Claim Your Offer
Unlock an amazing offer at www.programminghomeworkhelp.com with our latest promotion. Get an incredible 10% off on your all programming assignment, ensuring top-quality assistance at an affordable price. Our team of expert programmers is here to help you, making your academic journey smoother and more cost-effective. Don't miss this chance to improve your skills and save on your studies. Take advantage of our offer now and secure exceptional help for your programming assignments.
We Accept
Sample Queue Implementation Programming Assignments Solved Using Nodes & Pointers
Browse through our sample section to get a glimpse of the quality of work we deliver to our clients. These sample queue implementation programming assignments showcase our expertise in crafting well-structured, efficient, and error-free solutions.
Data Structures and Algorithms
Data Structures and Algorithms
Data Structures and Algorithms
Data Structures and Algorithms
Data Structures and Algorithms
Data Structures and Algorithms
Data Structures and Algorithms
Java
C++
Data Structures and Algorithms
Data Structures and Algorithms
Data Structures and Algorithms
Data Structures and Algorithms
Data Structures and Algorithms
Data Structures and Algorithms
Affordable Queue Implementation Programming Assignment Help Using Nodes and Pointers
ProgrammingHomeworkHelp.com understands the financial constraints that students often face. That's why we prioritize affordability by customizing our rates to suit your budget. Our flexible pricing structure ensures that you receive high-quality queue implementation programming assignment help using nodes and pointers at competitive prices. We believe in transparency and fairness, which is why we offer a range of pricing options tailored to your specific requirements. Whether you need assistance with a simple homework assignment or a complex project, our goal is to provide affordable solutions without compromising on quality.
Service Type | Price Range |
---|---|
Basic Queue Implementation Assignment | $30 - $50 |
Circular Queue Implementation Assignment | $40 - $60 |
Priority Queue Using Linked Lists | $50 - $70 |
Thread-Safe Queue Assignment | $60 - $80 |
Real-Time Queue Systems Project | $70 - $100 |
Multi-Level Queue Implementation Project | $80 - $120 |
- Hire Our Skilled Experts Experienced in Queue Implementation Programming Using Nodes and Pointers
- Qualities of Our Queue Implementation Programming Using Nodes and Pointers Homework Helpers:
- Do My Queue Implementation Programming Assignment Using Nodes and Pointers: Topics Our Tutors Specialize in
- Exclusive Discounts for Students Who Choose Our Help with Queue Implementation Programming Assignment Using Nodes and Pointers:
Hire Our Skilled Experts Experienced in Queue Implementation Programming Using Nodes and Pointers
Queue implementation using nodes and pointers involves creating a data structure where each element, known as a node, contains both the data and a reference to the next node in the queue. To implement a queue, you typically have two pointers: a front pointer indicating the first element in the queue and a rear pointer indicating the last element.
- Enqueuing: To enqueue (add) an element to the queue, you create a new node, set its data, and update the rear pointer to point to this new node. If the queue is empty, you also update the front pointer.
- Dequeuing: To dequeue (remove) an element from the queue, you retrieve the data from the node pointed to by the front pointer, update the front pointer to point to the next node, and deallocate memory for the removed node.
Throughout these operations, it's important to manage edge cases, such as when the queue is empty or full, and to ensure proper memory management to avoid memory leaks.
Here's a simple Python code example to illustrate how it's done:
class Node:
def __init__(self, data):
self.data = data
self.next = None
class Queue:
def __init__(self):
self.front = None
self.rear = None
def enqueue(self, data):
new_node = Node(data)
if self.rear is None:
self.front = new_node
self.rear = new_node
else:
self.rear.next = new_node
self.rear = new_node
def dequeue(self):
if self.front is None:
return None
else:
removed_data = self.front.data
self.front = self.front.next
if self.front is None:
self.rear = None
return removed_data
def display(self):
current = self.front
while current:
print(current.data, end=" ")
current = current.next
print()
# Example usage:
queue = Queue()
queue.enqueue(1)
queue.enqueue(2)
queue.enqueue(3)
queue.display() # Output: 1 2 3
queue.dequeue()
queue.display() # Output: 2 3
In this code:
- The Node class represents each element in the queue, containing data and a pointer to the next node.
- The Queue class manages the queue operations like enqueue (adding elements) and dequeue (removing elements).
- enqueue adds a new node to the rear of the queue.
- dequeue removes and returns the front node of the queue.
- display function is provided to visualize the queue.
Qualities of Our Queue Implementation Programming Using Nodes and Pointers Homework Helpers:
At ProgrammingHomeworkHelp.com, our queue implementation programming using nodes and pointers homework helpers are not just experts; they're dedicated professionals committed to ensuring your success. Our team possesses a unique blend of skills and qualities that set us apart in the field of programming assistance. Here's what makes our programming homework experts stand out:
- Expertise: Our homework helpers are seasoned professionals with extensive knowledge and experience in queue implementation programming using nodes and pointers. They are well-versed in the intricacies of data structures and algorithms, enabling them to provide comprehensive solutions to your assignments.
- Problem-Solving Skills: With a keen ability to analyze complex problems and devise effective solutions, our experts excel at tackling challenging programming tasks. They approach each assignment with a systematic methodology, breaking down problems into manageable steps to ensure clarity and efficiency.
- Attention to Detail: Precision is key in programming, and our homework helpers understand the importance of attention to detail. From writing clean, well-commented code to ensuring proper memory management, they take every measure to deliver high-quality solutions that meet the strictest standards.
- Timeliness: We understand the significance of deadlines, which is why our programming experts prioritize prompt deliveries. Whether you have an urgent assignment or a long-term project, you can rely on our team to provide timely assistance without compromising on quality.
- Commitment to Excellence: Above all, our queue implementation programming using nodes and pointers homework writers are driven by a commitment to excellence. They go above and beyond to ensure your satisfaction, providing personalized support and guidance every step of the way.
With our team of dedicated programming experts by your side, you can overcome any challenge and achieve academic success in queue implementation programming using nodes and pointers.
Do My Queue Implementation Programming Assignment Using Nodes and Pointers: Topics Our Tutors Specialize in
At ProgrammingHomeworkHelp.com, we excel in a diverse range of queue implementation programming using nodes and pointers assignment topics. Our team of experts can handle any “do my queue implementation programming assignment using nodes and pointers” request, proficiently crafting high-quality assignments tailored to your specific requirements. Here are eight topics in which we demonstrate our expertise:
- Basic Queue Operations: From enqueueing and dequeuing elements to checking the status of the queue, our experts are well-versed in the fundamentals of basic queue operations. Whether you need assistance with implementing these operations from scratch or optimizing existing code, we've got you covered.
- Circular Queue Implementation: Crafting a circular queue requires a deep understanding of pointers and memory management. Our experts have extensive experience in designing efficient circular queue implementations, ensuring seamless insertion and deletion of elements even in constrained memory environments.
- Priority Queue Using Linked Lists: Priority queues add an extra layer of complexity to queue implementation, requiring careful consideration of element priorities. Our team excels in designing priority queue implementations using linked lists, guaranteeing accurate prioritization and efficient homework completion.
- Queue Using Stacks: Implementing a queue using stacks involves clever manipulation of stack operations to achieve FIFO behavior. Our experts are adept at devising innovative solutions for this task, leveraging their expertise in both data structures to deliver impeccable homework assignments.
- Multi-Level Queue Implementation: Multi-level queues divide processes into different priority levels, each with its own queue. Crafting an efficient multi-level queue implementation demands meticulous planning and organization. Our experts possess the skills and knowledge necessary to design robust multi-level queue systems that meet your homework requirements.
- Thread-Safe Queue: Ensuring thread safety in queue implementations is essential for concurrent programming environments. Our team specializes in creating thread-safe queue implementations using nodes and pointers, employing synchronization techniques to prevent data corruption and race conditions in your homework assignments.
- Real-Time Queue Systems: Real-time queue systems require fast and predictable response times, making performance optimization a critical factor. Our experts are proficient in optimizing queue implementations for real-time applications, delivering homework assignments that meet stringent performance requirements without compromising correctness.
- Queue Applications in Data Structures: Beyond basic queue operations, our experts are skilled in applying queue data structures to solve real-world problems. Whether it's simulating queuing systems, implementing job scheduling algorithms, or designing network packet queues, we offer comprehensive homework assistance tailored to your specific application needs.
Pay our experts to do your queue implementation programming assignment using nodes and pointers, and rest assured that our team of experts will deliver exceptional results on these and other topics, meeting your academic requirements with precision and professionalism.
Exclusive Discounts for Students Who Choose Our Help with Queue Implementation Programming Assignment Using Nodes and Pointers:
At ProgrammingHomeworkHelp.com, we believe in making our services accessible and affordable to all students. That's why we offer a range of exclusive discounts to help you save on your queue implementation programming assignment using nodes and pointers homework. Here are the various discounts you can enjoy:
- Refer a Friend Discount: Spread the word about our services and reap the rewards! When you refer a friend to ProgrammingHomeworkHelp.com, you'll receive a generous discount of 50% off on your next homework assignment. It's our way of saying thank you for helping us grow our community of satisfied customers.
- 20% Discount on Your Second Order: We value your loyalty, which is why we offer a special discount of 20% on your second order with us. Whether you're returning for another assignment or seeking assistance with a different topic, you'll enjoy significant savings on your homework.
- Bulk Orders Discount: Planning to place multiple orders or tackling a series of assignments? Our bulk orders discount has you covered. Enjoy discounted rates when you place bulk orders for queue implementation programming assignments using nodes and pointers, making it more cost-effective to get the help you need.
- Seasonal Discounts: Keep an eye out for our seasonal discounts throughout the year! From back-to-school specials to holiday promotions, we frequently offer exclusive discounts on our services. Take advantage of these limited-time offers to save even more on your queue implementation programming homework.
With these exclusive discounts, getting help with your queue implementation programming assignment using nodes and pointers homework has never been more affordable.
Tips & Insights into Queue Implementation Programming Using Nodes and Pointers
Dive into our blog section to discover a wealth of programming insights, tips, and tutorials related to queue implementation programming using nodes and pointers. Our blog covers a wide range of topics, from basic concepts to advanced techniques, designed to enhance your understanding and proficiency in programming. Whether you're a beginner looking to learn the fundamentals or an experienced programmer seeking to expand your knowledge, you'll find valuable resources to support your learning journey.
Reviews from Students Who Have Benefitted from Our Reliable Service
In this section, you'll find authentic reviews from our satisfied customers who have benefited from our queue implementation programming assignment help using nodes and pointers. Our clients have praised our expert team for their professionalism, timely deliveries, and high-quality solutions. They appreciate the personalized support they receive and commend our commitment to ensuring their academic success.
80+ Brilliant Queue Implementation Programming Using Nodes and Pointers Assignment Experts
Our team of queue implementation programming using nodes and pointers assignment experts comprises seasoned professionals with years of experience in the field. They possess comprehensive knowledge of data structures, algorithms, and programming languages, allowing them to deliver top-notch solutions to your assignments. Our experts are dedicated to staying updated with the latest advancements in the industry, ensuring that they provide innovative and efficient approaches to solving your homework challenges.
.webp)
Carl Mitchel
PhD in Programming
🇨🇦 Canada
Carl Mitchel is a seasoned software engineer with over 10 years of experience in data structures and algorithms, specializing in Python and efficient data management techniques.
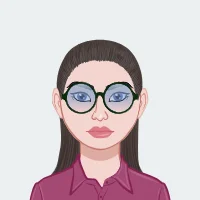
Helen Stevenson
PhD in Programming
🇺🇸 United States
Helen Stevenson, an expert in computer science, offers deep insights into data structures and algorithms. With extensive experience in C programming and software development efficient problem-solving and robust software design, offering valuable perspectives for advanced and aspiring developers alike.
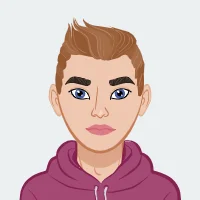
Fredrick Smith
Masters in Programming
🇺🇸 United States
Fredrick Smith is a seasoned software engineer specializing in data structures, algorithms, and C programming. With over 10 years of experience, he excels in designing efficient linked list operations and implementing robust file handling systems. His expertise lies in translating complex concepts into practical solutions, ensuring high performance and reliability in every project.
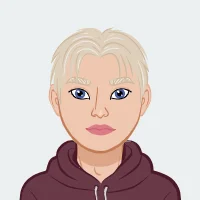
Jeremy Kramer
Masters in Programming
🇺🇸 United States
Jeremy Kramer is a software engineer with over 15 years of experience in algorithm design and performance optimization. He specializes in time complexity analysis and efficient code development.
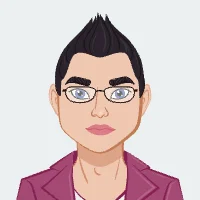
Jimmy Young
Masters in Programming
🇺🇸 United States
Jimmy Young, with 10+ years of experience, specializes in operating systems, covering process scheduling, memory management, concurrency, and file systems. He has successfully guided over 1,000 students, provided tailored solutions and ensured top grades in OS assignments with his in-depth knowledge and practical expertise.
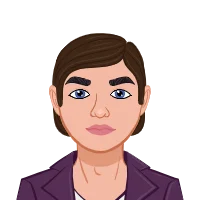
Richard Hayman
Masters in Programming
🇺🇸 United States
Richard Hayman is a seasoned software engineer with over 15 years of experience in algorithm design and implementation. He specializes in graph theory and optimization techniques, bringing a deep understanding of algorithms and their practical applications.

Rafael Barner
Ph.D. in Programming
🇺🇸 United States
Rafael Barner, an expert in multi-threaded programming with extensive experience in synchronization mechanisms and POSIX threads implementation.
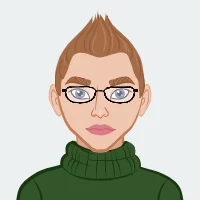
Nathan Carter
Ph.D. in Programming
🇺🇸 United States
Nathan Carter is a seasoned software engineer with 10+ years of experience in algorithm design and performance analysis.
.webp)
John Osborn
Masters in Computer Science
🇬🇧 United Kingdom
John Osborn is a seasoned algorithm expert with over a decade of experience. Specializing in algorithm design and analysis, he offers personalized tutoring, assignment assistance, and guidance on best practices. Proficient in multiple programming languages, John is dedicated to helping students master algorithms and excel academically.
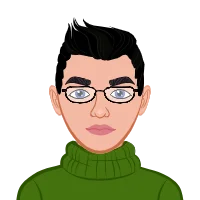
Johnny Houlihan
Masters in Computer Science
🇬🇧 United Kingdom
I’m Johnny Houlihan, a Data Structure Expert with a Master’s in Computer Science from Stanford University. I offer detailed assistance for data structure and algorithm assignments in Java, C++, Python, and more. With years of experience in teaching and problem-solving, I’m here to help you excel.
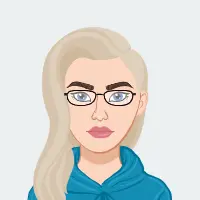
Dr. Amanda King
Ph.D. in Computer Science
🇬🇧 United Kingdom
Dr. Amanda King, a Ph.D. in Computer Science from MIT, is a seasoned Algorithm Assignment Expert with 15+ years of experience. Specializing in algorithm design, data structures, and machine learning, she excels in simplifying complex concepts and mentoring students.
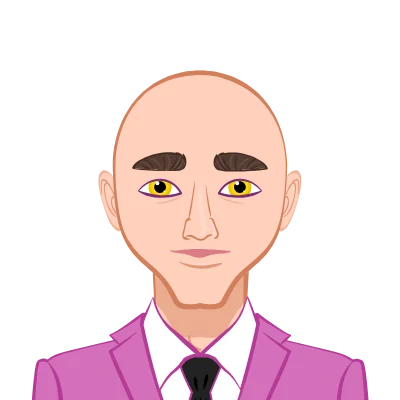
Hayden Ball
Ph.D. in Computer Science
🇬🇧 United Kingdom
Hayden Ball, Ph.D., brings 10 years of expertise in Linked List Assignments. Educated at the University of Warwick, UK, Hayden excels in data structures and algorithmic implementations.
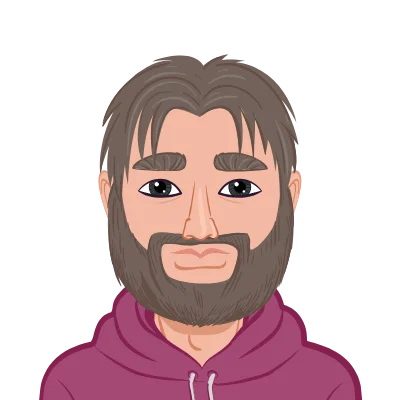
Charles Higgins
Ph.D. in Computer Science
🇺🇸 United States
Introducing Charles Higgins, a seasoned expert in Linked List Assignments with 13 years of experience. Charles earned his Ph.D. from Columbia University, specializing in advanced data structures and algorithmic analysis.
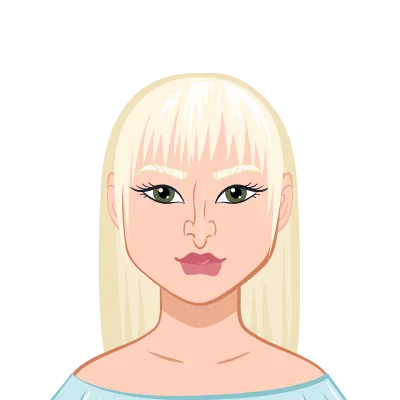
Maya Riley
Ph.D. in Computer Science
🇬🇧 United Kingdom
Maya Riley is an accomplished expert in Linked List Assignments with a Ph.D. from the University of Oxford, UK. With 15 years of experience, she excels in advanced data structures and algorithm implementations.
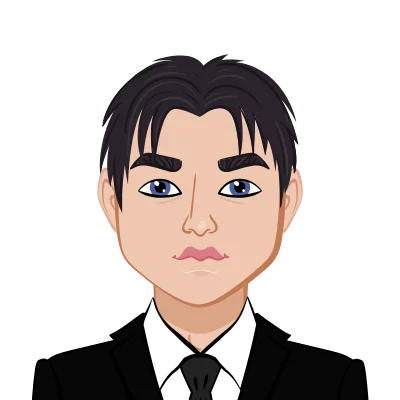
James Patterson
Ph.D. in Computer Science
🇨🇦 Canada
James Patterson, Ph.D., brings 18 years of expertise in Linked List Assignments. Educated at the University of Waterloo, Canada, he specializes in advanced data structures and algorithms.
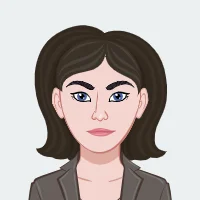
Linda Nguyen
Masters in Algorithm
🇨🇦 Canada
Linda Nguyen is a proficient Algorithm Assignment Help Expert boasting 10 years of expertise. She earned her Master's degree from the University of Toronto, Canada.
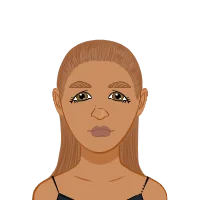
Dr. Rita T. Rose
PhD in Computer Science
🇺🇸 United States
Dr. Rita T. Rose holds a PhD in Computer Science from the University of Michigan - Ann Arbor, USA. With 7 years of experience, she has completed over 700 Design and Analysis of Algorithms assignments. Dr. Rose specializes in sorting algorithms and recursive algorithms, offering students structured guidance and practical insights to navigate through complex algorithmic challenges with confidence.
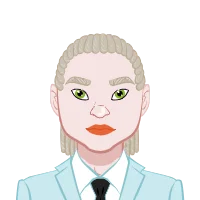
Dr. Terry N. Morris
PhD in Computer Science
🇨🇦 Canada
Dr. Terry N. Morris completed his PhD in Computer Science at the University of Toronto, Toronto, Canada. With 8 years of experience, he has successfully handled over 800 Design and Analysis of Algorithms assignments. Dr. Morris excels in dynamic programming and algorithm analysis, providing students with clear explanations and effective problem-solving strategies to excel in their studies.
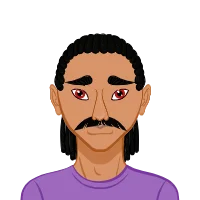
Dr. Jared J. Rooney
PhD in Computer Science
🇺🇸 United States
Dr. Jared J. Rooney earned his PhD in Computer Science from the University of Pennsylvania, Philadelphia, USA. With 9 years of experience, he has completed over 900 Design and Analysis of Algorithms assignments. Dr. Rooney specializes in algorithmic optimization and graph algorithms, offering students comprehensive assistance and strategic insights to enhance their understanding and academic performance.
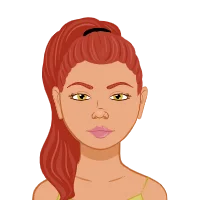
Dr. Marisol M. Brown
PhD in Computer Science
🇺🇸 United States
Dr. Marisol M. Brown holds a PhD in Computer Science from Columbia University, New York, USA. With over 10 years of experience, she has successfully completed over 1000 Design and Analysis of Algorithms assignments. Dr. Brown's expertise lies in advanced algorithm design and complexity theory, and she is committed to delivering meticulous guidance and insightful solutions to students seeking help with their assignments.
Related Topics
Frequently Asked Questions
Have questions about our queue implementation programming assignment help using nodes and pointers? Check out our FAQs section for answers to commonly asked questions. If you can't find the information you're looking for, don't hesitate to reach out to our 24/7 customer support team via live chat. We're here to address any concerns you may have and provide you with the assistance you need to succeed in your programming assignments.
Memory management in multi-level queue implementation requires careful consideration of resource allocation and deallocation. Implementing dynamic memory allocation techniques like malloc() and free() in conjunction with proper error handling can help prevent memory leaks and ensure efficient memory usage in multi-level queue systems.
Optimizing a priority queue implementation involves carefully selecting data structures and algorithms to minimize time complexity. Techniques such as heap-based priority queues or balanced binary search trees can improve insertion and deletion operations, ensuring efficient priority-based data management.
Circular queue implementation introduces complexities such as handling wrap-around logic and detecting queue full or empty conditions. Ensuring proper management of pointers to the front and rear of the queue is crucial to prevent errors like data overwriting or loss.
Thread safety in queue implementations can be achieved through synchronization mechanisms such as mutex locks or atomic operations. By controlling access to critical sections of code, we can prevent data corruption and race conditions when multiple threads concurrently enqueue or dequeue elements.
Queue implementation often relies on linked lists or arrays to store and manage data elements. Linked lists are preferred for their dynamic memory allocation, allowing for flexible queue sizes, while arrays offer contiguous memory allocation for efficient access to elements.