team of Design and Analysis of Algorithms Homework experts with advanced degrees and years of practical experience in algorithmic problem-solving.
- Customized Assistance: We provide personalized help tailored to your specific assignment requirements, ensuring clarity and thorough understanding of complex algorithmic concepts.
- Timely Delivery: We understand deadlines are crucial. Our experts are adept at delivering high-quality solutions promptly, ensuring you never miss a submission deadline.
- Comprehensive Coverage: From Sorting Algorithms to Complexity Analysis, our service covers a wide array of topics essential for mastering Design and Analysis of Algorithms.
- Quality Assurance: We adhere to rigorous quality standards, ensuring every assignment meets academic guidelines and is thoroughly checked for accuracy and completeness.
- 24/7 Support: Our dedicated customer support team is available round-the-clock to address any queries or concerns, providing continuous assistance throughout your academic journey.
Choose our Design and Analysis of Algorithms Assignment Help Service at programminghomeworkhelp.com to experience these unparalleled benefits and embark on a path to academic excellence with confidence. Our service offers top-notch programming homework help, ensuring you receive exceptional support tailored to your academic needs.
Extensive Coverage of Topics by Our Design and Analysis of Algorithms Assignment Experts
We take pride in our team's profound understanding and expertise in tackling diverse academic challenges. Our Design and Analysis of Algorithms Assignment experts at programminghomeworkhelp.com are dedicated to providing comprehensive assistance tailored to meet your specific needs.
- Algorithm Design: Our team of experts solves assignments involving the creation and implementation of algorithms for various computational problems.
- Algorithm Analysis: Assignments related to analyzing algorithms for time complexity, space complexity, and asymptotic behavior are expertly handled by our team.
- Sorting and Searching Algorithms: Solutions to assignments focusing on implementing and optimizing sorting and searching algorithms such as quicksort, mergesort, binary search, and more are provided by our experts.
- Dynamic Programming: Our experts assist students in solving assignments on dynamic programming techniques for solving optimization problems, including knapsack problem, shortest paths, and more.
- Greedy Algorithms: Assignments involving designing and analyzing greedy algorithms for solving combinatorial optimization problems are expertly tackled by our team.
- Approximation Algorithms: Our experts assist students in solving assignments on designing approximation algorithms for NP-hard optimization problems with provable performance guarantees.
- Online Algorithms: Assignments focusing on online algorithms for problems with dynamic input where decisions are made based on partial information are expertly tackled by our team.
Our experts are committed to providing accurate solutions to assignments on these topics, ensuring students receive comprehensive help with programming assignments necessary to excel in their coursework, without compromising on conceptual explanations.
Flexible Revision Policy from Our Design and Analysis of Algorithms Assignment Helpers
Our Design and Analysis of Algorithms Assignment Helpers understand the importance of delivering assignments that meet your expectations and academic standards. That's why we offer a flexible revision policy designed to ensure your complete satisfaction.
Firstly, our Design and Analysis of Algorithms Assignment Helpers prioritize your feedback. We encourage students to review their completed assignments thoroughly and provide detailed input on any aspects they feel need adjustment. Whether it's refining the solution approach, enhancing clarity in explanations, or aligning with specific formatting requirements, we're here to accommodate your needs.
Furthermore, our help with Design and Analysis of Algorithms Assignment includes unlimited revisions. This means you can request modifications multiple times until you are fully satisfied with the final result. Our aim is to assist you in achieving academic success without any compromise on the quality and comprehensiveness of your assignment.
Additionally, our Design and Analysis of Algorithms Assignment Helpers ensure prompt and efficient turnaround for revisions. We understand the importance of meeting deadlines, so we strive to implement your feedback quickly and effectively. This commitment to flexibility and responsiveness ensures that you receive a tailored assignment that meets both your academic requirements and personal preferences. If you need any programming homework help, we are here to assist you.
Students around the World Count on Our Design and Analysis of Algorithms Assignment Help
Students around the world trust our Design and Analysis of Algorithms Assignment Help Service for comprehensive academic support tailored to their needs. We are honored to serve students from prestigious universities such as:
- Queen's University Belfast
- Rice University
- The University of Sheffield
- Tohoku University
Our service has gained international recognition for its commitment to delivering high-quality solutions and expert guidance on complex algorithmic topics. Whether you are studying in North America, Europe, Asia, or beyond, our team of dedicated experts is here to assist you in mastering Design and Analysis of Algorithms.
Our approach emphasizes personalized assistance, ensuring that each student receives tailored support to navigate the intricacies of algorithm design effectively. We prioritize clarity, accuracy, and adherence to academic guidelines to help students achieve their academic goals with confidence. With a global student base relying on our expertise, we continue to uphold our reputation as a trusted provider of Design and Analysis of Algorithms Assignment Help worldwide.
Explore Our Comprehensive Blogs Covering Algorithm Design Updates
Dive into a world of expertly crafted insights and in-depth analyses that illuminate the intricacies of algorithm design. Whether you're navigating dynamic programming or exploring graph algorithms, our blog offers invaluable resources to enhance your understanding. Stay informed with the latest trends, practical tips, and real-world applications curated by our seasoned Design and Analysis of Algorithms homework experts. Each article is meticulously crafted to empower students with the knowledge needed to excel in their academic pursuits and beyond. Explore, learn, and discover the transformative power of algorithmic thinking through our educational blog today!
Verified Student Reviews on Our Design and Analysis of Algorithms Assignment Help
Explore genuine reviews of our Design and Analysis of Algorithms Assignment Help service here. Students share their genuine experiences about our dedicated team of Design and Analysis of Algorithms Homework experts. Our service provides invaluable help with Design and Analysis of Algorithms Assignment, ensuring thorough understanding and top-notch solutions. Students appreciate the personalized assistance and expert guidance offered by our service, which has earned acclaim for its reliability and effectiveness. Whether you're tackling sorting algorithms or complex graph theories, trust our experts to deliver exceptional support tailored to your academic needs.
Get Acquainted with Our Knowledgeable Design and Analysis of Algorithms Assignment Experts
Meet our Design and Analysis of Algorithms Assignment experts, dedicated to ensuring your academic success. Don’t worry about, "Who will do my Design and Analysis of Algorithms Assignment?" Our team comprises seasoned professionals with extensive experience in algorithmic problem-solving. They offer personalized help with Design and Analysis of Algorithms Assignment, guiding you through complex concepts and providing expert insights tailored to your needs. Whether you need assistance with algorithm analysis, sorting algorithms, or dynamic programming, our experts is here to support you every step of the way. Trust in our expertise to help you achieve excellence in your studies.
.webp)
Carl Mitchel
PhD in Programming
🇨🇦 Canada
Carl Mitchel is a seasoned software engineer with over 10 years of experience in data structures and algorithms, specializing in Python and efficient data management techniques.
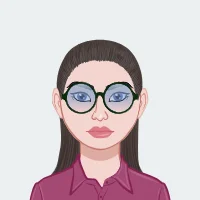
Helen Stevenson
PhD in Programming
🇺🇸 United States
Helen Stevenson, an expert in computer science, offers deep insights into data structures and algorithms. With extensive experience in C programming and software development efficient problem-solving and robust software design, offering valuable perspectives for advanced and aspiring developers alike.
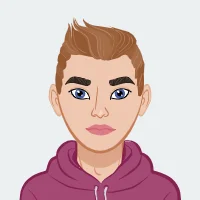
Fredrick Smith
Masters in Programming
🇺🇸 United States
Fredrick Smith is a seasoned software engineer specializing in data structures, algorithms, and C programming. With over 10 years of experience, he excels in designing efficient linked list operations and implementing robust file handling systems. His expertise lies in translating complex concepts into practical solutions, ensuring high performance and reliability in every project.
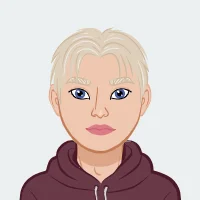
Jeremy Kramer
Masters in Programming
🇺🇸 United States
Jeremy Kramer is a software engineer with over 15 years of experience in algorithm design and performance optimization. He specializes in time complexity analysis and efficient code development.
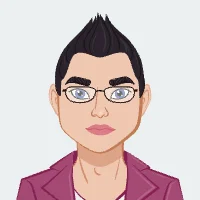
Jimmy Young
Masters in Programming
🇺🇸 United States
Jimmy Young, with 10+ years of experience, specializes in operating systems, covering process scheduling, memory management, concurrency, and file systems. He has successfully guided over 1,000 students, provided tailored solutions and ensured top grades in OS assignments with his in-depth knowledge and practical expertise.
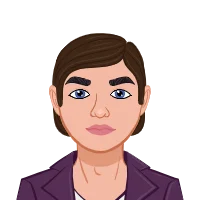
Richard Hayman
Masters in Programming
🇺🇸 United States
Richard Hayman is a seasoned software engineer with over 15 years of experience in algorithm design and implementation. He specializes in graph theory and optimization techniques, bringing a deep understanding of algorithms and their practical applications.

Rafael Barner
Ph.D. in Programming
🇺🇸 United States
Rafael Barner, an expert in multi-threaded programming with extensive experience in synchronization mechanisms and POSIX threads implementation.
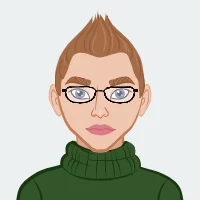
Nathan Carter
Ph.D. in Programming
🇺🇸 United States
Nathan Carter is a seasoned software engineer with 10+ years of experience in algorithm design and performance analysis.
.webp)
John Osborn
Masters in Computer Science
🇬🇧 United Kingdom
John Osborn is a seasoned algorithm expert with over a decade of experience. Specializing in algorithm design and analysis, he offers personalized tutoring, assignment assistance, and guidance on best practices. Proficient in multiple programming languages, John is dedicated to helping students master algorithms and excel academically.
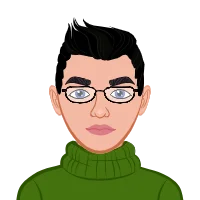
Johnny Houlihan
Masters in Computer Science
🇬🇧 United Kingdom
I’m Johnny Houlihan, a Data Structure Expert with a Master’s in Computer Science from Stanford University. I offer detailed assistance for data structure and algorithm assignments in Java, C++, Python, and more. With years of experience in teaching and problem-solving, I’m here to help you excel.
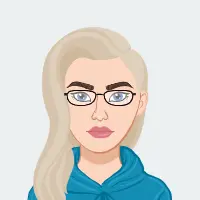
Dr. Amanda King
Ph.D. in Computer Science
🇬🇧 United Kingdom
Dr. Amanda King, a Ph.D. in Computer Science from MIT, is a seasoned Algorithm Assignment Expert with 15+ years of experience. Specializing in algorithm design, data structures, and machine learning, she excels in simplifying complex concepts and mentoring students.
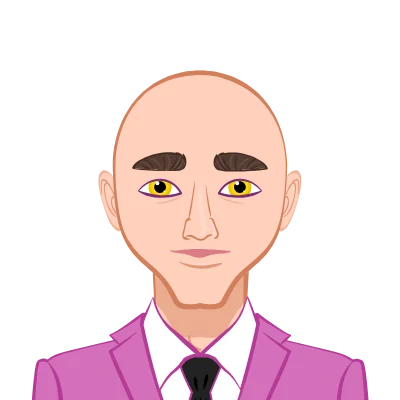
Hayden Ball
Ph.D. in Computer Science
🇬🇧 United Kingdom
Hayden Ball, Ph.D., brings 10 years of expertise in Linked List Assignments. Educated at the University of Warwick, UK, Hayden excels in data structures and algorithmic implementations.
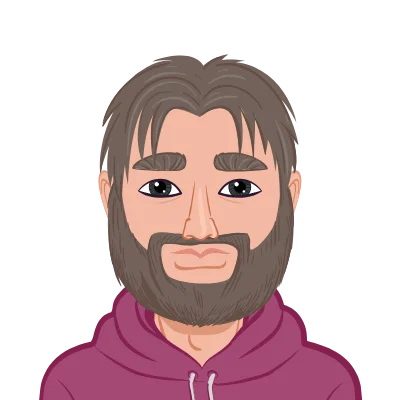
Charles Higgins
Ph.D. in Computer Science
🇺🇸 United States
Introducing Charles Higgins, a seasoned expert in Linked List Assignments with 13 years of experience. Charles earned his Ph.D. from Columbia University, specializing in advanced data structures and algorithmic analysis.
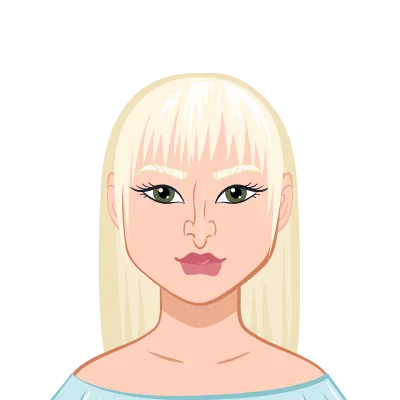
Maya Riley
Ph.D. in Computer Science
🇬🇧 United Kingdom
Maya Riley is an accomplished expert in Linked List Assignments with a Ph.D. from the University of Oxford, UK. With 15 years of experience, she excels in advanced data structures and algorithm implementations.
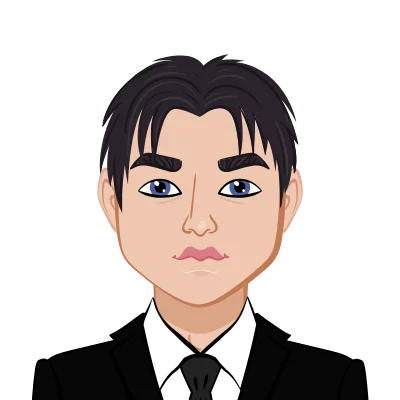
James Patterson
Ph.D. in Computer Science
🇨🇦 Canada
James Patterson, Ph.D., brings 18 years of expertise in Linked List Assignments. Educated at the University of Waterloo, Canada, he specializes in advanced data structures and algorithms.
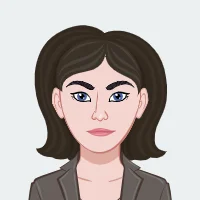
Linda Nguyen
Masters in Algorithm
🇨🇦 Canada
Linda Nguyen is a proficient Algorithm Assignment Help Expert boasting 10 years of expertise. She earned her Master's degree from the University of Toronto, Canada.
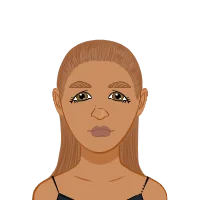
Dr. Rita T. Rose
PhD in Computer Science
🇺🇸 United States
Dr. Rita T. Rose holds a PhD in Computer Science from the University of Michigan - Ann Arbor, USA. With 7 years of experience, she has completed over 700 Design and Analysis of Algorithms assignments. Dr. Rose specializes in sorting algorithms and recursive algorithms, offering students structured guidance and practical insights to navigate through complex algorithmic challenges with confidence.
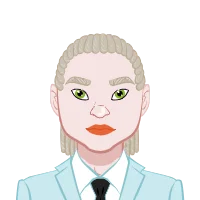
Dr. Terry N. Morris
PhD in Computer Science
🇨🇦 Canada
Dr. Terry N. Morris completed his PhD in Computer Science at the University of Toronto, Toronto, Canada. With 8 years of experience, he has successfully handled over 800 Design and Analysis of Algorithms assignments. Dr. Morris excels in dynamic programming and algorithm analysis, providing students with clear explanations and effective problem-solving strategies to excel in their studies.
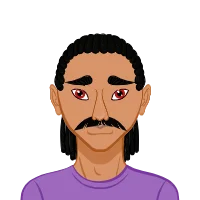
Dr. Jared J. Rooney
PhD in Computer Science
🇺🇸 United States
Dr. Jared J. Rooney earned his PhD in Computer Science from the University of Pennsylvania, Philadelphia, USA. With 9 years of experience, he has completed over 900 Design and Analysis of Algorithms assignments. Dr. Rooney specializes in algorithmic optimization and graph algorithms, offering students comprehensive assistance and strategic insights to enhance their understanding and academic performance.
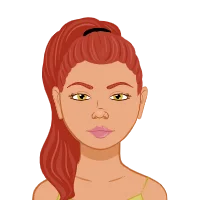
Dr. Marisol M. Brown
PhD in Computer Science
🇺🇸 United States
Dr. Marisol M. Brown holds a PhD in Computer Science from Columbia University, New York, USA. With over 10 years of experience, she has successfully completed over 1000 Design and Analysis of Algorithms assignments. Dr. Brown's expertise lies in advanced algorithm design and complexity theory, and she is committed to delivering meticulous guidance and insightful solutions to students seeking help with their assignments.
Related Topics
Frequently Asked Questions
Our service has designed some FAQs to provide clarity and insight into our Design and Analysis of Algorithms Assignment Help service. Discover essential information about how our Design and Analysis of Algorithms Assignment experts can assist you, get detailed answers about seeking help with Design and Analysis of Algorithms Assignment, and learn more about the process of assignment completion. Don’t worry about, "Who will do my Design and Analysis of Algorithms Assignment?" Our FAQs offers comprehensive explanations to ensure transparency and confidence in our service.
Yes, we guarantee high-quality solutions that meet academic standards, backed by our team of experienced professionals.
We offer unlimited revisions to ensure your satisfaction. Simply communicate your feedback, and we'll make the necessary adjustments promptly.
Absolutely. We prioritize confidentiality and adhere to strict privacy policies to protect your information.
Yes, you have the option to request a specific expert based on their specialization and availability.
You can submit your assignments through our online platform by filling out a simple form and uploading the assignment details.
We cover a wide range of topics including sorting algorithms, dynamic programming, graph algorithms, complexity analysis, and more.
Our experts are highly qualified professionals with advanced degrees and extensive experience in algorithmic problem-solving from top universities worldwide.
Our service provides expert guidance and assistance with understanding complex algorithms, solving assignments, and achieving academic excellence in Design and Analysis of Algorithms.