Claim Your Offer
Unlock an amazing offer at www.programminghomeworkhelp.com with our latest promotion. Get an incredible 10% off on your all programming assignment, ensuring top-quality assistance at an affordable price. Our team of expert programmers is here to help you, making your academic journey smoother and more cost-effective. Don't miss this chance to improve your skills and save on your studies. Take advantage of our offer now and secure exceptional help for your programming assignments.
We Accept
Access Our Free Sample Function Strings & Arrays Writing in C Assignments
Browse through our sample solutions to get a glimpse of the quality and expertise we bring to Function Strings and Arrays Writing in C assignments. These samples showcase our meticulous approach, attention to detail, and proficiency in solving complex programming tasks, ensuring clarity and accuracy in every solution.
Data Structures and Algorithms
Data Structures and Algorithms
Data Structures and Algorithms
Data Structures and Algorithms
Data Structures and Algorithms
Data Structures and Algorithms
Data Structures and Algorithms
Java
C++
Data Structures and Algorithms
Data Structures and Algorithms
Data Structures and Algorithms
Data Structures and Algorithms
Data Structures and Algorithms
Data Structures and Algorithms
Get Affordable Function Strings and Arrays Writing in C Assignment Help Online
At ProgrammingHomeworkHelp.com, affordability is key, and we strive to make Function Strings and Arrays Writing in C assignment help accessible to all students by customizing our rates. We understand the financial constraints students face, which is why we offer flexible pricing options tailored to your budget. Our transparent pricing model ensures that you only pay for the assistance you need, with no hidden fees or surprises. Check out our sample price ranges below to see how we cater to different needs and budgets:
Service Type | Price Range (USD) |
---|---|
Basic Function Assistance | $20 - $50 |
Complex Algorithm Solutions | $30 - $80 |
String Manipulation Tasks | $25 - $60 |
Array Sorting and Searching | $35 - $75 |
Comprehensive Assignment Help | $50 - $100 |
- How Our Experts Write Function Strings & Arrays in C?
- Why Thousands of Students Seek Our Help with Function Strings and Arrays Writing Assignments in C?
- Can I Pay Someone to Do My Function Strings and Arrays Writing Assignments in C?
- Streamlined Process for Availing Our Online Function Strings and Arrays Writing in C Homework Help Service
How Our Experts Write Function Strings & Arrays in C?
Function, strings, and arrays are fundamental concepts in programming, especially in languages like C. When writing functions for strings and arrays in C, it's essential to understand the fundamental concepts and syntax of the language. Here's a basic guide followed by our C programming homework experts:
- Function Declarations: Begin by declaring your functions, specifying the return type and parameters.
- Function Definitions: Define the behavior of your functions. This involves writing the actual code to perform the desired operations.
- String Handling: When working with strings, use character arrays (or pointers to characters) to store and manipulate strings. Utilize standard library functions like strcpy(), strcat(), strlen(), etc., for string operations.
- Array Manipulation: For array manipulation, ensure proper indexing and bounds checking to avoid memory errors. Use loops to iterate through arrays and perform operations like sorting, searching, or modifying elements.
- Memory Management: Be mindful of memory allocation and deallocation, especially when dynamically allocating memory for arrays or strings. Always free dynamically allocated memory to prevent memory leaks.
- Testing and Debugging: Test your functions thoroughly with various input scenarios to ensure correctness and robustness. Utilize debugging tools like gdb to identify and fix any errors or issues in your code.
By following these guidelines and practicing regularly, you'll gain proficiency in writing functions for strings and arrays in C. Feel free to contact us if you need instant help.
Why Thousands of Students Seek Our Help with Function Strings and Arrays Writing Assignments in C?
At ProgrammingHomeworkHelp.com, we know that completing a function strings and arrays writing assignments in C can be daunting. Our specialized assistance is tailored to your needs, offering a multitude of benefits to propel you towards success. Here's why availing our help with Function Strings and Arrays Writing assignments in C is the smart choice:
- Expert Guidance: Our team comprises seasoned professionals with extensive experience in programming and C language expertise. Benefit from their deep understanding and guidance to overcome any challenges you encounter.
- Customized Solutions: No task is too complex for our experts. Receive personalized solutions that address your specific requirements, ensuring clarity and accuracy in every aspect of your Function Strings and Arrays Writing assignments.
- Timely Delivery: With looming deadlines, time is of the essence. Rely on our prompt service to deliver impeccable solutions within the stipulated timeframe, allowing you to stay on track with your academic commitments.
- Error-Free Code: Say goodbye to syntax errors and debugging nightmares. Our meticulous approach guarantees clean, error-free code, meticulously crafted to meet the highest standards of programming excellence.
- Concept Clarity: Beyond mere completion, we prioritize your understanding of Function Strings and Arrays Writing concepts. Clarify doubts, grasp intricate concepts, and enhance your programming prowess under the guidance of our experts.
- Confidentiality: Your privacy is paramount to us. Rest assured that your personal information and academic details are kept confidential, ensuring a safe and secure collaboration experience.
Don't let Function Strings and Arrays Writing homework in C weigh you down. Embrace the support of our dedicated team and embark on a journey towards academic success today!
Can I Pay Someone to Do My Function Strings and Arrays Writing Assignments in C?
Completing Function Strings and Arrays Writing assignments in C requires not just understanding the concepts but also adeptness in applying them effectively. At ProgrammingHomeworkHelp.com, we excel in a diverse range of assignment topics, ensuring comprehensive assistance tailored to your needs. Here are some of the areas where our expertise shines:
- String Manipulation: Crafting assignments on string manipulation tasks, such as concatenation, copying, and comparison, is our forte. Pay someone to do my Function Strings and Arrays Writing assignment in C, and witness flawless implementation of string operations, meticulously executed to meet your requirements.
- Array Sorting and Searching: Need assistance with assignments involving array sorting algorithms like bubble sort or quicksort? Look no further! Our team possesses expertise in implementing efficient sorting and searching algorithms, ensuring optimal performance and accuracy in your assignments.
- Memory Allocation: Understanding memory allocation concepts like dynamic memory allocation and deallocation is crucial in C programming assignments. Entrust us with your assignments, and rest assured of meticulous handling of memory allocation tasks, adhering to best practices and ensuring memory efficiency.
- Pointer Arithmetic: Assignments involving pointer arithmetic can be challenging, but our experts thrive on such tasks. Whether it's traversing arrays using pointers or manipulating strings efficiently, count on us to deliver assignments showcasing proficiency in pointer arithmetic concepts.
- String Tokenization: String tokenization assignments often require a deep understanding of delimiter-based parsing techniques. Allow us to handle your assignments on string tokenization, demonstrating expertise in extracting meaningful tokens from input strings with precision.
- Array Operations: From basic array manipulation tasks to complex multidimensional array operations, our team is well-equipped to handle a wide array of assignments. Pay someone to do my Function Strings and Arrays Writing assignment in C and witness meticulous execution of array operations tailored to your specific requirements.
- Error Handling: Effective error handling is essential for writing robust C programs. In assignments involving error detection and handling mechanisms, rely on our expertise to deliver solutions that prioritize code reliability and robustness, ensuring smooth execution under various scenarios.
- Optimization Techniques: Crafting efficient C programs often involves implementing optimization techniques to enhance performance. Entrust us with assignments requiring optimization, and benefit from our expertise in employing techniques like loop unrolling, code refactoring, and algorithmic optimizations to improve program efficiency.
So, yes, you can trust us with your “do my data structures and algorithms homework” needs. With our comprehensive expertise and dedication to excellence, tackling your assignment becomes a seamless journey towards academic success.
Streamlined Process for Availing Our Online Function Strings and Arrays Writing in C Homework Help Service
With our efficient five-step process, you can breeze through your Function Strings and Arrays Writing homework in C with confidence. Experience seamless assistance and elevate your academic performance with our dedicated support. Here is how our online function strings and arrays writing in C homework help service works
- Submit Your Assignment
- Expert Assignment Matching
- Assignment Execution
- Quality Assurance
- Timely Delivery
Explore Our Blog for Tips & Insights Into Function Strings & Arrays Writing in C
Stay updated with the latest trends, tips, and insights in Function Strings and Arrays Writing in C programming through our informative blog. Our blog section covers a wide range of topics, from beginner guides to advanced techniques, curated by our experts to enhance your understanding and proficiency in Function Strings and Arrays Writing.
What Our Esteemed Customers are Saying About Our Services
In this section, you'll find honest reviews from our clients who have experienced our Function Strings and Arrays Writing in C homework help firsthand. Their feedback reflects the quality of our service, our commitment to excellence, and our dedication to customer satisfaction. Read through their testimonials to gain insight into how our assistance has made a difference in their academic journey.
Hire Eminent Functions Strings and Arrays Writing Assignment Experts Skilled in C Programming
Our team of experts comprises seasoned professionals with extensive experience in Function Strings and Arrays Writing in C programming. Equipped with advanced degrees and a passion for problem-solving, our experts are committed to providing personalized assistance tailored to your specific needs. With their in-depth knowledge and dedication to excellence, you can trust them to deliver impeccable solutions to your Function Strings and Arrays Writing assignments, ensuring your academic success.
.webp)
Carl Mitchel
PhD in Programming
🇨🇦 Canada
Carl Mitchel is a seasoned software engineer with over 10 years of experience in data structures and algorithms, specializing in Python and efficient data management techniques.
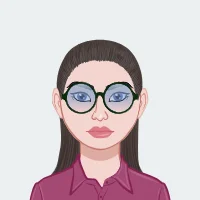
Helen Stevenson
PhD in Programming
🇺🇸 United States
Helen Stevenson, an expert in computer science, offers deep insights into data structures and algorithms. With extensive experience in C programming and software development efficient problem-solving and robust software design, offering valuable perspectives for advanced and aspiring developers alike.
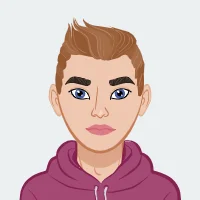
Fredrick Smith
Masters in Programming
🇺🇸 United States
Fredrick Smith is a seasoned software engineer specializing in data structures, algorithms, and C programming. With over 10 years of experience, he excels in designing efficient linked list operations and implementing robust file handling systems. His expertise lies in translating complex concepts into practical solutions, ensuring high performance and reliability in every project.
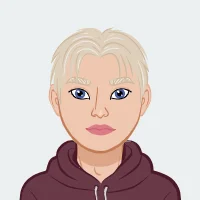
Jeremy Kramer
Masters in Programming
🇺🇸 United States
Jeremy Kramer is a software engineer with over 15 years of experience in algorithm design and performance optimization. He specializes in time complexity analysis and efficient code development.
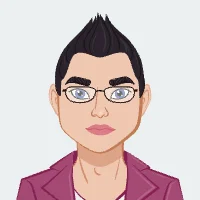
Jimmy Young
Masters in Programming
🇺🇸 United States
Jimmy Young, with 10+ years of experience, specializes in operating systems, covering process scheduling, memory management, concurrency, and file systems. He has successfully guided over 1,000 students, provided tailored solutions and ensured top grades in OS assignments with his in-depth knowledge and practical expertise.
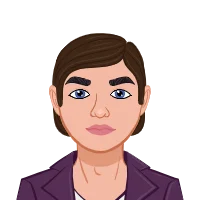
Richard Hayman
Masters in Programming
🇺🇸 United States
Richard Hayman is a seasoned software engineer with over 15 years of experience in algorithm design and implementation. He specializes in graph theory and optimization techniques, bringing a deep understanding of algorithms and their practical applications.

Rafael Barner
Ph.D. in Programming
🇺🇸 United States
Rafael Barner, an expert in multi-threaded programming with extensive experience in synchronization mechanisms and POSIX threads implementation.
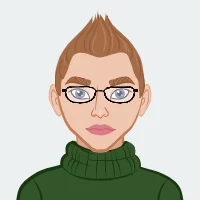
Nathan Carter
Ph.D. in Programming
🇺🇸 United States
Nathan Carter is a seasoned software engineer with 10+ years of experience in algorithm design and performance analysis.
.webp)
John Osborn
Masters in Computer Science
🇬🇧 United Kingdom
John Osborn is a seasoned algorithm expert with over a decade of experience. Specializing in algorithm design and analysis, he offers personalized tutoring, assignment assistance, and guidance on best practices. Proficient in multiple programming languages, John is dedicated to helping students master algorithms and excel academically.
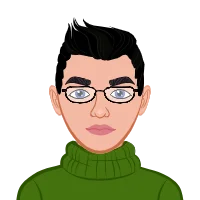
Johnny Houlihan
Masters in Computer Science
🇬🇧 United Kingdom
I’m Johnny Houlihan, a Data Structure Expert with a Master’s in Computer Science from Stanford University. I offer detailed assistance for data structure and algorithm assignments in Java, C++, Python, and more. With years of experience in teaching and problem-solving, I’m here to help you excel.
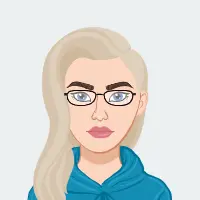
Dr. Amanda King
Ph.D. in Computer Science
🇬🇧 United Kingdom
Dr. Amanda King, a Ph.D. in Computer Science from MIT, is a seasoned Algorithm Assignment Expert with 15+ years of experience. Specializing in algorithm design, data structures, and machine learning, she excels in simplifying complex concepts and mentoring students.
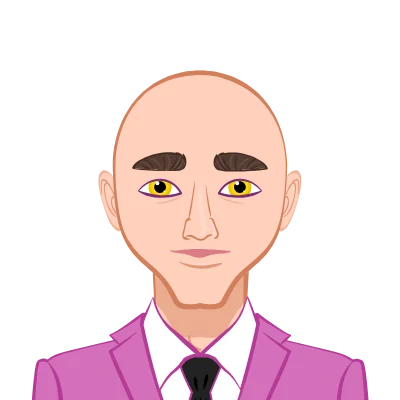
Hayden Ball
Ph.D. in Computer Science
🇬🇧 United Kingdom
Hayden Ball, Ph.D., brings 10 years of expertise in Linked List Assignments. Educated at the University of Warwick, UK, Hayden excels in data structures and algorithmic implementations.
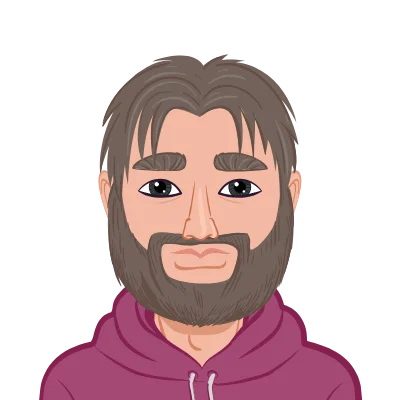
Charles Higgins
Ph.D. in Computer Science
🇺🇸 United States
Introducing Charles Higgins, a seasoned expert in Linked List Assignments with 13 years of experience. Charles earned his Ph.D. from Columbia University, specializing in advanced data structures and algorithmic analysis.
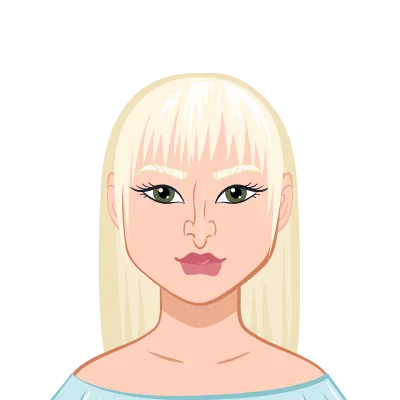
Maya Riley
Ph.D. in Computer Science
🇬🇧 United Kingdom
Maya Riley is an accomplished expert in Linked List Assignments with a Ph.D. from the University of Oxford, UK. With 15 years of experience, she excels in advanced data structures and algorithm implementations.
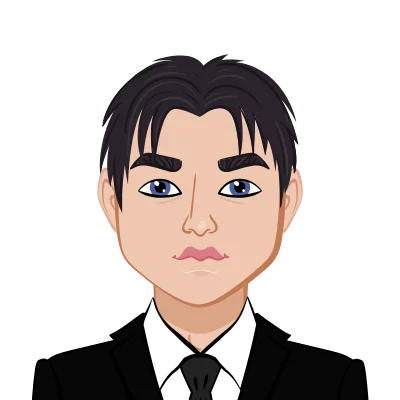
James Patterson
Ph.D. in Computer Science
🇨🇦 Canada
James Patterson, Ph.D., brings 18 years of expertise in Linked List Assignments. Educated at the University of Waterloo, Canada, he specializes in advanced data structures and algorithms.
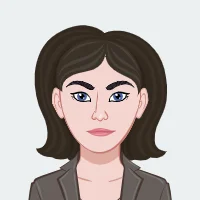
Linda Nguyen
Masters in Algorithm
🇨🇦 Canada
Linda Nguyen is a proficient Algorithm Assignment Help Expert boasting 10 years of expertise. She earned her Master's degree from the University of Toronto, Canada.
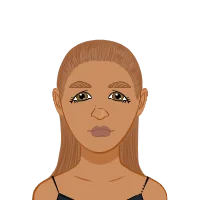
Dr. Rita T. Rose
PhD in Computer Science
🇺🇸 United States
Dr. Rita T. Rose holds a PhD in Computer Science from the University of Michigan - Ann Arbor, USA. With 7 years of experience, she has completed over 700 Design and Analysis of Algorithms assignments. Dr. Rose specializes in sorting algorithms and recursive algorithms, offering students structured guidance and practical insights to navigate through complex algorithmic challenges with confidence.
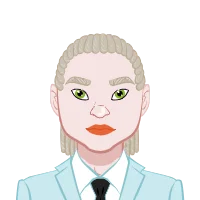
Dr. Terry N. Morris
PhD in Computer Science
🇨🇦 Canada
Dr. Terry N. Morris completed his PhD in Computer Science at the University of Toronto, Toronto, Canada. With 8 years of experience, he has successfully handled over 800 Design and Analysis of Algorithms assignments. Dr. Morris excels in dynamic programming and algorithm analysis, providing students with clear explanations and effective problem-solving strategies to excel in their studies.
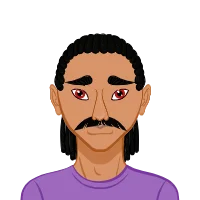
Dr. Jared J. Rooney
PhD in Computer Science
🇺🇸 United States
Dr. Jared J. Rooney earned his PhD in Computer Science from the University of Pennsylvania, Philadelphia, USA. With 9 years of experience, he has completed over 900 Design and Analysis of Algorithms assignments. Dr. Rooney specializes in algorithmic optimization and graph algorithms, offering students comprehensive assistance and strategic insights to enhance their understanding and academic performance.
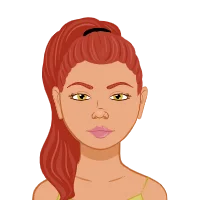
Dr. Marisol M. Brown
PhD in Computer Science
🇺🇸 United States
Dr. Marisol M. Brown holds a PhD in Computer Science from Columbia University, New York, USA. With over 10 years of experience, she has successfully completed over 1000 Design and Analysis of Algorithms assignments. Dr. Brown's expertise lies in advanced algorithm design and complexity theory, and she is committed to delivering meticulous guidance and insightful solutions to students seeking help with their assignments.
Related Topics
Frequently Asked Questions
Got questions about our Function Strings and Arrays Writing in C homework help service? Check out our FAQs section for answers to common queries. Can't find what you're looking for? Feel free to reach out to our friendly support team via live chat for immediate assistance.
Our team meticulously verifies each solution for pointer arithmetic tasks to ensure accuracy and reliability. We conduct thorough testing and debugging to eliminate any errors or inconsistencies in the code, ensuring that the solution meets the highest standards of quality and correctness.
Certainly! Our experts have extensive experience in handling memory allocation tasks, including dynamic memory allocation and deallocation in Function Strings and Arrays Writing assignments. We provide detailed explanations and examples to help you grasp these concepts effectively.
Yes, we excel in implementing a variety of sorting and searching algorithms for arrays in C programming assignments. From basic algorithms like bubble sort to more advanced techniques like quicksort and binary search, our experts ensure efficient and accurate solutions tailored to your requirements.
Absolutely! Our team specializes in Function Strings and Arrays Writing in C, including tasks like string tokenization, concatenation, and comparison. Whether you need assistance with parsing strings or implementing custom string manipulation functions, we've got you covered.